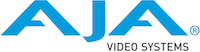 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
7 #ifndef __NTV2_CEA608_DECODER_
8 #define __NTV2_CEA608_DECODER_
18 #define odprintf printf
83 static const int CharacterFlashCycleFrames = 30;
95 virtual void Reset (
void);
117 virtual void IdleFrame (
void);
128 virtual bool ProcessNew608FrameData (
const CaptionData & inCC608Data);
146 virtual UByte GetOnAirCharacterWithAttributes (
const UWord inRow,
161 virtual UWord GetOnAirUTF16CharacterWithAttributes (
const UWord inRow,
177 virtual std::string GetOnAirCharacter (
const UWord inRow,
205 virtual std::string GetOnAirCharacters (
const UWord inRowNumber = 0)
const;
291 virtual inline bool IsFlashCycleOff (
void)
const {
return mFlashCount > CharacterFlashCycleFrames / 2;}
301 #if !defined(NTV2_DEPRECATE_16_0)
303 #endif // !defined(NTV2_DEPRECATE_16_0)
304 #if !defined(NTV2_DEPRECATE_16_2)
306 #endif // !defined(NTV2_DEPRECATE_16_2)
325 virtual void DebugPrintCurrentScreen (
const bool inAllChannels =
false,
const bool inShowChars =
true,
const bool inShowTextChannels =
false);
327 virtual void SetDebugRowsOfInterest (
const NTV2Line21Channel inChannel,
const UWord inFromRow,
const UWord inToRow,
const bool inAdd =
false);
328 virtual void SetDebugColumnsOfInterest (
const NTV2Line21Channel inChannel,
const UWord inFromCol,
const UWord inToCol,
const bool inAdd =
false);
335 static bool UseNewBurnCaptionsMethod;
349 typedef std::vector <CNTV2CaptionDecodeChannel608Ptr> ChannelDecoderArray;
354 ChannelDecoderArray mChannelDecoders;
359 unsigned short mLastControlCode [2];
363 void * mpSubscriberData;
364 #if defined (AJA_DEBUG)
366 static bool ClassTest (
void);
368 bool InstanceTest (
void);
373 #endif // __NTV2_CEA608_DECODER_
static bool AutoCallIdleFrame
Default is false. If true, ProcessNew608FrameData will automatically call IdleFrame.
Describes the horizontal and vertical size dimensions of a raster, bitmap, frame or image.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
virtual bool IsFlashCycleOff(void) const
static bool AutoFlashOnAirChars
Default is false. If true, GetOnAir... functions will automatically "flash" (periodically return spac...
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
AJARefPtr< CNTV2CaptionDecoder608 > CNTV2CaptionDecoder608Ptr
@ NTV2_FG_720x486
720x486, for NTSC 525i and 525p60, NTV2_VANCMODE_OFF
Declares the CNTV2XDSDecodeChannel608 class.
This class is used to respond to dynamic events that occur during CEA-608 caption decoding.
virtual NTV2CaptionLogMask SetLogMask(const NTV2CaptionLogMask inLogMask)
Specifies what, if any, debug information I will write to my log stream.
static bool RenderPrePostSpaces
Default is true. If true, BurnCaptions leads & follows character runs with blank space.
Declares the CNTV2CaptionDecodeChannel608 class.
void() NTV2Caption608Changed(void *pInstance, const NTV2Caption608ChangeInfo &inChangeInfo)
This callback is used to respond to dynamic events that occur during CEA-608 caption decoding.
#define NTV2_DEPRECATED_f(__f__)
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
NTV2FrameGeometry
Identifies a particular video frame geometry.
uint64_t NTV2CaptionLogMask
Selectors to control what information is logged.
NTV2Line21Field
The two CEA-608 interlace fields.
CEA-608 Character Attributes.
This structure encapsulates all possible CEA-608 caption data bytes that may be associated with a giv...
Defines the AJARefPtr template class.
virtual std::string GetOnAirCharacter(const UWord inRow, const UWord inCol) const
Retrieves the "on-air" character at the given on-screen row and column position.
virtual NTV2Line21Channel GetDisplayChannel(void) const
Answers with the caption channel that I'm currently focused on (or that I'm currently "burning" into ...