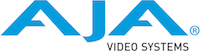 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
30 int main (
int argc,
const char ** argv)
35 int doMultiFormat (0);
45 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
47 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
48 {
"input",
'i',
POPT_ARG_STRING, &pInputSrcSpec, 0,
"SDI input to use",
"1-8, ?=list" },
56 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
58 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
61 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
70 if (inputSourceStr ==
"?" || inputSourceStr ==
"list")
71 {cout << legalSources << endl;
return 0;}
72 if (!inputSourceStr.empty())
76 {cerr <<
"## ERROR: Input source '" << inputSourceStr <<
"' not one of:" << endl << legalSources << endl;
return 1;}
80 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
82 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
86 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
104 const string hdg1 (
" Capture Playout Capture Playout"),
105 hdg2a(
" Fields Fields Fields Buffer Buffer"),
106 hdg2b(
" Frames Frames Frames Buffer Buffer"),
107 hdg3 (
"Processed Dropped Dropped Level Level"),
108 hdg2 (noFieldMode ? hdg2b : hdg2a);
113 {cerr <<
"## ERROR: Initialization failed, status=" << status << endl;
return 4;}
119 cout << hdg1 << endl << hdg2 << endl << hdg3 << endl;
122 ULWord totalFrames(0), inputDrops(0), outputDrops(0), inputBufferLevel(0), outputBufferLevel(0);
123 burner.
GetStatus (totalFrames, inputDrops, outputDrops, inputBufferLevel, outputBufferLevel);
124 cout << setw(9) << totalFrames << setw(9) << inputDrops << setw(9) << outputDrops
125 << setw(9) << inputBufferLevel << setw(9) << outputBufferLevel <<
"\r" << flush;
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
static NTV2InputSource GetInputSourceFromString(const std::string &inStr)
Returns the NTV2InputSource that matches the given string.
bool fIsFieldMode
True if Field Mode, otherwise Frame Mode.
virtual void GetStatus(ULWord &outNumProcessed, ULWord &outCaptureDrops, ULWord &outPlayoutDrops, ULWord &outCaptureLevel, ULWord &outPlayoutLevel)
Provides status information about my input (capture) and output (playout) processes.
void SignalHandler(int inSignal)
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
bool fSuppressAudio
If true, suppress audio; otherwise include audio.
NTV2TCIndex fTimecodeSource
Timecode source to use.
int main(int argc, const char **argv)
NTV2PixelFormat fPixelFormat
The pixel format to use.
static bool IsValidDevice(const std::string &inDeviceSpec)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
static NTV2FrameBufferFormat GetPixelFormatFromString(const std::string &inStr)
Returns the NTV2FrameBufferFormat that matches the given string.
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC=false, const bool inIsF2=false)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
I capture individual fields from an interlaced video signal provided to an SDI input....
static bool gGlobalQuit(false)
Set this "true" to exit gracefully.
static AJAStatus Open(bool incrementRefCount=false)
NTV2InputSource fInputSource
The device input connector to use.
static std::string GetInputSourceStrings(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDeviceSpecifier=std::string())
Header file for the NTV2FieldBurn demonstration class.
@ NTV2_IOKINDS_ALL
Specifies any/all input/output kinds.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDeviceSpecifier=std::string())
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
Configures an NTV2Burn or NTV2FieldBurn instance.
virtual AJAStatus Run(void)
Runs me.