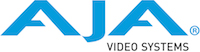 |
AJA NTV2 SDK
17.6.0.1688
NTV2 SDK 17.6.0.1688
|
Go to the documentation of this file.
9 #ifndef _NTV2CCPLAYER_H
10 #define _NTV2CCPLAYER_H
21 #define SIG_AJA_STOP 31 // Our own user-defined "stop" signal
156 virtual void Quit (
const bool inQuitImmediately =
false);
170 virtual void GetStatus (
size_t & outMessagesQueued,
size_t & outBytesQueued,
171 size_t & outTotMsgsEnq,
size_t & outTotBytesEnq,
172 size_t & outTotMsgsDeq,
size_t & outTotBytesDeq,
173 size_t & outMaxQueDepth,
size_t & outDroppedFrames)
const;
234 bool mCaptionGeneratorQuit;
243 #endif // _NTV2CCPLAYER_H
I am an object that can inject text captions into an SDI output of an AJA device in real time....
enum NTV2EveryFrameTaskMode NTV2TaskMode
Declares the AJASystemInfo class.
Declares several data types used with 608/SD captioning.
virtual bool DeviceAncExtractorIsAvailable(void)
struct CCGenConfig CCGenConfig
This class is used to configure a caption generator for a single caption channel.
virtual AJAStatus SetUpOutputVideo(void)
Sets up everything I need to play video.
virtual void PlayoutFrames(void)
Repeatedly plays frames (until time to quit).
CaptionChanGenMap::const_iterator CaptionChanGenMapCIter
Describes a user-space buffer on the host computer. I have an address and a length,...
bool fNewLinesAreNewRows
If true, newlines break caption rows; otherwise are treated as whitespace.
virtual void StartCaptionGeneratorThreads(void)
Starts my caption generator threads.
uint16_t fForceRTP
BIT(0):0=normal,1=forceRTP BIT(1):0=uniPkt,1=multiPkt BIT(2):0=normal,1=patchDeviceID.
@ AtEndAction_Idle
Repeat the file list (must Ctrl-C to terminate)
enum _AtEndAction_ AtEndAction
These are the actions that can be taken after the last file is "played".
Configures an NTV2CCPlayer instance.
NTV2Line21Mode fCaptionMode
The CEA-608 caption mode to use.
bool fSuppressTimecode
If true, suppress timecode; otherwise embed VITC/LTC.
CCGenConfig()
Constructs a default generator configuration.
NTV2StringList fFilesToPlay
A list of zero or more strings containing paths to text files to be "played".
CaptionChanGenMap::iterator CaptionChanGenMapIter
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
std::vector< AJAThread > AJAThreadList
CaptionChanGenMap fCapChanGenConfigs
Caption channel generator configs.
NTV2Line21Channel fCaptionChannel
The caption channel to use.
Declares the CNTV2CaptionEncoder608 class.
virtual AJAStatus SetUpBackgroundPatternBuffer(void)
Sets up my gray background field.
AJALabelValuePairs Get(void) const
NTV2FrameRate
Identifies a particular video frame rate.
static void PlayThreadStatic(AJAThread *pThread, void *pContext)
This is the playout thread's static callback function that gets called when the playout thread runs....
virtual void StartPlayoutThread(void)
Starts my playout thread.
std::ostream & operator<<(std::ostream &ioStrm, const CCPlayerConfig &inObj)
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
AtEndAction fEndAction
The action to take after the file list has finished playing.
bool fEmitStats
If true, show stats while playing; otherwise echo caption text being played.
NTV2Standard
Identifies a particular video standard.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
std::map< NTV2Line21Channel, CCGenConfig > CaptionChanGenMap
bool fSuppress608
If true, don't transmit CEA608 packets; otherwise include 608 packets.
_AtEndAction_
These are the actions that can be taken after the last file is "played".
This class is used to configure a caption generator for a single caption channel.
CCPlayerConfig(const std::string &inDeviceSpecifier="0")
Constructs a default CCPlayer configuration.
@ AtEndAction_Repeat
Terminate.
virtual AJAStatus RouteOutputSignal(void)
Sets up signal routing for playout.
bool fSuppressLine21
SD output only: if true, do not encode Line 21 waveform; otherwise encode Line 21 waveform.
I interrogate and control an AJA video/audio capture/playout device.
TimecodeFormat NTV2FrameRate2TimecodeFormat(NTV2FrameRate inFrameRate)
@ NTV2_CC608_CapModeRollUp4
4-row roll-up from bottom
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
std::vector< std::string > NTV2StringList
virtual ~NTV2CCPlayer(void)
NTV2Line21Mode
The CEA-608 modes: pop-on, roll-up (2, 3 and 4-line), and paint-on.
This file contains some structures, constants, classes and functions that are used in some of the dem...
static void CaptionGeneratorThreadStatic(AJAThread *pThread, void *pContext)
This is the caption generator thread's static callback function that gets called when the caption gen...
@ NTV2_CC608_CC1
Caption channel 1, the primary caption channel.
Declares the CNTV2CaptionEncoder708 class.
std::map< NTV2InputXptID, NTV2OutputXptID > NTV2XptConnections
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
AJAThreadList::const_iterator AJAThreadListConstIter
std::string fTestPatternName
The test pattern to use.
virtual void Quit(const bool inQuitImmediately=(0))
Stops me.
virtual void GenerateCaptions(const NTV2Line21Channel inCCChannel)
This is the thread function that produces caption messages for a given caption channel.
virtual AJAStatus Run(void)
Runs me.
bool fSuppress708
If true, don't transmit CEA708 packets; otherwise include 708 packets.
@ AtEndAction_Max
Continue to emit gray field on the device (must Ctrl-C to terminate)
Configures an NTV2Player instance.
NTV2Line21Attrs fAttributes
The character attributes to use.
CEA-608 Character Attributes.
double fCharsPerMinute
The rate at which caption characters get enqueued, in characters per minute.
virtual void GetStatus(size_t &outMessagesQueued, size_t &outBytesQueued, size_t &outTotMsgsEnq, size_t &outTotBytesEnq, size_t &outTotMsgsDeq, size_t &outTotBytesDeq, size_t &outMaxQueDepth, size_t &outDroppedFrames) const
Returns status information from my caption encoder.
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
Declares the AJAThread class.
void SignalHandler(int inSignal)
AJALabelValuePairs Get(const bool inCompact=(0)) const
CCPlayerConfig CCPlayerConfig
Configures an NTV2CCPlayer instance.
NTV2CCPlayer(const CCPlayerConfig &inConfigData)
Constructs me using the given configuration settings.