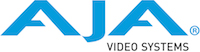 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
18 #define NTV2_AUDIOSIZE_MAX (401 * 1024)
25 const bool inQuadMode,
26 const uint32_t inAudioChannels,
27 const bool inTimeCodeBurn,
28 const bool inInfoMode,
30 const uint32_t inMaxFrames)
41 mDeviceSpecifier (inDeviceSpecifier),
42 mWithAudio (inAudioChannels != 0),
43 mInputChannel (inChannel),
48 mPixelFormat (inPixelFormat),
55 mWithInfo (inInfoMode),
56 mWithAnc (inTimeCodeBurn),
59 mNumAudioChannels (0),
60 mFileAudioChannels (inAudioChannels),
61 mMaxFrames (inMaxFrames),
63 mLastFrameInput (
false),
64 mLastFrameRaw (
false),
65 mLastFrameHevc (
false),
66 mLastFrameVideo (
false),
67 mLastFrameAudio (
false),
69 mVideoInputFrameCount (0),
70 mVideoProcessFrameCount (0),
71 mCodecRawFrameCount (0),
72 mCodecHevcFrameCount (0),
73 mVideoFileFrameCount (0),
74 mAudioFileFrameCount (0)
76 ::memset (mACInputBuffer, 0x0,
sizeof (mACInputBuffer));
77 ::memset (mVideoRawBuffer, 0x0,
sizeof (mVideoRawBuffer));
78 ::memset (mVideoHevcBuffer, 0x0,
sizeof (mVideoHevcBuffer));
79 ::memset (mAudioInputBuffer, 0x0,
sizeof (mAudioInputBuffer));
107 if (mACInputBuffer[bufferNdx].pVideoBuffer)
112 if (mACInputBuffer[bufferNdx].pInfoBuffer)
117 if (mACInputBuffer[bufferNdx].pAudioBuffer)
123 if (mVideoRawBuffer[bufferNdx].pVideoBuffer)
128 if (mVideoRawBuffer[bufferNdx].pInfoBuffer)
133 if (mVideoRawBuffer[bufferNdx].pAudioBuffer)
139 if (mVideoHevcBuffer[bufferNdx].pVideoBuffer)
144 if (mVideoHevcBuffer[bufferNdx].pInfoBuffer)
149 if (mVideoHevcBuffer[bufferNdx].pAudioBuffer)
160 if (mAudioInputBuffer[bufferNdx].pVideoBuffer)
165 if (mAudioInputBuffer[bufferNdx].pInfoBuffer)
167 delete [] mAudioInputBuffer[bufferNdx].
pInfoBuffer;
170 if (mAudioInputBuffer[bufferNdx].pAudioBuffer)
183 if (mM31 && !mLastFrame && !mGlobalQuit)
191 for (i = 0; i < timeout; i++)
193 if (mLastFrameVideo && (!mWithAudio || mLastFrameAudio))
break;
197 { cerr <<
"## ERROR: Wait for last frame timeout" << endl; }
201 { cerr <<
"## ERROR: ChangeEHState ready to stop failed" << endl; }
204 { cerr <<
"## ERROR: ChangeEHState stop failed" << endl; }
208 { cerr <<
"## ERROR: ChangeVInState stop failed" << endl; }
214 { cerr <<
"## ERROR: ChangeMainState to init failed" << endl; }
221 while (mACInputThread.
Active())
224 while (mVideoProcessThread.
Active())
227 while (mCodecRawThread.
Active())
230 while (mCodecHevcThread.
Active())
233 while (mVideoFileThread.
Active())
236 while (mAudioFileThread.
