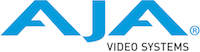 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
virtual ULWord StreamBufferQueue(const NTV2Channel inChannel, NTV2Buffer &inBuffer, ULWord64 bufferCookie, NTV2StreamBuffer &status)
Queue a buffer to the stream. The bufferCookie is a user defined identifier of the buffer used by the...
#define NTV2_STREAM_STATUS_FAIL
Used in NTV2Stream fail.
virtual bool StreamBufferOps(const NTV2Channel inChannel, NTV2Buffer &inBuffer, ULWord64 bufferCookie, ULWord flags, NTV2StreamBuffer &status)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
virtual bool StreamChannelOps(const NTV2Channel inChannel, ULWord flags, NTV2StreamChannel &status)
virtual ULWord StreamChannelFlush(const NTV2Channel inChannel, NTV2StreamChannel &status)
Flush a stream. Remove all the buffers from the stream except a currently active idle buffer....
#define NTV2_STREAM_CHANNEL_RELEASE
Used in NTV2StreamChannel to release stream.
virtual ULWord StreamChannelRelease(const NTV2Channel inChannel)
Release a stream. Releases all buffers remaining in the queue.
#define NTV2_STREAM_CHANNEL_START
Used in NTV2StreamChannel to start streaming.
ULWord mStatus
Action status.
#define NTV2_STREAM_CHANNEL_INITIALIZE
Used in NTV2StreamChannel to initialize the stream.
virtual ULWord StreamChannelStart(const NTV2Channel inChannel, NTV2StreamChannel &status)
Start a stream. Put the stream is the active state to start processing queued buffers....
virtual ULWord StreamChannelStatus(const NTV2Channel inChannel, NTV2StreamChannel &status)
Get the current stream status.
Declares the CNTV2Card class.
#define NTV2_STREAM_BUFFER_STATUS
Used in NTV2StreamBuffer to request buffer status.
#define NTV2_STREAM_CHANNEL_WAIT
Used in NTV2StreamChannel to wait for signal.
virtual ULWord StreamBufferStatus(const NTV2Channel inChannel, ULWord64 bufferCookie, NTV2StreamBuffer &status)
Get the status of the buffer identified by the bufferCookie.
#define NTV2_STREAM_STATUS_MESSAGE
Used in NTV2Stream driver message failure.
#define NTV2_STREAM_CHANNEL_FLUSH
Used in NTV2StreamChannel to flush buffer queue.
virtual ULWord StreamBufferRelease(const NTV2Channel inChannel, NTV2StreamBuffer &status)
Remove the oldest buffer released by the streaming engine from the buffer queue.
#define NTV2_STREAM_BUFFER_QUEUE
Used in NTV2StreamBuffer to add buffer to queue.
#define NTV2_STREAM_BUFFER_RELEASE
Used in NTV2StreamBuffer to signal on complete.
virtual ULWord StreamChannelStop(const NTV2Channel inChannel, NTV2StreamChannel &status)
Stop a stream. Put the stream is the idle state. For frame based video, the stream will idle on the b...
virtual ULWord StreamChannelWait(const NTV2Channel inChannel, NTV2StreamChannel &status)
Wait for any stream event. Returns for any state or buffer change.
virtual ULWord StreamChannelInitialize(const NTV2Channel inChannel)
Initialize a stream. Put the stream queue and hardware in a known good state ready for use....
Declares enums and structs used by all platform drivers and the SDK.
#define NTV2_STREAM_CHANNEL_STATUS
Used in NTV2StreamChannel to request stream status.
ULWord mStatus
Action status.
Declares the CNTV2DriverInterface base class.
#define NTV2_STREAM_CHANNEL_STOP
Used in NTV2StreamChannel to stop streaming.