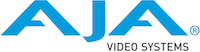 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
9 #if !defined (__DEVICE_NOTIFIER_H__)
10 #define __DEVICE_NOTIFIER_H__
12 #include <CoreFoundation/CoreFoundation.h>
13 #include <Carbon/Carbon.h>
14 #include <IOKit/IOMessage.h>
15 #include <IOKit/usb/IOUSBLib.h>
20 #define kAJADeviceInitialOpen 0xAA1
21 #define kAJADeviceTerminate 0xAA2
40 virtual bool Install (CFMutableDictionaryRef dict =
NULL);
54 virtual void DeviceChanged (io_service_t unitService, natural_t messageType,
void* message);
68 io_service_t unitService,
69 natural_t messageType,
93 virtual bool Install (CFMutableDictionaryRef dict =
NULL);
96 #endif // __DEVICE_NOTIFIER_H__
std::list< io_object_t > m_deviceMatchList
virtual void DeviceRemoved(io_iterator_t iterator)
Subclass of DeviceNotifier that notifies clients when Kona/Corvid/Io/TTap devices are attached/detach...
virtual bool Install(CFMutableDictionaryRef dict=NULL)
virtual bool Install(CFMutableDictionaryRef dict=NULL)
virtual void SetCallback(DeviceClientCallback callback, void *refcon)
static void DeviceAddedCallback(DeviceNotifier *thisObject, io_iterator_t iterator)
void(* DeviceClientCallback)(unsigned long message, void *refcon)
Mac-specific device add/change/remove event notification callback function.
virtual void DeviceAdded(io_iterator_t iterator)
static std::string MessageTypeToStr(const natural_t messageType)
std::list< io_object_t > m_deviceInterestList
KonaNotifier(DeviceClientCallback callback, void *refcon)
virtual ~DeviceNotifier()
CFMutableDictionaryRef m_matchingDictionary
static void DeviceRemovedCallback(DeviceNotifier *thisObject, io_iterator_t iterator)
virtual void AddGeneralInterest(io_object_t service)
virtual void DeviceChanged(io_service_t unitService, natural_t messageType, void *message)
IONotificationPortRef m_notificationPort
static void DeviceChangedCallback(DeviceNotifier *thisObject, io_service_t unitService, natural_t messageType, void *message)
DeviceClientCallback m_clientCallback
virtual CFMutableDictionaryRef CreateMatchingDictionary()
Mac-specific class that notifies clients when AJA devices are attached/detached, etc.
DeviceNotifier(DeviceClientCallback callback, void *refcon)