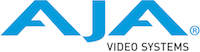 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
13 #if defined (INCLUDE_AJACC)
19 #define NTV2_NUM_IMAGES (10)
22 #define FGFAIL(_expr_) AJA_sERROR (AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
23 #define FGWARN(_expr_) AJA_sWARNING(AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
24 #define FGDBG(_expr_) AJA_sDEBUG (AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
25 #define FGNOTE(_expr_) AJA_sNOTICE (AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
26 #define FGINFO(_expr_) AJA_sINFO (AJA_DebugUnit_Application, AJAFUNC << ": " << _expr_)
35 mbFixedReference (
false),
44 mFormatIsProgressive (
true),
47 mDoMultiChannel (
false)
66 if (inInputSource != mInputSource)
70 mInputSource = inInputSource;
80 if (inDeviceIndex != mBoardNumber)
83 mBoardNumber = inDeviceIndex;
93 const UWord result (mBoardNumber);
103 ULWord framesCaptured (0);
107 if (mNTV2Card.
Open(mBoardNumber))
132 currentImage.load (
":/resources/splash.png");
137 bool waitForInput =
false;
139 for (
UWord offset = 0; offset < numInputs; offset++)
160 if (mNTV2Card.IsOpen ())
163 if (!mDoMultiChannel)
173 if (mNTV2Card.
Open(mBoardNumber))
178 qDebug() <<
"Could not acquire board number " <<
GetDeviceIndex();
194 qDebug (
"Device not ready");
206 qDebug() <<
"## WARNING: Cannot acquire stream channel " << (int)(mStream);
218 buffers[i].
Allocate (mFrameDimensions.Width () * mFrameDimensions.Height () * 4,
true);
225 qDebug() <<
"## WARNING: Cannot DMA lock input buffer";
236 qDebug() <<
"## WARNING: Cannot add buffer to stream: " << bfrStatus.
mStatus;
244 qDebug() <<
"## WARNING: Stream start failed: " << bfrStatus.
mStatus;
257 qDebug() <<
"## WARNING: Open failed for device " <<
GetDeviceIndex ();
267 QImage currentImage (mFrameDimensions.Width (),
268 mFrameDimensions.Height (),
269 QImage::Format_RGB32);
270 currentImage.fill (qRgba (40, 40, 40, 255));
279 if (mNTV2Card.IsOpen())
293 outString.append (
" ");
298 QImage img = QImage((uchar*)(buffers[index].GetHostAddress (0)),
299 mFrameDimensions.Width (),
300 mFrameDimensions.Height (),
301 QImage::Format_RGB32);
312 qDebug() <<
"## WARNING: Cannot add buffer to stream";
323 qDebug() <<
"## WARNING: stream wait failed";
330 if (mNTV2Card.IsOpen ())
334 if (!mDoMultiChannel)
356 bool validInput (
false);
372 switch (mCurrentVideoFormat)
386 mNTV2Card.
SetVideoFormat (mCurrentVideoFormat,
false,
false, mChannel);
392 qDebug() <<
"## DEBUG: mInputSource=" << mChannel <<
", mCurrentVideoFormat=" << vfString <<
", width=" << mFrameDimensions.Width() <<
", height=" << mFrameDimensions.Height();
395 if (!mbFixedReference)
417 if (!mbFixedReference)
449 if (mNTV2Card.IsOpen ())
478 mCurrentVideoFormat = videoFormat;
481 else if ((mCurrentVideoFormat != videoFormat) ||
482 (mCurrentColorSpace != colorSpace))
484 if (mDebounceCounter == 0)
487 mLastVideoFormat = videoFormat;
490 else if (mDebounceCounter == 6)
494 mCurrentVideoFormat = videoFormat;
495 mDebounceCounter = 0;
499 if (mLastVideoFormat == videoFormat)
502 mDebounceCounter = 0;
519 switch (mInputSource)
void newStatusString(const QString &inStatus)
This is signaled (called) when my status string changes.
virtual bool SetSDIInLevelBtoLevelAConversion(const NTV2ChannelSet &inSDIInputs, const bool inEnable)
Enables or disables 3G level B to 3G level A conversion at the SDI input(s).
virtual ULWord StreamBufferQueue(const NTV2Channel inChannel, NTV2Buffer &inBuffer, ULWord64 bufferCookie, NTV2StreamBuffer &status)
Queue a buffer to the stream. The bufferCookie is a user defined identifier of the buffer used by the...
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
Header file for the NTV2StreamGrabber class.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
@ NTV2_FBF_ARGB
See 8-Bit ARGB, RGBA, ABGR Formats.
NTV2OutputXptID GetSDIInputOutputXptFromChannel(const NTV2Channel inSDIInput, const bool inIsDS2=false)
ULWord64 mBufferCookie
Buffer User cookie.
@ NTV2_CHANNEL2
Specifies channel or Frame Store 2 (or the 2nd item).
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
Declares the AJAAncillaryData_Cea608_line21 class.
Declares device capability functions.
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
ULWord GetByteCount(void) const
#define NTV2_ASSERT(_expr_)
bool Deallocate(void)
Deallocates my user-space storage (if I own it – i.e. from a prior call to Allocate).
Declares the AJAAncillaryList class.
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
NTV2InputXptID GetFrameBufferInputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsBInput=false)
bool SetupInput(void)
Configures my AJA device for capture.
