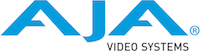 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
21 #define NTV2_AUDIOSIZE_MAX (401 * 1024)
22 #define NTV2_ANCILLARYSIZE_MAX (256 * 1024)
59 while (mConsumerThread.
Active())
62 while (mProducerThread.
Active())
97 cerr <<
"## ERROR: Unable to acquire device because another app (pid " << appPID <<
") owns it" << endl;
134 if (mDevice.IsRemote())
135 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl;
137 #endif // defined(_DEBUG)
156 cerr <<
"## ERROR: No input signal or unknown format" << endl;
167 <<
" instead" << endl;
203 ULWord F1AncSize(0), F2AncSize(0);
227 if (!mProgressive && F2AncSize)
229 mAVCircularBuffer.
Add(&frameData);
231 #ifdef NTV2_BUFFER_LOCK
256 const bool canVerify (mDevice.HasCanConnectROM());
271 if (isInputRGB && !isFrameRGB)
288 else if (!isInputRGB && isFrameRGB)
305 else if (isInputRGB && isFrameRGB)
332 if (isInputRGB && !isFrameRGB)
334 mDevice.
Connect (frameBufferInputXpt, cscWidgetYUVOutputXpt, canVerify);
335 mDevice.
Connect (cscWidgetVideoInputXpt, inputWidgetOutputXpt, canVerify);
337 else if (!isInputRGB && isFrameRGB)
339 mDevice.
Connect (frameBufferInputXpt, cscWidgetRGBOutputXpt, canVerify);
340 mDevice.
Connect (cscWidgetVideoInputXpt, inputWidgetOutputXpt, canVerify);
343 mDevice.
Connect (frameBufferInputXpt, inputWidgetOutputXpt, canVerify);
367 mConsumerThread.
Start();
387 uint64_t ancTally(0);
388 uint64_t audioTally(0);
389 uint64_t dolbyTally(0);
405 if (pData[i] == 0xff)
409 printf(
"f1 size reg %d ffs %d samples %d\n", pFrameData->
NumCapturedAncBytes(), i, audioSize/4);
419 if (pData[i] == 0xff)
423 printf(
"f2 size reg %d ffs %d samples %d\n", pFrameData->
NumCapturedAnc2Bytes(), i, audioSize/4);
431 if (!mProgressive && pFrameData->
AncBuffer2())
441 pAFS->write(pFrameData->
AudioBuffer(), streamsize(audioSize));
443 if (!mProgressive && pFrameData->
AncBuffer2())
446 pAFS->write(pFrameData->
AudioBuffer(), streamsize(audioSize));
454 pDFS->write(pFrameData->
AncBuffer(), streamsize(dolbySize));
456 if (!mProgressive && pFrameData->
AncBuffer2())
460 pDFS->write(pFrameData->
AncBuffer2(), streamsize(dolbySize));
469 {
delete pOFS; cerr <<
"Wrote " <<
DEC(ancTally) <<
" frames of raw anc data" << endl; }
471 {
delete pAFS; cerr <<
"Wrote " <<
DEC(audioTally) <<
" frames of raw audio data" << endl; }
473 {
delete pDFS; cerr <<
"Wrote " <<
DEC(dolbyTally) <<
" frames of dolby data" << endl; }
486 mProducerThread.
