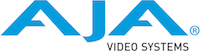 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
22 #define TCFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
23 #define TCWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
24 #define TCNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
25 #define TCINFO(_expr_) AJA_sINFO (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
26 #define TCDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_TimecodeGeneric, AJAFUNC << ": " << _expr_)
54 const bool inWithAudio,
58 const bool inDoMultiChannel,
66 mToneFrequency (440.0),
68 mDeviceSpecifier (inDeviceSpecifier),
70 mOutputChannel (inChannel),
71 mVideoFormat (inVideoFormat),
72 mPixelFormat (inPixelFormat),
76 mWithAudio (inWithAudio),
78 mDoMultiChannel (inDoMultiChannel),
90 mDolbyFile (inDolbyFile),
95 ::memset (mAVHostBuffer, 0,
sizeof (mAVHostBuffer));
107 delete mConsumerThread;
109 delete mProducerThread;
112 if (mTestPatternVideoBuffers)
114 for (uint32_t ndx(0); ndx < mNumTestPatterns; ndx++)
115 delete [] mTestPatternVideoBuffers [ndx];
116 delete [] mTestPatternVideoBuffers;
117 mTestPatternVideoBuffers =
AJA_NULL;
118 mNumTestPatterns = 0;
123 if (mAVHostBuffer [ndx].fVideoBuffer)
128 if (mAVHostBuffer [ndx].fAudioBuffer)
137 delete [] mDolbyBuffer;
143 delete [] mBurstBuffer;
147 if (!mDoMultiChannel && mDevice.IsOpen())
161 while (mProducerThread->
Active ())
165 while (mConsumerThread->
Active ())
180 {cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return AJA_STATUS_OPEN;}
183 {cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not ready" << endl;
return AJA_STATUS_INITIALIZE;}
185 if (!mDoMultiChannel)
207 cerr <<
"## ERROR: Cannot use channel '" << mOutputChannel+1 <<
"' -- device only supports channel 1"
248 mDevice.
SetVideoFormat (mVideoFormat,
false,
false, mOutputChannel);
282 const uint16_t numberOfAudioChannels (8);
326 mAVHostBuffer [ndx].
fVideoBuffer =
reinterpret_cast <uint32_t *
> (
new uint8_t [mVideoBufferSize]);
328 mAVHostBuffer [ndx].
fAudioBuffer = mWithAudio ?
reinterpret_cast<uint32_t*
>(
new uint8_t[mAudioBufferSize]) :
AJA_NULL;
331 ::memset (mAVHostBuffer [ndx].fVideoBuffer, 0x00, mVideoBufferSize);
332 ::memset (mAVHostBuffer [ndx].fAudioBuffer, 0x00, mWithAudio ? mAudioBufferSize : 0);
334 mAVCircularBuffer.
Add (&mAVHostBuffer [ndx]);
337 if (mDolbyFile !=
NULL)
340 mBurstSamples = 6144;
341 mBurstMax = mBurstSamples * 2;
342 mBurstBuffer =
new uint16_t [mBurstMax];
343 mDolbyBuffer =
new uint16_t [mBurstMax];
384 mConsumerThread->
Start ();
436 if (isPAL) tcF2.
fHi |=
BIT(27);
else tcF2.
fLo |=
BIT(27);
438 TCDBG(
"Playing " << tcF1);
454 PLNOTE(
"Thread completed, will exit");
469 mProducerThread->
Start ();
497 mTestPatternVideoBuffers =
new uint8_t * [mNumTestPatterns];
498 ::memset (mTestPatternVideoBuffers, 0, mNumTestPatterns *
sizeof(uint8_t *));
501 for (uint32_t testPatternIndex(0); testPatternIndex < mNumTestPatterns; testPatternIndex++)
504 mTestPatternVideoBuffers [testPatternIndex] =
new uint8_t[mVideoBufferSize];
509 NTV2Buffer vidBuffer (mTestPatternVideoBuffers [testPatternIndex], mVideoBufferSize);
511 if (!testPatternGen.
