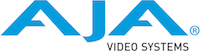 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
18 #define NTV2_AUDIOSIZE_MAX (401 * 1024)
25 const bool inQuadMode,
26 const uint32_t inAudioChannels,
27 const bool inTimeCodeBurn,
28 const bool inInfoData,
29 const uint32_t inMaxFrames)
40 mDeviceSpecifier (inDeviceSpecifier),
41 mWithAudio (inAudioChannels != 0),
42 mInputChannel (inChannel),
47 mPixelFormat (inPixelFormat),
51 mWithInfo (inInfoData),
52 mWithAnc (inTimeCodeBurn),
55 mNumAudioChannels (0),
56 mFileAudioChannels (inAudioChannels),
57 mMaxFrames (inMaxFrames),
59 mLastFrameInput (
false),
60 mLastFrameRaw (
false),
61 mLastFrameHevc (
false),
62 mLastFrameVideo (
false),
63 mLastFrameAudio (
false),
65 mVideoInputFrameCount (0),
66 mVideoProcessFrameCount (0),
67 mCodecRawFrameCount (0),
68 mCodecHevcFrameCount (0),
69 mVideoFileFrameCount (0),
70 mAudioFileFrameCount (0)
72 ::memset (mVideoInputBuffer, 0x0,
sizeof (mVideoInputBuffer));
73 ::memset (mVideoRawBuffer, 0x0,
sizeof (mVideoRawBuffer));
74 ::memset (mVideoHevcBuffer, 0x0,
sizeof (mVideoHevcBuffer));
75 ::memset (mAudioInputBuffer, 0x0,
sizeof (mAudioInputBuffer));
103 if (mVideoInputBuffer[bufferNdx].pVideoBuffer)
108 if (mVideoInputBuffer[bufferNdx].pInfoBuffer)
110 delete [] mVideoInputBuffer[bufferNdx].
pInfoBuffer;
113 if (mVideoInputBuffer[bufferNdx].pAudioBuffer)
119 if (mVideoRawBuffer[bufferNdx].pVideoBuffer)
124 if (mVideoRawBuffer[bufferNdx].pInfoBuffer)
129 if (mVideoRawBuffer[bufferNdx].pAudioBuffer)
135 if (mVideoHevcBuffer[bufferNdx].pVideoBuffer)
140 if (mVideoHevcBuffer[bufferNdx].pInfoBuffer)
145 if (mVideoHevcBuffer[bufferNdx].pAudioBuffer)
156 if (mAudioInputBuffer[bufferNdx].pVideoBuffer)
161 if (mAudioInputBuffer[bufferNdx].pInfoBuffer)
163 delete [] mAudioInputBuffer[bufferNdx].
pInfoBuffer;
166 if (mAudioInputBuffer[bufferNdx].pAudioBuffer)
178 if (mM31 && !mLastFrame && !mGlobalQuit)
186 for (i = 0; i < timeout; i++)
188 if (mLastFrameVideo && (!mWithAudio || mLastFrameAudio))
break;
192 { cerr <<
"## ERROR: Wait for last frame timeout" << endl; }
196 { cerr <<
"## ERROR: ChangeEHState ready to stop failed" << endl; }
199 { cerr <<
"## ERROR: ChangeEHState stop failed" << endl; }
203 { cerr <<
"## ERROR: ChangeVInState stop failed" << endl; }
209 { cerr <<
"## ERROR: ChangeMainState to init failed" << endl; }
216 while (mVideoInputThread.
Active())
219 while (mVideoProcessThread.
Active())
222 while (mCodecRawThread.
Active())
225 while (mCodecHevcThread.
Active())
228 while (mVideoFileThread.
Active())
231 while (mAudioFileThread.
