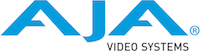 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
28 int main (
int argc,
const char ** argv)
35 int channelNumber (1);
36 int doMultiFormat (0);
48 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
49 {
"channel",
'c',
POPT_ARG_INT, &channelNumber, 0,
"channel to use",
"1-8" },
51 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
52 {
"frames", 0,
POPT_ARG_STRING, &pFramesSpec, 0,
"frames to AutoCirculate",
"num[@min] or min-max" },
53 {
"videoFormat",
'v',
POPT_ARG_STRING, &pVideoFormat, 0,
"video format to produce",
"'?' or 'list' to list" },
54 {
"hdrType",
't',
POPT_ARG_INT, &hdrType, 0,
"HDR pkt to send",
"0=none 1=SDR 2=HDR10 3=HLG"},
55 {
"anc",
'a',
POPT_ARG_STRING, &pAncFilePath, 0,
"play prerecorded anc",
"path/to/binary/data/file" },
64 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
66 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
69 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
76 if ((channelNumber < 1) || (channelNumber > 8))
77 {cerr <<
"## ERROR: Invalid channel number " << channelNumber <<
" -- expected 1 thru 8" << endl;
return 1;}
81 const string videoFormatStr (pVideoFormat ? pVideoFormat :
"");
84 if (videoFormatStr ==
"?" || videoFormatStr ==
"list")
87 { cerr <<
"## ERROR: Invalid '--videoFormat' value '" << videoFormatStr <<
"' -- expected values:" << endl
93 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
95 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
99 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
105 static const string legalFramesSpec (
"{frameCount}[@{firstFrameNum}] or {firstFrameNum}-{lastFrameNum}");
106 const string framesSpec (pFramesSpec ? pFramesSpec :
"");
107 if (!framesSpec.empty())
110 if (!parseResult.empty())
111 {cerr <<
"## ERROR: Bad 'frames' spec '" << framesSpec <<
"'\n## " << parseResult << endl;
return 1;}
114 {cerr <<
"## ERROR: Bad 'frames' spec '" << framesSpec <<
"'\n## Expected " << legalFramesSpec << endl;
return 1;}
116 if (noVideo && noAudio)
117 {cerr <<
"## ERROR: conflicting options '--novideo' and '--noaudio'" << endl;
return 1;}
120 string ancFilePath (pAncFilePath ? pAncFilePath :
"");
127 {cerr <<
"## ERROR: conflicting options '--hdrType' and '--anc'" << endl;
return 2;}
140 {cout <<
"## ERROR: Initialization failed: " <<
::AJAStatusToString(status) << endl;
return 1;}
151 cout <<
" Frames Frames Buffer" << endl
152 <<
" Played Dropped Level" << endl;
static std::string GetVideoFormatStrings(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_NON_4KUHD, const std::string inDeviceSpecifier=std::string())
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
std::string & strip(std::string &str, const std::string &ws)
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
void SignalHandler(int inSignal)
ULWord GetQueueDepth(void)
Gets the queue depth.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
int main(int argc, const char **argv)
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
I play out SD or HD test pattern (with timecode) to an output of an AJA device with or without audio ...
std::string setFromString(const std::string &inStr)
static NTV2VideoFormat GetVideoFormatFromString(const std::string &inStr, const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_NON_4KUHD)
Returns the NTV2VideoFormat that matches the given string.
AJAAncDataType fTransmitHDRType
Specifies the HDR anc data packet to transmit, if any.
static bool IsValidDevice(const std::string &inDeviceSpec)
NTV2OutputDestination NTV2ChannelToOutputDestination(const NTV2Channel inChannel)
Converts the given NTV2Channel value into its ordinary equivalent NTV2OutputDestination.
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
bool fSuppressAudio
If true, suppress audio; otherwise generate audio tone.
static NTV2FrameBufferFormat GetPixelFormatFromString(const std::string &inStr)
Returns the NTV2FrameBufferFormat that matches the given string.
bool fSuppressVideo
If true, suppress video; otherwise generate test patterns.
NTV2VideoFormat fVideoFormat
The video format to use.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
NTV2Channel fOutputChannel
The device channel to use.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
std::string fAncDataFilePath
Optional path to Anc binary data file to playout.
static AJAStatus Open(bool incrementRefCount=false)
virtual AJAStatus Run(void)
Runs me.
bool fTransmitLTC
If true, embed LTC; otherwise embed VITC.
@ VIDEO_FORMATS_NON_4KUHD
NTV2OutputDest fOutputDest
The desired output connector to use.
static bool gGlobalQuit(false)
std::string AJAStatusToString(const AJAStatus inStatus, const bool inDetailed)
ULWord64 mActiveCount
Number of buffers active.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDeviceSpecifier=std::string())
Configures an NTV2Player instance.
NTV2PixelFormat fPixelFormat
The pixel format to use.
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
ULWord64 mRepeatCount
Number of buffer repeats.
virtual void GetStreamStatus(NTV2StreamChannel &outStatus)
Provides status information about my output (playout) process.
@ AJAAncDataType_HDR_HDR10