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
UWord NTV2DeviceGetNumCSCs(const NTV2DeviceID inDeviceID)
virtual ULWord StreamChannelRelease(const NTV2Channel inChannel)
Release a stream. Releases all buffers remaining in the queue.
@ NTV2_LHIHDMIColorSpaceRGB
virtual bool GetVANCMode(NTV2VANCMode &outVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Retrieves the current VANC mode for the given FrameStore.
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
virtual bool GetSDITransmitEnable(const NTV2Channel inChannel, bool &outEnabled)
Answers whether or not the specified SDI connector is currently acting as a transmitter (i....
virtual void run(void)
My thread function.
NTV2FrameDimensions & Set(const ULWord inWidth, const ULWord inHeight)
Sets my dimension values.
bool IsProgressivePicture(const NTV2VideoFormat format)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=(0), bool inRDMA=(0))
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
virtual bool DMABufferUnlockAll()
Unlocks all previously-locked buffers used for DMA transfers.
virtual std::string GetDisplayName(void)
Answers with this device's display name.
NTV2Crosspoint NTV2InputSourceToChannelSpec(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Crosspoint value.
NTV2LHIHDMIColorSpace GetColorSpaceFromInputSource(void)
std::string NTV2InputSourceToString(const NTV2InputSource inValue, const bool inForRetailDisplay=false)
@ NTV2_FORMAT_1080p_5994_B
Declares the CNTV2DeviceScanner class.
virtual ULWord StreamChannelStart(const NTV2Channel inChannel, NTV2StreamChannel &status)
Start a stream. Put the stream is the active state to start processing queued buffers....
bool NTV2DeviceHasBiDirectionalSDI(const NTV2DeviceID inDeviceID)
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=(!(0)))
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
UWord NTV2DeviceGetNumVideoInputs(const NTV2DeviceID inDeviceID)
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
@ NTV2_REFERENCE_FREERUN
Specifies the device's internal clock.
Declares numerous NTV2 utility functions.
@ NTV2_FORMAT_1080p_5000_B
void newFrame(const QImage &inImage, const bool inClear)
This is signaled (called) when a new frame has been captured and is available for display.
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
#define NTV2_INPUT_SOURCE_IS_HDMI(_inpSrc_)
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
virtual ULWord StreamBufferRelease(const NTV2Channel inChannel, NTV2StreamBuffer &status)
Remove the oldest buffer released by the streaming engine from the buffer queue.
virtual bool IsDeviceReady(const bool inCheckValid=(0))
UWord GetDeviceIndex(void) const
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
@ NTV2_FORMAT_1080p_6000_A
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
NTV2VANCMode
These enum values identify the available VANC modes.
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
NTV2InputSource
Identifies a specific video input source.
void SetInputSource(const NTV2InputSource inInputSource)
Sets the input to be used for capture on the AJA device being used.
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=(0))
Connects the given widget signal input (sink) to the given widget signal output (source).
@ NTV2_LHIHDMIColorSpaceYCbCr
virtual ULWord StreamChannelWait(const NTV2Channel inChannel, NTV2StreamChannel &status)
Wait for any stream event. Returns for any state or buffer change.
This file contains some structures, constants, classes and functions that are used in some of the dem...
virtual ~NTV2StreamGrabber()
My destructor.
NTV2StreamGrabber(QObject *pInParentObject=NULL)
Constructs me.
@ NTV2_MODE_CAPTURE
Capture (input) mode, which writes into device SDRAM.
NTV2VideoFormat GetVideoFormatFromInputSource(void)
@ NTV2_FORMAT_1080p_5000_A
virtual bool AcquireStreamForApplicationWithReference(ULWord inApplicationType, int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::AcquireStreamForApplication useful for process g...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
virtual bool GetHDMIInputColor(NTV2LHIHDMIColorSpace &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current colorspace for the given HDMI input.
@ NTV2_FORMAT_1080p_6000_B
#define STREAMPREVIEW_WIDGET_Y
#define NTV2_STREAM_STATUS_SUCCESS
Used in NTV2Stream success.
virtual NTV2DeviceID GetDeviceID(void)
virtual ULWord StreamChannelInitialize(const NTV2Channel inChannel)
Initialize a stream. Put the stream queue and hardware in a known good state ready for use....
@ NTV2_FORMAT_1080p_5994_A
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
NTV2OutputXptID GetInputSourceOutputXpt(const NTV2InputSource inInputSource, const bool inIsSDI_DS2=false, const bool inIsHDMI_RGB=false, const UWord inHDMI_Quadrant=0)
#define NTV2_INPUT_SOURCE_IS_SDI(_inpSrc_)
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
bool IsInput3Gb(const NTV2InputSource inputSource)
void StopStream(void)
Stops capturing.
virtual bool ReleaseStreamForApplicationWithReference(ULWord inApplicationType, int32_t inProcessID)
A reference-counted version of CNTV2DriverInterface::ReleaseStreamForApplication useful for process g...
bool CheckForValidInput(void)
ULWord mStatus
Action status.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
virtual bool Open(const UWord inDeviceIndex)
Opens a local/physical AJA device so it can be monitored/controlled.
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
virtual bool GetSDIInput3GbPresent(bool &outValue, const NTV2Channel channel)
#define NTV2_IS_VALID_VIDEO_FORMAT(__f__)
virtual bool Close(void)
Closes me, releasing host resources that may have been allocated in a previous Open call.
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
void SetDeviceIndex(const UWord inDeviceIndex)
Sets the AJA device to be used for capture.
#define STREAMPREVIEW_WIDGET_X