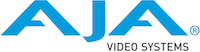 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
14 #if defined (AJALinux)
17 #if defined(AJAANCLISTIMPL_VECTOR)
23 #define LOGGING_ANCLIST AJADebug::IsActive(AJA_DebugUnit_AJAAncList)
24 #define LOGGING_ANC2110RX AJADebug::IsActive(AJA_DebugUnit_Anc2110Rcv)
25 #define LOGGING_ANC2110TX AJADebug::IsActive(AJA_DebugUnit_Anc2110Xmit)
27 #define LOGMYERROR(__x__) {if (LOGGING_ANCLIST) AJA_sERROR (AJA_DebugUnit_AJAAncList, AJAFUNC << ": " << __x__);}
28 #define LOGMYWARN(__x__) {if (LOGGING_ANCLIST) AJA_sWARNING(AJA_DebugUnit_AJAAncList, AJAFUNC << ": " << __x__);}
29 #define LOGMYNOTE(__x__) {if (LOGGING_ANCLIST) AJA_sNOTICE (AJA_DebugUnit_AJAAncList, AJAFUNC << ": " << __x__);}
30 #define LOGMYINFO(__x__) {if (LOGGING_ANCLIST) AJA_sINFO (AJA_DebugUnit_AJAAncList, AJAFUNC << ": " << __x__);}
31 #define LOGMYDEBUG(__x__) {if (LOGGING_ANCLIST) AJA_sDEBUG (AJA_DebugUnit_AJAAncList, AJAFUNC << ": " << __x__);}
33 #define RCVFAIL(__x__) {if (LOGGING_ANC2110RX) AJA_sERROR (AJA_DebugUnit_Anc2110Rcv, AJAFUNC << ": " << __x__);}
34 #define RCVWARN(__x__) {if (LOGGING_ANC2110RX) AJA_sWARNING(AJA_DebugUnit_Anc2110Rcv, AJAFUNC << ": " << __x__);}
35 #define RCVNOTE(__x__) {if (LOGGING_ANC2110RX) AJA_sNOTICE (AJA_DebugUnit_Anc2110Rcv, AJAFUNC << ": " << __x__);}
36 #define RCVINFO(__x__) {if (LOGGING_ANC2110RX) AJA_sINFO (AJA_DebugUnit_Anc2110Rcv, AJAFUNC << ": " << __x__);}
37 #define RCVDBG(__x__) {if (LOGGING_ANC2110RX) AJA_sDEBUG (AJA_DebugUnit_Anc2110Rcv, AJAFUNC << ": " << __x__);}
39 #define XMTFAIL(__x__) {if (LOGGING_ANC2110TX) AJA_sERROR (AJA_DebugUnit_Anc2110Xmit, AJAFUNC << ": " << __x__);}
40 #define XMTWARN(__x__) {if (LOGGING_ANC2110TX) AJA_sWARNING(AJA_DebugUnit_Anc2110Xmit, AJAFUNC << ": " << __x__);}
41 #define XMTNOTE(__x__) {if (LOGGING_ANC2110TX) AJA_sNOTICE (AJA_DebugUnit_Anc2110Xmit, AJAFUNC << ": " << __x__);}
42 #define XMTINFO(__x__) {if (LOGGING_ANC2110TX) AJA_sINFO (AJA_DebugUnit_Anc2110Xmit, AJAFUNC << ": " << __x__);}
43 #define XMTDBG(__x__) {if (LOGGING_ANC2110TX) AJA_sDEBUG (AJA_DebugUnit_Anc2110Xmit, AJAFUNC << ": " << __x__);}
45 #if defined(AJAHostIsBigEndian)
47 #define AJA_ENDIAN_16NtoH(__val__) (__val__)
48 #define AJA_ENDIAN_16HtoN(__val__) (__val__)
49 #define AJA_ENDIAN_32NtoH(__val__) (__val__)
50 #define AJA_ENDIAN_32HtoN(__val__) (__val__)
51 #define AJA_ENDIAN_64NtoH(__val__) (__val__)
52 #define AJA_ENDIAN_64HtoN(__val__) (__val__)
55 #define AJA_ENDIAN_16NtoH(__val__) AJA_ENDIAN_SWAP16(__val__)
56 #define AJA_ENDIAN_16HtoN(__val__) AJA_ENDIAN_SWAP16(__val__)
57 #define AJA_ENDIAN_32NtoH(__val__) AJA_ENDIAN_SWAP32(__val__)
58 #define AJA_ENDIAN_32HtoN(__val__) AJA_ENDIAN_SWAP32(__val__)
59 #define AJA_ENDIAN_64NtoH(__val__) AJA_ENDIAN_SWAP64(__val__)
60 #define AJA_ENDIAN_64HtoN(__val__) AJA_ENDIAN_SWAP64(__val__)
68 unsigned numPrinted (0);
69 inOutStream <<
DECN(inData.size(),3) <<
" U32s: ";
74 if (++iter != inData.end())
76 if (numPrinted > inMaxNum)
77 {inOutStream <<
"...";
break;}
93 unsigned rtpPktNum(0);
96 inOutStream <<
"RTP PKT " <<
DEC0N(rtpPktNum,3) <<
":";
106 { inOutStream <<
xHEX0N(*it,4);
107 if (++it != inSet.end())
153 m_rcvMultiRTP (
true),
154 m_xmitMultiRTP (
false),
177 m_xmitMultiRTP = inRHS.m_xmitMultiRTP;
178 m_rcvMultiRTP = inRHS.m_rcvMultiRTP;
179 m_ignoreCS = inRHS.m_ignoreCS;
193 if (!m_ancList.empty() && inIndex < m_ancList.size())
197 for (uint32_t i(0); i < inIndex; i++)
231 if (matchType == ancType)
248 if (matchType == ancType)
310 if (result.find(pktDIDSID) == result.end())
311 result.insert(pktDIDSID);
319 if (&inPackets ==
this)
329 m_ancList.push_back(pNewPkt);
343 const bool wasEmpty (m_ancList.empty());
350 m_ancList.push_back(pData);
352 LOGMYDEBUG(
DEC(m_ancList.size()) <<
" packet(s) stored" << (wasEmpty ?
