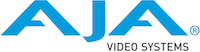 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
7 #ifndef __NTV2_CEA608_DECODECHANNEL_
8 #define __NTV2_CEA608_DECODECHANNEL_
86 virtual void Reset (
void);
124 virtual bool Parse608Data (
const UByte inByte1,
const UByte inByte2, std::string & outDebugStr);
160 virtual std::string GetOnAirCharacter (
const UWord inRow,
const UWord inCol)
const;
171 virtual std::string GetOnAirCharacterWithAttributes (
const UWord inRow,
const UWord inCol,
NTV2Line21Attrs & outAttr)
const;
209 virtual bool SetTextModeDisplayRowCount (
const UWord inNumRows);
229 virtual std::string GetDebugPrintRow (
const UWord inRow,
const bool inShowChars =
true)
const;
231 virtual std::string GetDebugPrintScreen (
const bool inShowChars =
true)
const;
232 virtual void SetDebugRowsOfInterest (
const UWord inFromRow,
const UWord inToRow,
const bool inAdd =
false);
233 virtual void SetDebugColumnsOfInterest (
const UWord inFromCol,
const UWord inToCol,
const bool inAdd =
false);
238 virtual std::vector<uint32_t> GetStats(
void)
const;
246 virtual bool Parse608CharacterData (
UByte char608_1,
UByte char608_2, std::string & outDebugStr);
247 virtual bool Parse608TabOffsetCommand (
UByte char608_1,
UByte char608_2, std::string & outDebugStr);
248 virtual bool Parse608CharacterSetCommand (
UByte char608_1,
UByte char608_2, std::string & outDebugStr);
249 virtual bool Parse608AttributeCommand (
UByte char608_1,
UByte char608_2, std::string & outDebugStr);
250 virtual bool Parse608PACCommand (
UByte char608_1,
UByte char608_2, std::string & outDebugStr);
251 virtual bool Parse608MidRowCommand (
UByte char608_1,
UByte char608_2, std::string & outDebugStr);
252 virtual bool Parse608SpecialCharacter (
UByte char608_1,
UByte char608_2, std::string & outDebugStr);
253 virtual bool Parse608MiscCommand (
UByte char608_1,
UByte char608_2, std::string & outDebugStr);
255 virtual bool DoResumeCaptionLoading (
void);
256 virtual bool DoBackspace (
void);
257 virtual bool DoDeleteToEndOfRow (
void);
258 virtual bool DoRollUpCaption (
const UWord inRows);
259 virtual bool DoFlashOn (
void);
260 virtual bool DoResumeDirectCaptioning (
void);
261 virtual bool DoTextRestart (
void);
262 virtual bool DoResumeTextDisplay (
void);
263 virtual bool DoEraseDisplayedMemory (
void);
264 virtual bool DoCarriageReturn (
void);
265 virtual bool DoEraseNonDisplayedMemory (
void);
266 virtual bool DoEndOfCaption (
void);
278 virtual bool EraseScreen (
const UWord screen);
280 virtual bool SetRow (
const UWord inNewRow);
281 virtual bool IncrementRow (
const int inDelta = 1);
283 virtual bool SetColumn (
const UWord inNewCol);
284 virtual bool IncrementColumn (
const int inDelta = 1);
286 virtual bool SetCaptionMode (
const NTV2Line21Mode inNewCaptionMode);
290 virtual bool SetCurrentScreen (
const UWord inNewScreen);
292 virtual void MoveRollUpWindow (
const UWord inNewBaseRow);
295 virtual void InsertCharacter (
const UByte char608_1,
const UByte char608_2);
296 virtual void InsertTextCharacter (
const UByte inASCIIChar);
300 virtual void Notify_CurrentRowChanged (
const UWord inOldRow,
const UWord inNewRow)
const;
301 virtual void Notify_CurrentColumnChanged (
const UWord inOldCol,
const UWord inNewCol)
const;
302 virtual void Notify_CurrentScreenChanged (
const UWord inOldScreen,
const UWord inNewScreen)
const;
304 virtual void Notify_ScreenCharChanged (
const UWord inScreenNum,
const UWord inRow,
const UWord inCol,
306 virtual void Notify_ScreenAttrChanged (
const UWord inScreenNum,
const UWord inRow,
const UWord inCol,
323 mutable void * mpScreenLock;
344 std::vector<uint32_t> mStats;
354 #if defined(AJA_DEBUG) // DEBUG BUILDS ONLY: ClassTest and InstanceTest
355 static bool ClassTest (
void);
356 bool InstanceTest (
void);
365 #endif // __NTV2_CEA608_DECODECHANNEL_
Declares several data types used with 608/SD captioning.
virtual void SetTextModeDisplayAttributes(const NTV2Line21Attrs &inAttributes)
Sets the display attributes that Text Mode captions will use henceforth.
I am a reference-counted pointer template class. I am intended to be a proxy for an underlying object...
@ kTotalMidRowCmds
Total Mid-Row commands parsed (color, underline, italic, flash, etc.)
virtual NTV2Line21Mode GetCaptionMode(void) const
@ kTotalMiscCmds
Total Misc commands parsed (RollUp, FlashOn, RCL, BS, EDM, EOC, etc.)
Line21RowSet Line21ColumnSet
A set of caption column numbers.
static bool sStaticBackBufferTestMode
virtual UWord GetCurrentScreen(void) const
enum _CaptionDecode608Stats CaptionDecode608Stats
The currently supported caption decoder stats.
virtual UWord GetColumn(void) const
Answers with the column at which the next caption character will be inserted.
std::set< int > Line21RowSet
A set of caption row numbers.
Declares the AJALock class.
bool Check608Parity(UByte char608_1, UByte char608_2, std::string &outDebugStr)
virtual NTV2Line21CharacterSet GetCurrentCharacterSet(void) const
Answers with my current character set.
_CaptionDecode608Stats
The currently supported caption decoder stats.
enum NTV2Line21CharacterSet NTV2Line21CharSet
NTV2_CC608_CodePoint NTV2CC608CodePoint
@ kParsedOKTally
Total Parse608Data successes.
@ kTotalCharData
Total plain ol' characters parsed.
UByte Add608OddParity(UByte ch)
@ kTotalAttribCmds
Total attribute commands parsed.
AJARefPtr< CNTV2CaptionDecodeChannel608 > CNTV2CaptionDecodeChannel608Ptr
@ kTotalTabOffsetCmds
Total tab offset commands parsed.
#define IsLine21TextChannel(_chan_)
const UWord NTV2_CC608_MaxCol(32)
The maximum column index number (located at the right edge of the screen).
@ kParseFailTally
Total Parse608Data failures.
ULWord NTV2_CC608_CodePoint
Describes a unique CEA-608 caption character code point in 32 bits: 0xSS00XXYY, where....
void() NTV2Caption608Changed(void *pInstance, const NTV2Caption608ChangeInfo &inChangeInfo)
This callback is used to respond to dynamic events that occur during CEA-608 caption decoding.
NTV2Line21Mode
The CEA-608 modes: pop-on, roll-up (2, 3 and 4-line), and paint-on.
virtual UWord GetRow(void) const
Answers with the row at which the next caption character will be inserted.
@ kTotalSpecialChars
Total special characters parsed.
virtual UWord GetTextModeDisplayRowCount(void) const
Returns the number of rows used for displaying Text Mode captions (Tx1/Tx2/Tx3/Tx4).
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
virtual bool IsTextChannel(void) const
Answers true if the caption channel I'm currently handling is Tx1, Tx2, Tx3 or Tx4.
virtual const NTV2Line21Attrs & GetTextModeDisplayAttributes(void) const
Returns the display attributes that Text Mode captions are currently using (assuming my caption chann...
@ kTotalPACCmds
Total PAC (preamble address code) commands parsed.
NTV2Line21Field
The two CEA-608 interlace fields.
CEA-608 Character Attributes.
@ kTotalCharSetCmds
Total character set commands parsed.
NTV2Line21CharacterSet
The available CEA-608 character sets.
Defines the AJARefPtr template class.
Declares the NTV2CaptionLogMask, and the CNTV2CaptionLogConfig class.
const UWord NTV2_CC608_MaxRow(15)
The maximum permissible row index number (located at the bottom of the screen).