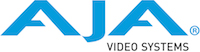 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
30 int main (
int argc,
const char ** argv)
39 int doMultiFormat (0);
52 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
54 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
55 {
"input",
'i',
POPT_ARG_STRING, &pInputSrcSpec, 0,
"input to use",
"1-8, ?=list" },
56 {
"output",
'o',
POPT_ARG_STRING, &pOutputDest, 0,
"output to use",
"'?' or 'list' to list" },
62 {
"iframes", 0,
POPT_ARG_STRING, &pInFramesSpec, 0,
"input AutoCirc frames",
"num[@min] or min-max" },
63 {
"oframes", 0,
POPT_ARG_STRING, &pOutFramesSpec,0,
"output AutoCirc frames",
"num[@min] or min-max" },
64 {
"tcsource",
't',
POPT_ARG_STRING, &pTcSource, 0,
"time code source",
"'?' to list" },
70 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
72 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
75 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
85 if (inputSourceStr ==
"?" || inputSourceStr ==
"list")
86 {cout << legalSources << endl;
return 0;}
88 {cerr <<
"## ERROR: Input source '" << inputSourceStr <<
"' not one of:" << endl << legalSources << endl;
return 1;}
94 if (outputDestStr ==
"?" || outputDestStr ==
"list")
95 {cout << legalOutputs << endl;
return 0;}
97 {cerr <<
"## ERROR: Output '" << outputDestStr <<
"' not of:" << endl << legalOutputs << endl;
return 1;}
100 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
102 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
106 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
115 if (tcSourceStr ==
"?" || tcSourceStr ==
"list")
116 {cout << legalTCSources << endl;
return 0;}
118 {cerr <<
"## ERROR: Timecode source '" << tcSourceStr <<
"' not one of these:" << endl << legalTCSources << endl;
return 1;}
121 string framesSpec(pInFramesSpec ? pInFramesSpec :
"");
122 static const string legalFrmSpec(
"{frameCount}[@{firstFrameNum}] or {firstFrameNum}-{lastFrameNum}");
123 if (!framesSpec.empty())
125 if (!parseResult.empty())
126 {cerr <<
"## ERROR: Bad '--iframes' spec '" << framesSpec <<
"'" << endl <<
"## " << parseResult << endl;
return 1;}
129 {cerr <<
"## ERROR: Bad '--iframes' spec '" << framesSpec <<
"'" << endl <<
"## Expected " << legalFrmSpec << endl;
return 1;}
132 framesSpec = pOutFramesSpec ? pOutFramesSpec :
"";
133 if (!framesSpec.empty())
135 if (!parseResult.empty())
136 {cerr <<
"## ERROR: Bad '--oframes' spec '" << framesSpec <<
"'" << endl <<
"## " << parseResult << endl;
return 1;}
139 {cerr <<
"## ERROR: Bad '--oframes' spec '" << framesSpec <<
"'" << endl <<
"## Expected " << legalFrmSpec << endl;
return 1;}
141 if (noVideo && noAudio)
142 {cerr <<
"## ERROR: '--novideo' and '--noaudio' cannot both be specified" << endl;
return 1;}
150 {cerr <<
"## ERROR: '--noanc' and '--vanc' are contradictory" << endl;
return 1;}
152 {cerr <<
"## ERROR: '--noanc' and '--hanc' are contradictory" << endl;
return 1;}
160 {cerr <<
"## ERROR: Initialization failed, status=" << status << endl;
return 4;}
172 cout <<
" Capture Playout Capture Playout" << endl
173 <<
" Frames Frames Frames Buffer Buffer" << endl
174 <<
"Processed Dropped Dropped Level Level" << endl;
178 burner.
GetStatus (inputStatus, outputStatus);
bool fVerbose
If true, emit explanatory messages to stdout/stderr. Defaults to false.
static bool gGlobalQuit((0))
Set this "true" to exit gracefully.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
static NTV2PixelFormat GetPixelFormatFromString(const std::string &inStr, const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2PixelFormat that matches the given string.
NTV2OutputDest fOutputDest
The device output connector to use (NTV2_OUTPUTDESTINATION_INVALID means unspecified)
ULWord GetProcessedFrameCount(void) const
bool fWithTallFrames
If true && fWithAnc, use "taller" VANC mode for anc. Defaults to false.
#define NTV2_IS_VALID_TIMECODE_INDEX(__x__)
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
bool fSuppressAudio
If true, suppress audio; otherwise include audio.
NTV2TCIndex fTimecodeSource
Timecode source to use.
ULWord GetBufferLevel(void) const
int main(int argc, const char **argv)
#define NTV2_IS_VALID_OUTPUT_DEST(_dest_)
bool fSuppressVideo
If true, suppress video; otherwise include video.
std::string setFromString(const std::string &inStr)
static std::string GetOutputDestinationStrings(const NTV2IOKinds inKinds, const std::string inDevSpec=std::string())
NTV2PixelFormat fPixelFormat
The pixel format to use.
virtual void GetStatus(AUTOCIRCULATE_STATUS &outInputStatus, AUTOCIRCULATE_STATUS &outOutputStatus)
Provides status information about my input (capture) and output (playout) processes.
NTV2ACFrameRange fOutputFrames
Playout frame count or range.
static bool IsValidDevice(const std::string &inDeviceSpec)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
bool fWithHanc
If true, capture & play HANC data, including audio (LLBurn). Defaults to false.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
static NTV2OutputDestination GetOutputDestinationFromString(const std::string &inStr, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2OutputDestination that matches the given string.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
static std::string GetTCIndexStrings(const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDeviceSpecifier=std::string(), const bool inIsInputOnly=(!(0)))
bool fWithAnc
If true, capture & play anc data (LLBurn). Defaults to false.
static AJAStatus Open(bool incrementRefCount=false)
static void SignalHandler(int inSignal)
NTV2InputSource fInputSource
The device input connector to use.
I capture frames from a video signal provided to an AJA device's video input. I burn timecode into th...
static std::string GetInputSourceStrings(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
@ NTV2_IOKINDS_ALL
Specifies any/all input/output kinds.
Header file for the NTV2Burn demonstration class.
ULWord GetDroppedFrameCount(void) const
virtual AJAStatus Run(void)
Runs me.
static NTV2TCIndex GetTCIndexFromString(const std::string &inStr, const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDevSpec=std::string())
Returns the NTV2TCIndex that matches the given string.
static NTV2InputSource GetInputSourceFromString(const std::string &inStr, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2InputSource that matches the given string.
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
NTV2ACFrameRange fInputFrames
Ingest frame count or range.
Configures an NTV2Burn or NTV2FieldBurn instance.