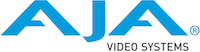 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
18 const int maxbufsize = 256;
19 char buffer[maxbufsize];
20 std::string result =
"";
21 FILE* pipe = popen(cmd,
"r");
24 throw std::runtime_error(
"popen() failed!");
31 if (fgets(buffer, maxbufsize, pipe) !=
NULL)
53 throw std::runtime_error(
"aja_cmd() failed!");
58 std::string
aja_procfs(
const char* procfs_file,
const char* value_key)
70 std::ostringstream oss;
71 oss <<
"cat /proc/" << procfs_file <<
" | grep '" << value_key <<
"' | head -n 1 | cut -d ':' -f 2 | xargs | tr -d '\n' | tr -s ' '";
73 return aja_cmd(oss.str().c_str());
82 std::ostringstream oss;
83 oss <<
"date -d \"`cut -f1 -d. /proc/uptime` seconds ago\" \"+%Y-%m-%d %H:%M:%S\"";
84 out =
aja_cmd(oss.str().c_str());
89 out =
aja_cmd(
"uptime -s 2>/dev/null");
99 out =
aja_cmd(
"lsb_release -d -s 2>/dev/null");
109 out =
aja_cmd(
"cat /etc/redhat-release 2>/dev/null");
113 out =
aja_cmd(
"cat /etc/os-release 2>/dev/null | grep 'PRETTY_NAME' | head -n 1 | cut -d '=' -f 2 | tr -d '\"' | tr -d '\n'");
124 out =
aja_cmd(
"lsb_release -r -s 2>/dev/null");
132 out =
aja_cmd(
"cat /etc/os-release 2>/dev/null | grep 'VERSION_ID' | head -n 1 | cut -d '=' -f 2 | tr -d '\"' | tr -d '\n'");
141 std::vector<std::string>& outFoundDevices)
143 if (inDevicePart.size() >= 2)
148 if (inDevicePart.find(
"SVendor") != inDevicePart.end())
149 vend = inDevicePart.at(
"SVendor");
150 else if (inDevicePart.find(
"Vendor") != inDevicePart.end())
151 vend = inDevicePart.at(
"Vendor");
153 if (inDevicePart.find(
"SDevice") != inDevicePart.end())
154 device = inDevicePart.at(
"SDevice");
155 else if (inDevicePart.find(
"Device") != inDevicePart.end())
156 device = inDevicePart.at(
"Device");
158 outFoundDevices.push_back(vend +
" " + device);
165 out =
aja_cmd(
"lspci -vmm | grep VGA -A 4");
184 std::ostringstream oss;
185 std::vector<std::string> lines =
aja::split(out,
'\n');
186 if (lines.empty() ==
false)
188 std::vector<std::string>::iterator it;
189 std::vector<std::string> foundDevices;
190 std::map<std::string, std::string> deviceParts;
191 for(it=lines.begin();it!=lines.end();++it)
193 if (*it ==
"--" || *it ==
"")
195 if (deviceParts.size() >= 2)
203 std::vector<std::string> cols =
aja::split(*it,
':');
207 std::string val = cols.at(1);
209 for(
size_t i=2;i<cols.size();++i)
211 val = val +
":" + cols.at(i);
220 else if (key ==
"Vendor" || key ==
"Device" ||
221 key ==
"SVendor" || key ==
"SDevice")
223 deviceParts[key] = val;
228 if (deviceParts.size() >= 2)
234 for(
size_t i=0;i<foundDevices.size();++i)
240 oss << foundDevices.at(i);
262 static char tmp_buf[4096];
267 gethostname(tmp_buf,
sizeof(tmp_buf));
287 long int numProcs = sysconf(_SC_NPROCESSORS_ONLN);
288 std::ostringstream num_cores;
289 num_cores << numProcs;
297 std::string memTotalStr =
aja_procfs(
"meminfo",
"MemTotal");
298 int64_t memtotalbytes=0;
299 if (memTotalStr.find(
" kB") != std::string::npos)
303 std::istringstream(memTotalStr) >> memtotalbytes;
304 memtotalbytes *= 1024;
309 std::istringstream(memTotalStr) >> memtotalbytes;
312 std::string memFreeStr =
aja_procfs(
"meminfo",
"MemFree");
313 int64_t memfreebytes=0;
314 if (memFreeStr.find(
" kB") != std::string::npos)
318 std::istringstream(memFreeStr) >> memfreebytes;
319 memfreebytes *= 1024;
324 std::istringstream(memFreeStr) >> memfreebytes;
327 std::string unitsLabel;
328 double divisor = 1.0;
345 divisor = 1073741824.0;
349 int64_t memusedbytes = memtotalbytes - memfreebytes;
351 std::ostringstream t,u,f;
352 t << int64_t(memtotalbytes / divisor) <<
" " << unitsLabel;
353 u << int64_t(memusedbytes / divisor) <<
" " << unitsLabel;
354 f << int64_t(memfreebytes / divisor) <<
" " << unitsLabel;
372 const char* homePath = getenv(
"HOME");
373 if (homePath !=
NULL)
@ AJA_SystemInfoSection_Path
Declares the AJASystemInfo class.
@ AJA_SystemInfoMemoryUnit_Megabytes
@ AJA_SystemInfoSection_CPU
std::string & strip(std::string &str, const std::string &ws)
void split(const std::string &str, const char delim, std::vector< std::string > &elems)
@ AJA_SystemInfoTag_Path_Firmware
@ AJA_SystemInfoTag_OS_KernelVersion
std::string aja_procfs(const char *procfs_file, const char *value_key)
@ AJA_SystemInfoTag_Path_Applications
virtual ~AJASystemInfoImpl()
void get_vendor_and_device(std::map< std::string, std::string > &inDevicePart, std::vector< std::string > &outFoundDevices)
@ AJA_SystemInfoMemoryUnit_Kilobytes
@ AJA_SystemInfoTag_CPU_Type
std::string aja_productname()
std::string aja_cmd(const char *cmd)
@ AJA_SystemInfoTag_CPU_NumCores
std::string & replace(std::string &str, const std::string &from, const std::string &to)
@ AJA_SystemInfoTag_System_Name
std::string aja_osversion()
std::map< int, std::string > mValueMap
@ AJA_SystemInfoTag_System_BootTime
@ AJA_SystemInfoTag_OS_Version
@ AJA_SystemInfoTag_Mem_Used
@ AJA_SystemInfoSection_GPU
@ AJA_SystemInfoTag_Path_PersistenceStoreUser
AJASystemInfoImpl(int units)
@ AJA_SystemInfoTag_Path_PersistenceStoreSystem
Declares the AJAFileIO class.
@ AJA_SystemInfoMemoryUnit_Gigabytes
@ AJA_SystemInfoTag_OS_ProductName
static bool FileExists(const std::wstring &fileName)
@ AJA_SystemInfoSection_OS
@ AJA_SystemInfoTag_Path_Utilities
@ AJA_SystemInfoTag_Path_UserHome
virtual AJAStatus Rescan(AJASystemInfoSections sections)
@ AJA_SystemInfoSection_Mem
@ AJA_SystemInfoTag_Mem_Free
@ AJA_SystemInfoTag_GPU_Type
std::string aja_getgputype()
@ AJA_SystemInfoTag_OS_VersionBuild
@ AJA_SystemInfoMemoryUnit_Bytes
@ AJA_SystemInfoSection_System
@ AJA_SystemInfoTag_Mem_Total
Declares the AJASystemInfoImpl class.
@ AJA_SystemInfoTag_System_Model