" from" :
" after appending") <<
" packet " << pData->
AsString(32));
359 uint32_t numDeleted (0);
360 const uint32_t oldSize (uint32_t(m_ancList.size()));
372 if (oldSize || numDeleted)
373 LOGMYDEBUG(numDeleted <<
" packet(s) deleted -- list emptied");
383 #if defined(AJAANCLISTIMPL_VECTOR)
385 for (it = m_ancList.begin(); it != m_ancList.end(); ++it)
388 if (it == m_ancList.end())
392 m_ancList.remove(pAncData);
395 LOGMYDEBUG(
DEC(m_ancList.size()) <<
" packet(s) remain after removing packet " << pAncData->
AsString(32));
416 return lhs->GetDID() < rhs->GetDID();
422 return lhs->GetSID() < rhs->GetSID();
429 return locLHS < locRHS;
437 #if defined(AJAANCLISTIMPL_VECTOR)
438 std::sort(m_ancList.begin(), m_ancList.end(),
SortByDID);
448 #if defined(AJAANCLISTIMPL_VECTOR)
449 std::sort(m_ancList.begin(), m_ancList.end(),
SortBySID);
458 #if defined(AJAANCLISTIMPL_VECTOR)
494 const string info (pPkt->
CompareWithInfo(*pPktRHS, inIgnoreLocation, inIgnoreChecksum));
512 outDiffInfo.push_back(oss.str());
520 const string info (pPkt->CompareWithInfo(*pPktRHS, inIgnoreLocation, inIgnoreChecksum));
525 <<
"LHS " << pPkt->AsString(250) << endl
526 <<
"RHS " << pPktRHS->AsString(250) << endl
528 outDiffInfo.push_back(oss.str());
531 return outDiffInfo.empty();
551 int32_t remainingSize (int32_t(inReceivedData.
GetByteCount()));
552 const uint8_t * pInputData (inReceivedData);
553 bool bMoreData (
true);
557 bool bInsertNew (
false);
559 uint32_t packetSize (0);
563 status = newAncData.
InitWithReceivedData (pInputData,
size_t(remainingSize), defaultLoc, packetSize);
569 else if (packetSize == 0)
573 if (newAncData.IsDigital())
580 else if (newAncData.IsRaw())
594 const uint64_t desiredLocation (newAncData.GetDataLocation().OrdinalValue());
597 if ((*pRaw)->GetDataLocation().OrdinalValue() == desiredLocation)
601 pContinuationPkt = *pRaw;
606 if (!pContinuationPkt)
628 rawPkts.push_back(pData);
630 m_ancList.push_back(pData);
634 if (inFrameNum && !pData->GetFrameID())
641 remainingSize -= packetSize;
642 pInputData += packetSize;
643 if (remainingSize <= 0)
652 m_ancList.push_back(rawPkts.back());
668 const uint32_t inFrameNum)
671 const uint8_t * pRcvData = inReceivedData;
672 const uint32_t dataSize = inReceivedData.
GetByteCount();
673 if (!pRcvData || !dataSize)
678 int32_t remainingSize (int32_t(dataSize + 0));
679 const uint8_t * pInputData (pRcvData);
680 bool bMoreData (
true);
684 uint32_t packetSize (0);
691 else if (packetSize == 0)
701 try {m_ancList.push_back(pData);}
705 if (inFrameNum && !pData->GetFrameID())
712 remainingSize -= packetSize;
713 pInputData += packetSize;
714 if (remainingSize <= 0)
727 if (inReceivedData.empty())
735 {
LOGMYERROR(
"AJARTPAncPayloadHeader::ReadULWordVector failed, " <<
DEC(4*inReceivedData.size()) <<
" header bytes");
return AJA_STATUS_FAIL;}
741 const size_t predictedPayloadSize (RTPheader.GetPayloadLength() /
sizeof(uint32_t));
742 const size_t actualPayloadSize (inReceivedData.size() - AJARTPAncPayloadHeader::GetHeaderWordCount());
743 const uint32_t numPackets (RTPheader.GetAncPacketCount());
744 uint32_t pktsAdded (0);
747 if (actualPayloadSize < predictedPayloadSize)
751 if (!actualPayloadSize)
759 for (; pktNum < numPackets &&
AJA_SUCCESS(status); pktNum++)
772 pNewPkt->
SetFrameID(RTPheader.GetTimeStamp());
775 try {m_ancList.push_back(pNewPkt); pktsAdded++;}
784 {
LOGMYWARN(
DEC(pktsAdded) <<
" of " <<
DEC(numPackets) <<
" anc pkt(s) decoded from RTP pkt");}
786 LOGMYINFO(
DEC(numPackets) <<
" pkts added from RTP pkt: " << *
this);
800 if (inPacketWords.size () < 7)
820 outGumpPkt.reserve (outGumpPkt.size() + inPacketWords.size());
821 const UByteSequence::size_type dataByte1Ndx (outGumpPkt.size()+1);
822 outGumpPkt.push_back(0xFF);
823 outGumpPkt.push_back(0x80);
824 outGumpPkt[dataByte1Ndx] |= (inLoc.GetLineNumber() >> 7) & 0x0F;
825 if (inLoc.IsLumaChannel())
826 outGumpPkt[dataByte1Ndx] |= 0x20;
828 outGumpPkt[dataByte1Ndx] |= 0x10;
829 outGumpPkt.push_back (inLoc.GetLineNumber() & 0x7F);
831 while (iter != inPacketWords.end())
833 outGumpPkt.push_back(*iter & 0xFF);
864 try {m_ancList.push_back(pData);}
867 if (inFrameNum && pData->GetDID())
883 const uint32_t inFrameNum)
888 LOGMYERROR(
"AJA_STATUS_NULL: NULL frame buffer pointer");
893 LOGMYERROR(
"AJA_STATUS_BAD_PARAM: bad NTV2FormatDescriptor");
898 LOGMYERROR(
"AJA_STATUS_BAD_PARAM: format descriptor has no VANC lines");
907 LOGMYERROR(
"AJA_STATUS_FAIL: " << inFB.
