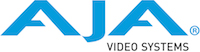 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
13 #if defined(MSWindows) || defined(AJAWindows)
14 #define SIGQUIT SIGBREAK
36 if (gChannelNames.empty())
39 return aja::join(gChannelNames, inDelimiterStr);
43 int main (
int argc,
const char ** argv)
55 int channelNumber (1);
56 int doMultiFormat (0);
62 int breakNewLines (0);
76 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
77 {
"channel",
'c',
POPT_ARG_INT, &channelNumber, 0,
"channel to use",
"1-8" },
79 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
80 {
"frames", 0,
POPT_ARG_STRING, &pFramesSpec, 0,
"frames to AutoCirculate",
"num[@min] or min-max" },
81 {
"videoFormat",
'v',
POPT_ARG_STRING, &pVideoFormat, 0,
"video format to produce",
"'?' or 'list' to list" },
84 {
"output",
'o',
POPT_ARG_STRING, &pOutputDest, 0,
"output connector to use",
"'?' or 'list' to list" },
85 {
"pattern", 0,
POPT_ARG_STRING, &pTestPattern, 0,
"test pattern to use",
"'?' or 'list' to list" },
97 {
"end",
'e',
POPT_ARG_STRING, &pEndAction, 0,
"end action",
"exit|loop|idle,..." },
98 {
"rate",
'r',
POPT_ARG_STRING, &pCaptionRate, 0,
"caption rate",
"chars/min,..." },
99 {
"608mode", 0,
POPT_ARG_STRING, &pMode, 0,
"608 caption mode",
"roll[N]|paint|pop,..." },
100 {
"608chan", 0,
POPT_ARG_STRING, &pCaptionChan, 0,
"608 caption channel", L21ChanNames.c_str() },
108 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
110 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
113 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
120 if ((channelNumber < 1) || (channelNumber > 8))
121 {cerr <<
"## ERROR: Invalid channel number " << channelNumber <<
" -- expected 1 thru 8" << endl;
return 1;}
125 const string videoFormatStr (pVideoFormat ? pVideoFormat :
"");
128 if (videoFormatStr ==
"?" || videoFormatStr ==
"list")
131 { cerr <<
"## ERROR: Invalid '--videoFormat' value '" << videoFormatStr <<
"' -- expected values:" << endl
140 cerr <<
"## WARNING: '--noline21' and '--no608' options will result in no captions in SD" << endl;
142 cerr <<
"## WARNING: '--no608' and '--no708' options will result in no captions in HD" << endl;
149 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
151 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
155 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
161 static const string legalFramesSpec (
"{frameCount}[@{firstFrameNum}] or {firstFrameNum}-{lastFrameNum}");
162 const string framesSpec (pFramesSpec ? pFramesSpec :
"");
163 if (!framesSpec.empty())
166 if (!parseResult.empty())
167 {cerr <<
"## ERROR: Bad 'frames' spec '" << framesSpec <<
"'\n## " << parseResult << endl;
return 1;}
170 {cerr <<
"## ERROR: Bad 'frames' spec '" << framesSpec <<
"'\n## Expected " << legalFramesSpec << endl;
return 1;}
174 pDeviceSpec ? deviceSpec :
""));
177 if (outputDestStr ==
"?" || outputDestStr ==
"list")
178 {cout << legalOutputs << endl;
return 0;}
179 if (!outputDestStr.empty())
183 pDeviceSpec ? deviceSpec :
"");
185 {cerr <<
"## ERROR: Output '" << outputDestStr <<
"' not of:" << endl << legalOutputs << endl;
return 1;}
199 string testPattern(pTestPattern ? pTestPattern :
"");
200 if (testPattern ==
"?" || testPattern ==
"list")
202 if (!testPattern.empty())
205 if (testPattern.empty())
207 cerr <<
"## ERROR: Invalid '--pattern' value '" << pTestPattern <<
"' -- expected values:" << endl
213 testPattern =
"Flat Field";
240 {cerr <<
"## ERROR: Bad '608chan' value '" << pCaptionChan <<
"' -- expected '" <<
::GetLine21ChannelNames () <<
"'" << endl;
return 1;}
243 const string sCaptionMode (ndx < sCaptionModes.size() ? sCaptionModes.at(ndx) : sCaptionModes.back());
250 else {cerr <<
"## ERROR: Bad '608mode' parameter '" << sCaptionMode <<
"'" << endl;
return 1;}
261 const string sEndAction (ndx < sEndActions.size() ? sEndActions.at(ndx) : sEndActions.back());
262 if (sEndAction.empty () || sEndAction ==
"quit" || sEndAction ==
"exit" || sEndAction ==
"terminate" || sEndAction ==
"end")
264 else if (sEndAction ==
"loop" || sEndAction ==
"repeat")
266 else if (sEndAction ==
"sleep" || sEndAction ==
"idle" || sEndAction ==
"rest")
269 {cerr <<
"## ERROR: Bad 'end' parameter '" << sEndAction <<
"' -- expected 'loop|repeat | rest|sleep|idle | quit|end|exit|terminate'" << endl;
return 1;}
272 const string sCaptionRate (ndx < sCaptionRates.size() ? sCaptionRates.at(ndx) : sCaptionRates.back());
273 istringstream iss(sCaptionRate);
274 uint32_t charsPerMin;
278 if (!pathList.empty())
280 generatorConfig.