Start();
582 CAPNOTE(
"Thread completed, will exit");
599 uint32_t audioSize = 0;
603 for (uint32_t i = 0; i < ancSize/32; i++)
606 if (ancData[0] == 0x02)
609 if ((ancData[1] & 0x01) != 0)
612 if ((ancData[2] & 0x01) != 0)
621 *audioData++ = ancData[4];
622 *audioData++ = ancData[5];
623 *audioData++ = ancData[7];
624 *audioData++ = ancData[8];
629 if ((ancData[1] & 0x10) == 0)
632 if ((ancData[1] & 0x02) != 0)
635 if ((ancData[2] & 0x02) != 0)
644 *audioData++ = ancData[11];
645 *audioData++ = ancData[12];
646 *audioData++ = ancData[14];
647 *audioData++ = ancData[15];
652 if ((ancData[1] & 0x04) != 0)
655 if ((ancData[2] & 0x04) != 0)
664 *audioData++ = ancData[18];
665 *audioData++ = ancData[19];
666 *audioData++ = ancData[21];
667 *audioData++ = ancData[22];
672 if ((ancData[1] & 0x08) != 0)
675 if ((ancData[2] & 0x08) != 0)
684 *audioData++ = ancData[25];
685 *audioData++ = ancData[26];
686 *audioData++ = ancData[28];
687 *audioData++ = ancData[29];
702 uint32_t dolbySize = 0;
706 for (uint32_t i = 0; i < audioSize / 2; i++)
712 mDolbyState = (*audioData == 0xf872)? 1 : 0;
715 mDolbyState = (*audioData == 0x4e1f)? 2 : 0;
719 mDolbyState = ((*audioData & 0xff) == 0x15)? 3 : 0;
723 mDolbyLength = (uint32_t)*audioData / 2;
728 if (mDolbyLength > 0)
733 *dolbyData = (*audioData >> 8) & 0xff;
734 *dolbyData |= (*audioData & 0xff) << 8;
752 return dolbySize * 2;
ULWord NumCapturedAnc2Bytes(void) const
bool NTV2DeviceCanDoCapture(const NTV2DeviceID inDeviceID)
NTV2ReferenceSource NTV2InputSourceToReferenceSource(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2ReferenceSource value.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the capture thread's static callback function that gets called when the capture thread runs....
NTV2InputSource NTV2ChannelToInputSource(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
Declares the NTV2DolbyCapture class.
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
ULWord acFramesProcessed
Total number of frames successfully processed since CNTV2Card::AutoCirculateStart called.
UWord NTV2DeviceGetNumHDMIVideoInputs(const NTV2DeviceID inDeviceID)
bool fDoAudioFilter
If true, capture only audio anc.
@ AJA_ThreadPriority_High
UWord firstFrame(void) const
#define NTV2_ANCILLARYSIZE_MAX
Declares device capability functions.
std::string fDeviceSpec
The AJA device to use.
std::string fDolbyDataFilePath
Optional path to Dolby binary data file.
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
#define NTV2_VIDEO_FORMAT_HAS_PROGRESSIVE_PICTURE(__f__)
ULWord fNumAncBytes
Actual number of captured F1 anc bytes.
A generic user-space buffer object that has an address and a length. Used most often to share an arbi...
virtual bool GetStandard(NTV2Standard &outValue, NTV2Channel inChannel=NTV2_CHANNEL1)
virtual void GetACStatus(ULWord &outGoodFrames, ULWord &outDroppedFrames, ULWord &outBufferLevel)
Provides status information about my input (capture) process.
ULWord GetByteCount(void) const
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool EnableInputInterrupt(const NTV2Channel channel=NTV2_CHANNEL1)
Allows the CNTV2Card instance to wait for and respond to input vertical blanking interrupts originati...
NTV2Buffer & AncBuffer2(void)
Declares the AJATime class.
bool fDoFrameData
if true, output per frame statistics
virtual void CaptureFrames(void)
Repeatedly captures frames using AutoCirculate (until global quit flag set).
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
std::ostream & operator<<(std::ostream &ioStrm, const DolbyConfig &inObj)
NTV2Channel fInputChannel
The device channel to use.
This class is used to configure an NTV2Capture instance.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
virtual ~NTV2DolbyCapture()
virtual bool AncSetFrameBufferSize(const ULWord inF1Size, const ULWord inF2Size)
Sets the capacity of the ANC buffers in device frame memory. (Call NTV2DeviceCanDoCustomAnc to determ...
bool NTV2DeviceCanDoMultiFormat(const NTV2DeviceID inDeviceID)
NTV2InputXptID GetFrameBufferInputXptFromChannel(const NTV2Channel inFrameStore, const bool inIsBInput=false)
NTV2InputSource fInputSource
The device input connector to use.
