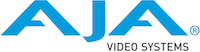 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
13 #include <ajabase/network/network.h>
16 #if defined (AJALinux) || defined (AJAMac)
41 return !(*
this == other);
90 return !(*
this == other);
112 (ssrc == other.
ssrc) &&
141 return (!(*
this == other));
150 (mbps == other.
mbps) &&
153 (pmtPid == other.
pmtPid) &&
155 (pcrPid == other.
pcrPid) &&
176 return (!(*
this == other));
196 numAvailablePrograms = 0;
197 numAvailableAudios = 0;
198 availableProgramNumbers.clear();
199 availableProgramPIDs.clear();
200 availableAudioPIDs.clear();
218 _numRxChans = _numRx0Chans + _numRx1Chans;
219 _numTxChans = _numTx0Chans + _numTx1Chans;
226 _biDirectionalChannels =
false;
228 _tstreamConfig =
NULL;
241 delete _tstreamConfig;
247 string ip, subnet, gateway;
249 addr.s_addr = (uint32_t)netConfig.
ipc_ip;
250 ip = AJANetwork::aja_inet_ntoa(addr);
252 subnet = AJANetwork::aja_inet_ntoa(addr);
254 gateway = AJANetwork::aja_inet_ntoa(addr);
262 string ip, subnet, gateway;
286 uint32_t addr = AJANetwork::aja_inet_addr(localIPAddress.c_str());
295 macAddressRegister++;
298 uint32_t boardHi = (macHi & 0xffff0000) >>16;
299 uint32_t boardLo = ((macHi & 0x0000ffff) << 16) + ((macLo & 0xffff0000) >> 16);
302 macAddressRegister++;
304 macAddressRegister++;
307 uint32_t boardHi2 = (macHi & 0xffff0000) >>16;
308 uint32_t boardLo2 = ((macHi & 0x0000ffff) << 16) + ((macLo & 0xffff0000) >> 16);
364 localIPAddress = AJANetwork::aja_inet_ntoa(addr);
368 subnetMask = AJANetwork::aja_inet_ntoa(addr);
372 gateway = AJANetwork::aja_inet_ntoa(addr);
379 localIPAddress = AJANetwork::aja_inet_ntoa(addr);
383 subnetMask = AJANetwork::aja_inet_ntoa(addr);
387 gateway = AJANetwork::aja_inet_ntoa(addr);
403 bool enabled_7 =
false;
430 rv = SelectRxChannel(channel,
SFP_2, baseAddr);
431 if (!rv)
return false;
439 uint32_t sourceIp = AJANetwork::aja_inet_addr(rxConfig.
sfp2SourceIP.c_str());
444 uint32_t destIp = AJANetwork::aja_inet_addr(rxConfig.
sfp2DestIP.c_str());
464 uint8_t ip0 = (destIp & 0xff000000)>> 24;
465 if ((ip0 >= 224 && ip0 <= 239) && sfp2)
468 bool enabled =
false;
488 rv = SelectRxChannel(channel,
SFP_1, baseAddr);
489 if (!rv)
return false;
497 uint32_t sourceIp = AJANetwork::aja_inet_addr(rxConfig.
sfp1SourceIP.c_str());
502 uint32_t destIp = AJANetwork::aja_inet_addr(rxConfig.
sfp1DestIP.c_str());
527 if (_is2022_2 || (enabled_7 ==
false))
553 uint32_t pllMatch = 0;
564 uint8_t ip0 = (destIp & 0xff000000)>> 24;
565 if ((ip0 >= 224 && ip0 <= 239) && sfp1)
568 bool enabled =
false;
595 rv = SelectRxChannel(channel,
SFP_2, baseAddr);
596 if (!rv)
return false;
602 char * ip = AJANetwork::aja_inet_ntoa(in);
608 ip = AJANetwork::aja_inet_ntoa(in);
631 rv = SelectRxChannel(channel,
SFP_1, baseAddr);
632 if (!rv)
return false;
638 char * ip = AJANetwork::aja_inet_ntoa(in);
644 ip = AJANetwork::aja_inet_ntoa(in);
665 rxConfig.