fFilesToPlay.push_back (fileNdx < pathList.size() ? pathList.at(fileNdx) : pathList.back());
282 if ((ndx+1) == sCaptionChannels.size())
283 while (fileNdx < pathList.size())
284 generatorConfig.
fFilesToPlay.push_back (pathList.at (fileNdx++));
307 {cout <<
"## ERROR: Initialization failed: " <<
::AJAStatusToString(status) << endl;
return 1;}
318 size_t msgsQued (0), bytesQued (0), totMsgsEnq (0), totBytesEnq (0), totMsgsDeq (0), totBytesDeq (0), maxQueDepth (0), droppedFrames (0);
320 cout <<
" Current Current Total Total Total Total Max " << endl
321 <<
" Messages Bytes Messages Bytes Messages Bytes Queue Dropped" << endl
322 <<
" Queued Queued Enqueued Enqueued Dequeued Dequeued Depth Frames" << endl;
327 player.
GetStatus (msgsQued, bytesQued, totMsgsEnq, totBytesEnq, totMsgsDeq, totBytesDeq, maxQueDepth, droppedFrames);
329 cout << setw(9) << msgsQued
330 << setw(9) << bytesQued
331 << setw(9) << totMsgsEnq
332 << setw(9) << totBytesEnq
333 << setw(9) << totMsgsDeq
334 << setw(9) << totBytesDeq
335 << setw(9) << maxQueDepth
336 << setw(9) << droppedFrames <<
"\r" << flush;
342 player.
GetStatus (msgsQued, bytesQued, totMsgsEnq, totBytesEnq, totMsgsDeq, totBytesDeq, maxQueDepth, droppedFrames);
344 << msgsQued <<
" message(s) left in queue" << endl
345 << bytesQued <<
" byte(s) left in queue" << endl
346 << totMsgsEnq <<
" total message(s) enqueued" << endl
347 << totBytesEnq <<
" total byte(s) enqueued" << endl
348 << totMsgsDeq <<
" total message(s) dequeued" << endl
349 << totBytesDeq <<
" total byte(s) dequeued" << endl
350 << maxQueDepth <<
" maximum message(s) enqueued" << endl;
I am an object that can inject text captions into an SDI output of an AJA device in real time....
virtual const NTV2StringList & otherArgs(void) const
static NTV2VideoFormat GetVideoFormatFromString(const std::string &inStr, const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD, const std::string &inDevSpec=std::string())
Returns the NTV2VideoFormat that matches the given string.
#define NTV2_IS_SD_VIDEO_FORMAT(__f__)
NTV2OutputDestination
Identifies a specific video output destination.
static NTV2PixelFormat GetPixelFormatFromString(const std::string &inStr, const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2PixelFormat that matches the given string.
static bool gQuitImmediately((0))
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
bool fNewLinesAreNewRows
If true, newlines break caption rows; otherwise are treated as whitespace.
uint16_t fForceRTP
BIT(0):0=normal,1=forceRTP BIT(1):0=uniPkt,1=multiPkt BIT(2):0=normal,1=patchDeviceID.
std::string join(const std::vector< std::string > &parts, const std::string &delim)
void split(const std::string &str, const char delim, std::vector< std::string > &elems)
@ AtEndAction_Idle
Repeat the file list (must Ctrl-C to terminate)
Configures an NTV2CCPlayer instance.
NTV2Line21Mode fCaptionMode
The CEA-608 caption mode to use.
@ NTV2_CC608_CapModePaintOn
Paint-on caption mode.
void SignalHandler(int inSignal)
static string GetLine21ChannelNames(const string inDelimiterStr="|")
bool fSuppressTimecode
If true, suppress timecode; otherwise embed VITC/LTC.
NTV2Channel NTV2OutputDestinationToChannel(const NTV2OutputDestination inOutputDest)
Converts a given NTV2OutputDestination to its equivalent NTV2Channel value.
NTV2StringList fFilesToPlay
A list of zero or more strings containing paths to text files to be "played".
int main(int argc, const char **argv)
CaptionChanGenMap fCapChanGenConfigs
Caption channel generator configs.
NTV2Line21Channel fCaptionChannel
The caption channel to use.
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
#define NTV2_IS_VALID_OUTPUT_DEST(_dest_)
std::string setFromString(const std::string &inStr)
NTV2VANCMode fVancMode
VANC mode to use.
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
NTV2OutputDestination NTV2ChannelToOutputDestination(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
Converts the given NTV2Channel value into its ordinary equivalent NTV2OutputDestination.
@ NTV2_IOKINDS_ANALOG
Specifies analog input/output kinds.
static std::string GetOutputDestinationStrings(const NTV2IOKinds inKinds, const std::string inDevSpec=std::string())
bool fDoTsiRouting
If true, enable TSI routing; otherwise route for square division (4K/8K)
#define IsLine21XDSChannel(_chan_)
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
@ NTV2_IOKINDS_SDI
Specifies SDI input/output kinds.
static bool IsValidDevice(const std::string &inDeviceSpec)
static std::string GetTestPatternStrings(void)
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
AtEndAction fEndAction
The action to take after the file list has finished playing.
bool fEmitStats
If true, show stats while playing; otherwise echo caption text being played.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
@ NTV2_CC608_CapModeUnknown
Unknown or invalid caption mode.
bool fSuppressAudio
If true, suppress audio; otherwise generate & xfer audio tone.
bool fSuppress608
If true, don't transmit CEA608 packets; otherwise include 608 packets.
This class is used to configure a caption generator for a single caption channel.
#define IsValidLine21Channel(_chan_)
@ AtEndAction_Repeat
Terminate.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
NTV2Line21Channel StrToNTV2Line21Channel(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21Channel value.
NTV2VideoFormat fVideoFormat
The video format to use.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
static NTV2OutputDestination GetOutputDestinationFromString(const std::string &inStr, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2OutputDestination that matches the given string.
NTV2Channel fOutputChannel
The device channel to use.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
static std::string GetVideoFormatStrings(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD, const std::string inDevSpec=std::string())
bool fSuppressLine21
SD output only: if true, do not encode Line 21 waveform; otherwise encode Line 21 waveform.
static bool gGlobalQuit((0))
@ NTV2_CC608_CapModeRollUp4
4-row roll-up from bottom
static std::string GetTestPatternNameFromString(const std::string &inStr)
std::vector< std::string > NTV2StringList
#define IsLine21TextChannel(_chan_)
static AJAStatus Open(bool incrementRefCount=false)
#define NTV2_IS_VALID_CHANNEL(__x__)
@ NTV2_CC608_CapModeRollUp2
2-row roll-up from bottom
@ NTV2_VANCMODE_TALLER
This identifies the mode in which there are some + extra VANC lines in the frame buffer.
bool fDoRGBOnWire
If true, produce RGB on the wire; otherwise output YUV.
const std::string & NTV2Line21ChannelToStr(const NTV2Line21Channel inLine21Channel, const bool inCompact=true)
Converts the given NTV2Line21Channel value into a human-readable string.
NTV2OutputDest fOutputDest
The desired output connector to use.
@ NTV2_OUTPUTDESTINATION_INVALID
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
std::string fTestPatternName
The test pattern to use.
std::string AJAStatusToString(const AJAStatus inStatus, const bool inDetailed)
@ NTV2_CC608_CapModeRollUp3
3-row roll-up from bottom
virtual void Quit(const bool inQuitImmediately=(0))
Stops me.
virtual AJAStatus Run(void)
Runs me.
bool fSuppress708
If true, don't transmit CEA708 packets; otherwise include 708 packets.
NTV2StringList::const_iterator NTV2StringListConstIter
NTV2PixelFormat fPixelFormat
The pixel format to use.
double fCharsPerMinute
The rate at which caption characters get enqueued, in characters per minute.
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
virtual void GetStatus(size_t &outMessagesQueued, size_t &outBytesQueued, size_t &outTotMsgsEnq, size_t &outTotBytesEnq, size_t &outTotMsgsDeq, size_t &outTotBytesDeq, size_t &outMaxQueDepth, size_t &outDroppedFrames) const
Returns status information from my caption encoder.
#define AJA_FAILURE(_status_)
@ NTV2_CC608_CapModePopOn
Pop-on caption mode.
#define NTV2_IS_VALID_VIDEO_FORMAT(__f__)