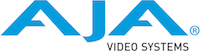 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
7 #ifndef __NTV2_CAPTIONLOGGING_
8 #define __NTV2_CAPTIONLOGGING_
28 #define AJACC_ASSERT NTV2_ASSERT
31 #define UHEX2(_c_) HEX0N(uint16_t(_c_),2)
32 #define HEX4(_uword_) HEX0N((_uword_),4)
61 #define Line21ColumnSetToString Line21RowSetToString
97 static std::ostream & DumpMemory (
const void * pInStartAddress,
98 const size_t inByteCount,
99 std::ostream & inOutputStream = std::cout,
100 const size_t inRadix = 16,
101 const size_t inBytesPerGroup = 4,
102 const size_t inGroupsPerLine = 8,
103 const size_t inAddressRadix = 16,
104 const bool inShowAscii =
true,
105 const size_t inAddrOffset = 0);
113 static std::string HexDump32Bytes (
const void * pInStartAddress,
const size_t inByteCount,
const size_t inLimitBytes = 32);
134 static std::ostream & DumpYBytes_2vuy (
const UByte * pInVideoLine,
135 std::ostream & inOutputStream,
136 const unsigned inFromPixel = 0,
137 const unsigned inToPixel = 719,
138 const bool inShowRuler =
true,
139 const unsigned inHiliteRangeFrom = 9999,
140 const unsigned inHiliteRangeTo = 9999);
161 static std::ostream & DumpYBytes_2vuy (
const std::vector<uint8_t> & inVideoLine,
162 std::ostream & inOutputStream,
163 const size_t inFromPixel = 0,
164 const size_t inToPixel = 719,
165 const bool inShowRuler =
true,
166 const size_t inHiliteRangeFrom = 9999,
167 const size_t inHiliteRangeTo = 9999);
169 static std::string GetSeverityLabel(
const unsigned inSeverity);
202 virtual inline bool TestLogMask (
const NTV2CaptionLogMask inLogMask,
const bool inExact =
false)
const {
if (inExact)
return (GetLogMask() & inLogMask) == inLogMask;
else return (GetLogMask() & inLogMask) ?
true :
false;}
208 virtual void SetLogLabel (
const std::string & inNewLabel);
214 virtual void AppendToLogLabel (
const std::string & inString);
220 virtual const std::string & GetLogLabel (
void)
const;
224 virtual void SetLogStream (std::ostream & inOutputStream);
226 virtual std::ostream & Log (
void)
const;
242 #endif // __NTV2_CAPTIONLOGGING_
void * mpLabelLock
Protects my debug label from simultaneous access by more than one thread.
Line21RowSetConstIter Line21ColumnSetConstIter
A const iterator for a Line21ColumnSet.
void SetDefaultCaptionLogMask(const NTV2CaptionLogMask inMask)
Sets the default log mask that will be used by newly-created objects in the caption library.
const uint64_t kCaptionLog_608ShowAllScreens(0x0000000000010000)
Log screens for all 608 channels.
const uint64_t kCaptionLog_Line21DetectSuccess(0x0000000000000100)
Log line 21 waveform detect successes.
NTV2CaptionLogMask GetDefaultCaptionLogMask(void)
Answers with the default log mask used when creating new objects in the caption library.
const uint64_t kCaptionLog_Line21DecodeFail(0x0000000000000800)
Log line 21 waveform decode failures.
const uint64_t kCaptionLog_608ShowScreenAttrs(0x0000000000020000)
Log screen attributes for 608 channel of interest.
std::ostream & GetDefaultCaptionLogOutputStream(void)
const uint64_t kCaptionLog_Decode608(0x0000000000000001)
Log decode (input) 608 events.
std::string mLogLabel
My debug label.
void SetDefaultCaptionLogOutputStream(std::ostream &inOutputStream)
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
Line21RowSet Line21ColumnSet
A set of caption column numbers.
virtual NTV2CaptionLogMask GetLogMask(void) const
Answers with my current caption logging bit mask.
std::set< int > Line21RowSet
A set of caption row numbers.
virtual NTV2CaptionLogMask SetLogMask(const NTV2CaptionLogMask inLogMask)
Specifies what, if any, debug information I will write to my log stream.
const uint64_t kCaptionLog_All(0xFFFFFFFFFFFFFFFF)
Log everything possible.
const uint64_t kCaptionLog_DecodeXDS(0x0000000000000002)
Log decode (input) XDS info.
const uint64_t kCaptionLog_Line21DecodeSuccess(0x0000000000000400)
Log line 21 waveform decode successes.
const uint64_t kCaptionLog_Off(0x0000000000000000)
Don't log anything.
Line21RowSet::const_iterator Line21RowSetConstIter
A const iterator for a Line21RowSet.
const uint64_t kCaptionLog_Line21DetectFail(0x0000000000000200)
Log line 21 waveform detect failures.
uint64_t NTV2CaptionLogMask
Selectors to control what information is logged.
const uint64_t kCaptionLog_DecodeVANC(0x0000000000100000)
Log decode (input) VANC data.
Declares enums and structs used by all platform drivers and the SDK.
virtual bool TestLogMask(const NTV2CaptionLogMask inLogMask, const bool inExact=false) const
Answers true if the given log mask bits are set in my current log mask.
const uint64_t kCaptionLog_608ShowScreen(0x0000000000008000)
Log screen for 608 channel of interest.
NTV2CaptionLogMask mLogMask
Determines what messages are logged.
std::string Line21RowSetToString(const Line21RowSet &inRowSet)
const uint64_t kCaptionLog_DecodeCDP(0x0000000000200000)
Log decode (input) CDP data.