GetByteCount() <<
"-byte frame buffer smaller than " << vancBytes <<
"-byte VANC region");
929 uwords, inFD.GetRasterWidth());
932 uwords, inFD.GetRasterWidth());
940 NTV2_ASSERT(ycPackets.size() == ycHOffsets.size());
942 for (AJAAncillaryData::U16Packets::const_iterator it(ycPackets.begin()); it != ycPackets.end(); ++it, ndx++)
958 for (AJAAncillaryData::U16Packets::const_iterator it(yPackets.begin()); it != yPackets.end(); ++it, ndxx++)
962 for (AJAAncillaryData::U16Packets::const_iterator it(cPackets.begin()); it != cPackets.end(); ++it, ndxx++)
973 const uint32_t inFrameNum)
975 uint32_t RTPPacketCount (0);
990 size_t ULWordCount (0);
991 size_t ULWordOffset (0);
992 unsigned retries (0);
993 const unsigned MAX_RETRIES (4);
995 while (ancBuffer && retries++ < MAX_RETRIES)
1007 RCVWARN(
"On RTP pkt " <<
DEC(RTPPacketCount) <<
", RTP hdr ReadFromBuffer failed at: " << ancBuffer.
AsString(40));
1011 ULWordCount = rtpHeader.GetPayloadLength() /
sizeof(uint32_t)
1012 + AJARTPAncPayloadHeader::GetHeaderWordCount();
1015 if (!ancBuffer.
GetU32s (U32s, 0, ULWordCount))
1017 RCVFAIL(
"On RTP pkt " <<
DEC(RTPPacketCount) <<
", GetU32s(" <<
DEC(ULWordCount) <<
") at: " << ancBuffer.
AsString(40));
1035 ULWordOffset += ULWordCount;
1037 inAncBuffer.
GetByteCount() - ULWordOffset *
sizeof(uint32_t));
1038 RCVDBG(
"Moved buffer " << inAncBuffer <<
" forward by " <<
DEC(ULWordCount) <<
" U32s: " << ancBuffer.
AsString(20));
1044 {
LOGMYDEBUG(
"Success: " <<
DEC(pktsAdded) <<
" pkts added");}
1054 const uint32_t inFrameNum)
1067 {
LOGMYDEBUG(
"Success: " <<
DEC(pktsAdded) <<
" pkts added");}
1079 const uint32_t inFrameNum)
1096 const uint32_t inFrameNum)
1118 const bool inIsProgressive,
const uint32_t inF2StartLine)
1121 uint32_t actF1PktCnt (0);
1122 uint32_t actF2PktCnt (0);
1123 uint32_t pktNdx (0);
1124 size_t oldPktLengthWords (0);
1125 unsigned countOverflows (0);
1126 size_t overflowWords (0);
1131 outF1AncCounts.clear(); outF2AncCounts.clear();
1135 if (!inIsProgressive)
1137 F1U32s.clear(); F2U32s.clear();
1153 if (inIsProgressive || pkt.GetLocationLineNumber() < inF2StartLine)
1161 oldPktLengthWords = F1U32s.size();
1167 overflowWords += F1U32s.size() - oldPktLengthWords;
1169 while (F1U32s.size() > oldPktLengthWords)
1176 outF1U32Pkts.push_back(F1U32s);
1177 outF1AncCounts.push_back(1);
1190 oldPktLengthWords = F2U32s.size();
1196 overflowWords += F2U32s.size() - oldPktLengthWords;
1198 while (F2U32s.size() > oldPktLengthWords)
1205 outF2U32Pkts.push_back(F2U32s);
1206 outF2AncCounts.push_back(1);
1217 outF1U32Pkts.push_back(F1U32s);
1218 outF1AncCounts.push_back(uint8_t(actF1PktCnt));
1219 outF2U32Pkts.push_back(F2U32s);
1220 outF2AncCounts.push_back(uint8_t(actF2PktCnt));
1222 if (overflowWords && countOverflows)
1223 {
LOGMYWARN(
"Overflow: " <<
DEC(countOverflows) <<
" pkts skipped, " <<
DEC(overflowWords) <<
" U32s dropped");}
1224 else if (overflowWords)
1225 {
LOGMYWARN(
"Data overflow: " <<
DEC(overflowWords) <<
" U32s dropped");}
1226 else if (countOverflows)
1227 LOGMYWARN(
"Packet overflow: " <<
DEC(countOverflows) <<
" pkts skipped");
1228 XMTDBG(
"F1 (Content Only): " << outF1U32Pkts);
1229 XMTDBG(
"F2 (Content Only): " << outF2U32Pkts);
1243 uint32_t f1Size (0);
1244 uint32_t f2Size (0);
1249 uint32_t packetSize (0);
1254 if (bProgressive || pAncData->GetLocationLineNumber () < f2StartLine)
1255 f1Size += packetSize;
1257 f2Size += packetSize;
1268 NTV2Buffer F1Buffer(pF1AncData, inMaxF1Data), F2Buffer(pF2AncData, inMaxF2Data);
1269 if (!F1Buffer.IsNULL())
1273 return GetTransmitData(F1Buffer, F2Buffer, bProgressive, f2StartLine);
1278 const bool inIsProgressive,
const uint32_t inF2StartLine)
1283 uint8_t * pF1AncData (
reinterpret_cast<uint8_t*
>(F1Buffer.
GetHostPointer()));
1284 uint8_t * pF2AncData (
reinterpret_cast<uint8_t*
>(F2Buffer.
GetHostPointer()));
1286 F1Buffer.
Fill(uint64_t(0)); F2Buffer.
