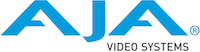 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
52 while (mPlayThread.
Active())
55 while (mCaptureThread.
Active())
79 if (!mDevice.
features().CanDoCapture())
80 {cerr <<
"## ERROR: Device '" << mDeviceID <<
"' cannot capture" << endl;
return AJA_STATUS_FEATURE;}
81 if (!mDevice.
features().CanDoPlayback())
82 {cerr <<
"## ERROR: Device '" << mDeviceID <<
"' cannot playout" << endl;
return AJA_STATUS_FEATURE;}
92 cerr <<
"## ERROR: Unable to acquire device because another app (pid " << appPID <<
") owns it" << endl;
100 if (mDevice.
features().HasSDIRelays())
117 if (mConfig.WithAnc())
144 if (mDevice.IsRemote())
145 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl;
148 BURNINFO(
"Configuration: " << mConfig);
156 const UWord numFrameStores (mDevice.
features().GetNumFrameStores());
160 {cerr <<
"## ERROR: Device does not have the specified input source" << endl;
return AJA_STATUS_BAD_PARAM;}
210 if (mDevice.
features().HasBiDirectionalSDI()
216 if (mDevice.
features().HasBiDirectionalSDI()
228 {cerr <<
"## ERROR: No input signal, or can't handle its format" << endl;
return AJA_STATUS_NOINPUT;}
238 if (tcChannel >= endNum)
242 if (mDevice.
features().HasBiDirectionalSDI()
263 if (mDevice.
features().CanDoMultiFormat())
268 if (mDevice.
features().CanDoMultiFormat())
286 bool bypassIsEnabled (
false);
306 if (mFormatDesc.IsPlanar())
307 {cerr <<
"## ERROR: This demo doesn't work with planar pixel formats" << endl;
return AJA_STATUS_UNSUPPORTED;}
332 if (mDevice.
features().GetNumAudioSystems() > 1)
337 if (SDIoutput >= mDevice.
features().GetNumVideoOutputs())
338 SDIoutput = mDevice.
features().GetNumVideoOutputs() - 1;
341 if (mDevice.
features().GetNumHDMIVideoOutputs() > 0)
369 ULWord ancBuffSizeBytes (0);
384 BURNFAIL(
"Failed to allocate " <<
xHEX0N(vidBuffSizeBytes,8) <<
"-byte video buffer");
387 #ifdef NTV2_BUFFER_LOCKING
402 if (mConfig.WithAnc())
406 BURNFAIL(
"Failed to allocate " <<
xHEX0N(ancBuffSizeBytes,8) <<
"-byte anc buffer");
412 BURNFAIL(
"Failed to allocate " <<
xHEX0N(ancBuffSizeBytes,8) <<
"-byte F2 anc buffer");
422 mFrameDataRing.
Add(&frameData);
444 mDevice.
Connect (cscVideoInputXpt, inputOutputXpt);
445 mDevice.
Connect (fbInputXpt, cscOutputXpt);
448 mDevice.
Connect (fbInputXpt, inputOutputXpt);
465 mDevice.
Connect (cscVidInputXpt, fbOutputXpt);
466 mDevice.
Connect (outputInputXpt, cscVidOutputXpt);
467 outputXpt = cscVidOutputXpt;
470 mDevice.
Connect (outputInputXpt, outputXpt);
492 if (mDevice.
features().HasBiDirectionalSDI())
504 if (mDevice.
features().GetNumHDMIVideoOutputs() > 0)
511 PLDBG(mTCOutputs.size() <<
" timecode destination(s): " << mTCOutputs);
554 ULWord goodXfers(0), badXfers(0), starves(0), noRoomWaits(0);
566 {
BURNFAIL(
"AutoCirculateInitForOutput failed"); mGlobalQuit =
true;}
567 else if (!mConfig.WithVideo())
589 {starves++;
continue;}
625 BURNNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
626 <<
DEC(starves) <<
" ring starves, " <<
DEC(noRoomWaits) <<
" device starves");
643 mCaptureThread.
Start();
667 ULWord goodXfers(0), badXfers(0), ringFulls(0), devWaits(0);
681 {
BURNFAIL(
"AutoCirculateInitForInput failed"); mGlobalQuit =
true;}
691 if (mDevice.
features().HasSDIRelays())
701 {ringFulls++;
continue;}
720 string timeCodeString;
726 CRP188 inputRP188Info (timecodeValue);
733 CRP188 analogRP188Info (timecodeValue);
780 BURNNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
781 <<
DEC(ringFulls) <<
" ring full(s), " <<
DEC(devWaits) <<
" device waits");
799 switch (inInputSource)
821 return regNum && mDevice.
ReadRegister(regNum, regValue) && regValue &
BIT(16);
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
ULWord NumCapturedAnc2Bytes(void) const
static ULWord GetRP188RegisterForInput(const NTV2InputSource inInputSource)
NTV2Channel NTV2TimecodeIndexToChannel(const NTV2TCIndex inTCIndex)
Converts the given NTV2TCIndex value into the appropriate NTV2Channel value.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
@ NTV2_INPUTSOURCE_SDI4
Identifies the 4th SDI video input.
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
const AUTOCIRCULATE_TRANSFER_STATUS & GetTransferStatus(void) const
Returns a constant reference to my AUTOCIRCULATE_TRANSFER_STATUS.
#define NTV2_IS_SDI_TIMECODE_INDEX(__x__)
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
virtual bool GetAncRegionOffsetFromBottom(ULWord &outByteOffsetFromBottom, const NTV2AncillaryDataRegion inAncRegion=NTV2_AncRgn_All)
Answers with the byte offset to the start of an ancillary data region within a device frame buffer,...
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
ULWord GetCapturedAudioByteCount(void) const
@ NTV2_INPUTSOURCE_SDI6
Identifies the 6th SDI video input.
#define NTV2_IS_VALID_TASK_MODE(__m__)
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
UWord firstFrame(void) const
virtual bool KickSDIWatchdog(void)
Restarts the countdown timer to prevent the watchdog timer from timing out.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
ULWord GetProcessedFrameCount(void) const
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
virtual bool SetSDIWatchdogEnable(const bool inEnable, const UWord inIndex0)
Sets the connector pair relays to be under watchdog timer control or manual control.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
Declares common types used in the ajabase library.
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
bool Is8BitFrameBufferFormat(const NTV2FrameBufferFormat fbFormat)
bool GetRP188Reg(RP188_STRUCT &outRP188) const
@ NTV2_INPUTSOURCE_SDI7
Identifies the 7th SDI video input.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
ULWord fNumAncBytes
Actual number of captured F1 anc bytes.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
virtual void RouteOutputSignal(void)
Sets up board routing for playout.
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
NTV2Buffer & AncBuffer2(void)
virtual bool ReadAnalogLTCInput(const UWord inLTCInput, RP188_STRUCT &outRP188Data)
Reads the current contents of the device's analog LTC input registers.
Declares the AJATime class.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
NTV2TCIndex fTimecodeSource
Timecode source to use.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
static void CaptureThreadStatic(AJAThread *pThread, void *pContext)
This is the capture thread's static callback function that gets called when the capture thread runs....
NTV2EmbeddedAudioInput NTV2InputSourceToEmbeddedAudioInput(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2EmbeddedAudioInput value.
Declares the NTV2TestPatternGen class.
NTV2InputXptID GetFrameBufferInputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsBInput=false)
virtual void StartCaptureThread(void)
Starts my capture thread.
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
@ NTV2_AncRgn_Field2
Identifies the "normal" Field 2 ancillary data region.
NTV2Buffer fAncBuffer2
Additional "F2" host anc buffer.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
bool fSuppressVideo
If true, suppress video; otherwise include video.
bool SetAncBuffers(ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my ancillary data buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2Buffer fAncBuffer
Host ancillary data buffer.
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool GetSDITransmitEnable(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the specified SDI connector is currently acting as a transmitter (i....
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
bool CanAcceptMoreOutputFrames(void) const
@ NTV2_FIELD0
Identifies the first field in time for an interlaced video frame, or the first and only field in a pr...
NTV2FrameRate
Identifies a particular video frame rate.
@ NTV2_INPUTSOURCE_HDMI3
Identifies the 3rd HDMI video input.
virtual void StartPlayThread(void)
Starts my playout thread.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
NTV2AudioSystem NTV2InputSourceToAudioSystem(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2AudioSystem value.
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
NTV2Buffer & VideoBuffer(void)
bool IsProgressivePicture(const NTV2VideoFormat format)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=(0), bool inRDMA=(0))
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
virtual bool EnableOutputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to output vertical blanking interrupts originat...
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
NTV2PixelFormat fPixelFormat
The pixel format to use.
virtual void CaptureFrames(void)
Repeatedly captures frames using AutoCirculate (until global quit flag set).
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
virtual void GetStatus(AUTOCIRCULATE_STATUS &outInputStatus, AUTOCIRCULATE_STATUS &outOutputStatus)
Provides status information about my input (capture) and output (playout) processes.
virtual class DeviceCapabilities & features(void)
NTV2ACFrameRange fOutputFrames
Playout frame count or range.
@ NTV2_INPUTSOURCE_ANALOG1
Identifies the first analog video input.
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
ULWord NumCapturedAncBytes(void) const
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
#define NTV2_IS_ANALOG_TIMECODE_INDEX(__x__)
NTV2OutputDestination NTV2ChannelToOutputDestination(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its ordinary equivalent NTV2OutputDestination.
static size_t DefaultPageSize(void)
NTV2Burn(const BurnConfig &inConfig)
Constructs me using the given configuration settings.
@ NTV2_OUTPUTDESTINATION_SDI1
virtual bool GetRP188Data(const NTV2Channel inSDIInput, NTV2_RP188 &outRP188Data)
Reads the raw RP188 data from the DBB/Low/Hi registers for the given SDI input. On newer devices with...
Declares the AJAProcess class.
virtual bool SetRP188SourceFilter(const NTV2Channel inSDIInput, const UWord inFilterValue)
Sets the RP188 DBB filter for the given SDI input.
std::string NTV2TCIndexToString(const NTV2TCIndex inValue, const bool inCompactDisplay=false)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_AUDIOSYSTEM_2
This identifies the 2nd Audio System.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
bool HasValidTimecode(const NTV2TCIndex inTCNdx) const
ULWord NumCapturedAudioBytes(void) const
virtual std::string GetDescription(void) const
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
ULWord VideoBufferSize(void) const
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
bool SetOutputTimeCodes(const NTV2TimeCodes &inValues)
Intended for playout, replaces the contents of my acOutputTimeCodes member.
static size_t SetDefaultPageSize(void)
@ NTV2_INPUTSOURCE_INVALID
The invalid video input.
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC=false, const bool inIsF2=false)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
@ NTV2_INPUTSOURCE_SDI5
Identifies the 5th SDI video input.
ULWord AncBufferSize(void) const
static void PlayThreadStatic(AJAThread *pThread, void *pContext)
This is the playout thread's static callback function that gets called when the playout thread runs....
virtual bool SetLTCInputEnable(const bool inEnable)
Enables or disables the ability for the device to read analog LTC on the reference input connector.
virtual CNTV2DriverInterface & driverInterface(void)
ULWord GetCapturedAncByteCount(const bool inField2=false) const
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord AudioBufferSize(void) const
ULWord AncBuffer2Size(void) const
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
NTV2InputCrosspointID
Identifies a widget input that potentially can accept a signal emitted from another widget's output (...
NTV2Buffer & AudioBuffer(void)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
virtual bool IsDeviceReady(const bool inCheckValid=(0))
@ NTV2_INPUTSOURCE_HDMI4
Identifies the 4th HDMI video input.
virtual bool InputSignalHasTimecode(void)
Returns true if the current input signal has timecode embedded in it; otherwise returns false.
virtual void Quit(void)
Gracefully stops me from running.
bool CanDoInputSource(const NTV2InputSource inSrc)
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
bool fWithAnc
If true, capture & play anc data (LLBurn). Defaults to false.
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
uint16_t GetEndFrame(void) const
#define NTV2_AUDIOSIZE_MAX
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual bool SetSDIWatchdogTimeout(const ULWord inValue)
Specifies the amount of time that must elapse before the watchdog timer times out.
#define NTV2_OUTPUT_DEST_IS_SDI(_dest_)
#define NTV2_IS_VALID_CHANNEL(__x__)
@ NTV2_DISABLE_TASKS
0: Disabled: Device is completely configured by controlling application(s) – no driver involvement.
NTV2Channel fInputChannel
The input channel to use.
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing and playing video.
The NTV2 test pattern generator.
@ NTV2_INPUTSOURCE_HDMI2
Identifies the 2nd HDMI video input.
NTV2OutputCrosspointID
Identifies a widget output, a signal source, that potentially can drive another widget's input (ident...
std::string fDeviceSpec
The AJA device to use.
NTV2InputSource
Identifies a specific video input source.
NTV2InputSource fInputSource
The device input connector to use.
NTV2Buffer fAudioBuffer
Host audio buffer.
I capture frames from a video signal provided to an AJA device's video input. I burn timecode into th...
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=(0))
Connects the given widget signal input (sink) to the given widget signal output (source).
virtual bool SetHDMIOutAudioSource2Channel(const NTV2AudioChannelPair inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the HDMI output's 2-channel audio source.
virtual void PlayFrames(void)
Repeatedly plays out frames using AutoCirculate (until global quit flag set).
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
Header file for the NTV2Burn demonstration class.
UWord lastFrame(void) const
bool IsRunning(void) const
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
@ NTV2_OUTPUTDESTINATION_INVALID
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
@ NTV2_INPUTSOURCE_SDI8
Identifies the 8th SDI video input.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
@ NTV2_INPUTSOURCE_SDI2
Identifies the 2nd SDI video input.
This struct replaces the old RP188_STRUCT.
@ NTV2_TCINDEX_LTC2
Analog LTC 2.
virtual bool AnalogLTCInputHasTimecode(void)
Returns true if there is a valid LTC signal on my device's primary analog LTC input port; otherwise r...
bool IsRGBFormat(const NTV2FrameBufferFormat format)
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
uint16_t GetStartFrame(void) const
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
ULWord fNumAnc2Bytes
Actual number of captured F2 anc bytes.
A handy class that makes it easy to "bounce" an unsigned integer value between a minimum and maximum ...
virtual AJAStatus SetupHostBuffers(void)
Sets up my circular buffers.
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
bool CanDoWidget(const NTV2WidgetID inWgtID)
virtual void RouteInputSignal(void)
Sets up board routing for capture.
static bool GetWidgetForInput(const NTV2InputXptID inInputXpt, NTV2WidgetID &outWidgetID, const NTV2DeviceID inDeviceID=DEVICE_ID_NOTFOUND)
Returns the widget that "owns" the specified input crosspoint.
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
NTV2OutputXptID GetInputSourceOutputXpt(const NTV2InputSource inInputSource, const bool inIsSDI_DS2=false, const bool inIsHDMI_RGB=false, const UWord inHDMI_Quadrant=0)
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing and playing audio.
NTV2InputSource NTV2TimecodeIndexToInputSource(const NTV2TCIndex inTCIndex)
Converts the given NTV2TCIndex value into the appropriate NTV2InputSource value.
virtual bool IsRP188BypassEnabled(const NTV2Channel inSDIOutput, bool &outIsBypassEnabled)
Answers if the SDI output's RP-188 bypass mode is enabled or not.
virtual bool DisableRP188Bypass(const NTV2Channel inSDIOutput)
Configures the SDI output's embedder to embed SMPTE 12M timecode specified in calls to CNTV2Card::Set...
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
virtual AJAStatus Run(void)
Runs me.
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
virtual bool WaitForInputFieldID(const NTV2FieldID inFieldID, const NTV2Channel inChannel=NTV2_CHANNEL1)
Efficiently sleeps the calling thread/process until the next input VBI for the given field and input ...
bool GetInputTimeCodes(NTV2TimeCodeList &outValues) const
Intended for capture, answers with the timecodes captured in my acTransferStatus member's acFrameStam...
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
@ NTV2_INPUTSOURCE_SDI3
Identifies the 3rd SDI video input.
virtual AJAStatus Start()
NTV2AudioSource NTV2InputSourceToAudioSource(const NTV2InputSource inInputSource)
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
#define xHEX0N(__x__, __n__)
#define AJA_FAILURE(_status_)
NTV2ACFrameRange fInputFrames
Ingest frame count or range.
#define NTV2_IS_VALID_AUDIO_SYSTEM(__x__)
@ NTV2_AudioChannel1_2
This selects audio channels 1 and 2 (Group 1 channels 1 and 2)
@ NTV2_TCINDEX_LTC1
Analog LTC 1.
#define NTV2_INPUT_SOURCE_IS_ANALOG(_inpSrc_)
Configures an NTV2Burn or NTV2FieldBurn instance.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
bool CanDoFrameBufferFormat(const NTV2PixelFormat inPF)
NTV2Buffer & AncBuffer(void)
static const uint32_t kAppSignature(((((uint32_t)( 'B'))<< 24)|(((uint32_t)( 'u'))<< 16)|(((uint32_t)( 'r'))<< 8)|(((uint32_t)( 'n'))<< 0)))
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
bool GetRP188Str(std::string &sRP188) const
NTV2Channel fOutputChannel
The output channel to use.
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
NTV2TimeCodes fTimecodes
Map of TC indexes to NTV2_RP188 values.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID