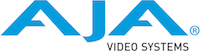 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
7 #ifndef __NTV2_CEA708_SERVICEINFO_
8 #define __NTV2_CEA708_SERVICEINFO_
30 std::ostream & Print (std::ostream & inOutStream)
const;
82 std::ostream &
Print (std::ostream & inOutStream)
const;
95 std::ostream &
Print (std::ostream & inOutStream)
const;
122 virtual bool InitAllServiceInfo (
void);
123 virtual bool InitCCServiceInfo (
const int inServiceIndex);
129 virtual bool CopyOneServiceInfo (
const int inServiceIndex,
const NTV2_CC708ServiceInfo & inSrcSvcInfo);
131 virtual bool CompareOneServiceInfo (
const int inServiceIndex,
const NTV2_CC708ServiceInfo & inSrcSvcInfo)
const;
133 virtual int NumActiveCDPServiceInfo (
const int startIndex = 0);
134 virtual bool ResetStartIndex (
void);
138 virtual int AdvanceToNextStartIndex (
const bool bIncludeCurrentIndex);
140 virtual bool SetServiceInfoActive (
const int inServiceIndex,
const bool inIsActive);
144 return (inServiceIndex >= 0 && inServiceIndex <
NTV2_CC708MaxNumServices) ? m_serviceData.serviceInfo [inServiceIndex].bSvcActive :
false;
149 return (inServiceIndex >= 0 && inServiceIndex <
NTV2_CC708MaxNumServices) ? m_serviceData.serviceInfo [inServiceIndex].captionSvcNumber : 0;
156 virtual bool SetServiceInfoEasyReader (
const int inServiceIndex,
const bool inIsEasyReader);
160 return (inServiceIndex >= 0 && inServiceIndex <
NTV2_CC708MaxNumServices) ? m_serviceData.serviceInfo [inServiceIndex].easyReader :
false;
163 virtual bool SetServiceInfoWideAspect (
const int inServiceIndex,
const bool inIsWideAspect);
167 return (inServiceIndex >= 0 && inServiceIndex <
NTV2_CC708MaxNumServices) ? m_serviceData.serviceInfo [inServiceIndex].wideAspect :
false;
170 virtual bool SetServiceInfoDigitalCC (
const int inServiceIndex,
const bool inIsDigitalCC);
174 return (inServiceIndex >= 0 && inServiceIndex <
NTV2_CC708MaxNumServices) ? m_serviceData.serviceInfo [inServiceIndex].digitalCC :
false;
177 virtual bool SetServiceInfoChangeFlag (
const bool inChangeFlag);
181 return m_serviceData.bChange;
185 virtual std::ostream & Print (std::ostream & inOutStream)
const;
199 #endif // __NTV2_CEA708_SERVICEINFO_
std::ostream & Print(std::ostream &inOutStream) const
virtual bool GetServiceInfoEasyReader(const int inServiceIndex)
struct NTV2_CC708ServiceInfo NTV2_CC708ServiceInfo
bool bSvcActive
True if service is active and should be included in ccsvcinfo_section.
const int NTV2_CC708MaxNumServices
struct NTV2_CC708ServiceData NTV2_CC708ServiceData
@ NTV2_CC708CDPSvcInfo_SvcInfoComplete
virtual bool GetServiceInfoWideAspect(const int inServiceIndex) const
@ NTV2_CC708CDPSvcInfo_Line21Field
@ NTV2_CC708CDPSvcInfo_EasyReader
const NTV2_CC708ServiceLanguage NTV2_CC708SvcLang_Spanish(NTV2_CC708ServiceLanguage('s', 'p', 'a'))
int captionSvcNumber
Service number (Line 21 data = 0; 708 data = 1 - 16)
@ NTV2_CC708CDPSvcInfo_DigitalCC
I am a container for all of the CEA-708 "Service Information" that a decoder or encoder needs to keep...
const NTV2_CC708ServiceLanguage NTV2_CC708SvcLang_English(NTV2_CC708ServiceLanguage('e', 'n', 'g'))
bool digitalCC
True if 708 captions, false if Line 21 (608)
virtual bool GetServiceInfoChangeFlag(void) const
virtual int GetStartIndex(void) const
std::ostream & Print(std::ostream &inOutStream) const
virtual int GetCaptionServiceNumber(const int inServiceIndex) const
std::ostream & Print(std::ostream &inOutStream) const
virtual bool GetServiceInfoDigitalCC(const int inServiceIndex) const
@ NTV2_CC708CDPSvcInfo_SvcInfoStart
bool IsEqual(const NTV2_CC708ServiceInfo &inCompareInfo) const
UByte csn_line21field
The remainder of that byte.
@ NTV2_CC708CDPSvcInfo_SvcInfoChange
NTV2_CC708ServiceInfo serviceInfo[NTV2_CC708MaxNumServices]
struct NTV2_CC708ServiceLanguage NTV2_CC708ServiceLanguage
@ NTV2_CC708CDPSvcInfo_WideAspectRatio
const int NTV2_CC708MaxCDPServices
virtual const NTV2_CC708ServiceData & GetAllServiceInfoPtr(void) const
const NTV2_CC708ServiceLanguage NTV2_CC708SvcLang_French(NTV2_CC708ServiceLanguage('f', 'r', 'e'))
NTV2_CC708ServiceLanguage language
Caption language (e.g. 'ENG')
const int NTV2_CC708PrimaryCaptionServiceNum
virtual bool GetServiceInfoActive(const int inServiceIndex) const
std::ostream & operator<<(std::ostream &inOutStream, const NTV2_CC708ServiceLanguage &inLanguage)
Declares the NTV2CaptionLogMask, and the CNTV2CaptionLogConfig class.