Fill(uint64_t(0));
1294 uint32_t pktSize(0);
1299 if (inIsProgressive || pPkt->GetLocationLineNumber() < inF2StartLine)
1301 if (pF1AncData && maxF1Data)
1307 pF1AncData += pktSize;
1308 maxF1Data -= pktSize;
1311 else if (pF2AncData && maxF2Data)
1317 pF2AncData += pktSize;
1318 maxF2Data -= pktSize;
1327 if (inFrameBuffer.
IsNULL())
1329 LOGMYERROR(
"AJA_STATUS_NULL: null frame buffer");
1334 LOGMYERROR(
"AJA_STATUS_BAD_PARAM: Invalid format descriptor");
1337 if (!inFormatDesc.IsVANC())
1339 LOGMYERROR(
"AJA_STATUS_BAD_PARAM: Not a VANC geometry");
1344 LOGMYERROR(
"AJA_STATUS_UNSUPPORTED: unsupported pixel format: " << inFormatDesc);
1357 const bool isSD (inFormatDesc.IsSD());
1359 set <uint16_t> lineOffsetsWritten;
1364 ULWord smpteLine (0);
bool isF2 (
false);
1370 bool muxedOK (
false);
1381 if (loc.GetLineNumber() != smpteLine)
1388 LOGMYWARN(
"Skipped packet with invalid data channel " << loc);
1401 for (
unsigned ndx(0); ndx < u16PktComponents.size(); ndx++)
1402 pLine[ndx] = uint8_t(u16PktComponents[ndx] & 0xFF);
1407 for (
unsigned srcNdx(0); srcNdx < u16PktComponents.size(); srcNdx++)
1408 pLine[dstNdx + 2*srcNdx] = uint8_t(u16PktComponents[srcNdx] & 0x00FF);
1415 while (u16PktComponents.size() < 12 || u16PktComponents.size() % 12)
1416 u16PktComponents.push_back(0x040);
1430 for (
unsigned srcNdx(0); srcNdx < u16PktComponents.size(); srcNdx++)
1431 YUV16Line[dstNdx + 2*srcNdx] = u16PktComponents[srcNdx] & 0x03FF;
1437 while (YUV16Line.size() < 12 || YUV16Line.size() % 12)
1438 YUV16Line.push_back(0x040);
1449 lineOffsetsWritten.insert(uint16_t(fbLineOffset));
1460 bool success (inFormatDesc.IsSD());
1464 if (!ancData.IsRaw())
1469 if (success && lineOffsetsWritten.find(uint16_t(lineOffset)) != lineOffsetsWritten.end())
1486 lineOffsetsWritten.insert(uint16_t(lineOffset));
1507 const bool inIsF2,
const bool inIsProgressive)
1510 const string sFld (inIsF2 ?
" F2" :
" F1");
1511 const string sPrg (inIsProgressive ?
" Prg" :
" Int");
1512 ULWord u32offset(0), pktNum(1);
1514 outBytesWritten = 0;
1515 if (inRTPPkts.size() != inAncCounts.size())
1519 for (
AJAU32PktsConstIter RTPPktIter(inRTPPkts.begin()); RTPPktIter != inRTPPkts.end(); pktNum++)
1524 const bool isLastRTPPkt (++RTPPktIter == inRTPPkts.end());
1525 const size_t totalRTPPktBytes(AJARTPAncPayloadHeader::GetHeaderByteCount()
1526 + origRTPPkt.size() *
sizeof(uint32_t));
1527 ostringstream pktNumInfo;
1528 pktNumInfo <<
" for RTP pkt " <<
DEC(pktNum) <<
" of " <<
DEC(totPkts);
1531 if (inIsProgressive)
1539 RTPHeader.
SetPayloadLength(uint16_t(origRTPPkt.size() *
sizeof(uint32_t)));
1545 NTV2_ASSERT(RTPHeaderU32s.size() == AJARTPAncPayloadHeader::GetHeaderWordCount());
1550 if (!theBuffer.
PutU32s(RTPHeaderU32s, u32offset))
1551 {
LOGMYERROR(
"RTP hdr WriteBuffer failed for buffer " << theBuffer <<
" at u32offset=" <<
DEC(u32offset)
1554 u32offset +=
ULWord(RTPHeaderU32s.size());
1559 if (!theBuffer.
PutU32s(origRTPPkt, u32offset))
1560 {
LOGMYERROR(
"PutU32s failed writing " <<
DEC(origRTPPkt.size()) <<
" U32s in buffer " << theBuffer <<
" at u32offset=" <<
DEC(u32offset)
1562 LOGMYDEBUG(
"PutU32s OK @u32offset=" <<
xHEX0N(u32offset,4) <<
": " << RTPHeader << pktNumInfo.str());
1566 u32offset +=
ULWord(origRTPPkt.size());
1573 outBytesWritten = u32offset *
ULWord(
sizeof(uint32_t));
1575 LOGMYDEBUG(
DEC(totPkts) <<
" RTP pkt(s), " <<
DEC(u32offset) <<
" U32s (" <<
DEC(outBytesWritten)
1576 <<
" bytes) written for" << sFld << sPrg);
1582 const bool inIsProgressive,
const uint32_t inF2StartLine)
1587 uint32_t byteCount(0);
1590 F1Buffer.
Fill(uint64_t(0)); F2Buffer.