Active())
260 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return AJA_STATUS_OPEN; }
276 cerr <<
"## ERROR: M31 not found" << endl;
281 mM31 =
new CNTV2m31 (&mDevice);
293 if (CNTV2m31::IsPresetVIF(mPreset))
297 mVideoFormat = CNTV2m31::GetPresetVideoFormat(mPreset);
298 mPixelFormat = CNTV2m31::GetPresetFrameBufferFormat(mPreset);
299 mQuad = CNTV2m31::IsPresetUHD(mPreset);
300 mInterlaced = CNTV2m31::IsPresetInterlaced(mPreset);
317 switch (mInputChannel)
339 if(!CNTV2m31::ConvertVideoFormatToPreset(mVideoFormat, mPixelFormat,
false, mPreset))
342 mQuad = CNTV2m31::IsPresetUHD(mPreset);
343 mInterlaced = CNTV2m31::IsPresetInterlaced(mPreset);
363 status = mHevcCommon->
SetupHEVC (mM31, mPreset, mEncodeChannel, mMultiStream, mWithInfo);
372 ostringstream fileName;
374 fileName <<
"raw_" << (mInputChannel+1) <<
".hevc";
376 fileName <<
"raw.hevc";
377 status = mHevcCommon->
CreateHevcFile (fileName.str(), mMaxFrames);
385 ostringstream fileName;
387 fileName <<
"raw_" << (mInputChannel+1) <<
".txt";
389 fileName <<
"raw.txt";
390 status = mHevcCommon->
CreateEncFile (fileName.str(), mMaxFrames);
398 ostringstream fileName;
400 fileName <<
"raw_" << (mInputChannel+1) <<
".aiff";
402 fileName <<
"raw.aiff";
467 else if (mMultiStream)
474 mDevice.
SetVideoFormat (mVideoFormat,
false,
false, mInputChannel);
576 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
579 mVideoInputBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
583 mVideoInputBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
587 mVideoInputCircularBuffer.
Add (& mVideoInputBuffer[bufferNdx]);
592 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
595 mVideoRawBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
599 mVideoRawBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
603 mVideoRawCircularBuffer.
Add (& mVideoRawBuffer[bufferNdx]);
608 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
611 mVideoHevcBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
615 mVideoHevcBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mEncInfoBufferSize/4];
619 mVideoHevcCircularBuffer.
Add (& mVideoHevcBuffer[bufferNdx]);
626 for (
unsigned bufferNdx = 0; bufferNdx <
AUDIO_RING_SIZE; bufferNdx++ )
629 mAudioInputBuffer[bufferNdx].
pAudioBuffer =
new uint32_t [mAudioBufferSize/4];
632 mAudioInputCircularBuffer.
Add (& mAudioInputBuffer[bufferNdx]);
709 cout << endl <<
"## WARNING: No video signal present on the input connector" << endl;
732 mVideoInputThread.
Start();
755 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
797 if (mWithAudio && pAudioData)
827 pPicData->serialNumber = mVideoInputFrameCount;
828 pPicData->ptsValueLow = (uint32_t)(pts & 0xffffffff);
829 pPicData->ptsValueHigh = (uint32_t)((pts >> 32) & 0x1);
830 pPicData->pictureNumber = mVideoInputFrameCount + 1;
837 pPicData->serialNumber = mVideoInputFrameCount*2;
838 pPicData->pictureNumber = mVideoInputFrameCount*2 + 1;
848 pPicData->serialNumber = mVideoInputFrameCount*2 + 1;
849 pPicData->ptsValueLow = (uint32_t)(pts & 0xffffffff);
850 pPicData->ptsValueHigh = (uint32_t)((pts >> 32) & 0x1);
851 pPicData->pictureNumber = mVideoInputFrameCount*2 + 2;
858 if(pVideoData->
lastFrame && !mLastFrameInput)
860 printf (
"\nCapture last frame number %d\n", mVideoInputFrameCount );
861 mLastFrameInput =
true;
864 mVideoInputFrameCount++;
866 if (mWithAudio && pAudioData)
897 mVideoProcessThread.
Start();
928 mVideoProcessFrameCount++;
948 mCodecRawThread.