DrawTestPattern (testPatternTypes[testPatternIndex], formatDesc, vidBuffer))
513 cerr <<
"## ERROR: DrawTestPattern failed, formatDesc: " << formatDesc << endl;
526 static const double gAmplitudes [] = { 0.10, 0.15, 0.20, 0.25, 0.30, 0.35, 0.40, 0.45, 0.50, 0.55, 0.60, 0.65, 0.70, 0.75, 0.80, 0.85,
527 0.85, 0.80, 0.75, 0.70, 0.65, 0.60, 0.55, 0.50, 0.45, 0.40, 0.35, 0.30, 0.25, 0.20, 0.15, 0.10};
532 ULWord frequencyIndex (0);
533 double timeOfLastSwitch (0.0);
534 ULWord testPatternIndex (0);
552 ::memcpy (frameData->
fVideoBuffer, mTestPatternVideoBuffers [testPatternIndex], mVideoBufferSize);
556 const CRP188 rp188Info (mCurrentFrame++, 0, 0, 10, tcFormat);
557 string timeCodeString;
567 if (mDolbyFile !=
NULL)
581 if (currentTime > timeOfLastSwitch + 4.0)
584 testPatternIndex = (testPatternIndex + 1) % mNumTestPatterns;
586 timeOfLastSwitch = currentTime;
587 PLINFO(
"F" <<
DEC0N(mCurrentFrame,6) <<
": " << timeCodeString <<
": tone=" << mToneFrequency
588 <<
"Hz, pattern='" << tpNames.at(testPatternIndex) <<
"'");
595 PLNOTE(
"Thread completed, will exit");
622 pFrequencies [0] = (mToneFrequency / 2.0);
623 for (
ULWord chan (1); chan < numChannels; chan++)
626 pFrequencies [chan] = pFrequencies [chan - 1] * 1.154782;
632 const double sampleRateHertz (audioRate ==
NTV2_AUDIO_192K ? 192000.0 : 48000.0);
647 ULWord* audioBuffer (pInAudioBuffer);
661 for (uint32_t i = 0; i < numSamples; i++)
663 for (uint32_t j = 0; j < numChannels; j++)
665 *audioBuffer = ((uint32_t)mRampSample) << 16;
671 return numSamples * numChannels * 4;
674 #ifdef DOLBY_FULL_PARSER
690 if ((mDolbyFile ==
NULL) || (mDolbyBuffer ==
NULL))
694 while (sampleOffset < numSamples)
696 if ((mBurstSize != 0) && (mBurstIndex < mBurstSamples))
698 if(mBurstOffset < mBurstSize)
702 if (mBurstIndex == 0)
708 else if (mBurstIndex == 1)
711 data0 = ((bsi.
bsmod & 0x7) << 8) | 0x0015;
712 data1 = mBurstSize * 2;
717 data0 = (uint32_t)(mBurstBuffer[mBurstOffset]);
718 data0 = ((data0 & 0x00ff) << 8) | ((data0 & 0xff00) >> 8);
720 data1 = (uint32_t)(mBurstBuffer[mBurstOffset]);
721 data1 = ((data1 & 0x00ff) << 8) | ((data1 & 0xff00) >> 8);
728 for (
ULWord i = 0; i < numChannels; i += 2)
730 pInAudioBuffer[sampleOffset * numChannels + i] = data0;
731 pInAudioBuffer[sampleOffset * numChannels + i + 1] = data1;
737 for (
ULWord i = 0; i < numChannels; i++)
739 pInAudioBuffer[sampleOffset * numChannels + i] = 0;
761 cerr <<
"## ERROR: Dolby frame not found" << endl;
766 if (!
ParseBSI(&mDolbyBuffer[1], sampleCount - 1, &bsi))
776 mDolbySize = sampleCount;
779 case 0: mDolbyBlocks = 1;
break;
780 case 1: mDolbyBlocks = 2;
break;
781 case 2: mDolbyBlocks = 3;
break;
782 case 3: mDolbyBlocks = 6;
break;
783 default:
goto silence;
787 while (numBlocks <= 6)
790 while (dolbyOffset < mDolbySize)
793 if (burstOffset >= mBurstMax)
795 cerr <<
"## ERROR: Dolby burst too large" << endl;
801 mBurstBuffer[burstOffset] = mDolbyBuffer[dolbyOffset];
812 cerr <<
"## ERROR: Dolby frame not found" << endl;
819 if (!
ParseBSI(&mDolbyBuffer[1], sampleCount - 1, &bsi))
825 cerr <<
"## ERROR: Dolby frame bad bsid = " << bsi.
bsid << endl;
829 mDolbySize = sampleCount;
836 numBlocks += mDolbyBlocks;
840 case 0: mDolbyBlocks = 1;
break;
841 case 1: mDolbyBlocks = 2;
break;
842 case 2: mDolbyBlocks = 3;
break;
843 case 3: mDolbyBlocks = 6;
break;
845 cerr <<
"## ERROR: Dolby frame bad numblkscod = " << bsi.
numblkscod << endl;
857 cerr <<
"## ERROR: Dolby frame unexpected convsync = " << bsi.
convsync << endl;
863 mBurstSize = burstOffset;
870 return numSamples * numChannels * 4;
874 memset(&pInAudioBuffer[sampleOffset * numChannels], 0, (numSamples - sampleOffset) * numChannels * 4);
875 return numSamples * numChannels * 4;
886 bytes = mDolbyFile->
Read((uint8_t*)(&pInDolbyBuffer[0]), 2);
895 if ((mDolbyBuffer[0] == 0x7705) ||
896 (mDolbyBuffer[0] == 0x770b))
901 bytes = mDolbyFile->
Read((uint8_t*)(&pInDolbyBuffer[1]), 4);
906 uint32_t size = (uint32_t)mDolbyBuffer[1];
907 size = (((size & 0x00ff) << 8) | ((size & 0xff00) >> 8));
908 size = (size & 0x7ff) + 1;
911 uint32_t len = (size - 3) * 2;
912 bytes = mDolbyFile->
Read((uint8_t*)(&pInDolbyBuffer[3]), len);
924 if ((pInDolbyBuffer ==
NULL) || (pBsi ==
NULL))
945 if (pBsi->
acmod == 0x0)
965 if (pBsi->
acmod > 0x2)
969 if ((pBsi->
acmod & 0x1) && (pBsi->
acmod > 0x2))
974 if (pBsi->
acmod & 0x4)
994 if (pBsi->
acmod == 0x0)
1014 else if (pBsi->
mixdef == 0x2)
1018 else if (pBsi->
mixdef == 0x3)
1096 uint32_t size = 8 * (pBsi->
mixdeflen + 2);
1097 size = (size + 7) / 8 * 8;
1101 if (!
GetBits(data, (size > 8)? 8 : size))
return false;
1107 if (pBsi->
acmod < 0x2)
1115 if (pBsi->
acmod == 0x0)
1144 for(blk = 0; blk < numblk; blk++)
1162 if (pBsi->
acmod == 0x2)
1167 if (pBsi->
acmod >= 0x6)
1178 if (pBsi->
acmod == 0x0)
1188 if (pBsi->
fscod < 0x3)
1218 uint32_t size = 8 * (pBsi->
addbsil + 1);
1222 if (!
GetBits(data, 8))
return false;
1234 mBitBuffer = pBuffer;
1242 static uint8_t bitMask[] = { 0x00, 0x01, 0x03, 0x07, 0x0f, 0x1f, 0x3f, 0x7f, 0xff };
1262 data |= ((*mBitBuffer >> mBitIndex) & bitMask[cb]) << bits;
1282 if ((mDolbyFile ==
NULL) || (mDolbyBuffer ==
NULL))
1286 while (sampleOffset < numSamples)
1289 if (mBurstIndex >= mBurstSamples)
1293 if (mBurstIndex == 0)
1296 uint32_t bytes = mDolbyFile->
Read((uint8_t*)(&mDolbyBuffer[0]), 2);
1301 bytes = mDolbyFile->
Read((uint8_t*)(&mDolbyBuffer[0]), 2);
1307 if ((mDolbyBuffer[0] != 0x7705) &&
1308 (mDolbyBuffer[0] != 0x770b))
1312 bytes = mDolbyFile->
Read((uint8_t*)(&mDolbyBuffer[1]), 4);
1317 uint32_t size = (uint32_t)mDolbyBuffer[1];
1318 size = (((size & 0x00ff) << 8) | ((size & 0xff00) >> 8)) + 1;
1321 uint32_t len = (size - 3) * 2;
1322 bytes = mDolbyFile->
Read((uint8_t*)(&mDolbyBuffer[3]), len);
1332 if (mBurstOffset < mBurstSize)
1336 if (mBurstIndex == 0)
1342 else if (mBurstIndex == 1)
1346 data1 = mBurstSize * 2;
1351 data0 = (uint32_t)(mDolbyBuffer[mBurstOffset]);
1352 data0 = ((data0 & 0x00ff) << 8) | ((data0 & 0xff00) >> 8);
1354 data1 = (uint32_t)(mDolbyBuffer[mBurstOffset]);
1355 data1 = ((data1 & 0x00ff) << 8) | ((data1 & 0xff00) >> 8);
1362 for (
ULWord i = 0; i < numChannels; i += 2)
1364 pInAudioBuffer[sampleOffset * numChannels + i] = data0;
1365 pInAudioBuffer[sampleOffset * numChannels + i + 1] = data1;
1371 for (
ULWord i = 0; i < numChannels; i++)
1373 pInAudioBuffer[sampleOffset * numChannels + i] = 0;
1381 return numSamples * numChannels * 4;
1385 memset(&pInAudioBuffer[sampleOffset * numChannels], 0, (numSamples - sampleOffset) * numChannels * 4);
1386 return numSamples * numChannels * 4;
virtual bool DrawTestPattern(const std::string &inTPName, const NTV2FormatDescriptor &inFormatDesc, NTV2Buffer &inBuffer)
Renders the given test pattern or color into a host raster buffer.
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
virtual uint32_t AddTone(ULWord *audioBuffer)
Inserts audio tone (based on my current tone frequency) into the given audio buffer.
virtual ~NTV2DolbyPlayer(void)
ULWord acFramesProcessed
Total number of frames successfully processed since CNTV2Card::AutoCirculateStart called.
virtual AJAStatus SetUpTestPatternVideoBuffers(void)
Creates my test pattern buffers.
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
@ NTV2_TestPatt_CheckField
@ NTV2_REFERENCE_SFP1_PTP
Specifies the PTP source on SFP 1.
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
virtual void RouteOutputSignal(void)
Sets up device routing for playout.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
RP188_STRUCT fRP188Data
For future use.
@ NTV2_TestPatt_MultiBurst
NTV2TestPatternSelect
Identifies a predefined NTV2 test pattern.
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
bool GetRP188Reg(RP188_STRUCT &outRP188) const
#define NTV2_VIDEO_FORMAT_HAS_PROGRESSIVE_PICTURE(__f__)
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
This structure encapsulates the video, audio and anc buffers used in the AutoCirculate demos....
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
Declares the AJATime class.
@ NTV2_AUDIO_FORMAT_DOLBY
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
static const uint32_t AUDIOBYTES_MAX_48K(201 *1024)
The maximum number of bytes of 48KHz audio that can be transferred for a single frame....
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
Declares the NTV2TestPatternGen class.
@ NTV2_TestPatt_ColorBars100
virtual void PlayFrames(void)
Repeatedly plays out frames using AutoCirculate (until quit).
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
#define DEC0N(__x__, __n__)
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
uint8_t mixdatabuffer[64]
NTV2DolbyPlayer(const std::string &inDeviceSpecifier="0", const bool inWithAudio=true, const NTV2Channel inChannel=NTV2_CHANNEL1, const NTV2FrameBufferFormat inPixelFormat=NTV2_FBF_8BIT_YCBCR, const NTV2VideoFormat inVideoFormat=NTV2_FORMAT_1080i_5994, const bool inDoMultiFormat=false, const bool inDoRamp=false, AJAFileIO *inDolbyFile=NULL)
Constructs me using the given configuration settings.
virtual AJAStatus Run(void)
Runs me.
bool NTV2DeviceCanDoFrameStore1Display(const NTV2DeviceID inDeviceID)
Header file for NTV2DolbyPlayer demonstration class.
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
bool NTV2DeviceCanDoFrameBufferFormat(const NTV2DeviceID inDeviceID, const NTV2FrameBufferFormat inFBFormat)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual void SetBitBuffer(uint8_t *pBuffer, uint32_t size)
Set the bitstream buffer for bit retrieval.
ULWord GetNumAvailableOutputFrames(void) const
const char * NTV2FrameBufferFormatString(NTV2FrameBufferFormat fmt)
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
NTV2FrameRate
Identifies a particular video frame rate.
bool NTV2DeviceCanDoVideoFormat(const NTV2DeviceID inDeviceID, const NTV2VideoFormat inVideoFormat)
virtual uint32_t AddDolby(ULWord *audioBuffer)
Inserts dolby audio into the given audio buffer.
NTV2InputXptID GetOutputDestInputXpt(const NTV2OutputDestination inOutputDest, const bool inIsSDI_DS2=false, const UWord inHDMI_Quadrant=99)
virtual AJAStatus SetUpAudio(void)
Sets up everything I need to play audio.
static const ULWord gNumFrequencies(sizeof(gFrequencies)/sizeof(double))
ULWord fLo
| BG 4 | Secs10 | BG 3 | Secs 1 | BG 2 | Frms10 | BG 1 | Frms 1 |
I am an object that can play out a test pattern (with timecode) to an output of an AJA device with or...
Declares the AJAProcess class.
virtual AJAStatus SetUpVideo(void)
Sets up everything I need to play video.
ULWord fHi
| BG 8 | Hrs 10 | BG 7 | Hrs 1 | BG 6 | Mins10 | BG 5 | Mins 1 |
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool GetNumberAudioChannels(ULWord &outNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current number of audio channels being captured or played by a given Audio System on the ...
virtual bool GetVideoFormat(NTV2VideoFormat &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
@ NTV2_TestPatt_FlatField
AJAStatus Seek(const int64_t distance, const AJAFileSetFlag flag) const
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
uint32_t fAudioBufferSize
Size of host audio buffer, in bytes.
virtual void SetUpHostBuffers(void)
Sets up my circular buffers.
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=false)
Connects the given widget signal input (sink) to the given widget signal output (source).
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
#define NTV2_IS_PAL_VIDEO_FORMAT(__f__)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
Declares numerous NTV2 utility functions.
uint32_t blkmixcfginfo[6]
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
UWord NTV2DeviceGetNumFrameStores(const NTV2DeviceID inDeviceID)
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
@ NTV2_TestPatt_ColorBars75
virtual bool IsDeviceReady(const bool inCheckValid=(0))
ULWord GetVideoWriteSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
Identical to the GetVideoActiveSize function, except rounds the result up to the nearest 4K page size...
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
ULWord acBufferLevel
Number of buffered frames in driver ready to capture or play.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
virtual void ProduceFrames(void)
Repeatedly produces test pattern frames (until global quit flag set).
virtual bool SetHDMIOutAudioRate(const NTV2AudioRate inNewValue)
Sets the HDMI output's audio rate.
bool AddAudioTone(ULWord &outNumBytesWritten, NTV2Buffer &inAudioBuffer, ULWord &inOutCurrentSample, const ULWord inNumSamples, const double inSampleRate, const double inAmplitude, const double inFrequency, const ULWord inNumBits, const bool inByteSwap, const ULWord inNumChannels)
Fills the given buffer with 32-bit (ULWord) audio tone samples.
@ NTV2_DISABLE_TASKS
0: Disabled: Device is completely configured by controlling application(s) – no driver involvement.
static AJA_FrameRate GetAJAFrameRate(const NTV2FrameRate inFrameRate)
uint32_t * fAudioBuffer
Pointer to host audio buffer.
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
uint32_t * fVideoBuffer
Pointer to host video buffer.
The NTV2 test pattern generator.
NTV2OutputCrosspointID
Identifies a widget output, a signal source, that potentially can drive another widget's input (ident...
uint32_t Read(uint8_t *pBuffer, const uint32_t length)
virtual bool SetHDMIOutAudioSource2Channel(const NTV2AudioChannelPair inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the HDMI output's 2-channel audio source.
virtual void Quit(void)
Gracefully stops me from running.
@ NTV2_OUTPUTDESTINATION_HDMI
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
virtual bool GetDolbyFrame(uint16_t *pInDolbyBuffer, uint32_t &numSamples)
Get a dolby audio audio frame from the input file.
NTV2OutputXptID GetFrameBufferOutputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsRGB=false, const bool inIs425=false)
std::map< NTV2TCIndex, NTV2_RP188 > NTV2TimeCodes
A mapping of NTV2TCIndex enum values to NTV2_RP188 structures.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool GetBits(uint32_t &data, uint32_t inBitCount)
Retreive the specified number of bits from the bitstream buffer.
This struct replaces the old RP188_STRUCT.
bool IsRGBFormat(const NTV2FrameBufferFormat format)
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
virtual uint32_t AddRamp(ULWord *audioBuffer)
Inserts audio test ramp into the given audio buffer.
uint32_t fVideoBufferSize
Size of host video buffer, in bytes.
ULWord GetAudioSamplesPerFrame(const NTV2FrameRate inFrameRate, const NTV2AudioRate inAudioRate, ULWord inCadenceFrame=0, bool inIsSMPTE372Enabled=false)
Returns the number of audio samples for a given video frame rate, audio sample rate,...
virtual bool ParseBSI(uint16_t *pInDolbyBuffer, uint32_t numSamples, NTV2DolbyBSI *pBsi)
Parse the dolby audio bit stream information block.
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual NTV2DeviceID GetDeviceID(void)
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual bool GetAudioRate(NTV2AudioRate &outRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Returns the current NTV2AudioRate for the given Audio System.
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the producer thread's static callback function that gets called when the producer thread star...
static const double gFrequencies[]
virtual bool GetFrameRate(NTV2FrameRate &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
Returns the AJA device's currently configured frame rate via its "value" parameter.
virtual bool SetEnableVANCData(const bool inVANCenabled, const bool inTallerVANC, const NTV2Standard inStandard, const NTV2FrameGeometry inGeometry, const NTV2Channel inChannel=NTV2_CHANNEL1)
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
@ NTV2_TestPatt_MultiPattern
virtual void StartProducerThread(void)
Starts my test pattern producer thread.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
static NTV2TestPatternNames getTestPatternNames(void)
virtual void GetACStatus(ULWord &outGoodFrames, ULWord &outDroppedFrames, ULWord &outBufferLevel)
Provides status information about my output (playout) process.
double FramesToSeconds(int64_t frames) const
virtual void StartConsumerThread(void)
Starts my playout thread.
static TimecodeFormat NTV2FrameRate2TimecodeFormat(const NTV2FrameRate inFrameRate)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
@ NTV2_TestPatt_LineSweep
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
virtual AJAStatus Start()
ULWord acFramesDropped
Total number of frames dropped since CNTV2Card::AutoCirculateStart called.
static AJA_PixelFormat GetAJAPixelFormat(const NTV2FrameBufferFormat inFormat)
#define AJA_FAILURE(_status_)
@ NTV2_AUDIO_RATE_INVALID
@ NTV2_AudioChannel1_2
This selects audio channels 1 and 2 (Group 1 channels 1 and 2)
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
static const ULWord kAppSignature(((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0)))
Used when reserving the AJA device, this specifies the application signature.
bool NTV2DeviceCanDo2110(const NTV2DeviceID inDeviceID)
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
virtual bool SetHDMIOutAudioFormat(const NTV2AudioFormat inNewValue)
Sets the HDMI output's audio format.
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread star...
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
static const double gAmplitudes[]
bool GetRP188Str(std::string &sRP188) const
Declares the AJADebug class.
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
NTV2StringList NTV2TestPatternNames
A list (std::vector) of pattern names.
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
static const uint32_t AUDIOBYTES_MAX_192K(824 *1024)
The maximum number of bytes of 192KHz audio that can be transferred for a single frame....
@ NTV2_AUDIOSYSTEM_INVALID
Declares the AJATimeBase class.