Fill(uint64_t(0));
1594 result =
GetRTPPackets (F1U32Pkts, F2U32Pkts, F1AncCounts, F2AncCounts, inIsProgressive, inF2StartLine);
1605 result =
WriteRTPPackets (F1Buffer, byteCount, F1U32Pkts, F1AncCounts,
false, inIsProgressive);
1610 if (!inIsProgressive)
1611 result =
WriteRTPPackets (F2Buffer, byteCount, F2U32Pkts, F2AncCounts,
true, inIsProgressive);
1619 const bool inIsProgressive,
const uint32_t inF2StartLine)
1621 outF1ByteCount = outF2ByteCount = 0;
1626 AJAStatus result (
GetRTPPackets (F1U32Pkts, F2U32Pkts, F1AncCounts, F2AncCounts, inIsProgressive, inF2StartLine));
1631 result =
WriteRTPPackets (nullBuffer, outF1ByteCount, F1U32Pkts, F1AncCounts,
false, inIsProgressive);
1633 result =
WriteRTPPackets (nullBuffer, outF2ByteCount, F2U32Pkts, F2AncCounts,
true, inIsProgressive);
1646 inOutStream <<
"Pkt" <<
DEC0N(++num,3) <<
": " << pPkt->
AsString(inDumpPayload ? 16 : 0);
1647 if (++it != m_ancList.end())
1648 inOutStream << endl;
1656 if (!inSrc || !outDst)
1661 uint8_t * srcPtr = inSrc;
1662 uint8_t * ptr = srcPtr;
1663 size_t srcBufSize = inSrc;
1664 uint8_t * tgtPtr = outDst;
1665 size_t uncopied = 0;
1668 const size_t kGUMPHeaderSize(7);
1670 for (
size_t ndx(0); ndx < srcBufSize; )
1672 if (ptr[0] == 0xff && (ndx + kGUMPHeaderSize) < srcBufSize)
1674 bool bFiltered{
false};
1675 const uint8_t payloadSize(ptr[5]);
1679 else if (ptr[3] == 0x60 && ptr[4] == 0x60 && payloadSize == 16)
1681 else if (ptr[3] == 0x41 && ptr[4] == 0x01 && payloadSize == 4)
1683 else if (ptr[3] == 0xF4 && ptr[4] == 0x00 && payloadSize == 16)
1687 const uint16_t lineNum (uint16_t((ptr[1] & 0x0F) << 7) + uint16_t(ptr[2] & 0x7F));
1688 bFiltered = (ptr[1] & 0x40) && (lineNum == 14 || lineNum == 277);
1694 ::memcpy(tgtPtr, srcPtr, uncopied);
1697 srcPtr += uncopied + payloadSize + kGUMPHeaderSize;
1699 ndx += payloadSize + kGUMPHeaderSize;
1706 ptr += payloadSize + kGUMPHeaderSize;
1707 ndx += payloadSize + kGUMPHeaderSize;
1708 uncopied += payloadSize + kGUMPHeaderSize;
1712 {ptr++; ndx++; uncopied++;}
1716 ::memcpy(tgtPtr, srcPtr, uncopied);
1774 AJAAncillaryAnalogTypeMap::const_iterator it (
gAnalogTypeMap.find (inLineNum));
1776 ancType = it->second;
static AJALock gGlobalLock
virtual bool AllowMultiRTPReceive(void) const
Answers true if multiple RTP packets are allowed for capture/receive. The default behavior is to proc...
UByteSequence AJAAncPktCounts
Ordered sequence of SMPTE Anc packet counts.
@ AJAAncBufferFormat_FBVANC
Frame buffer VANC lines.
@ AJA_STATUS_BADBUFFERSIZE
static string ULWordSequenceToStringBE(const ULWordSequence &inData, const size_t inMaxNum=32)
static bool SortByDID(AJAAncillaryData *lhs, AJAAncillaryData *rhs)
std::vector< AJAAncillaryData * > AJAAncillaryDataList
ULWordSequence::const_iterator ULWordSequenceConstIter
A handy const iterator for iterating over a ULWordSequence.
static AJAStatus ClearAnalogAncillaryDataTypeMap(void)
Clears my global Analog Ancillary Data Type map.
virtual AJAStatus GetRawPacketSize(uint32_t &outPacketSize) const
Returns the number of "raw" ancillary data bytes that will be generated by AJAAncillaryData::Generate...
Defines where the ancillary data can be found within a video stream.
static AJAStatus AppendUWordPacketToGump(UByteSequence &outGumpPkt, const UWordSequence &inPacketWords, const AJAAncDataLoc inLoc=AJAAncDataLoc(AJAAncDataLink_A, AJAAncDataChannel_Y, AJAAncDataSpace_VANC, 0))
virtual AJAStatus AddVANCData(const UWordSequence &inPacketWords, const AJAAncillaryDataLocation &inLocation, const uint32_t inFrameNum=0)
Adds the packet that originated in the VANC lines of an NTV2 frame buffer to my list.
bool GetU32s(ULWordSequence &outU32s, const size_t inU32Offset=0, const size_t inMaxSize=32, const bool inByteSwap=false) const
Answers with my contents as a vector of unsigned 32-bit values.
virtual AJAStatus Compare(const AJAAncillaryList &inCompareList, const bool inIgnoreLocation=true, const bool inIgnoreChecksum=true) const
Compares me with another list.
virtual AJAStatus GetAncillaryDataTransmitData(const bool inIsProgressive, const uint32_t inF2StartLine, uint8_t *pOutF1AncData, const uint32_t inF1ByteCountMax, uint8_t *pOutF2AncData, const uint32_t inF2ByteCountMax)
Builds one or two ancillary data buffers (one for field 1, one for field 2) with the anc data inserte...
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
static AJAStatus SetAnalogAncillaryDataTypeMap(const AJAAncillaryAnalogTypeMap &inMap)
Copies the given map to the global Analog Ancillary Data Type map.
virtual AJAStatus RemoveAncillaryData(AJAAncillaryData *pInAncData)
Removes all copies of the AJAAncillaryData object from me.
static AJAStatus SetFromDeviceAncBuffers(const NTV2Buffer &inF1AncBuffer, const NTV2Buffer &inF2AncBuffer, AJAAncillaryList &outPackets, const uint32_t inFrameNum=0)
Returns all ancillary data packets found in the given F1 and F2 ancillary data buffers.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
#define ToAJAAncPktDIDSID(_d_, _s_)
ULWord GetByteCount(void) const
#define NTV2_ASSERT(_expr_)
static AJAStatus SetFromDeviceAuxBuffers(const NTV2Buffer &inF1AuxBuffer, const NTV2Buffer &inF2AuxBuffer, AJAAncillaryList &outPackets, const uint32_t inFrameNum=0)
Returns all HDMI Aux data packets found in the given F1 and F2 aux data buffers.
bool IsProvidedByClient(void) const
virtual AJAStatus GetIPTransmitData(NTV2Buffer &F1Buffer, NTV2Buffer &F2Buffer, const bool inIsProgressive=true, const uint32_t inF2StartLine=0)
Explicitly encodes my AJAAncillaryData packets into the given buffers in RTP Anc Buffer Data Format ....
std::vector< uint8_t > UByteSequence
An ordered sequence of UByte (uint8_t) values.
std::set< AJAAncPktDIDSID > AJAAncPktDIDSIDSet
Set of distinct packet DID/SIDs (New in SDK 16.0)
@ AncChannelSearch_C
Only look in chroma samples.
Declares the AJAAncillaryList class.
bool YUVComponentsTo10BitYUVPackedBuffer(const std::vector< uint16_t > &inYCbCrLine, NTV2Buffer &inFrameBuffer, const NTV2FormatDescriptor &inDescriptor, const UWord inLineOffset)
Packs up to one raster line of uint16_t YUV components into an NTV2_FBF_10BIT_YCBCR frame buffer.
static bool GetAncPacketsFromVANCLine(const UWordSequence &inYUV16Line, const AncChannelSearchSelect inChanSelect, U16Packets &outRawPackets, U16Packet &outWordOffsets)
Extracts whatever VANC packets are found inside the given 16-bit YUV line buffer.
AJAU32Pkts::const_iterator AJAU32PktsConstIter
Handy const iterator over AJAU32Pkts.
AJAAncDataType
Identifies the ancillary data types that are known to this module.
virtual uint32_t CountAncillaryDataWithType(const AJAAncDataType inMatchType) const
Answers with the number of AJAAncillaryData objects having the given type.
virtual AJAStatus InitAuxWithReceivedData(const uint8_t *pInData, const size_t inMaxBytes, uint32_t &outPacketByteCount)
Initializes me from "raw" ancillary data received from hardware (ingest) – see SDI Anc Buffer Data Fo...
virtual AJAStatus InitWithReceivedData(const uint8_t *pInData, const size_t inMaxBytes, const AJAAncDataLoc &inLocationInfo, uint32_t &outPacketByteCount)
Initializes me from "raw" ancillary data received from hardware (ingest) – see SDI Anc Buffer Data Fo...
virtual AJAStatus ParseAllAncillaryData(void)
Sends a "ParsePayloadData" command to all of my AJAAncillaryData objects.
#define DEC0N(__x__, __n__)
virtual AJAAncillaryData * GetAncillaryDataWithID(const uint8_t inDID, const uint8_t inSID, const uint32_t inIndex=0) const
Answers with the AJAAncillaryData object having the given DataID and SecondaryID, at the given index.
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
Declaration of the AJAAncillaryDataFactory class.
bool PutU32s(const ULWordSequence &inU32s, const size_t inU32Offset=0, const bool inByteSwap=false)
Copies a vector of unsigned 32-bit values into me.
virtual AJAAncPktDIDSIDSet GetAncillaryPacketIDs(void) const
virtual AJAAncillaryData * GetAncillaryDataWithType(const AJAAncDataType inMatchType, const uint32_t inIndex=0) const
Answers with the AJAAncillaryData object having the given type and index.
static uint32_t ENDIAN_32NtoH(const uint32_t inValue)
#define AJA_ENDIAN_32NtoH(__val__)
I am an ordered collection of AJAAncillaryData instances which represent one or more SMPTE 291 data p...
virtual AJAStatus SortListBySID(void)
Sort the AncillaryDataList by Secondary ID (SID) value.
@ AJAAncBufferFormat_HDMI
HDMI.
#define LOGMYDEBUG(__x__)
static bool Unpack8BitYCbCrToU16sVANCLine(const void *pInYUV8Line, U16Packet &outU16YUVLine, const uint32_t inNumPixels)
Converts a single line of NTV2_FBF_8BIT_YCBCR data from the given source buffer into an ordered seque...
static void SetIncludeZeroLengthPackets(const bool inExclude)
Sets whether or not zero-length packets are included or not.
static AJAAncillaryData * Create(const AJAAncDataType inAncType, const AJAAncillaryData &inAncData)
Creates a new particular subtype of AJAAncillaryData object.
bool IsLumaChannel(void) const
static AJAStatus GetAnalogAncillaryDataTypeMap(AJAAncillaryAnalogTypeMap &outMap)
Returns a copy of the global Analog Ancillary Data Type map.
AJAAncillaryDataList::const_iterator AJAAncDataListConstIter
Handy const iterator for iterating over members of an AJAAncillaryDataList.
std::map< uint16_t, AJAAncDataType > AJAAncillaryAnalogTypeMap
Associates certain frame line numbers with specific types of "raw" or "analog" ancillary data....
AJAAncillaryDataList::iterator AJAAncDataListIter
Handy non-const iterator for iterating over members of an AJAAncillaryDataList.
Declares the AJALock class.
virtual AJAStatus Compare(const AJAAncillaryData &inRHS, const bool inIgnoreLocation=true, const bool inIgnoreChecksum=true) const
Compares me with another packet.
static AJALock gAnalogTypeMapMutex
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
virtual AJAStatus SortListByDID(void)
Sort the AncillaryDataList by DataID (DID) value.
@ AJAAncDataChannel_Y
The ancillary data is associated with the luminance (Y) channel of the video stream.
static uint32_t GetExcludedZeroLengthPacketCount(void)
virtual const uint8_t * GetPayloadData(void) const
UWordSequence::const_iterator UWordSequenceConstIter
A handy const iterator for iterating over a UWordSequence.
std::vector< uint16_t > UWordSequence
An ordered sequence of UWord (uint16_t) values.
static const size_t MAX_RTP_PKT_LENGTH_BYTES(0x0000FFFF)
static AJAStatus SetAnalogAncillaryDataTypeForLine(const uint16_t inLineNum, const AJAAncDataType inType)
Sets (or changes) the map entry for the designated line to the designated type.
bool UnpackLine_10BitYUVtoU16s(std::vector< uint16_t > &outYCbCrLine, const NTV2Buffer &inFrameBuffer, const NTV2FormatDescriptor &inDescriptor, const UWord inLineOffset)
Unpacks up to one raster line of an NTV2_FBF_10BIT_YCBCR frame buffer into an array of uint16_t value...
virtual AJAStatus DeleteAncillaryData(AJAAncillaryData *pInAncData)
Removes all copies of the AJAAncillaryData object from me and deletes the object itself.
static bool SortBySID(AJAAncillaryData *lhs, AJAAncillaryData *rhs)
const uint8_t AJAAncillaryDataWildcard_DID
static bool IsIncludingZeroLengthPackets(void)
virtual AJAStatus AppendPayload(const AJAAncillaryData &inAncData)
Appends payload data from another AJAAncillaryData object to my existing payload.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
Declares numerous NTV2 utility functions.
std::vector< ULWordSequence > AJAU32Pkts
Ordered sequence of U32 RTP packets (U32s in network byte order)
#define AJA_SUCCESS(_status_)
@ AJAAncDataType_HDMI_Aux
HDMI Auxiliary data.
virtual AJAAncillaryData * GetAncillaryDataAtIndex(const uint32_t inIndex) const
Answers with the AJAAncillaryData object at the given index.
virtual std::string AsString(const uint16_t inDumpMaxBytes=0) const
virtual AJAAncillaryData & SetBufferFormat(const AJAAncBufferFormat inFmt)
Sets my originating buffer format.
static void BumpZeroLengthPacketCount(void)
@ AJAAncBufferFormat_SDI
SDI (AJA "GUMP")
virtual AJAStatus AddAncillaryData(const AJAAncillaryList &inPackets)
Appends a copy of the given list's packets to me.
#define LOGMYERROR(__x__)
struct AJAAncDataLoc AJAAncDataLoc
Defines where the ancillary data can be found within a video stream.
static bool StripNativeInserterGUMPPackets(const NTV2Buffer &inSrc, NTV2Buffer &outDst)
Copies GUMP from inSrc to outDst buffers, but removes ATC, VPID, VITC, EDH & raw/analog packets.
virtual std::ostream & Print(std::ostream &inOutStream, const bool inDetailed=true) const
Dumps a human-readable description of every packet in my list to the given output stream.
virtual bool AllowMultiRTPTransmit(void) const
Answers true if multiple RTP packets will be transmitted/encoded. The default behavior is to transmit...
virtual uint32_t CountAncillaryData(void) const
Answers with the number of AJAAncillaryData objects I contain (any/all types).
static AJAStatus WriteRTPPackets(NTV2Buffer &theBuffer, uint32_t &outBytesWritten, const AJAU32Pkts &inRTPPkts, const AJAAncPktCounts &inAncCounts, const bool inIsF2, const bool inIsProgressive)
Fills the buffer with the given RTP packets.
#define IS_VALID_AJAAncDataChannel(_x_)
static AJAAncDataType GetAnalogAncillaryDataTypeForLine(const uint16_t inLineNum)
Answers with the ancillary data type associated with the designated line.
virtual AJAStatus GetAncillaryDataTransmitSize(const bool inIsProgressive, const uint32_t inF2StartLine, uint32_t &outF1ByteCount, uint32_t &outF2ByteCount)
Answers with the sizes of the buffers (one for field 1, one for field 2) needed to hold the anc data ...
static bool Unpack8BitYCbCrToU16sVANCLineSD(const void *pInYUV8Line, UWordSequence &outU16YUVLine, const uint32_t inNumPixels)
SD version of Unpack8BitYCbCrToU16sVANCLine.
virtual ~AJAAncillaryList()
My destructor.
virtual std::string CompareWithInfo(const AJAAncillaryList &inCompareList, const bool inIgnoreLocation=true, const bool inIgnoreChecksum=true) const
Compares me with another list and returns a std::string that contains a human-readable explanation of...
AJAAncDataLoc & SetHorizontalOffset(uint16_t inHOffset)
Specifies the horizontal packet position in the raster.
AJAAncDataLink
Identifies which link of a video stream the ancillary data is associated with.
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
virtual AJAStatus GetVANCTransmitData(NTV2Buffer &inFrameBuffer, const NTV2FormatDescriptor &inFormatDesc)
Writes my AJAAncillaryData objects into the given tall/taller frame buffer having the given raster/fo...
void * GetHostPointer(void) const
std::string AsString(UWord inDumpMaxBytes=0) const
virtual uint32_t CountAncillaryDataWithID(const uint8_t inDID, const uint8_t inSID) const
Answers with the number of AncillaryData objects having the given DataID and SecondaryID.
virtual AJAAncillaryData & SetFrameID(const uint32_t inFrameID)
Sets my originating frame identifier.
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
static int32_t Increment(int32_t volatile *pTarget)
static bool gIncludeZeroLengthPackets(false)
ostream & operator<<(ostream &inOutStream, const AJAU32Pkts &inPkts)
static bool BufferHasGUMPData(const NTV2Buffer &inBuffer)
static const size_t MAX_RTP_PKT_LENGTH_WORDS((MAX_RTP_PKT_LENGTH_BYTES+1)/sizeof(uint32_t) - 1)
virtual void Clear(void)
Frees my allocated memory, if any, and resets my members to their default values.
static ostream & PrintULWordsBE(ostream &inOutStream, const ULWordSequence &inData, const size_t inMaxNum=32)
static AJAAncillaryAnalogTypeMap gAnalogTypeMap
@ AJAAncDataChannel_C
The ancillary data is associated with the chrominance (C) channel of the video stream.
#define NTV2_IS_SD_STANDARD(__s__)
virtual AJAStatus AddReceivedAncillaryData(const NTV2Buffer &inReceivedData, const uint32_t inFrameNum=0)
Parse "raw" ancillary data bytes received from hardware (ingest) – see SDI Anc Buffer Data Format – i...
