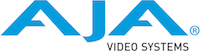 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
18 #define NTV2_AUDIOSIZE_MAX (401 * 1024)
25 const bool inQuadMode,
26 const uint32_t inAudioChannels,
27 const bool inTimeCodeBurn,
28 const bool inInfoData,
29 const uint32_t inMaxFrames)
40 mDeviceSpecifier (inDeviceSpecifier),
41 mWithAudio (inAudioChannels != 0),
42 mInputChannel (inChannel),
47 mPixelFormat (inPixelFormat),
51 mWithInfo (inInfoData),
52 mWithAnc (inTimeCodeBurn),
55 mNumAudioChannels (0),
56 mFileAudioChannels (inAudioChannels),
57 mMaxFrames (inMaxFrames),
59 mLastFrameInput (
false),
60 mLastFrameRaw (
false),
61 mLastFrameHevc (
false),
62 mLastFrameVideo (
false),
63 mLastFrameAudio (
false),
65 mVideoInputFrameCount (0),
66 mVideoProcessFrameCount (0),
67 mCodecRawFrameCount (0),
68 mCodecHevcFrameCount (0),
69 mVideoFileFrameCount (0),
70 mAudioFileFrameCount (0)
72 ::memset (mVideoInputBuffer, 0x0,
sizeof (mVideoInputBuffer));
73 ::memset (mVideoRawBuffer, 0x0,
sizeof (mVideoRawBuffer));
74 ::memset (mVideoHevcBuffer, 0x0,
sizeof (mVideoHevcBuffer));
75 ::memset (mAudioInputBuffer, 0x0,
sizeof (mAudioInputBuffer));
103 if (mVideoInputBuffer[bufferNdx].pVideoBuffer)
108 if (mVideoInputBuffer[bufferNdx].pInfoBuffer)
110 delete [] mVideoInputBuffer[bufferNdx].
pInfoBuffer;
113 if (mVideoInputBuffer[bufferNdx].pAudioBuffer)
119 if (mVideoRawBuffer[bufferNdx].pVideoBuffer)
124 if (mVideoRawBuffer[bufferNdx].pInfoBuffer)
129 if (mVideoRawBuffer[bufferNdx].pAudioBuffer)
135 if (mVideoHevcBuffer[bufferNdx].pVideoBuffer)
140 if (mVideoHevcBuffer[bufferNdx].pInfoBuffer)
145 if (mVideoHevcBuffer[bufferNdx].pAudioBuffer)
156 if (mAudioInputBuffer[bufferNdx].pVideoBuffer)
161 if (mAudioInputBuffer[bufferNdx].pInfoBuffer)
163 delete [] mAudioInputBuffer[bufferNdx].
pInfoBuffer;
166 if (mAudioInputBuffer[bufferNdx].pAudioBuffer)
179 if (mM31 && !mLastFrame && !mGlobalQuit)
187 for (i = 0; i < timeout; i++)
189 if (mLastFrameVideo && (!mWithAudio || mLastFrameAudio))
break;
193 { cerr <<
"## ERROR: Wait for last frame timeout" << endl; }
197 { cerr <<
"## ERROR: ChangeEHState ready to stop failed" << endl; }
200 { cerr <<
"## ERROR: ChangeEHState stop failed" << endl; }
204 { cerr <<
"## ERROR: ChangeVInState stop failed" << endl; }
210 { cerr <<
"## ERROR: ChangeMainState to init failed" << endl; }
217 while (mVideoInputThread.
Active())
220 while (mVideoProcessThread.
Active())
223 while (mCodecRawThread.
Active())
226 while (mCodecHevcThread.
Active())
229 while (mVideoFileThread.
Active())
232 while (mAudioFileThread.
Active())
261 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return AJA_STATUS_OPEN; }
277 cerr <<
"## ERROR: M31 not found" << endl;
282 mM31 =
new CNTV2m31 (&mDevice);
294 if (!CNTV2m31::IsPresetVIF(mPreset))
298 mVideoFormat = CNTV2m31::GetPresetVideoFormat(mPreset);
299 mPixelFormat = CNTV2m31::GetPresetFrameBufferFormat(mPreset);
300 mQuad = CNTV2m31::IsPresetUHD(mPreset);
301 mInterlaced = CNTV2m31::IsPresetInterlaced(mPreset);
319 switch (mInputChannel)
338 if(!CNTV2m31::ConvertVideoFormatToPreset(mVideoFormat, mPixelFormat,
true, mPreset))
341 mQuad = CNTV2m31::IsPresetUHD(mPreset);
342 mInterlaced = CNTV2m31::IsPresetInterlaced(mPreset);
362 status = mHevcCommon->
SetupHEVC (mM31, mPreset, mEncodeChannel, mMultiStream, mWithInfo);
371 ostringstream fileName;
373 fileName <<
"raw_" << (mInputChannel+1) <<
".hevc";
375 fileName <<
"raw.hevc";
376 status = mHevcCommon->
CreateHevcFile (fileName.str(), mMaxFrames);
384 ostringstream fileName;
386 fileName <<
"raw_" << (mInputChannel+1) <<
".txt";
388 fileName <<
"raw.txt";
389 status = mHevcCommon->
CreateEncFile (fileName.str(), mMaxFrames);
397 ostringstream fileName;
399 fileName <<
"raw_" << (mInputChannel+1) <<
".aiff";
401 fileName <<
"raw.aiff";
466 else if (mMultiStream)
473 mDevice.
SetVideoFormat (mVideoFormat,
false,
false, mInputChannel);
474 mDevice.
SetVideoFormat (mVideoFormat,
false,
false, mOutputChannel);
582 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
585 mVideoInputBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
589 mVideoInputBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
593 mVideoInputCircularBuffer.
Add (& mVideoInputBuffer[bufferNdx]);
598 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
601 mVideoRawBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
605 mVideoRawBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mPicInfoBufferSize/4];
609 mVideoRawCircularBuffer.
Add (& mVideoRawBuffer[bufferNdx]);
614 for (
unsigned bufferNdx = 0; bufferNdx <
VIDEO_RING_SIZE; bufferNdx++ )
617 mVideoHevcBuffer[bufferNdx].
pVideoBuffer =
new uint32_t [mVideoBufferSize/4];
621 mVideoHevcBuffer[bufferNdx].
pInfoBuffer =
new uint32_t [mEncInfoBufferSize/4];
625 mVideoHevcCircularBuffer.
Add (& mVideoHevcBuffer[bufferNdx]);
632 for (
unsigned bufferNdx = 0; bufferNdx <
AUDIO_RING_SIZE; bufferNdx++ )
635 mAudioInputBuffer[bufferNdx].
pAudioBuffer =
new uint32_t [mAudioBufferSize/4];
638 mAudioInputCircularBuffer.
Add (& mAudioInputBuffer[bufferNdx]);
716 cout << endl <<
"## WARNING: No video signal present on the input connector" << endl;
739 mVideoInputThread.
Start();
762 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
804 if (mWithAudio && pAudioData)
834 pPicData->serialNumber = mVideoInputFrameCount;
835 pPicData->ptsValueLow = (uint32_t)(pts & 0xffffffff);
836 pPicData->ptsValueHigh = (uint32_t)((pts >> 32) & 0x1);
837 pPicData->pictureNumber = mVideoInputFrameCount + 1;
844 pPicData->serialNumber = mVideoInputFrameCount*2;
845 pPicData->pictureNumber = mVideoInputFrameCount*2 + 1;
855 pPicData->serialNumber = mVideoInputFrameCount*2 + 1;
856 pPicData->ptsValueLow = (uint32_t)(pts & 0xffffffff);
857 pPicData->ptsValueHigh = (uint32_t)((pts >> 32) & 0x1);
858 pPicData->pictureNumber = mVideoInputFrameCount*2 + 2;
865 if(pVideoData->
lastFrame && !mLastFrameInput)
867 printf (
"\nCapture last frame number %d\n", mVideoInputFrameCount );
868 mLastFrameInput =
true;
871 mVideoInputFrameCount++;
873 if (mWithAudio && pAudioData)
904 mVideoProcessThread.
Start();
935 mVideoProcessFrameCount++;
955 mCodecRawThread.
Start();
978 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
994 if (mCodecRawFrameCount == 3)
1002 mLastFrameRaw =
true;
1005 mCodecRawFrameCount++;
1024 mCodecHevcThread.
Start();
1047 { cerr <<
"## ERROR: Device '" << mDeviceSpecifier <<
"' not found" << endl;
return; }
1050 m31 =
new CNTV2m31 (&ntv2Device);
1052 while (!mGlobalQuit)
1058 if (!mLastFrameHevc)
1063 uint8_t* pVideoBuffer = (uint8_t*)pFrameData->
pVideoBuffer;
1064 uint8_t* pInfoBuffer = (uint8_t*)pFrameData->
pInfoBuffer;
1069 m31->EncTransfer(mEncodeChannel,
1096 m31->EncTransfer(mEncodeChannel,
1119 m31->EncTransfer(mEncodeChannel,
1142 mLastFrameHevc =
true;
1145 mCodecHevcFrameCount++;
1162 mVideoFileThread.
Start();
1180 while (!mGlobalQuit)
1186 if (!mLastFrameVideo)
1201 printf (
"Video file last frame number %d\n", mVideoFileFrameCount );
1202 mLastFrameVideo =
true;
1205 mVideoFileFrameCount++;
1221 mAudioFileThread.
Start();
1239 while (!mGlobalQuit)
1245 if (!mLastFrameAudio)
1252 printf (
"Audio file last frame number %d\n", mAudioFileFrameCount );
1253 mLastFrameAudio =
true;
1257 mAudioFileFrameCount++;
1301 std::string timeString;
1302 mTimeCode.
Set(frameNumber);
1304 mTimeCode.
QueryString(timeString, mTimeBase,
false);
1307 mTimeCode.
QueryString(timeString, mTimeBase,
false);
@ NTV2_XptFrameBuffer6YUV
void WriteAiffData(void *pBuffer, uint32_t numChannels, uint32_t numSamples)
virtual bool SetSDIInLevelBtoLevelAConversion(const NTV2ChannelSet &inSDIInputs, const bool inEnable)
Enables or disables 3G level B to 3G level A conversion at the SDI input(s).
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
void QueryString(std::string &str, const AJATimeBase &timeBase, bool bDropFrame)
@ NTV2_CHANNEL8
Specifies channel or Frame Store 8 (or the 8th item).
ULWord GetCapturedAudioByteCount(void) const
AJA_PixelFormat GetAJAPixelFormat(NTV2FrameBufferFormat pixelFormat)
uint32_t timeCodeLow
Time code data low.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
@ AJA_ThreadPriority_High
AJA_EXPORT bool RenderTimeCodeFont(AJA_PixelFormat pixelFormat, uint32_t numPixels, uint32_t numLines)
virtual bool SetEmbeddedAudioClock(const NTV2EmbeddedAudioClock inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2EmbeddedAudioClock setting for the given NTV2AudioSystem.
Declares device capability functions.
bool SetAudioBuffer(ULWord *pInAudioBuffer, const ULWord inAudioByteCount)
Sets my audio buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
uint32_t videoDataSize2
Size of field 2 video data (bytes)
uint32_t audioDataSize
Size of audio data (bytes)
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
void WriteHevcData(void *pBuffer, uint32_t bufferSize)
NTV2FrameRate GetNTV2FrameRateFromVideoFormat(const NTV2VideoFormat inVideoFormat)
static void VideoProcessThreadStatic(AJAThread *pThread, void *pContext)
This is the video process thread's static callback function that gets called when the thread starts....
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
virtual void CodecRawWorker(void)
Repeatedly removes video frames from the raw video ring and transfers them to the codec.
bool lastFrame
Indicates last captured frame.
Declares the AJATime class.
AJA_EXPORT bool BurnTimeCode(void *pBaseVideoAddress, const std::string &inTimeCodeStr, const uint32_t inYPercent)
@ NTV2_XptFrameBuffer8YUV
virtual void SetupAutoCirculate(void)
Initializes AutoCirculate.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
ULWord GetProcessedFrameCount(void) const
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
uint32_t * pVideoBuffer
Pointer to host video buffer.
static void CodecRawThreadStatic(AJAThread *pThread, void *pContext)
This is the codec raw thread's static callback function that gets called when the thread starts....
void WriteEncData(void *pBuffer, uint32_t bufferSize)
ULWord GetBufferLevel(void) const
bool GetInputTimeCode(NTV2_RP188 &outTimeCode, const NTV2TCIndex inTCIndex=NTV2_TCINDEX_SDI1) const
Intended for capture, answers with a specific timecode captured in my acTransferStatus member's acFra...
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
@ NTV2_XptFrameBuffer4Input
virtual void StartVideoFileThread(void)
Start the video file writer thread.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
AJAStatus DetermineInputFormat(NTV2VideoFormat sdiFormat, bool quad, NTV2VideoFormat &videoFormat)
@ NTV2_XptFrameBuffer3Input
uint32_t infoDataSize
Size of the information data (bytes)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual AJAStatus ProcessVideoFrame(AVHevcDataBuffer *pSrcFrame, AVHevcDataBuffer *pDstFrame, uint32_t frameNumber)
Default do-nothing function for processing the captured frames.
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
virtual void GetStatus(AVHevcStatus *outInputStatus)
Provides status information about my input (capture) process.
@ NTV2_CHANNEL6
Specifies channel or Frame Store 6 (or the 6th item).
virtual bool SetAudioSystemInputSource(const NTV2AudioSystem inAudioSystem, const NTV2AudioSource inAudioSource, const NTV2EmbeddedAudioInput inEmbeddedInput)
Sets the audio source for the given NTV2AudioSystem on the device.
ULWord GetDisplayHeight(const NTV2VideoFormat videoFormat)
uint32_t * pInfoBuffer
Picture information (raw) or encode information (hevc)
uint32_t infoDataSize2
Size of the field 2 information data (bytes)
virtual void VideoInputWorker(void)
Repeatedly captures video frames using AutoCirculate and add them to the video input ring.
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
@ NTV2_CHANNEL5
Specifies channel or Frame Store 5 (or the 5th item).
Declares the NTV2EncodeHEVCVifAc class.
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
uint32_t videoDataSize
Size of video data (bytes)
void SetAJAFrameRate(AJA_FrameRate ajaFrameRate)
ULWord fLo
| BG 4 | Secs10 | BG 3 | Secs 1 | BG 2 | Frms10 | BG 1 | Frms 1 |
Declares the AJAProcess class.
ULWord fHi
| BG 8 | Hrs 10 | BG 7 | Hrs 1 | BG 6 | Mins10 | BG 5 | Mins 1 |
@ NTV2_XptFrameBuffer1Input
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
virtual void Quit(void)
Gracefully stops me from running.
NTV2EmbeddedAudioInput NTV2ChannelToEmbeddedAudioInput(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2EmbeddedAudioInput.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual void VideoFileWorker(void)
Repeatedly removes hevc frame from the hevc ring and writes them to the hevc output file.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
@ NTV2_CHANNEL7
Specifies channel or Frame Store 7 (or the 7th item).
static void AudioFileThreadStatic(AJAThread *pThread, void *pContext)
This is the audio file writer thread's static callback function that gets called when the thread star...
virtual void CodecHevcWorker(void)
Repeatedly transfers hevc frames from the codec and adds them to the hevc ring.
static void VideoFileThreadStatic(AJAThread *pThread, void *pContext)
This is the video file writer thread's static callback function that gets called when the thread star...
void SetRP188(const uint32_t inDBB, const uint32_t inLo, const uint32_t inHi, const AJATimeBase &inTimeBase)
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
@ NTV2_MAX_NUM_VIDEO_FORMATS
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
virtual void AudioFileWorker(void)
Repeatedly removes audio samples from the audio input ring and writes them to the audio output file.
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=false)
Connects the given widget signal input (sink) to the given widget signal output (source).
@ NTV2_CHANNEL3
Specifies channel or Frame Store 3 (or the 3rd item).
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
@ NTV2_EMBEDDED_AUDIO_CLOCK_VIDEO_INPUT
Audio clock derived from the video input.
Declares numerous NTV2 utility functions.
uint32_t * pAudioBuffer
Pointer to host audio buffer.
void SetStdTimecodeForHfr(bool bStdTc)
virtual void StartAudioFileThread(void)
Start the audio file writer thread.
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
I interrogate and control an AJA video/audio capture/playout device.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
struct HevcPictureInfo HevcPictureInfo
ULWord GetVideoActiveSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
AJAStatus CreateEncFile(const std::string &inFileName, uint32_t maxFrames)
static void VideoInputThreadStatic(AJAThread *pThread, void *pContext)
This is the video input thread's static callback function that gets called when the thread starts....
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
virtual void RouteInputSignal(void)
Sets up device routing for capture.
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
bool NTV2DeviceHasHEVCM31(const NTV2DeviceID inDeviceID)
#define NTV2_AUDIOSIZE_MAX
struct HevcEncodedInfo HevcEncodedInfo
@ NTV2_STANDARD_TASKS
1: Standard/Retail: Device is completely controlled by AJA ControlPanel, service/daemon,...
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
uint32_t timeCodeDBB
Time code data dbb.
UWord NTV2DeviceGetNumAudioSystems(const NTV2DeviceID inDeviceID)
static void CodecHevcThreadStatic(AJAThread *pThread, void *pContext)
This is the codec hevc thread's static callback function that gets called when the thread starts....
virtual void StartVideoInputThread(void)
Start the video input thread.
struct HevcPictureData HevcPictureData
@ NTV2_XptFrameBuffer2Input
#define AUTOCIRCULATE_WITH_RP188
Use this to AutoCirculate with RP188.
bool IsRunning(void) const
virtual void StartVideoProcessThread(void)
Start the video process thread.
@ NTV2_MODE_CAPTURE
Capture (input) mode, which writes into device SDRAM.
virtual void StartCodecHevcThread(void)
Start the codec hevc thread.
NTV2EncodeHEVCVifAc(const std::string inDeviceSpecifier="0", const NTV2Channel inChannel=NTV2_CHANNEL1, const M31VideoPreset inM31Preset=M31_FILE_1280X720_420_8_5994p, const NTV2FrameBufferFormat inPixelFormat=NTV2_FBF_10BIT_YCBCR_420PL2, const bool inQuadMode=(0), const uint32_t inAudioChannels=0, const bool inTimeCodeBurn=(0), const bool inInfoData=(0), const uint32_t inMaxFrames=0xffffffff)
Constructs me using the given settings.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
This struct replaces the old RP188_STRUCT.
@ NTV2_AUDIO_EMBEDDED
Obtain audio samples from the audio that's embedded in the video HANC.
@ NTV2_XptFrameBuffer7YUV
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
ULWord GetDroppedFrameCount(void) const
int64_t FramesToMicroseconds(int64_t frames, bool round=false) const
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
virtual void StartCodecRawThread(void)
Start the codec raw thread.
virtual NTV2VideoFormat GetSDIInputVideoFormat(NTV2Channel inChannel, bool inIsProgressive=false)
Returns the video format of the signal that is present on the given SDI input source.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
AJAStatus CreateAiffFile(const std::string &inFileName, uint32_t numChannels, uint32_t maxFrames, uint32_t bufferSize)
@ Hevc_EhState_ReadyToStop
HevcPictureData pictureData
ULWord GetDisplayWidth(const NTV2VideoFormat videoFormat)
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
virtual AJAStatus Run(void)
Runs me.
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=false)
Returns the video format of the signal that is present on the given input source.
AJAStatus CreateHevcFile(const std::string &inFileName, uint32_t maxFrames)
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
uint32_t videoBufferSize
Size of host video buffer (bytes)
uint32_t timeCodeHigh
Time code data high.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
uint32_t infoBufferSize
Size of the host information buffer (bytes)
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
uint32_t AlignDataBuffer(void *pBuffer, uint32_t bufferSize, uint32_t dataSize, uint32_t alignBytes, uint8_t fill)
virtual bool SetNumberAudioChannels(const ULWord inNumChannels, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the number of audio channels to be concurrently captured or played for a given Audio System on t...
virtual bool SetAudioBufferSize(const NTV2AudioBufferSize inValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Changes the size of the audio buffer that is used for a given Audio System in the AJA device.
virtual AJAStatus Start()
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=true)
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
AJA_FrameRate GetAJAFrameRate(NTV2FrameRate frameRate)
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
This structure encapsulates the video and audio buffers used by the HEVC demo applications....
#define AJA_FAILURE(_status_)
@ NTV2_XptFrameBuffer5YUV
virtual ~NTV2EncodeHEVCVifAc()
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
virtual void VideoProcessWorker(void)
Repeatedly removes video frames from the video input ring, calls a custom video process method and ad...
NTV2AudioSystem NTV2ChannelToAudioSystem(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its equivalent NTV2AudioSystem.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
virtual bool GetSDIInput3GbPresent(bool &outValue, const NTV2Channel channel)
bool SetBuffers(ULWord *pInVideoBuffer, const ULWord inVideoByteCount, ULWord *pInAudioBuffer, const ULWord inAudioByteCount, ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
UWord NTV2DeviceGetMaxAudioChannels(const NTV2DeviceID inDeviceID)
uint32_t audioBufferSize
Size of host audio buffer (bytes)
virtual bool SetSDIOutLevelAtoLevelBConversion(const UWord inOutputSpigot, const bool inEnable)
Enables or disables 3G level A to 3G level B conversion at the SDI output widget (assuming the AJA de...
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
AJAStatus SetupHEVC(CNTV2m31 *pM31, M31VideoPreset preset, M31Channel encodeChannel, bool multiStream, bool withInfo)
virtual bool SetAudioRate(const NTV2AudioRate inRate, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the NTV2AudioRate for the given Audio System.
@ NTV2_AUDIOSYSTEM_INVALID