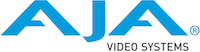 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
8 #ifndef NTV2STREAMGRABBER_H
9 #define NTV2STREAMGRABBER_H
11 #include <QBasicTimer>
13 #if (QT_VERSION >= QT_VERSION_CHECK(5, 0, 0))
19 #include <QtMultimedia>
25 #if defined (INCLUDE_AJACC)
28 #endif // INCLUDE_AJACC
30 #define STREAMPREVIEW_WIDGET_X (960)
31 #define STREAMPREVIEW_WIDGET_Y (540)
80 void newFrame (
const QImage &inImage,
const bool inClear);
91 virtual void run (
void);
107 bool mbFixedReference;
118 bool mFormatIsProgressive;
123 bool mDoMultiChannel;
128 #endif // NTV2STREAMGRABBER_H
void newStatusString(const QString &inStatus)
This is signaled (called) when my status string changes.
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
Declares common types used in the ajabase library.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
Declares the CNTV2Task class (deprecated).
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
bool SetupInput(void)
Configures my AJA device for capture.
virtual void run(void)
My thread function.
NTV2LHIHDMIColorSpace GetColorSpaceFromInputSource(void)
Declares the AJAProcess class.
void SetFixedReference(bool fixed)
Declares the CNTV2Card class.
Declares the CNTV2CaptionDecoder708 class.
Enumerations for controlling NTV2 devices.
void newFrame(const QImage &inImage, const bool inClear)
This is signaled (called) when a new frame has been captured and is available for display.
I interrogate and control an AJA video/audio capture/playout device.
UWord GetDeviceIndex(void) const
NTV2InputSource
Identifies a specific video input source.
void SetInputSource(const NTV2InputSource inInputSource)
Sets the input to be used for capture on the AJA device being used.
A QThread that captures audio/video from NTV2-compatible AJA devices and uses Qt signals to emit ARGB...
virtual ~NTV2StreamGrabber()
My destructor.
NTV2StreamGrabber(QObject *pInParentObject=NULL)
Constructs me.
NTV2VideoFormat GetVideoFormatFromInputSource(void)
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
NTV2EveryFrameTaskMode
Describes the task mode state. See also: Sharing AJA Devices With Other Applications.
bool IsInput3Gb(const NTV2InputSource inputSource)
void StopStream(void)
Stops capturing.
bool CheckForValidInput(void)
Declares the CNTV2CaptionDecoder608 class.
void SetDeviceIndex(const UWord inDeviceIndex)
Sets the AJA device to be used for capture.