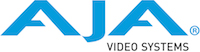 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
28 cout <<
"Device " <<
DEC(inCard.GetIndexNumber()+1) <<
":" << endl
33 <<
"\t" <<
"Device ID: " <<
xHEX0N(deviceID,8) << endl
34 <<
"\t" <<
"Serial Number: '" << (inCard.
GetSerialNumberString(serial) ? serial : serial) <<
"'" << endl
37 <<
"\t" << inCard.
features().GetNumVideoInputs() <<
" SDI Input(s)" << endl
38 <<
"\t" << inCard.
features().GetNumVideoOutputs() <<
" SDI Output(s)" << endl
39 <<
"\t" << inCard.
features().GetNumHDMIVideoInputs() <<
" HDMI Input(s)" << endl
40 <<
"\t" << inCard.
features().GetNumHDMIVideoOutputs() <<
" HDMI Output(s)" << endl
41 <<
"\t" << inCard.
features().GetNumAnalogVideoInputs() <<
" Analog Input(s)" << endl
42 <<
"\t" << inCard.
features().GetNumAnalogVideoOutputs() <<
" Analog Output(s)" << endl
43 <<
"\t" << inCard.
features().GetNumEmbeddedAudioInputChannels() <<
" channel(s) of Embedded Audio Input" << endl
44 <<
"\t" << inCard.
features().GetNumEmbeddedAudioOutputChannels() <<
" channel(s) of Embedded Audio Output" << endl;
49 cout <<
"\t" << videoFormats << endl
50 <<
"\t" << pixelFormats << endl;
57 int main (
int argc,
const char ** argv)
67 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
73 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
75 {cerr <<
"## ERROR: Unexpected argument(s): " << popt.
otherArgs() << endl;
return 2;}
77 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
81 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"");
82 if (!deviceSpec.empty())
89 {cerr <<
"## ERROR: Failed to open '" << deviceSpec <<
"'";
return 2;}
99 cout << endl << endl << endl;
104 cout <<
"No AJA devices found" << endl;
106 return deviceCount ? 0 : 1;
virtual const NTV2StringList & otherArgs(void) const
NTV2FrameBufferFormatSet NTV2PixelFormats
NTV2DeviceIDSet NTV2GetSupportedDevices(const NTV2DeviceKinds inKinds=NTV2_DEVICEKIND_ALL)
Returns an NTV2DeviceIDSet of devices supported by the SDK.
std::set< NTV2VideoFormat > NTV2VideoFormatSet
A set of distinct NTV2VideoFormat values.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
NTV2VideoFormatSet VideoFormats(void)
static int ShowDeviceInfo(CNTV2Card &inCard)
Prints a variety of information about the given device.
int main(int argc, const char **argv)
NTV2PixelFormats PixelFormats(void)
virtual class DeviceCapabilities & features(void)
virtual std::string GetDisplayName(void)
Answers with this device's display name.
static bool IsValidDevice(const std::string &inDeviceSpec)
virtual std::string GetDescription(void) const
Declares the CNTV2DeviceScanner class.
virtual bool GetSerialNumberString(std::string &outSerialNumberString)
Answers with a string that contains my human-readable serial number.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
I interrogate and control an AJA video/audio capture/playout device.
static AJAStatus Open(bool incrementRefCount=false)
This file contains some structures, constants, classes and functions that are used in some of the dem...
static bool GetDeviceAtIndex(const ULWord inDeviceIndexNumber, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device having the given zero-bas...
virtual NTV2DeviceID GetDeviceID(void)
#define xHEX0N(__x__, __n__)