Active())
265 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return AJA_STATUS_OPEN; }
268 mM31 =
new CNTV2m31 (&mDevice);
281 mVideoFormat = CNTV2m31::GetPresetVideoFormat(mPreset);
282 mPixelFormat = CNTV2m31::GetPresetFrameBufferFormat(mPreset);
283 mVif = CNTV2m31::IsPresetVIF(mPreset);
284 mQuad = CNTV2m31::IsPresetUHD(mPreset);
285 mInterlaced = CNTV2m31::IsPresetInterlaced(mPreset);
307 switch (mInputChannel)
340 if(!CNTV2m31::ConvertVideoFormatToPreset(mVideoFormat, mPixelFormat, mVif, mPreset))
343 mQuad = CNTV2m31::IsPresetUHD(mPreset);
344 mInterlaced = CNTV2m31::IsPresetInterlaced(mPreset);
367 status = mHevcCommon->
SetupHEVC (mM31, mPreset, mEncodeChannel, mMultiStream, mWithInfo);
377 if (mMultiStream) oss <<
"raw_" << (mInputChannel+1) <<
".hevc";
else oss <<
"raw.hevc";
387 if (mMultiStream) oss <<
"raw_" << (mInputChannel+1) <<
".txt";
else oss <<
"raw.txt";
397 if (mMultiStream) oss <<
"raw_" << (mInputChannel+1) <<
".aiff";
else oss <<
"raw.aiff";
423 if (mDevice.
features().CanDoMultiFormat())
476 else if (mMultiStream)
479 if (mDevice.
features().CanDoMultiFormat())
483 mDevice.
SetVideoFormat (mVideoFormat,
false,
false, mInputChannel);
503 if (mDevice.
features().CanDoMultiFormat())
562 if (mMultiStream && mDevice.
features().GetNumAudioSystems() > 1 &&
UWord(mInputChannel) < mDevice.
features().GetNumAudioSystems())
568 mNumAudioChannels = mDevice.
features().GetMaxAudioChannels();
590 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
593 mACInputBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
597 mACInputBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
601 mACInputCircularBuffer.
Add (& mACInputBuffer[bufferNdx]);
606 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
609 mVideoRawBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
613 mVideoRawBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
617 mVideoRawCircularBuffer.
Add (& mVideoRawBuffer[bufferNdx]);
622 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
625 mVideoHevcBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
629 mVideoHevcBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mEncInfoBufferSize/4];
633 mVideoHevcCircularBuffer.
Add (& mVideoHevcBuffer[bufferNdx]);
640 for (
unsigned bufferNdx = 0; bufferNdx <
AUDIO_RING_SIZE; bufferNdx++ )
643 mAudioInputBuffer[bufferNdx].
pAudioBuffer =
new uint32_t [mAudioBufferSize/4];
646 mAudioInputCircularBuffer.
Add (& mAudioInputBuffer[bufferNdx]);
775 cout << endl <<
"## WARNING: No video signal present on the input connector" << endl;
798 mACInputThread.
Start();
821 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
862 if (mWithAudio && pAudioData)
893 pPicData->ptsValueLow = (uint32_t)(pts & 0xffffffff);
894 pPicData->ptsValueHigh = (uint32_t)((pts >> 32) & 0x1);
895 pPicData->pictureNumber = mVideoInputFrameCount + 1;
902 pPicData->serialNumber = mVideoInputFrameCount*2;
903 pPicData->pictureNumber = mVideoInputFrameCount*2 + 1;
913 pPicData->serialNumber = mVideoInputFrameCount*2 + 1;
914 pPicData->ptsValueLow = (uint32_t)(pts & 0xffffffff);
915 pPicData->ptsValueHigh = (uint32_t)((pts >> 32) & 0x1);
916 pPicData->pictureNumber = mVideoInputFrameCount*2 + 2;
923 if(pVideoData->
lastFrame && !mLastFrameInput)
925 printf (
"\nCapture last frame number %d\n", mVideoInputFrameCount );
926 mLastFrameInput =
true;
929 mVideoInputFrameCount++;
931 if (mWithAudio && pAudioData)
961 mVideoProcessThread.
Start();
992 mVideoProcessFrameCount++;
1012 mCodecRawThread.
Start();
1036 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
1039 m31 =
new CNTV2m31 (&ntv2Device);
1041 while (!mGlobalQuit)
1055 if (mCodecRawFrameCount == 3)
1068 m31->RawTransfer(mPreset, mEncodeChannel,
1075 m31->RawTransfer(mPreset, mEncodeChannel,
1084 m31->RawTransfer(mPreset, mEncodeChannel,
1089 m31->RawTransfer(mPreset, mEncodeChannel,
1099 m31->RawTransfer(mEncodeChannel,
1108 m31->RawTransfer(mEncodeChannel,
1117 mLastFrameRaw =
true;
1120 mCodecRawFrameCount++;
1144 mCodecHevcThread.