Start();
971 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
974 m31 =
new CNTV2m31 (&ntv2Device);
989 m31->RawTransfer(mPreset, mEncodeChannel,
996 m31->RawTransfer(mPreset, mEncodeChannel,
1005 m31->RawTransfer(mPreset, mEncodeChannel,
1010 m31->RawTransfer(mPreset, mEncodeChannel,
1020 m31->RawTransfer(mEncodeChannel,
1029 m31->RawTransfer(mEncodeChannel,
1037 mLastFrameRaw =
true;
1040 mCodecRawFrameCount++;
1057 mCodecHevcThread.
Start();
1080 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
1083 m31 =
new CNTV2m31 (&ntv2Device);
1085 while (!mGlobalQuit)
1091 if (!mLastFrameHevc)
1096 uint8_t* pVideoBuffer = (uint8_t*)pFrameData->
pVideoBuffer;
1097 uint8_t* pInfoBuffer = (uint8_t*)pFrameData->
pInfoBuffer;
1102 m31->EncTransfer(mEncodeChannel,
1129 m31->EncTransfer(mEncodeChannel,
1152 m31->EncTransfer(mEncodeChannel,
1175 mLastFrameHevc =
true;
1178 mCodecHevcFrameCount++;
1195 mVideoFileThread.
Start();
1213 while (!mGlobalQuit)
1219 if (!mLastFrameVideo)
1234 printf (
"Video file last frame number %d\n", mVideoFileFrameCount );
1235 mLastFrameVideo =
true;
1238 mVideoFileFrameCount++;
1254 mAudioFileThread.
Start();
1272 while (!mGlobalQuit)
1278 if (!mLastFrameAudio)
1285 printf (
"Audio file last frame number %d\n", mAudioFileFrameCount );
1286 mLastFrameAudio =
true;
1290 mAudioFileFrameCount++;
1332 std::string timeString;
1333 mTimeCode.
Set(frameNumber);
1335 mTimeCode.
QueryString(timeString, mTimeBase,
false);
1338 mTimeCode.
QueryString(timeString, mTimeBase,
false);
@ NTV2_XptFrameBuffer6YUV
void WriteAiffData(void *pBuffer, uint32_t numChannels, uint32_t numSamples)
virtual bool SetSDIInLevelBtoLevelAConversion(const NTV2ChannelSet &inSDIInputs, const bool inEnable)
Enables or disables 3G level B to 3G level A conversion at the SDI input(s).
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
virtual void StartCodecRawThread(void)
Start the codec raw thread.
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
void QueryString(std::string &str, const AJATimeBase &timeBase, bool bDropFrame)
virtual void CodecHevcWorker(void)
Repeatedly transfers hevc frames from the codec and adds them to the hevc ring.
virtual void StartVideoProcessThread(void)
Start the video process thread.
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
ULWord GetCapturedAudioByteCount(void) const
AJA_PixelFormat GetAJAPixelFormat(NTV2FrameBufferFormat pixelFormat)
uint32_t timeCodeLow
Time code data low.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
virtual bool SetEmbeddedAudioClock(const NTV2EmbeddedAudioClock inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2EmbeddedAudioClock setting for the given NTV2AudioSystem.
Declares device capability functions.
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
uint32_t videoDataSize2
Size of field 2 video data (bytes)
uint32_t audioDataSize
Size of audio data (bytes)
virtual bool ApplySignalRoute(const CNTV2SignalRouter &inRouter, const bool inReplace=false)
Applies the given routing table to the AJA device.
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
void WriteHevcData(void *pBuffer, uint32_t bufferSize)
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
static void VideoFileThreadStatic(AJAThread *pThread, void *pContext)
This is the video file writer thread's static callback function that gets called when the thread star...
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
bool lastFrame
Indicates last captured frame.
Declares the AJATime class.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
@ NTV2_XptFrameBuffer8YUV
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
ULWord GetProcessedFrameCount(void) const
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
uint32_t * pVideoBuffer
Pointer to host video buffer.
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
void WriteEncData(void *pBuffer, uint32_t bufferSize)
ULWord GetBufferLevel(void) const
bool GetInputTimeCode(NTV2_RP188 &outTimeCode, const NTV2TCIndex inTCIndex=NTV2_TCINDEX_SDI1) const
Intended for capture, answers with a specific timecode captured in my acTransferStatus member's acFra...
