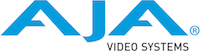 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
39 int main (
int argc,
const char ** argv)
43 int constLuminance (0);
53 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model"},
54 {
"eotf",
'e',
POPT_ARG_INT, &eotf, 0,
"0=TradGammaSDR 1=TradGammaHDR 2=ST2084 3=HLG",
"EOTF to use"},
65 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 1;}
67 {cout <<
"NTV2HDRSetup, NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
69 if ((eotf < 0) || (eotf > 3))
70 {cerr <<
"## ERROR: Bad EOTF value '" <<
DEC(eotf) <<
"' -- expected 0, 1, 2 or 3" << endl;
return 2;}
75 {cerr <<
"## ERROR: Device '" << pDeviceSpec <<
"' not found" << endl;
return 3;}
78 {cerr <<
"## ERROR: Device '" << pDeviceSpec <<
"' not ready" << endl;
return 4;}
81 {cerr <<
"## ERROR: Device '" << pDeviceSpec <<
"' does not support HDMI HDR" << endl;
return 5;}
91 cerr <<
"## ERROR: Unable to acquire device because another app (pid " << appPID <<
") owns it" << endl;
98 static const string sEOTFs[] = {
"Traditional Gamma SDR",
"Traditional Gamma HDR",
"ST-2084",
"HLG",
""};
99 cout <<
"## NOTE: Options specified:" << endl
100 <<
"\tEOTF: " <<
DEC(eotf) <<
" (" << sEOTFs[eotf] <<
")" << endl
101 <<
"\tLuminance: " << (constLuminance ?
"Constant" :
"Non-Constant") << endl
102 <<
"\tDolbyVision: " << (dolbyVision ?
"Enabled" :
"Disabled") << endl;
104 cout <<
"## WARNING: --nohdr option will disable HDMI HDR output" << endl;
void setHDRDefaultsForBT2020(HDRRegValues &outRegisterValues)
virtual bool EnableHDMIHDR(const bool inEnableHDMIHDR)
Enables or disables HDMI HDR.
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
Declares device capability functions.
Declares common types used in the ajabase library.
Declares the AJATime class.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
virtual bool SetHDMIHDRConstantLuminance(const bool inEnableConstantLuminance)
Enables or disables BT.2020 Y'cC'bcC'rc versus BT.2020 Y'C'bC'r or R'G'B'.
int main(int argc, const char **argv)
const uint32_t kAppSignature(((((uint32_t)( 'H'))<< 24)|(((uint32_t)( 'd'))<< 16)|(((uint32_t)( 'r'))<< 8)|(((uint32_t)( 's'))<< 0)))
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
Declares the AJAProcess class.
Declares the CNTV2DeviceScanner class.
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
Declares the CNTV2Card class.
virtual bool SetHDMIHDRElectroOpticalTransferFunction(const uint8_t inEOTFByte)
Declares numerous NTV2 utility functions.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
virtual bool EnableHDMIHDRDolbyVision(const bool inEnable)
Enables or disables HDMI HDR Dolby Vision.
I interrogate and control an AJA video/audio capture/playout device.
static void WaitForEnterKeyPress(void)
Prompts the user (via stdout) to press the Return or Enter key, then waits for it to happen.
virtual bool IsDeviceReady(const bool inCheckValid=(0))
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
static AJAStatus Open(bool incrementRefCount=false)
This file contains some structures, constants, classes and functions that are used in some of the dem...
bool NTV2DeviceCanDoHDMIHDROut(const NTV2DeviceID inDeviceID)
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
virtual NTV2DeviceID GetDeviceID(void)
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual bool SetHDRData(const HDRFloatValues &inFloatValues)
uint8_t electroOpticalTransferFunction
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.