Start();
1167 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
1170 m31 =
new CNTV2m31 (&ntv2Device);
1172 while (!mGlobalQuit)
1178 if (!mLastFrameHevc)
1183 uint8_t* pVideoBuffer = (uint8_t*)pFrameData->
pVideoBuffer;
1184 uint8_t* pInfoBuffer = (uint8_t*)pFrameData->
pInfoBuffer;
1189 m31->EncTransfer(mEncodeChannel,
1216 m31->EncTransfer(mEncodeChannel,
1239 m31->EncTransfer(mEncodeChannel,
1262 mLastFrameHevc =
true;
1265 mCodecHevcFrameCount++;
1282 mVideoFileThread.
Start();
1300 while (!mGlobalQuit)
1306 if (!mLastFrameVideo)
1321 printf (
"Video file last frame number %d\n", mVideoFileFrameCount );
1322 mLastFrameVideo =
true;
1325 mVideoFileFrameCount++;
1341 mAudioFileThread.
Start();
1359 while (!mGlobalQuit)
1365 if (!mLastFrameAudio)
1372 printf (
"Audio file last frame number %d\n", mAudioFileFrameCount );
1373 mLastFrameAudio =
true;
1377 mAudioFileFrameCount++;
1419 std::string timeString;
1421 mTimeCode.
Set(frameNumber);
1423 mTimeCode.
QueryString(timeString, mTimeBase,
false);
1426 mTimeCode.
QueryString(timeString, mTimeBase,
false);
@ NTV2_XptFrameBuffer6YUV
void WriteAiffData(void *pBuffer, uint32_t numChannels, uint32_t numSamples)
virtual bool SetSDIInLevelBtoLevelAConversion(const NTV2ChannelSet &inSDIInputs, const bool inEnable)
Enables or disables 3G level B to 3G level A conversion at the SDI input(s).
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
static void VideoFileThreadStatic(AJAThread *pThread, void *pContext)
This is the video file writer thread's static callback function that gets called when the thread star...
@ NTV2_CHANNEL8
Specifies channel or FrameStore 8 (or the 8th item).
AJA_PixelFormat GetAJAPixelFormat(NTV2FrameBufferFormat pixelFormat)
uint32_t timeCodeLow
Time code data low.
virtual void VideoInputWorker(void)
Repeatedly captures video frames using AutoCirculate and add them to the video input ring.
@ NTV2_CHANNEL2
Specifies channel or FrameStore 2 (or the 2nd item).
@ AJA_ThreadPriority_High
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
virtual bool Set4kSquaresEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 "2K quadrants" mode for the given FrameStore bank on the device....
virtual bool SetEmbeddedAudioClock(const NTV2EmbeddedAudioClock inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2EmbeddedAudioClock setting for the given NTV2AudioSystem.
Declares device capability functions.
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
uint32_t videoDataSize2
Size of field 2 video data (bytes)
virtual void StartVideoFileThread(void)
Start the video file writer thread.
uint32_t audioDataSize
Size of audio data (bytes)
virtual AJAStatus ProcessVideoFrame(AVHevcDataBuffer *pSrcFrame, AVHevcDataBuffer *pDstFrame, uint32_t frameNumber)
Default do-nothing function for processing the captured frames.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
void WriteHevcData(void *pBuffer, uint32_t bufferSize)
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
#define NTV2_AUDIOSIZE_MAX
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
bool lastFrame
Indicates last captured frame.
Declares the AJATime class.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
@ NTV2_XptFrameBuffer8YUV
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
ULWord GetProcessedFrameCount(void) const
static void CodecRawThreadStatic(AJAThread *pThread, void *pContext)
This is the codec raw thread's static callback function that gets called when the thread starts....
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
uint32_t * pVideoBuffer
Pointer to host video buffer.
virtual void StartAudioFileThread(void)
Start the audio file writer thread.
void WriteEncData(void *pBuffer, uint32_t bufferSize)
ULWord GetBufferLevel(void) const
bool GetInputTimeCode(NTV2_RP188 &outTimeCode, const NTV2TCIndex inTCIndex=NTV2_TCINDEX_SDI1) const
Intended for capture, answers with a specific timecode captured in my acTransferStatus member's acFra...
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual void CodecHevcWorker(void)
Repeatedly transfers hevc frames from the codec and adds them to the hevc ring.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
static void VideoInputThreadStatic(AJAThread *pThread, void *pContext)
This is the video input thread's static callback function that gets called when the thread starts....
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=(0))
Applies the given routing table to the AJA device.
@ NTV2_XptFrameBuffer4Input
AJAStatus DetermineInputFormat(NTV2VideoFormat sdiFormat, bool quad, NTV2VideoFormat &videoFormat)
@ NTV2_XptFrameBuffer3Input
static void VideoProcessThreadStatic(AJAThread *pThread, void *pContext)
This is the video process thread's static callback function that gets called when the thread starts....
uint32_t infoDataSize
Size of the information data (bytes)
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
double GetFramesPerSecond(const NTV2FrameRate inFrameRate)
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
@ NTV2_CHANNEL6
Specifies channel or FrameStore 6 (or the 6th item).
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
ULWord GetDisplayHeight(const NTV2VideoFormat videoFormat)
uint32_t * pInfoBuffer
Picture information (raw) or encode information (hevc)
uint32_t infoDataSize2
Size of the field 2 information data (bytes)
@ NTV2_CHANNEL4
Specifies channel or FrameStore 4 (or the 4th item).
@ NTV2_CHANNEL5
Specifies channel or FrameStore 5 (or the 5th item).
virtual class DeviceCapabilities & features(void)
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
virtual void VideoProcessWorker(void)
Repeatedly removes video frames from the video input ring, calls a custom video process method and ad...
uint32_t videoDataSize
Size of video data (bytes)
void SetAJAFrameRate(AJA_FrameRate ajaFrameRate)
ULWord fLo
| BG 4 | Secs10 | BG 3 | Secs 1 | BG 2 | Frms10 | BG 1 | Frms 1 |
Declares the AJAProcess class.
ULWord fHi
| BG 8 | Hrs 10 | BG 7 | Hrs 1 | BG 6 | Mins10 | BG 5 | Mins 1 |
@ NTV2_XptFrameBuffer1Input
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
static void CodecHevcThreadStatic(AJAThread *pThread, void *pContext)
This is the codec hevc thread's static callback function that gets called when the thread starts....
NTV2EmbeddedAudioInput NTV2ChannelToEmbeddedAudioInput(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2EmbeddedAudioInput.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual void StartCodecRawThread(void)
Start the codec raw thread.
static void AudioFileThreadStatic(AJAThread *pThread, void *pContext)
This is the audio file writer thread's static callback function that gets called when the thread star...
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
@ NTV2_CHANNEL7
Specifies channel or FrameStore 7 (or the 7th item).
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=(!(0)))
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
virtual void StartVideoInputThread(void)
Start the video input thread.
void SetRP188(const uint32_t inDBB, const uint32_t inLo, const uint32_t inHi, const AJATimeBase &inTimeBase)
@ NTV2_MAX_NUM_VIDEO_FORMATS
virtual void AudioFileWorker(void)
Repeatedly removes audio samples from the audio input ring and writes them to the audio output file.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
NTV2EncodeHEVC(const std::string inDeviceSpecifier="0", const NTV2Channel inChannel=NTV2_CHANNEL1, const M31VideoPreset inM31Preset=M31_FILE_1280X720_420_8_5994p, const NTV2FrameBufferFormat inPixelFormat=NTV2_FBF_10BIT_YCBCR_420PL2, const bool inQuadMode=(0), const uint32_t inAudioChannels=0, const bool inTimeCodeBurn=(0), const bool inInfoMode=(0), const bool inTsiMode=(0), const uint32_t inMaxFrames=0xffffffff)
Constructs me using the given settings.
@ NTV2_CHANNEL3
Specifies channel or FrameStore 3 (or the 3rd item).
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
@ NTV2_EMBEDDED_AUDIO_CLOCK_VIDEO_INPUT
Audio clock derived from the video input.
Declares numerous NTV2 utility functions.
virtual AJAStatus Run(void)
Runs me.
uint32_t * pAudioBuffer
Pointer to host audio buffer.
void SetStdTimecodeForHfr(bool bStdTc)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
I interrogate and control an AJA video/audio capture/playout device.
@ NTV2_XptFrameBuffer1DS2Input
struct HevcPictureInfo HevcPictureInfo
ULWord GetVideoActiveSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
AJAStatus CreateEncFile(const std::string &inFileName, uint32_t maxFrames)
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
virtual bool SetTsiFrameEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 two-sample interleave (Tsi) frame mode on the device.
struct HevcEncodedInfo HevcEncodedInfo
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
This class is a collection of widget input-to-output connections that can be applied all-at-once to a...
@ NTV2_STANDARD_TASKS
1: Standard/Retail: device configured by AJA ControlPanel, service/daemon, and driver.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual ~NTV2EncodeHEVC()
virtual void RouteInputSignal(void)
Sets up device routing for capture.
AUTOCIRCULATE_TRANSFER_STATUS acTransferStatus
Contains status information that's valid after CNTV2Card::AutoCirculateTransfer returns,...
uint32_t timeCodeDBB
Time code data dbb.
struct HevcPictureData HevcPictureData
@ NTV2_XptFrameBuffer2Input
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
bool IsRunning(void) const
@ NTV2_MODE_CAPTURE
Capture (input) mode, which writes into device SDRAM.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
This struct replaces the old RP188_STRUCT.
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
@ NTV2_XptFrameBuffer7YUV
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
ULWord GetDroppedFrameCount(void) const
int64_t FramesToMicroseconds(int64_t frames, bool round=false) const
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
AJAStatus CreateAiffFile(const std::string &inFileName, uint32_t numChannels, uint32_t maxFrames, uint32_t bufferSize)
@ Hevc_EhState_ReadyToStop
virtual void Quit(void)
Gracefully stops me from running.
HevcPictureData pictureData
ULWord GetDisplayWidth(const NTV2VideoFormat videoFormat)
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual void GetStatus(AVHevcStatus &outStatus)
Provides status information about my input (capture) process.
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
AJAStatus CreateHevcFile(const std::string &inFileName, uint32_t maxFrames)
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
uint32_t videoBufferSize
Size of host video buffer (bytes)
void QueryString(std::string &str, const AJATimeBase &timeBase, bool bDropFrame, bool bStdTcForHfr, AJATimecodeNotation notation=AJA_TIMECODE_LEGACY)
uint32_t timeCodeHigh
Time code data high.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
uint32_t infoBufferSize
Size of the host information buffer (bytes)
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
ULWord acAudioTransferSize
Number of bytes captured into the audio buffer.
uint32_t AlignDataBuffer(void *pBuffer, uint32_t bufferSize, uint32_t dataSize, uint32_t alignBytes, uint8_t fill)
virtual void StartCodecHevcThread(void)
Start the codec hevc thread.
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
virtual AJAStatus Start()
AJA_FrameRate GetAJAFrameRate(NTV2FrameRate frameRate)
virtual NTV2VideoFormat GetSDIInputVideoFormat(NTV2Channel inChannel, bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given SDI input source.
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
This structure encapsulates the video and audio buffers used by the HEVC demo applications....
#define AJA_FAILURE(_status_)
@ NTV2_XptFrameBuffer5YUV
virtual void SetupAutoCirculate(void)
Initializes AutoCirculate.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
@ NTV2_XptFrameBuffer2DS2Input
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
virtual bool AddConnection(const NTV2InputXptID inSignalInput, const NTV2OutputXptID inSignalOutput=NTV2_XptBlack)
Adds a connection between a widget's signal input (sink) and another widget's signal output (source).
Declares the NTV2EncodeHEVC class.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
virtual bool GetSDIInput3GbPresent(bool &outValue, const NTV2Channel channel)
virtual void StartVideoProcessThread(void)
Start the video process thread.
bool SetBuffers(ULWord *pInVideoBuffer, const ULWord inVideoByteCount, ULWord *pInAudioBuffer, const ULWord inAudioByteCount, ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
uint32_t audioBufferSize
Size of host audio buffer (bytes)
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
virtual void VideoFileWorker(void)
Repeatedly removes hevc frame from the hevc ring and writes them to the hevc output file.
@ NTV2_OEM_TASKS
2: OEM (recommended): device configured by client application(s) with some driver involvement.
AJAStatus SetupHEVC(CNTV2m31 *pM31, M31VideoPreset preset, M31Channel encodeChannel, bool multiStream, bool withInfo)
virtual void CodecRawWorker(void)
Repeatedly removes video frames from the raw video ring and transfers them to the codec.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID