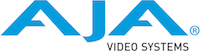 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
15 #define AsULWordPtr(_p_) reinterpret_cast<const ULWord*> (_p_)
75 cerr <<
"## WARNING: '" << mDevice.
GetDisplayName() <<
"' switched to Ch2 (Ch1 is input-only)" << endl;
80 <<
" -- only supports Ch1" << (maxNumChannels > 1 ? string(
"-Ch") + string(1,
char(maxNumChannels+
'0')) :
"") << endl;
104 cerr << mConfig << endl;
105 #endif // defined(_DEBUG)
210 for (
size_t ndx(0); ndx < sdiOutputs.size(); ndx++)
248 uint32_t currentOutputFrame(0);
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool GetConnections(NTV2XptConnections &outConnections)
Answers with the device's current widget routing connections.
AJALabelValuePairs Get(const bool inCompact=false) const
Renders a human-readable representation of me.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputXptID GetSDIOutputInputXpt(const NTV2Channel inSDIOutput, const bool inIsDS2=false)
#define NTV2_IS_VANCMODE_TALLER(__v__)
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=false)
Applies the given routing table to the AJA device.
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
Configures an NTV2OutputTestPattern instance.
ULWord GetByteCount(void) const
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
NTV2ChannelList NTV2MakeChannelList(const NTV2Channel inFirstChannel, const UWord inNumChannels=1)
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
Declares the NTV2TestPatternGen class.
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
bool NTV2DeviceCanDoFrameStore1Display(const NTV2DeviceID inDeviceID)
virtual bool GetVANCMode(NTV2VANCMode &outVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Retrieves the current VANC mode for the given FrameStore.
NTV2OutputTestPattern(const TestPatConfig &inConfig)
Constructs me using the given configuration settings.
#define NTV2_IS_8K_VIDEO_FORMAT(__f__)
NTV2VANCMode fVancMode
VANC mode to use.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
void RouteOutputSignal(void)
Sets up board routing for playout.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
bool NTV2DeviceCanDoVideoFormat(const NTV2DeviceID inDeviceID, const NTV2VideoFormat inVideoFormat)
#define NTV2_IS_4K_VIDEO_FORMAT(__f__)
AJAStatus EmitPattern(void)
Generates, transfers and displays the test pattern on the output.
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
virtual std::string GetDisplayName(void)
Answers with this device's display name.
~NTV2OutputTestPattern(void)
Declares the AJAProcess class.
virtual bool GetOutputFrame(const NTV2Channel inChannel, ULWord &outValue)
Answers with the current output frame number for the given FrameStore (expressed as an NTV2Channel).
NTV2Standard
Identifies a particular video standard.
virtual bool GetVideoFormat(NTV2VideoFormat &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
std::string fTestPatternName
Name of the test pattern to use.
static AJALabelValuePairs & append(AJALabelValuePairs &inOutTable, const std::string &inLabel, const std::string &inValue=std::string())
A convenience function that appends the given label and value strings to the provided AJALabelValuePa...
Declares the CNTV2DeviceScanner class.
bool NTV2DeviceHasBiDirectionalSDI(const NTV2DeviceID inDeviceID)
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
virtual bool SetSDIOutRGBLevelAConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables an RGB-over-3G-level-A conversion at the SDI output widget (assuming the AJA devi...
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
UWord NTV2DeviceGetNumHDMIVideoOutputs(const NTV2DeviceID inDeviceID)
NTV2VideoFormat fVideoFormat
The video format to use.
std::string NTV2VANCModeToString(const NTV2VANCMode inValue, const bool inCompactDisplay=false)
#define NTV2_IS_VANCMODE_ON(__v__)
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
NTV2Channel fOutputChannel
The device channel to use.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
UWord NTV2DeviceGetNumFrameStores(const NTV2DeviceID inDeviceID)
UWord NTV2DeviceGetNumAnalogVideoOutputs(const NTV2DeviceID inDeviceID)
std::pair< NTV2InputXptID, NTV2OutputXptID > NTV2Connection
This links an NTV2InputXptID and an NTV2OutputXptID.
virtual bool IsDeviceReady(const bool inCheckValid=(0))
bool NTV2DeviceCanDoPlayback(const NTV2DeviceID inDeviceID)
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
The NTV2 test pattern generator.
std::vector< NTV2Channel > NTV2ChannelList
An ordered sequence of NTV2Channel values.
void * GetHostPointer(void) const
virtual bool SetSDIOutputStandard(const UWord inOutputSpigot, const NTV2Standard inValue)
Sets the SDI output spigot's video standard.
@ NTV2_OUTPUTDESTINATION_HDMI
std::ostream & operator<<(std::ostream &ioStrm, const TestPatConfig &inObj)
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
std::string fDeviceSpec
The AJA device to use.
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
NTV2ChannelSet NTV2MakeChannelSet(const NTV2Channel inFirstChannel, const UWord inNumChannels=1)
bool IsRGBFormat(const NTV2FrameBufferFormat format)
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual bool IsChannelEnabled(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the given FrameStore is enabled.
virtual NTV2DeviceID GetDeviceID(void)
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
virtual bool SetEnableVANCData(const bool inVANCenabled, const bool inTallerVANC, const NTV2Standard inStandard, const NTV2FrameGeometry inGeometry, const NTV2Channel inChannel=NTV2_CHANNEL1)
const uint32_t kAppSignature(((((uint32_t)( 'T'))<< 24)|(((uint32_t)( 'e'))<< 16)|(((uint32_t)( 's'))<< 8)|(((uint32_t)( 't'))<< 0)))
NTV2Standard GetNTV2StandardFromVideoFormat(const NTV2VideoFormat inVideoFormat)
NTV2PixelFormat fPixelFormat
The pixel format to use.
enum NTV2OutputCrosspointID NTV2OutputXptID
UWord NTV2DeviceGetNumVideoOutputs(const NTV2DeviceID inDeviceID)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=true)
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
#define AJA_FAILURE(_status_)
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
@ NTV2_OUTPUTDESTINATION_ANALOG
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
AJAStatus SetUpVideo(void)
Sets up my AJA device to play video.
AJALabelValuePairs Get(const bool inCompact=false) const
NTV2TestPatternGen & setVANCToLegalBlack(const bool inClearVANC)
Changes my "clear VANC lines to legal black" setting.
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.