#define HEX0N(__x__, __n__)
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
virtual AJAStatus ParsePayloadData(void)
Parses (interprets) the "local" ancillary data from my payload data.
static void ResetExcludedZeroLengthPacketCount(void)
Resets my tally of excluded zero-length packets to zero.
virtual AJAStatus GetTransmitData(NTV2Buffer &F1Buffer, NTV2Buffer &F2Buffer, const bool inIsProgressive=true, const uint32_t inF2StartLine=0)
Encodes my AJAAncillaryData packets into the given buffers in the default SDI Anc Buffer Data Format ...
const uint8_t AJAAncillaryDataWildcard_SID
std::string AJAStatusToString(const AJAStatus inStatus, const bool inDetailed)
virtual AJAStatus SortListByLocation(void)
Sort the AncillaryDataList by "location", i.e. where in the video (field, line num,...
std::vector< uint32_t > ULWordSequence
An ordered sequence of ULWord (uint32_t) values.
static AJAAncDataType GuessAncillaryDataType(const AJAAncillaryData &inAncData)
Given a generic AJAAncillaryData object, attempts to guess what kind of specific AJAAncillaryData obj...
Declares the AJAAtomic class.
virtual AJAStatus GenerateTransmitData(uint8_t *pBuffer, const size_t inMaxBytes, uint32_t &outPacketSize)
Generates "raw" ancillary data from my internal ancillary data (playback) – see SDI Anc Buffer Data F...
static bool SortByLocation(AJAAncillaryData *lhs, AJAAncillaryData *rhs)
virtual AJAAncillaryList & operator=(const AJAAncillaryList &inRHS)
Assignment operator – replaces my contents with the right-hand-side value.
virtual AJAAncillaryData * Clone(void) const
@ AJAAncDataCoding_Digital
The ancillary data is in the form of a SMPTE-291 Ancillary Packet.
static uint32_t gExcludedZeroLengthPackets(0)
virtual AJAAncDataType GetAnalogAncillaryDataType(const AJAAncillaryData &inAncData)
virtual std::string CompareWithInfo(const AJAAncillaryData &inRHS, const bool inIgnoreLocation=true, const bool inIgnoreChecksum=true) const
Compares me with another packet and returns a string that describes what's different.
AJAAncillaryList()
Instantiate and initialize with a default set of values.
static AJAStatus AddFromDeviceAuxBuffer(const NTV2Buffer &inAuxBuffer, AJAAncillaryList &outPacketList, const uint32_t inFrameNum=0)
Appends whatever can be decoded from the given device HDIM aux buffer to the AJAAncillaryList.
@ AJAAncBufferFormat_RTP
RTP/IP.
bool Fill(const T &inValue)
Fills me with the given scalar value.
@ AJAAncDataSpace_VANC
Ancillary data found between SAV and EAV (.
virtual AJAStatus Clear(void)
Removes and frees all of my AJAAncillaryData objects.
virtual AJAStatus GetIPTransmitDataLength(uint32_t &outF1ByteCount, uint32_t &outF2ByteCount, const bool inIsProgressive=true, const uint32_t inF2StartLine=0)
Answers with the number of bytes required to store IP/RTP for my AJAAncillaryData packets in RTP Anc ...
@ AJA_STATUS_BADBUFFERCOUNT
virtual bool IgnoreChecksumErrors(void) const
Answers if checksum errors are to be ignored or not. The default behavior is to not ignore them.
#define xHEX0N(__x__, __n__)
#define AJA_FAILURE(_status_)
@ AJAAncDataType_Cea608_Line21
CEA608 SD Closed Captioning ("Line 21" waveform)
static AJAStatus AddFromDeviceAncBuffer(const NTV2Buffer &inAncBuffer, AJAAncillaryList &outPacketList, const uint32_t inFrameNum=0)
Appends whatever can be decoded from the given device Anc buffer to the AJAAncillaryList.
#define IS_VALID_AJAAncDataType(_x_)
virtual AJAStatus GetRTPPackets(AJAU32Pkts &outF1U32Pkts, AJAU32Pkts &outF2U32Pkts, AJAAncPktCounts &outF1AncCounts, AJAAncPktCounts &outF2AncCounts, const bool inIsProgressive, const uint32_t inF2StartLine)
Answers with my F1 & F2 SMPTE anc packets encoded as RTP ULWordSequences. The returned ULWords are al...
AJAAncPktDIDSIDSet::const_iterator AJAAncPktDIDSIDSetConstIter
Handy const iterator for AJAAncPktDIDSIDSet (New in SDK 16.0)
static const uint32_t MAX_ANC_PKTS_PER_RTP_PKT(0x000000FF)
@ AJAAncDataLink_A
The ancillary data is associated with Link A of the video stream.
@ AJAAncDataChannel_Both
SD ONLY – The ancillary data is associated with both the chroma and luma channels.
std::vector< U16Packet > U16Packets
An ordered sequence of zero or more U16Packet values.
Declares the AJADebug class.
@ AncChannelSearch_Both
Look both luma and chroma samples (SD only)
bool Set(const void *pInUserPointer, const size_t inByteCount)
Sets (or resets) me from a client-supplied address and size.
static AJAStatus SetFromVANCData(const NTV2Buffer &inFrameBuffer, const NTV2FormatDescriptor &inFormatDesc, AJAAncillaryList &outPackets, const uint32_t inFrameNum=0)
Returns all packets found in the VANC lines of the given NTV2 frame buffer.
#define DECN(__x__, __n__)
AJAAncDataSpace GetDataSpace(void) const
@ AncChannelSearch_Y
Only look in luma samples.
virtual AJAStatus AddReceivedAuxiliaryData(const NTV2Buffer &inReceivedData, const uint32_t inFrameNum=0)
Parse "raw" HDMI auxillary data bytes received from hardware (ingest) into separate AJAAncillaryData ...