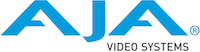 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
9 #if defined(AJA_WINDOWS)
11 #elif defined(AJA_LINUX)
13 #elif defined(AJA_MAC)
15 #elif defined(AJA_BAREMETAL)
30 typedef std::vector<string> ValueLines;
31 typedef ValueLines::const_iterator ValueLinesConstIter;
32 const string gutterStr (inGutterWidth,
' ');
36 size_t longestLabelLen(0);
38 if (it->first.length() > longestLabelLen)
39 longestLabelLen = it->first.length();
46 static const string lineBreakChars(
"\r\n");
47 string label(it->first), value(it->second);
48 const bool hasLineBreaks (value.find_first_of(lineBreakChars) != string::npos);
49 if (value.empty() && it != inLabelValuePairs.begin())
54 if (!hasLineBreaks && !inMaxValWidth)
56 oss << setw(
int(longestLabelLen)) << left << label << gutterStr << value << endl;
61 ValueLines valueLines, finalLines;
64 static const string lineBreakDelims[] = {
"\r\n",
"\r",
"\n"};
65 aja::replace(value, lineBreakDelims[0], lineBreakDelims[2]);
66 aja::replace(value, lineBreakDelims[1], lineBreakDelims[2]);
67 valueLines =
aja::split(value, lineBreakDelims[2][0]);
70 valueLines.push_back(value);
72 for (ValueLinesConstIter iter(valueLines.begin()); iter != valueLines.end(); ++iter)
74 const string & lineStr(*iter);
78 finalLines.push_back(lineStr.substr(pos, inMaxValWidth));
80 }
while (pos < lineStr.length());
83 finalLines = valueLines;
85 const string wrapIndentStr (longestLabelLen + inGutterWidth,
' ');
86 for (
size_t ndx(0); ndx < finalLines.size(); ndx++)
88 const string & valStr(finalLines.at(ndx));
90 oss << wrapIndentStr << valStr << endl;
92 oss << setw(
int(longestLabelLen)) << left << label << gutterStr << valStr << endl;
145 ret = mpImpl->Rescan(sections);
154 if (mpImpl && mpImpl->mValueMap.count(
int(tag)) != 0)
156 outValue = mpImpl->mValueMap[int(tag)];
165 if (mpImpl && mpImpl->mLabelMap.count(
int(tag)) != 0)
167 outLabel = mpImpl->mLabelMap[int(tag)];
183 append(outTable, label, value);
192 append(infoTable,
"HOST INFO");
193 GetLabelValuePairs(infoTable,
false);
194 return ToString(infoTable, inValueWrapLen, inGutterWidth);
199 allLabelsAndValues = ToString();
210 string label(inData.first);
211 const string & value(inData.second);
215 if (label.at(label.length()-1) ==
':')
216 label.resize(label.length()-1);
218 outStream << label <<
"=" << value;
227 if (++it != inData.end())
Declares the AJASystemInfo class.
@ AJA_SystemInfoTag_System_Bios
std::string & strip(std::string &str, const std::string &ws)
void split(const std::string &str, const char delim, std::vector< std::string > &elems)
@ AJA_SystemInfoTag_Path_Firmware
@ AJA_SystemInfoTag_OS_KernelVersion
@ AJA_SystemInfoTag_Path_Applications
@ AJA_SystemInfoTag_CPU_Type
@ AJA_SystemInfoTag_CPU_NumCores
std::string & replace(std::string &str, const std::string &from, const std::string &to)
virtual AJAStatus GetValue(const AJASystemInfoTag inTag, std::string &outValue) const
Answers with the host system info value string for the given AJASystemInfoTag.
@ AJA_SystemInfoTag_System_Name
AJASystemInfo(const AJASystemInfoMemoryUnit inUnits=AJA_SystemInfoMemoryUnit_Megabytes, AJASystemInfoSections sections=AJA_SystemInfoSection_All)
Default constructor that instantiates the platform-specific implementation, then calls Rescan.
@ AJA_SystemInfoTag_System_BootTime
@ AJA_SystemInfoTag_OS_Version
std::pair< std::string, std::string > AJALabelValuePair
A pair of strings comprising a label and a value.
@ AJA_SystemInfoTag_Mem_Used
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
virtual AJAStatus GetLabelValuePairs(AJALabelValuePairs &outTable, bool clearTable=false) const
Generates a "table" of label/value pairs that contains the complete host system info table.
virtual AJAStatus Rescan(AJASystemInfoSections sections=AJA_SystemInfoSection_All)
#define AJA_SUCCESS(_status_)
@ AJA_SystemInfoTag_Path_PersistenceStoreUser
AJALabelValuePairs::const_iterator AJALabelValuePairsConstIter
@ AJA_SystemInfoTag_Path_PersistenceStoreSystem
@ AJA_SystemInfoTag_OS_ProductName
Declares the AJASystemInfoImpl class.
ostream & operator<<(ostream &outStream, const AJASystemInfo &inData)
@ AJA_SystemInfoTag_Path_Utilities
@ AJA_SystemInfoTag_Path_UserHome
@ AJA_SystemInfoTag_Mem_Free
@ AJA_SystemInfoTag_GPU_Type
Declares the AJASystemInfoImpl class.
@ AJA_SystemInfoTag_OS_VersionBuild
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
virtual AJAStatus GetLabel(const AJASystemInfoTag inTag, std::string &outLabel) const
Answers with the host system info label string for the given AJASystemInfoTag.
@ AJA_SystemInfoTag_Mem_Total
Declares the AJASystemInfoImpl class.
@ AJA_SystemInfoTag_System_Model