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
static void VideoProcessThreadStatic(AJAThread *pThread, void *pContext)
This is the video process thread's static callback function that gets called when the thread starts....
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
@ NTV2_XptFrameBuffer4Input
AJAStatus DetermineInputFormat(NTV2VideoFormat sdiFormat, bool quad, NTV2VideoFormat &videoFormat)
@ NTV2_XptFrameBuffer3Input
uint32_t infoDataSize
Size of the information data (bytes)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual void SetupAutoCirculate(void)
Initializes AutoCirculate.
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
virtual AJAStatus ProcessVideoFrame(AVHevcDataBuffer *pSrcFrame, AVHevcDataBuffer *pDstFrame, uint32_t frameNumber)
Default do-nothing function for processing the captured frames.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
ULWord GetDisplayHeight(const NTV2VideoFormat videoFormat)
uint32_t * pInfoBuffer
Picture information (raw) or encode information (hevc)
uint32_t infoDataSize2
Size of the field 2 information data (bytes)
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
virtual void CodecRawWorker(void)
Repeatedly removes video frames from the raw video ring and transfers them to the codec.
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
static void CodecRawThreadStatic(AJAThread *pThread, void *pContext)
This is the codec raw thread's static callback function that gets called when the thread starts....
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
virtual void GetStatus(AVHevcStatus &outStatus)
Provides status information about my input (capture) process.
uint32_t videoDataSize
Size of video data (bytes)
void SetAJAFrameRate(AJA_FrameRate ajaFrameRate)
ULWord fLo
| BG 4 | Secs10 | BG 3 | Secs 1 | BG 2 | Frms10 | BG 1 | Frms 1 |
virtual void StartVideoFileThread(void)
Start the video file writer thread.
Declares the AJAProcess class.
ULWord fHi
| BG 8 | Hrs 10 | BG 7 | Hrs 1 | BG 6 | Mins10 | BG 5 | Mins 1 |
@ NTV2_XptFrameBuffer1Input
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
NTV2EmbeddedAudioInput NTV2ChannelToEmbeddedAudioInput(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2EmbeddedAudioInput.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
Declares the NTV2EncodeHEVCFileAc class.
virtual AJAStatus Run(void)
Runs me.
void SetRP188(const uint32_t inDBB, const uint32_t inLo, const uint32_t inHi, const AJATimeBase &inTimeBase)
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
@ NTV2_MAX_NUM_VIDEO_FORMATS
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
@ NTV2_EMBEDDED_AUDIO_CLOCK_VIDEO_INPUT
Audio clock derived from the video input.
Declares numerous NTV2 utility functions.
uint32_t * pAudioBuffer
Pointer to host audio buffer.
void SetStdTimecodeForHfr(bool bStdTc)
virtual void VideoProcessWorker(void)
Repeatedly removes video frames from the video input ring, calls a custom video process method and ad...
static void CodecHevcThreadStatic(AJAThread *pThread, void *pContext)
This is the codec hevc thread's static callback function that gets called when the thread starts....
I interrogate and control an AJA video/audio capture/playout device.
virtual void StartVideoInputThread(void)
Start the video input thread.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
struct HevcPictureInfo HevcPictureInfo
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
ULWord GetVideoActiveSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
AJAStatus CreateEncFile(const std::string &inFileName, uint32_t maxFrames)
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
bool NTV2DeviceHasHEVCM31(const NTV2DeviceID inDeviceID)
struct HevcEncodedInfo HevcEncodedInfo
This class is a collection of widget input-to-output connections that can be applied all-at-once to a...
@ NTV2_STANDARD_TASKS
1: Standard/Retail: Device is completely controlled by AJA ControlPanel, service/daemon,...
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
uint32_t timeCodeDBB
Time code data dbb.
static void VideoInputThreadStatic(AJAThread *pThread, void *pContext)
This is the video input thread's static callback function that gets called when the thread starts....
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
struct HevcPictureData HevcPictureData
virtual void RouteInputSignal(void)
Sets up device routing for capture.
@ NTV2_XptFrameBuffer2Input
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
bool IsRunning(void) const
#define NTV2_AUDIOSIZE_MAX
@ NTV2_MODE_CAPTURE
Capture (input) mode, which writes into device SDRAM.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
This struct replaces the old RP188_STRUCT.
virtual void StartCodecHevcThread(void)
Start the codec hevc thread.
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
static void AudioFileThreadStatic(AJAThread *pThread, void *pContext)
This is the audio file writer thread's static callback function that gets called when the thread star...
@ NTV2_XptFrameBuffer7YUV
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
ULWord GetDroppedFrameCount(void) const
int64_t FramesToMicroseconds(int64_t frames, bool round=false) const
virtual NTV2VideoFormat GetSDIInputVideoFormat(NTV2Channel inChannel, bool inIsProgressive=false)
Returns the video format of the signal that is present on the given SDI input source.
virtual ~NTV2EncodeHEVCFileAc()
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
AJAStatus CreateAiffFile(const std::string &inFileName, uint32_t numChannels, uint32_t maxFrames, uint32_t bufferSize)
@ Hevc_EhState_ReadyToStop
virtual void StartAudioFileThread(void)
Start the audio file writer thread.
HevcPictureData pictureData
ULWord GetDisplayWidth(const NTV2VideoFormat videoFormat)
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual void AudioFileWorker(void)
Repeatedly removes audio samples from the audio input ring and writes them to the audio output file.
virtual void VideoFileWorker(void)
Repeatedly removes hevc frame from the hevc ring and writes them to the hevc output file.
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=false)
Returns the video format of the signal that is present on the given input source.
AJAStatus CreateHevcFile(const std::string &inFileName, uint32_t maxFrames)
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
uint32_t videoBufferSize
Size of host video buffer (bytes)
virtual void VideoInputWorker(void)
Repeatedly captures video frames using AutoCirculate and add them to the video input ring.
uint32_t timeCodeHigh
Time code data high.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
uint32_t infoBufferSize
Size of the host information buffer (bytes)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
uint32_t AlignDataBuffer(void *pBuffer, uint32_t bufferSize, uint32_t dataSize, uint32_t alignBytes, uint8_t fill)
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
virtual AJAStatus Start()
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=true)
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
AJA_FrameRate GetAJAFrameRate(NTV2FrameRate frameRate)
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
This structure encapsulates the video and audio buffers used by the HEVC demo applications....
#define AJA_FAILURE(_status_)
@ NTV2_XptFrameBuffer5YUV
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
virtual bool AddConnection(const NTV2InputXptID inSignalInput, const NTV2OutputXptID inSignalOutput=NTV2_XptBlack)
Adds a connection between a widget's signal input (sink) and another widget's signal output (source).
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
virtual bool GetSDIInput3GbPresent(bool &outValue, const NTV2Channel channel)
bool SetBuffers(ULWord *pInVideoBuffer, const ULWord inVideoByteCount, ULWord *pInAudioBuffer, const ULWord inAudioByteCount, ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
NTV2EncodeHEVCFileAc(const std::string inDeviceSpecifier="0", const NTV2Channel inChannel=NTV2_CHANNEL1, const M31VideoPreset inM31Preset=M31_FILE_1280X720_420_8_5994p, const NTV2FrameBufferFormat inPixelFormat=NTV2_FBF_10BIT_YCBCR_420PL2, const bool inQuadMode=(0), const uint32_t inAudioChannels=0, const bool inTimeCodeBurn=(0), const bool inInfoData=(0), const uint32_t inMaxFrames=0xffffffff)
Constructs me using the given settings.
uint32_t audioBufferSize
Size of host audio buffer (bytes)
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
AJAStatus SetupHEVC(CNTV2m31 *pM31, M31VideoPreset preset, M31Channel encodeChannel, bool multiStream, bool withInfo)
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID
virtual void Quit(void)
Gracefully stops me from running.