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
#define NTV2_AUDIOSIZE_MAX
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
@ NTV2_IOKINDS_HDMI
Specifies HDMI input/output kinds.
bool fDoMultiFormat
If true, use multi-format/multi-channel mode, if device supports it; otherwise normal mode.
NTV2Buffer fAncBuffer2
Additional "F2" host anc buffer.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
@ NTV2_LHIHDMIColorSpaceRGB
virtual bool GetVANCMode(NTV2VANCMode &outVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Retrieves the current VANC mode for the given FrameStore.
bool SetAncBuffers(ULWord *pInANCBuffer, const ULWord inANCByteCount, ULWord *pInANCF2Buffer=NULL, const ULWord inANCF2ByteCount=0)
Sets my ancillary data buffers for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2Buffer fAncBuffer
Host ancillary data buffer.
bool NTV2DeviceCanDoFrameBufferFormat(const NTV2DeviceID inDeviceID, const NTV2FrameBufferFormat inFBFormat)
@ NTV2_CHANNEL1
Specifies channel or Frame Store 1 (or the first item).
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=true, const bool inKeepVancSettings=false, const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
virtual void RouteInputSignal(void)
Sets up device routing for capture.
NTV2Buffer & VideoBuffer(void)
#define NTV2_IS_4K_VIDEO_FORMAT(__f__)
#define AUTOCIRCULATE_WITH_ANC
Use this to AutoCirculate with ancillary data.
ULWord NumCapturedAncBytes(void) const
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
std::string NTV2InputSourceToString(const NTV2InputSource inValue, const bool inForRetailDisplay=false)
Declares the AJAProcess class.
@ NTV2_XptFrameBuffer1Input
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
NTV2Standard
Identifies a particular video standard.
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread runs...
NTV2DolbyCapture(const DolbyConfig &inConfig)
Constructs me using the given settings.
static AJALabelValuePairs & append(AJALabelValuePairs &inOutTable, const std::string &inLabel, const std::string &inValue=std::string())
A convenience function that appends the given label and value strings to the provided AJALabelValuePa...
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
ULWord VideoBufferSize(void) const
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=false)
Sets the device's clock reference source. See Device Clocking and Synchronization for more informatio...
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
NTV2OutputXptID GetCSCOutputXptFromChannel(const NTV2Channel inCSC, const bool inIsKey=false, const bool inIsRGB=false)
virtual void ToString(std::string &outAllLabelsAndValues) const
Answers with a multi-line string that contains the complete host system info table.
ULWord AncBufferSize(void) const
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=false)
Connects the given widget signal input (sink) to the given widget signal output (source).
ULWord GetCapturedAncByteCount(const bool inField2=false) const
virtual void StartConsumerThread(void)
Starts my frame consumer thread.
virtual uint32_t RecoverDolby(NTV2Buffer &audio, uint32_t audioSize, NTV2Buffer &dolby)
Recover dolby from audio data.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
ULWord AncBuffer2Size(void) const
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
Declares numerous NTV2 utility functions.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
#define NTV2_INPUT_SOURCE_IS_HDMI(_inpSrc_)
NTV2InputCrosspointID
Identifies a widget input that potentially can accept a signal emitted from another widget's output (...
NTV2Buffer & AudioBuffer(void)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
NTV2InputXptID GetCSCInputXptFromChannel(const NTV2Channel inCSC, const bool inIsKeyInput=false)
virtual bool DMABufferLock(const NTV2Buffer &inBuffer, bool inMap=false, bool inRDMA=false)
Page-locks the given host buffer to reduce transfer time and CPU usage of DMA transfers.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=false)
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
@ NTV2_XptFrameBuffer1DS2Input
virtual bool IsDeviceReady(const bool inCheckValid=(0))
static const ULWord kDemoAppSignature((((uint32_t)( 'D'))<< 24)|(((uint32_t)( 'E'))<< 16)|(((uint32_t)( 'M'))<< 8)|(((uint32_t)( 'O'))<< 0))
ULWord GetVideoWriteSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
Identical to the GetVideoActiveSize function, except rounds the result up to the nearest 4K page size...
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
virtual void StartProducerThread(void)
Starts my capture thread.
virtual bool SetTsiFrameEnable(const bool inIsEnabled, const NTV2Channel inChannel)
Enables or disables SMPTE 425 two-sample interleave (Tsi) frame mode on the device.
@ NTV2_INPUTSOURCE_HDMI1
Identifies the 1st HDMI video input.
ULWord acBufferLevel
Number of buffered frames in driver ready to capture or play.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
NTV2VANCMode
These enum values identify the available VANC modes.
virtual uint32_t RecoverAudio(NTV2Buffer &anc, uint32_t ancSize, NTV2Buffer &audio)
Recover audio from ancillary data.
bool fWithAnc
If true, also capture Anc.
#define NTV2_IS_VALID_CHANNEL(__x__)
@ NTV2_DISABLE_TASKS
0: Disabled: Device is completely configured by controlling application(s) – no driver involvement.
@ NTV2_VANCMODE_INVALID
This identifies the invalid (unspecified, uninitialized) VANC mode.
void * GetHostAddress(const ULWord inByteOffset, const bool inFromEnd=false) const
NTV2OutputCrosspointID
Identifies a widget output, a signal source, that potentially can drive another widget's input (ident...
virtual std::string GetDescription(void) const
std::string toString(const bool inNormalized=false) const
NTV2Buffer fAudioBuffer
Host audio buffer.
@ NTV2_LHIHDMIColorSpaceYCbCr
CNTV2DemoCommon::ACFrameRange fFrames
AutoCirculate frame count or range.
@ NTV2_XptFrameBuffer2Input
bool fWithDolby
If true, also capture Dolby.
UWord lastFrame(void) const
bool IsRunning(void) const
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
bool NTV2DeviceCanDoInputSource(const NTV2DeviceID inDeviceID, const NTV2InputSource inInputSource)
virtual AJAStatus Run(void)
Runs me.
#define AUTOCIRCULATE_WITH_HDMIAUX
Use this to AutoCirculate with HDMI auxiliary data.
bool IsRGBFormat(const NTV2FrameBufferFormat format)
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
ULWord fNumAnc2Bytes
Actual number of captured F2 anc bytes.
virtual bool GetHDMIInputColor(NTV2LHIHDMIColorSpace &outValue, const NTV2Channel inChannel=NTV2_CHANNEL1)
Answers with the current colorspace for the given HDMI input.
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
NTV2OutputXptID GetInputSourceOutputXpt(const NTV2InputSource inInputSource, const bool inIsSDI_DS2=false, const bool inIsHDMI_RGB=false, const UWord inHDMI_Quadrant=0)
virtual void Quit(void)
Gracefully stops me from running.
AJALabelValuePairs Get(const bool inCompact=(0)) const
bool fWithAudio
If true, also capture Audio.
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=false)
Returns the video format of the signal that is present on the given input source.
bool WithCustomAnc(void) const
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
std::string NTV2ChannelToString(const NTV2Channel inValue, const bool inForRetailDisplay=false)
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
NTV2PixelFormat fPixelFormat
Pixel format to use.
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=true, NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
virtual AJAStatus Start()
ULWord acFramesDropped
Total number of frames dropped since CNTV2Card::AutoCirculateStart called.
std::vector< AJALabelValuePair > AJALabelValuePairs
An ordered sequence of label/value pairs.
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
#define AJA_FAILURE(_status_)
std::string fAudioDataFilePath
Optional path to Audio binary data file.
std::string fAncDataFilePath
Optional path to Anc binary data file.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
NTV2Buffer & AncBuffer(void)
@ NTV2_XptFrameBuffer2DS2Input
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
@ DEVICE_ID_NOTFOUND
Invalid or "not found".
@ NTV2_OEM_TASKS
2: OEM: Device is configured by controlling application(s), with minimal driver involvement.
virtual void ConsumeFrames(void)
Repeatedly consumes frames from the circular buffer (until global quit flag set).