playoutDelay = (_is2022_2) ? (val >>9)/90 : val/27000;
681 if (enable && sfp1Enable)
690 if (enable && sfp2Enable)
699 if (enable && _biDirectionalChannels)
739 rv = SelectRxChannel(channel,
SFP_1, baseAddr);
740 if (!rv)
return false;
741 if (enable & sfp1Enable)
753 rv = SelectRxChannel(channel,
SFP_2, baseAddr);
754 if (!rv)
return false;
755 if (enable & sfp2Enable)
768 rv = SelectRxChannel(channel,
SFP_1, baseAddr);
792 bool rv = SelectRxChannel(channel,
SFP_1, baseAddr);
793 if (!rv)
return false;
797 if (!rv)
return false;
805 rv = SelectRxChannel(channel,
SFP_2, baseAddr);
806 if (!rv)
return false;
809 if (!rv)
return false;
821 hi = macaddr.
mac[0] << 8;
822 hi += macaddr.
mac[1];
824 lo = macaddr.
mac[2] << 24;
825 lo += macaddr.
mac[3] << 16;
826 lo += macaddr.
mac[4] << 8;
827 lo += macaddr.
mac[5];
857 rv = SelectTxChannel(channel,
SFP_2, baseAddr);
858 if (!rv)
return false;
864 uint32_t val = (txConfig.
tos << 8) | txConfig.
ttl;
878 destIp = AJANetwork::aja_inet_addr(txConfig.
sfp2RemoteIP.c_str());
892 if (!rv)
return false;
908 rv = SelectTxChannel(channel,
SFP_1, baseAddr);
909 if (!rv)
return false;
915 uint32_t val = (txConfig.
tos << 8) | txConfig.
ttl;
928 destIp = AJANetwork::aja_inet_addr(txConfig.
sfp1RemoteIP.c_str());
941 if (!rv)
return false;
960 rv = SelectTxChannel(channel,
SFP_2, baseAddr);
961 if (!rv)
return false;
967 txConfig.
ttl = val & 0xff;
968 txConfig.
tos = (val & 0xff00) >> 8;
975 char * ip = AJANetwork::aja_inet_ntoa(in);
989 rv = SelectTxChannel(channel,
SFP_1, baseAddr);
990 if (!rv)
return false;
996 char * ip = AJANetwork::aja_inet_ntoa(in);
1018 if (enable && sfp1Enable)
1027 if (enable && sfp2Enable)
1036 if (_biDirectionalChannels)
1049 rv = SelectTxChannel(channel,
SFP_1, baseAddr);
1050 if (!rv)
return false;
1054 rv = SelectTxChannel(channel,
SFP_1, baseAddr);
1055 if (!rv)
return false;
1065 if (enable && sfp1Enable)
1067 if (GetTxLink(channel) ==
SFP_1)
1090 rv = SelectTxChannel(channel,
SFP_2, baseAddr);
1091 if (!rv)
return false;
1098 if (enable && sfp2Enable)
1118 SelectTxChannel(channel,
SFP_1, baseAddr);
1133 rv = SelectTxChannel(channel,
SFP_1, baseAddr);
1134 if (!rv)
return false;
1159 bool old_enable =
false;
1160 uint32_t unused = 0;
1163 bool enableChange = (old_enable != enable);
1179 uint32_t delay = rx_networkPathDifferential * 27000;
1189 if (_numTxChans && enableChange)
1208 macAddressRegister++;
1211 uint32_t boardHi = (macHi & 0xffff0000) >>16;
1212 uint32_t boardLo = ((macHi & 0x0000ffff) << 16) + ((macLo & 0xffff0000) >> 16);
1215 macAddressRegister++;
1217 macAddressRegister++;
1220 uint32_t boardHi2 = (macHi & 0xffff0000) >>16;
1221 uint32_t boardLo2 = ((macHi & 0x0000ffff) << 16) + ((macLo & 0xffff0000) >> 16);
1243 rx_networkPathDifferential = 0;
1260 rx_networkPathDifferential = val/27000;
1267 uint32_t val = (disable) ? 1 : 0;
1291 disabled = (val == 1) ?
