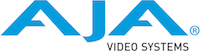 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
17 #define ToCharPtr(_p_) reinterpret_cast<char*>(_p_)
51 while (mPlayThread.
Active())
54 while (mCaptureThread.
Active())
79 {cerr <<
"## ERROR: Device '" << mDeviceID <<
"' cannot capture" << endl;
return AJA_STATUS_FEATURE;}
81 {cerr <<
"## ERROR: Device '" << mDeviceID <<
"' cannot playout" << endl;
return AJA_STATUS_FEATURE;}
91 cerr <<
"## ERROR: Unable to acquire device because another app (pid " << appPID <<
") owns it" << endl;
123 cerr << mConfig << endl;
125 BURNINFO(
"Configuration: " << mConfig);
137 {cerr <<
"## ERROR: Device does not have the specified input source" << endl;
return AJA_STATUS_BAD_PARAM;}
201 {cerr <<
"## ERROR: No input signal, or can't handle its format" << endl;
return AJA_STATUS_NOINPUT;}
238 bool bypassIsEnabled (
false);
255 if (mFormatDesc.IsPlanar())
256 {cerr <<
"## ERROR: This demo doesn't work with planar pixel formats" << endl;
return AJA_STATUS_UNSUPPORTED;}
330 if (!mConfig.FieldMode())
346 failures.push_back(
ULWord(mHostBuffers.size()+1));
349 mFrameDataRing.
Add (&frameData);
352 if (!failures.empty())
354 cerr <<
"## ERROR: " <<
DEC(failures.size()) <<
" allocation failures in buffer(s): " << failures << endl;
376 mDevice.
Connect (cscVideoInputXpt, inputOutputXpt);
377 mDevice.
Connect (fbInputXpt, cscOutputXpt);
380 mDevice.
Connect (fbInputXpt, inputOutputXpt);
397 mDevice.
Connect (cscVidInputXpt, fbOutputXpt);
398 mDevice.
Connect (outputInputXpt, cscVidOutputXpt);
399 outputXpt = cscVidOutputXpt;
402 mDevice.
Connect (outputInputXpt, outputXpt);
424 mTCOutputs.push_back(chan);
484 ULWord goodXfers(0), badXfers(0), starves(0), noRoomWaits(0);
494 if (!mConfig.FieldMode())
515 {
PLFAIL(
"AutoCirculateInitForOutput failed"); mGlobalQuit =
true;}
528 {starves++;
continue;}
539 if (mConfig.FieldMode())
552 if (!mConfig.FieldMode())
579 BURNNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
580 <<
DEC(starves) <<
" ring starves, " <<
DEC(noRoomWaits) <<
" device starves");
597 mCaptureThread.
Start();
623 ULWord goodXfers(0), badXfers(0), ringFulls(0), devWaits(0);
627 for (
size_t ndx(0); ndx < mTCOutputs.size(); ndx++)
634 if (!mConfig.FieldMode())
664 {
BURNFAIL(
"AutoCirculateInitForInput failed"); mGlobalQuit =
true;}
681 {ringFulls++;
continue;}
695 if (mConfig.FieldMode())
703 thisFrameTC = pFrameData->
fTimecodes.begin()->second;
717 if (!mConfig.FieldMode())
729 if (!mConfig.FieldMode())
747 if (mConfig.FieldMode())
756 BURNNOTE(
"Thread completed: " <<
DEC(goodXfers) <<
" xfers, " <<
DEC(badXfers) <<
" failed, "
757 <<
DEC(ringFulls) <<
" ring full(s), " <<
DEC(devWaits) <<
" device waits");
766 ULWord & outCaptureBufferLevel,
ULWord & outPlayoutBufferLevel)
784 switch (inInputSource)
806 if (regNum && mDevice.
ReadRegister(regNum, regValue) && regValue &
BIT(16))
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
uint32_t fFrameFlags
Frame data flags.
bool NTV2DeviceCanDoCapture(const NTV2DeviceID inDeviceID)
#define AUTOCIRCULATE_FRAME_FIELD0
Frame contains field 0 of an interlaced image (first field in time)
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
@ NTV2_INPUTSOURCE_SDI4
Identifies the 4th SDI video input.
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
ULWord acFramesProcessed
Total number of frames successfully processed since CNTV2Card::AutoCirculateStart called.
virtual void StartCaptureThread(void)
Starts my capture thread.
const AUTOCIRCULATE_TRANSFER_STATUS & GetTransferStatus(void) const
Returns a constant reference to my AUTOCIRCULATE_TRANSFER_STATUS.
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
std::string NTV2ChannelListToStr(const NTV2ChannelList &inObj, const bool inCompact=true)
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
virtual bool InputSignalHasTimecode(void)
Returns true if the current input signal has timecode embedded in it; otherwise returns false.
ULWord GetCapturedAudioByteCount(void) const
@ NTV2_INPUTSOURCE_SDI6
Identifies the 6th SDI video input.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing and playing audio.
#define NTV2_IS_VALID_TASK_MODE(__m__)
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
UWord firstFrame(void) const
#define AUTOCIRCULATE_WITH_FIELDS
Use this to AutoCirculate with fields as frames for interlaced formats.
ULWord GetProcessedFrameCount(void) const
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
Declares common types used in the ajabase library.
std::set< NTV2TCIndex > NTV2TCIndexes
A set of distinct NTV2TCIndex values.
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
bool Is8BitFrameBufferFormat(const NTV2FrameBufferFormat fbFormat)
bool GetRP188Reg(RP188_STRUCT &outRP188) const
@ NTV2_INPUTSOURCE_SDI7
Identifies the 7th SDI video input.
virtual void StartPlayThread(void)
Starts my playout thread.
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
static const uint32_t kAppSignature(((((uint32_t)( 'F'))<< 24)|(((uint32_t)( 'l'))<< 16)|(((uint32_t)( 'd'))<< 8)|(((uint32_t)( 'B'))<< 0)))
virtual void GetStatus(ULWord &outNumProcessed, ULWord &outCaptureDrops, ULWord &outPlayoutDrops, ULWord &outCaptureLevel, ULWord &outPlayoutLevel)
Provides status information about my input (capture) and output (playout) processes.
#define NTV2_ASSERT(_expr_)
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
LWord acTransferFrame
Frame buffer number the frame was transferred to/from. (-1 if failed)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
Declares the AJATime class.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
bool EnableSegmentedDMAs(const ULWord inNumSegments, const ULWord inNumActiveBytesPerLine, const ULWord inHostBytesPerLine, const ULWord inDeviceBytesPerLine)
Enables segmented DMAs given a segment count, a host pitch, and device pitch value.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
NTV2TCIndex fTimecodeSource
Timecode source to use.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
NTV2EmbeddedAudioInput NTV2InputSourceToEmbeddedAudioInput(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2EmbeddedAudioInput value.
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
NTV2InputXptID GetFrameBufferInputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsBInput=false)
bool NTV2DeviceCanDoDualLink(const NTV2DeviceID inDeviceID)
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
bool IsProgressiveTransport(const NTV2VideoFormat format)
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
bool NTV2DeviceCanDoFrameBufferFormat(const NTV2DeviceID inDeviceID, const NTV2FrameBufferFormat inFBFormat)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
NTV2FieldBurn(const BurnConfig &inConfig)
Constructs me using the given configuration settings.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool GetSDITransmitEnable(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the specified SDI connector is currently acting as a transmitter (i....
virtual bool SetVANCShiftMode(NTV2Channel inChannel, NTV2VANCDataShiftMode inMode)
Enables or disables the "VANC Shift Mode" feature for the given channel.
bool CanAcceptMoreOutputFrames(void) const
@ NTV2_FIELD0
Identifies the first field in time for an interlaced video frame, or the first and only field in a pr...
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
NTV2FrameRate
Identifies a particular video frame rate.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
virtual void RouteInputSignal(void)
Sets up board routing for capture.
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
NTV2Buffer & VideoBuffer(void)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
virtual bool EnableOutputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to output vertical blanking interrupts originat...
virtual void RouteOutputSignal(void)
Sets up board routing for playout.
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
NTV2PixelFormat fPixelFormat
The pixel format to use.
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
static ULWord GetRP188RegisterForInput(const NTV2InputSource inInputSource)
NTV2ACFrameRange fOutputFrames
Playout frame count or range.
@ NTV2_INPUTSOURCE_ANALOG1
Identifies the first analog video input.
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
NTV2OutputDestination NTV2ChannelToOutputDestination(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its ordinary equivalent NTV2OutputDestination.
@ NTV2_OUTPUTDESTINATION_SDI1
Declares the AJAProcess class.
std::string NTV2TCIndexToString(const NTV2TCIndex inValue, const bool inCompactDisplay=false)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
bool NTV2DeviceCanDoWidget(const NTV2DeviceID inDeviceID, const NTV2WidgetID inWidgetID)
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
ULWord VideoBufferSize(void) const
bool NTV2DeviceHasBiDirectionalSDI(const NTV2DeviceID inDeviceID)
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
bool SetOutputTimeCodes(const NTV2TimeCodes &inValues)
Intended for playout, replaces the contents of my acOutputTimeCodes member.
static size_t SetDefaultPageSize(void)
@ NTV2_INPUTSOURCE_INVALID
The invalid video input.
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC=false, const bool inIsF2=false)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
static void CaptureThreadStatic(AJAThread *pThread, void *pContext)
This is the capture thread's static callback function that gets called when the capture thread runs....
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
@ NTV2_INPUTSOURCE_SDI5
Identifies the 5th SDI video input.
virtual void PlayFrames(void)
Repeatedly plays out frames using AutoCirculate (until global quit flag set).
LWord acDesiredFrame
Used to specify a different frame in the circulate ring to transfer to/from.
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=false)
Connects the given widget signal input (sink) to the given widget signal output (source).
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord AudioBufferSize(void) const
ULWord fNumAudioBytes
Actual number of captured audio bytes.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
NTV2InputCrosspointID
Identifies a widget input that potentially can accept a signal emitted from another widget's output (...
NTV2Buffer & AudioBuffer(void)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
UWord NTV2DeviceGetNumFrameStores(const NTV2DeviceID inDeviceID)
virtual void CaptureFrames(void)
Repeatedly captures frames using AutoCirculate (until global quit flag set).
I capture individual fields from an interlaced video signal provided to an SDI input....
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
virtual bool IsDeviceReady(const bool inCheckValid=(0))
bool NTV2DeviceCanDoPlayback(const NTV2DeviceID inDeviceID)
NTV2Buffer fVideoBuffer2
Additional host video buffer, usually F2.
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
ULWord acBufferLevel
Number of buffered frames in driver ready to capture or play.
#define NTV2_AUDIOSIZE_MAX
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
#define NTV2_OUTPUT_DEST_IS_SDI(_dest_)
AUTOCIRCULATE_TRANSFER_STATUS acTransferStatus
Contains status information that's valid after CNTV2Card::AutoCirculateTransfer returns,...
@ NTV2_DISABLE_TASKS
0: Disabled: Device is completely configured by controlling application(s) – no driver involvement.
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
NTV2Channel fInputChannel
The input channel to use.
NTV2OutputCrosspointID
Identifies a widget output, a signal source, that potentially can drive another widget's input (ident...
std::string fDeviceSpec
The AJA device to use.
void * GetHostPointer(void) const
NTV2InputSource
Identifies a specific video input source.
NTV2InputSource fInputSource
The device input connector to use.
NTV2Buffer fAudioBuffer
Host audio buffer.
NTV2Buffer & VideoBuffer2(void)
virtual AJAStatus SetupHostBuffers(void)
Sets up my circular buffers.
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
NTV2TCIndexes::const_iterator NTV2TCIndexesConstIter
A handy const interator for iterating over an NTV2TCIndexes set.
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing and playing video.
UWord lastFrame(void) const
bool IsRunning(void) const
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
@ NTV2_OUTPUTDESTINATION_INVALID
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
virtual void Quit(void)
Gracefully stops me from running.
@ NTV2_INPUTSOURCE_SDI8
Identifies the 8th SDI video input.
bool NTV2DeviceCanDoInputSource(const NTV2DeviceID inDeviceID, const NTV2InputSource inInputSource)
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
@ NTV2_INPUTSOURCE_SDI2
Identifies the 2nd SDI video input.
This struct replaces the old RP188_STRUCT.
NTV2Buffer acVideoBuffer
The host video buffer. This field is owned by the client application, and thus is responsible for all...
bool IsRGBFormat(const NTV2FrameBufferFormat format)
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
ULWord acInVideoDMAOffset
Optional byte offset into the device frame buffer. Defaults to zero. If non-zero, should be a multipl...
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
Header file for the NTV2FieldBurn demonstration class.
ULWord NTV2DeviceGetNumVideoChannels(const NTV2DeviceID inDeviceID)
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
static bool GetWidgetForInput(const NTV2InputXptID inInputXpt, NTV2WidgetID &outWidgetID, const NTV2DeviceID inDeviceID=DEVICE_ID_NOTFOUND)
Returns the widget that "owns" the specified input crosspoint.
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
NTV2OutputXptID GetInputSourceOutputXpt(const NTV2InputSource inInputSource, const bool inIsSDI_DS2=false, const bool inIsHDMI_RGB=false, const UWord inHDMI_Quadrant=0)
static void PlayThreadStatic(AJAThread *pThread, void *pContext)
This is the playout thread's static callback function that gets called when the playout thread runs....
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=false)
Returns the video format of the signal that is present on the given input source.
virtual bool IsRP188BypassEnabled(const NTV2Channel inSDIOutput, bool &outIsBypassEnabled)
Answers if the SDI output's RP-188 bypass mode is enabled or not.
virtual bool DisableRP188Bypass(const NTV2Channel inSDIOutput)
Configures the SDI output's embedder to embed SMPTE 12M timecode specified in calls to CNTV2Card::Set...
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
virtual bool WaitForInputFieldID(const NTV2FieldID inFieldID, const NTV2Channel inChannel=NTV2_CHANNEL1)
Efficiently sleeps the calling thread/process until the next input VBI for the given field and input ...
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
bool GetInputTimeCodes(NTV2TimeCodeList &outValues) const
Intended for capture, answers with the timecodes captured in my acTransferStatus member's acFrameStam...
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
@ NTV2_INPUTSOURCE_SDI3
Identifies the 3rd SDI video input.
virtual AJAStatus Start()
ULWord acFramesDropped
Total number of frames dropped since CNTV2Card::AutoCirculateStart called.
NTV2AudioSource NTV2InputSourceToAudioSource(const NTV2InputSource inInputSource)
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
#define AJA_FAILURE(_status_)
NTV2ACFrameRange fInputFrames
Ingest frame count or range.
#define NTV2_IS_VALID_AUDIO_SYSTEM(__x__)
Configures an NTV2Burn or NTV2FieldBurn instance.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
virtual AJAStatus Run(void)
Runs me.
bool GetRP188Str(std::string &sRP188) const
NTV2Channel fOutputChannel
The output channel to use.
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
NTV2TimeCodes fTimecodes
Map of TC indexes to NTV2_RP188 values.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID