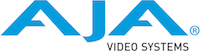 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
57 cout <<
"M31 Presets" << endl;
60 if (!presetStr.empty())
61 cout << ndx <<
": " << presetStr << endl;
68 cout <<
"M31 Formats" << endl;
71 if (!pixFormatStr.empty())
72 cout << ndx <<
": " << pixFormatStr << endl;
78 int main (
int argc,
const char ** argv)
88 int audioChannels (0);
96 {
"audio",
'a',
POPT_ARG_INT, &audioChannels, 0,
"number of audio channels",
"1-16"},
97 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model"},
98 {
"format",
'f',
POPT_ARG_INT, &codecFormat, 0,
"format to use",
"use '-lf' for list"},
99 {
"preset",
'p',
POPT_ARG_INT, &codecPreset, 0,
"codec preset to use",
"use '-lp' for list"},
100 {
"list",
'l',
POPT_ARG_STRING, &pWhatToList, 0,
"list options",
"p or f (presets or formats)"},
101 {
"info",
'i',
POPT_ARG_NONE, &infoData, 0,
"write encoded info file",
""},
113 if (pWhatToList &&
string(pWhatToList) ==
"p")
115 else if (pWhatToList &&
string(pWhatToList) ==
"f")
117 else if (pWhatToList)
118 {cerr <<
"## ERROR: Invalid argument to --list option, expected 'p' or 'f'" << endl;
return 2;}
121 {cerr <<
"## ERROR: Invalid M31 format " << codecFormat <<
" -- expected 0 thru " << (
gNumCodecFormats) << endl;
return 2;}
127 {cerr <<
"## ERROR: Invalid M31 preset " << codecPreset <<
" -- expected 0 thru " << (
gNumCodecPresets) << endl;
return 2;}
139 const bool infoMode (infoData ?
true :
false);
140 const uint32_t hevcMaxFrames (maxFrames >=
kNoSelection ? 0xffffffff : uint32_t(maxFrames));
142 m31Preset, pixelFormat,
UWord(audioChannels),
143 infoMode, hevcMaxFrames);
147 {cerr <<
"## ERROR: Initialization failed" << endl;
return 1;}
157 hevcVifEncoder.
Run();
159 cout <<
" Capture Capture" << endl
160 <<
" Frames Frames Buffer" << endl
161 <<
"Processed Dropped Level" << endl;
177 hevcVifEncoder.
Quit();
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
@ NTV2_FBF_10BIT_YCBCR_420PL2
10-Bit 4:2:0 2-Plane YCbCr
@ M31_VIF_3840X2160_422_10_50p
@ M31_VIF_3840X2160_420_10_50p
static const size_t gNumCodecFormats(sizeof(kCodecFormat)/sizeof(NTV2FrameBufferFormat))
Declares the AJATime class.
void SignalHandler(int inSignal)
@ M31_VIF_3840X2160_422_10_5994p
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ M31_VIF_3840X2160_420_8_50p
int main(int argc, const char **argv)
@ M31_VIF_3840X2160_420_10_5994p
@ M31_VIF_3840X2160_420_8_5994p
static const size_t gNumCodecPresets(sizeof(kCodecPreset)/sizeof(M31VideoPreset))
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
static int printFormats(void)
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
@ M31_VIF_3840X2160_420_10_60p
std::string NTV2M31VideoPresetToString(const M31VideoPreset inValue, const bool inForRetailDisplay=false)
Declares structs used for the Corvid HEVC.
Declares numerous NTV2 utility functions.
poptContext poptFreeContext(poptContext con)
@ M31_VIF_3840X2160_422_10_60p
const M31VideoPreset kCodecPreset[]
static bool gGlobalQuit((0))
@ NTV2_FBF_10BIT_YCBCR_422PL2
10-Bit 4:2:2 2-Plane YCbCr
#define POPT_ARGFLAG_OPTIONAL
static int printPresets(void)
virtual AJAStatus Run(void)
Runs me.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
static AJAStatus Open(bool incrementRefCount=false)
const int kNoSelection(1000000000)
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual void Quit(void)
Gracefully stops me from running.
poptContext poptGetContext(const char *name, int argc, const char **argv, const struct poptOption *options, unsigned int flags)
int poptGetNextOpt(poptContext con)
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
const NTV2FrameBufferFormat kCodecFormat[]
virtual void GetStatus(AVHevcStatus *outInputStatus)
Provides status information about my input (capture) process.
@ NTV2_FBF_8BIT_YCBCR_422PL2
8-Bit 4:2:2 2-Plane YCbCr
#define AJA_FAILURE(_status_)
Declares the NTV2EncodeHEVCVif class.
@ M31_VIF_3840X2160_420_8_60p