true :
false;
1393 if ((uint32_t)chan >= _numTx0Chans)
1404 if ((uint32_t)chan >= _numTx0Chans)
1416 bool CNTV2Config2022::SelectRxChannel(
NTV2Channel channel,
eSFP sfp, uint32_t & baseAddr)
1418 uint32_t iChannel = (uint32_t) channel;
1419 uint32_t channelIndex = iChannel;
1421 if (iChannel > _numRxChans)
1426 if (iChannel >= _numRx0Chans)
1428 channelIndex = iChannel - _numRx0Chans;
1433 channelIndex = iChannel;
1439 if (iChannel >= _numRx0Chans)
1441 channelIndex = iChannel - _numRx0Chans;
1446 channelIndex = iChannel;
1452 channelIndex |= 0x80000000;
1460 bool CNTV2Config2022::SelectTxChannel(
NTV2Channel channel,
eSFP sfp, uint32_t & baseAddr)
1462 uint32_t iChannel = (uint32_t) channel;
1463 uint32_t channelIndex = iChannel;
1465 if (iChannel > _numTxChans)
1470 if (iChannel >= _numTx0Chans)
1472 channelIndex = iChannel - _numTx0Chans;
1477 channelIndex = iChannel;
1483 if (iChannel >= _numTx0Chans)
1485 channelIndex = iChannel - _numTx0Chans;
1490 channelIndex = iChannel;
1496 channelIndex |= 0x80000000;
1516 void CNTV2Config2022::ChannelSemaphoreSet(uint32_t controlReg, uint32_t baseAddr)
1523 void CNTV2Config2022::ChannelSemaphoreClear(uint32_t controlReg, uint32_t baseAddr)
1533 uint32_t destIp = AJANetwork::aja_inet_addr(remoteIP.c_str());
1540 uint8_t ip0 = (destIp & 0xff000000)>> 24;
1541 if (ip0 >= 224 && ip0 <= 239)
1544 mac = destIp & 0x7fffff;
1546 macaddr.
mac[0] = 0x01;
1547 macaddr.
mac[1] = 0x00;
1548 macaddr.
mac[2] = 0x5e;
1549 macaddr.
mac[3] = mac >> 16;
1550 macaddr.
mac[4] = (mac & 0xffff) >> 8;
1551 macaddr.
mac[5] = mac & 0xff;
1563 struct in_addr addr;
1565 string gateIp = AJANetwork::aja_inet_ntoa(addr);
1579 istringstream ss(macAddr);
1584 getline (ss, token,
':');
1585 macaddr.
mac[i++] = (uint8_t)strtoul(token.c_str(),
NULL,16);
1626 SelectRxChannel(channel,
SFP_2, addr);
1630 SelectRxChannel(channel,
SFP_1, addr);
1662 forceReconfig =
true;
1664 forceReconfig =
false;
#define kReg2022_6_tx_sec_mac_hi_addr
#define kReg2022_6_tx_ssrc
@ NTV2IpErrCannotGetMacAddress
void SetIGMPGroup(eSFP port, NTV2Stream stream, uint32_t mcast_addr, uint32_t src_addr, bool enable)
bool GetTxChannelEnable(const NTV2Channel channel, bool &enabled)
Configures a SMPTE 2022 Transmit Channel.
