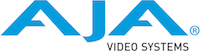 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
7 #ifndef __NTV2_CEA708_DECODER_
8 #define __NTV2_CEA708_DECODER_
84 virtual void Reset (
void);
107 virtual bool SetSMPTE334AncData (
const UByte * pInAncData,
const size_t inByteCount);
118 virtual bool SetSMPTE334AncData (
const UWord * pInAncData,
const size_t inWordCount);
126 virtual bool GetSMPTE334AncData (
const UByte ** ppAncData,
size_t & outAncSize)
const;
140 virtual bool ParseSMPTE334AncPacket (
bool & outHasParityErrors);
153 virtual bool ParseSMPTE334AncPacket (
void);
154 virtual bool ParseSMPTE334CCTimeCodeSection (
size_t & index);
155 virtual bool ParseSMPTE334CCDataSection (
size_t & index);
156 virtual bool ParseSMPTE334CCServiceInfoSection (
size_t & index);
157 virtual bool UpdateServiceInfo (
void);
159 virtual bool Clear608CaptionData (
void);
169 virtual bool GetCaptionChannelPacket (
UBytePtr & outDataPtr,
size_t & outSize);
173 virtual bool GetNextServiceBlockInfoFromQueue (
const size_t svcIndex,
size_t & outBlockSize,
size_t & outDataSize,
int & outServiceNum,
bool & outIsExtended)
const;
174 virtual size_t GetNextServiceBlockFromQueue (
const size_t svcIndex, std::vector<UByte> & outData);
175 virtual size_t GetNextServiceBlockDataFromQueue (
const size_t svcIndex, std::vector<UByte> & outData);
176 virtual size_t GetNextServiceBlockFromQueue (
const size_t svcIndex,
UByte * pOutDataBuffer);
177 virtual size_t GetNextServiceBlockDataFromQueue (
const size_t svcIndex,
UByte * pOutDataBuffer);
181 virtual void ClearAllCaptionChannelPacketInfo (
void);
183 virtual int GetNumCompleteCaptionChannelPacketInfo (
int * pCompleteCount =
NULL,
int * pStartedCount =
NULL);
184 virtual void ResetCaptionChannelPacketInfoForNewCDP (
void);
185 virtual void CloseCurrentCaptionChannelPacketInfo (
void);
186 virtual bool AddCaptionChannelPacketInfoData (
UByte newData);
187 virtual bool GetCaptionChannelPacketInfoData (
int index,
UByte ** ppData,
int * pSize);
189 virtual bool ParseAllCaptionChannelPackets (
void);
197 virtual bool DebugParseSMPTE334AncPacket (
bool & outHasErrors);
198 virtual bool DebugParseCDPHeader (
const UByte * pInData,
size_t pktIndex,
size_t maxPacketSize,
size_t * pNewIndex,
NTV2_CC708CDPHeaderPtr pHdr,
bool & outHasErrors);
199 virtual bool DebugParseCDPTimecode (
const UByte * pInData,
size_t pktIndex,
size_t maxPacketSize,
size_t * pNewIndex,
NTV2_CC708CDPTimecodeSectionPtr pTC,
bool & outHasErrors);
200 virtual bool DebugParseCDPData (
const UByte * pInData,
size_t pktIndex,
size_t maxPacketSize,
size_t * pNewIndex,
NTV2_CC708CDPDataSectionPtr pCC,
bool & outHasErrors);
202 virtual bool DebugParseCDPFutureSection (
const UByte * pInData,
size_t pktIndex,
size_t maxPacketSize,
size_t * pNewIndex,
bool & outHasErrors);
203 virtual bool DebugParseCDPFooter (
const UByte * pInData,
size_t pktIndex,
size_t maxPacketSize,
size_t * pNewIndex,
NTV2_CC708CDPFooterPtr pFtr,
bool & outHasErrors);
212 virtual bool DebugParse608CaptionData (
UByte inChar1,
UByte inChar2,
const int inFieldNum);
213 virtual bool DebugParse708CaptionData (
const UByte * pInData,
const size_t byteCount,
const bool bHexDump =
false)
const;
214 virtual size_t DebugParse708CaptionChannelPacket (
const UByte * pInData,
const size_t byteCount,
size_t currIndex)
const;
215 virtual size_t DebugParse708ServiceBlock (
const UByte * pInData,
const size_t byteCount,
size_t currIndex)
const;
217 virtual inline int GetDebugFrameNum (
void)
const {
return mDebugFrameNumber;}
228 virtual void StartNewCaptionChannelPacketInfo (
int seqNum,
int expectedSize);
233 UByte mRawVancDataBuffer [256];
234 size_t mRawVancByteCount;
238 UByte mCC708FrameData [256];
239 size_t mCC708FrameDataByteCount;
242 int mDebugFrameNumber;
244 UWord mPreviousSequenceCount;
245 bool mIsNonContiguousFrame;
250 size_t mCurrentCCPIndex;
265 int mDebugPrintOffset;
269 #endif // __NTV2_CEA708_DECODER_
virtual void GetCDPData(NTV2_CC708CDPPtr pCDP) const
Declares the CNTV2Caption708Service class.
const int NTV2_CC708MaxNumServices
AJARefPtr< CNTV2CaptionDecoder708 > CNTV2CaptionDecoder708Ptr
struct CaptionChannelPacketInfo * CaptionChannelPacketInfoPtr
const size_t NTV2_CC708_MaxCaptionChannelPacketSize
int ccpStatus
kCaptionChannelClear/Started/Complete
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
struct CaptionChannelPacketInfo CaptionChannelPacketInfo
I am a container for a "Caption Channel Packet" – i.e., a wrapper for a clump of CEA-708 caption data...
I am a container for all of the CEA-708 "Service Information" that a decoder or encoder needs to keep...
virtual NTV2CaptionLogMask SetLogMask(const NTV2CaptionLogMask inLogMask)
Specifies what, if any, debug information I will write to my log stream.
virtual const NTV2_CC708ServiceData & GetAllServiceInfoPtr(void) const
const int kMaxNumCaptionChannelPacketInfo
The max number of CaptionChannelPackets we can deal with in a single frame (a Caption Channel Packet ...
int ccpSize
The number of data words we're expecting according to the packet header (note, NOT the "packet_size_c...
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
@ kCaptionChannelPacketClear
"Empty" Caption Channel Packet
@ kCaptionChannelPacketStarted
We've started pouring data into a Caption Channel Packet but haven't yet finished.
I am a container for a "Caption Channel Packet" – i.e., a wrapper for a clump of CEA-708 caption data...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
UByte ccpData[NTV2_CC708_MaxCaptionChannelPacketSize]
Raw packet data.
int ccpCurrentSize
The number of data words actually loaded into the ccpData array.
uint64_t NTV2CaptionLogMask
Selectors to control what information is logged.
I am a simple, thread-safe queue of CEA-608 caption byte pairs.
Declaration for the CNTV2Caption608DataQueue class.
int ccpSequenceNum
The sequence number (0, 1, 2, or 3) from the header.
This structure encapsulates all possible CEA-608 caption data bytes that may be associated with a giv...
I am a CEA-708 captioning decoder used primarily to obtain CEA-608 captions carried in CEA-708 anc da...
virtual const CaptionData & GetCurrentFrameCC608Data(void) const
@ kCaptionChannelPacketComplete
The Caption Channel Packet is "complete": it has all the data it's going to get.
virtual NTV2Line21Channel GetDisplayChannel(void) const
Answers with the caption channel that I'm currently focused on (or that I'm currently "burning" into ...