@ kJ2KChromaSubSamp_422_Standard
bool GetIPServicesControl(bool &enable, bool &forceReconfig)
#define kReg2022_6_rx_chan_enable
#define kReg2022_6_rx_media_buf_base_addr
#define kRegSarekSFPStatus
bool operator!=(const tx_2022_channel &other)
bool SetJ2KDecoderConfiguration(const j2kDecoderConfig &j2kConfig)
#define kReg2022_6_rx_channel_access
#define kReg2022_6_tx_link_enable
#define kReg2022_6_tx_pri_mac_hi_addr
uint32_t sfp1ValidRxPackets
#define kReg2022_6_rx_match_dest_ip_addr
#define SAREK_2022_2_TX_CORE_0
bool operator!=(const rx_2022_channel &other)
uint32_t mbps
Specifies the mbits per-second for J2K encode.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
#define RX_MATCH_2022_SOURCE_IP
#define kReg2022_6_rx_network_path_differential
bool GetIGMPDisable(eSFP sfp, bool &disabled)
void SetChannel(ULWord channelOffset, ULWord channelNumber)
bool Set2022_7_Mode(bool enable, uint32_t rx_networkPathDifferential)
#define PLL_MATCH_SOURCE_IP
@ NTV2IpErrSFP2NotConfigured
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
#define kReg2022_6_tx_udp_src_port
void UnsetIGMPGroup(eSFP port, NTV2Stream stream)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
#define SAREK_2022_6_TX_CORE_0
std::string sfp1RemoteIP
Specifies remote (destination) IP address.
bool SetTxChannelConfiguration(const NTV2Channel channel, const tx_2022_channel &txConfig)
bool GetRemoteMAC(std::string remote_IPAddress, eSFP port, NTV2Stream stream, std::string &MACaddress)
#define kRegSarekIGMPVersion
bool SetRxMatch(NTV2Channel channel, eSFP link, uint8_t match)
@ NTV2_EMBEDDED_AUDIO_INPUT_VIDEO_1
uint32_t videoPid
Specifies the PID for the video.
#define kReg2022_6_rx_link_valid_media_pkt_cnt
uint32_t playoutDelay
Specifies the wait time in milliseconds to SDI playout from incoming packet (0-150).
#define SAREK_2022_6_RX_CORE_0
bool ReadChannelRegister(const ULWord inReg, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0x0)
@ DEVICE_ID_IOIP_2022
See Io IP.
Defines a number of handy byte-swapping macros.
#define kReg2022_6_rx_playout_delay
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
#define kReg2022_6_rx_match_vlan
uint32_t sfp2RemotePort
Specifies the remote (destination) port number.
uint32_t ssrc
Specifies the SSRC identifier (if RX_MATCH_2022_SSRC set)
bool GetJ2KDecoderStatus(j2kDecoderStatus &status)
bool GetTxChannelConfiguration(const NTV2Channel channel, tx_2022_channel &txConfig)
uint32_t sfp1RemotePort
Specifies the remote (destination) port number.
#define SFP_2_NOT_PRESENT
bool GetRxLinkState(NTV2Channel channel, bool &sfp1Enable, bool &sfp2Enable)
uint16_t sfp1Vlan
Specifies the VLAN TCI (if RX_MATCH_2022_VLAN set)
std::string sfp2RemoteIP
Specifies remote (destination) IP address.
J2KStreamType streamType
Specifies the stream type for J2K encode.
#define RX_MATCH_2022_DEST_IP
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
uint32_t audioChannels
Specifies the number of audio channels for J2K encode, a value of 0 indicates no audio.
bool GetRxMatch(NTV2Channel channel, eSFP link, uint8_t &match)
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
bool SetIGMPVersion(eIGMPVersion_t version)
#define VOIP_SEMAPHORE_SET
bool GetJ2KDecoderConfiguration(j2kDecoderConfig &j2kConfig)
bool SetJ2KEncoderConfiguration(const NTV2Channel channel, const j2kEncoderConfig &j2kConfig)
bool GetNetworkConfiguration(const eSFP sfp, IPVNetConfig &netConfig)
std::string sfp1SourceIP
Specifies the source (sender) IP address (if RX_MATCH_2022_SOURCE_IP set). If it's in the multiclass ...
#define kReg2022_6_tx_dest_mac_low_addr
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
bool SetupJ2KEncoder(const NTV2Channel channel, const j2kEncoderConfig &config)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
uint32_t sfp2LocalPort
Specifies the local (source) port number.
bool GetDualLinkMode(bool &enable)
uint32_t sfp1LocalPort
Specifies the local (source) port number.
Declares the CNTV2Config2022 class.
bool DisableNetworkInterface(eSFP port)
uint32_t bitDepth
Specifies the bit depth for J2K encode.
bool ReadbackJ2KEncoder(const NTV2Channel channel, j2kEncoderConfig &config)
bool DisableNetworkInterface(const eSFP sfp)
bool SetIGMPVersion(uint32_t version)
#define kReg2022_6_rx_control
bool GetSFPActive(eSFP sfp)
#define kReg2022_6_rx_match_ssrc
#define NTV2EndianSwap32(__val__)
bool SetTxChannelEnable(const NTV2Channel channel, bool enable)
bool operator==(const tx_2022_channel &other)
bool GetSFPMSAData(eSFP sfp, SFPMSAData &data)
#define kReg2022_6_rx_chan_timeout
bool SetTxLinkState(NTV2Channel channel, bool sfp1Enable, bool sfp2Enable)
NTV2IpError getLastErrorCode()
#define kRegSarekServices
Declares the CNTV2Card class.
#define RX_MATCH_2022_SOURCE_PORT
bool SetIPServicesControl(const bool enable, const bool forceReconfig)
bool WriteChannelRegister(ULWord reg, ULWord value, ULWord mask=0xFFFFFFFF, ULWord shift=0x0)
bool GetLinkStatus(eSFP sfp, SFPStatus &sfpStatus)
#define kReg2022_6_tx_chan_enable
#define kReg2022_6_tx_src_ip_addr
uint8_t sfp1RxMatch
Bitmap of rxMatch criteria used.
#define SAREK_2022_6_RX_CORE_1
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
#define kSarekRegIGMPDisable
bool operator!=(const j2kEncoderConfig &other)
@ NTV2IpErrInvalidIGMPVersion
#define SFP_1_NOT_PRESENT
Declares numerous NTV2 utility functions.
std::string sfp1DestIP
Specifies the destination (target) IP address (if RX_MATCH_2022_DEST_IP set)
#define RX_MATCH_2022_DEST_PORT
#define kReg2022_6_rx_match_dest_port
uint32_t ullMode
Specifies the ull mode for J2K encode.
I interrogate and control an AJA video/audio capture/playout device.
#define PLL_MATCH_SOURCE_PORT
#define kReg2022_6_tx_dest_ip_addr
#define SAREK_2022_2_TX_CORE_1
#define kSarekRegIGMPDisable2
#define kReg2022_6_tx_ip_header
#define kReg2022_6_tx_reset
virtual bool IsMBSystemValid(void)
bool GetIGMPVersion(eIGMPVersion_t &version)
#define kReg2022_6_tx_control
std::string sfp2DestIP
Specifies the destination (target) IP address (if RX_MATCH_2022_DEST_IP set)
bool GetRxChannelEnable(const NTV2Channel channel, bool &enabled)
#define kReg2022_6_tx_dest_mac_hi_addr
NTV2Stream
Identifies a specific IP-based data stream.
#define PLL_MATCH_DEST_PORT
bool SetDualLinkMode(bool enable)
uint32_t sfp1DestPort
Specifies the destination (target) port number (if RX_MATCH_2022_DEST_PORT set)
bool operator==(const j2kEncoderConfig &other)
#define kReg2022_6_tx_sec_mac_low_addr
@ NTV2IpErrSoftwareMismatch
bool SetRxChannelEnable(const NTV2Channel channel, bool enable)
uint32_t sfp2SourcePort
Specifies the source (sender) port number (if RX_MATCH_2022_SOURCE_PORT set)
bool ReadbackJ2KDecoder(j2kDecoderConfig &config)
#define kReg2022_6_tx_sys_mem_conf
@ NTV2_AUDIO_AES
Obtain audio samples from the device AES inputs, if available.
#define kReg2022_6_rx_reset
bool GetSFPInfo(eSFP port, SFPMSAData &sfpdata)
#define SAREK_2022_2_RX_CORE_1
#define SAREK_2022_2_RX_CORE_0
bool GetTxLinkState(NTV2Channel channel, bool &sfp1Enable, bool &sfp2Enable)
#define kReg2022_6_tx_video_para_config
uint32_t pmtPid
Specifies the PID for the PMT.
bool operator!=(const j2kDecoderConfig &other)
bool Get2022ChannelRxStatus(NTV2Channel channel, s2022RxChannelStatus &status)
bool GetMACAddress(eSFP sfp, NTV2Stream stream, std::string remoteIP, uint32_t &hi, uint32_t &lo)
uint32_t sfp2ValidRxPackets
#define kReg2022_2_tx_ts_config
#define kReg2022_6_rx_match_sel
std::string sfp2SourceIP
Specifies the source (sender) IP address (if RX_MATCH_2022_SOURCE_IP set). If it's in the multiclass ...
bool SetupJ2KDecoder(const j2kDecoderConfig &config)
CNTV2Config2022(CNTV2Card &device)
bool operator==(const rx_2022_channel &other)
#define SAREK_2022_6_TX_CORE_1
bool Get2022_7_Mode(bool &enable, uint32_t &rx_networkPathDifferential)
NTV2VideoFormat videoFormat
Specifies the video format for J2K encode.
#define kReg2022_6_tx_udp_dest_port
virtual NTV2DeviceID GetDeviceID(void)
#define kRegSarekLinkStatus
bool SetMBNetworkConfiguration(eSFP port, std::string ipaddr, std::string netmask, std::string gateway)
bool GetJ2KEncoderConfiguration(const NTV2Channel channel, j2kEncoderConfig &j2kConfig)
#define PLL_MATCH_DEST_IP
void EnableIGMPGroup(eSFP port, NTV2Stream stream, bool enable)
Configures a SMPTE 2022 Receive Channel.
J2KChromaSubSampling chromaSubsamp
Specifies the chroma sub sampling for J2K encode.
uint8_t sfp2RxMatch
Bitmap of rxMatch criteria used.
std::string getLastError()
@ NTV2IpErrSFP1NotConfigured
uint32_t sfp2DestPort
Specifies the destination (target) port number (if RX_MATCH_2022_DEST_PORT set)
#define kReg2022_6_rx_match_src_port
bool GetJ2KDecoderStatus(j2kDecoderStatus &j2kStatus)
bool SetIGMPDisable(eSFP sfp, bool disable)
Disables the automatic (default) joining of multicast groups using IGMP, based on remote IP address f...
static bool MacAddressToRegisters(const MACAddr &macaddr, uint32_t &hi, uint32_t &lo)
uint32_t pcrPid
Specifies the PID for the PCR.
#define kReg2022_6_tx_pri_mac_low_addr
std::string NTV2IpErrorEnumToString(const NTV2IpError inIpErrorEnumValue)
NTV2IpError getLastErrorCode()
bool operator==(const j2kDecoderConfig &other)
bool SetRxChannelConfiguration(const NTV2Channel channel, const rx_2022_channel &rxConfig)
Declares the CNTV2ConfigTs2022 class.
uint32_t audio1Pid
Specifies the PID for audio 1.
#define kReg2022_6_rx_sec_recv_pkt_cnt
uint32_t sfp1SourcePort
Specifies the source (sender) port number (if RX_MATCH_2022_SOURCE_PORT set)
#define kReg2022_6_rx_media_pkt_buf_size
bool GetRxChannelConfiguration(const NTV2Channel channel, rx_2022_channel &rxConfig)
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
bool SetNetworkConfiguration(const eSFP sfp, const IPVNetConfig &netConfig)
virtual bool IsMBSystemReady(void)
#define VOIP_SEMAPHORE_CLEAR
bool SetRxLinkState(NTV2Channel channel, bool sfp1Enable, bool sfp2Enable)
#define kReg2022_6_rx_pri_recv_pkt_cnt
#define kReg2022_6_tx_channel_access
eProgSelMode_t selectionMode
#define kReg2022_6_tx_hitless_config
#define kReg2022_6_rx_match_src_ip_addr
The CNTV2ConfigTs2022 class is the interface to Kona-IP SMPTE 2022 J2K encoder and TS chips.
uint16_t sfp2Vlan
Specifies the VLAN TCI (if RX_MATCH_2022_VLAN set)