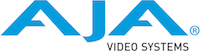 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
32 int main (
int argc,
const char ** argv)
38 uint32_t channelNumber (2);
40 int doMultiChannel (0);
50 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
51 {
"dolbyFile",
'f',
POPT_ARG_STRING, &pDolbyName, 0,
"dolby audio to play",
"file name" },
52 {
"videoFormat",
'v',
POPT_ARG_STRING, &pVideoFormat, 0,
"video format to produce",
"'?' or 'list' to list"},
53 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list"},
54 {
"channel",
'c',
POPT_ARG_INT, &channelNumber, 0,
"channel to use",
"1-8"},
67 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
68 const string videoFormatStr (pVideoFormat ? pVideoFormat :
"");
72 if (videoFormatStr ==
"?" || videoFormatStr ==
"list")
77 cerr <<
"## ERROR: Invalid '--videoFormat' value '" << videoFormatStr <<
"' -- expected values:" << endl
83 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
85 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
89 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
94 if (channelNumber < 2 || channelNumber > 4)
95 {cerr <<
"## ERROR: Invalid channel number '" << channelNumber <<
"' -- expected 2 thru 4" << endl;
return 2;}
99 const string fileStr (pDolbyName ? pDolbyName :
"");
100 if (!fileStr.empty ())
104 pDolbyFile = &fileIO;
110 (noAudio ?
false :
true),
114 doMultiChannel ?
true :
false,
115 doRamp ?
true :
false,
126 {cerr <<
"## ERROR: Initialization failed" << endl;
return 3;}
131 cout <<
" Playout Playout Frames" << endl
132 <<
" Frames Buffer Dropped" << endl;
135 ULWord framesProcessed, framesDropped, bufferLevel;
138 player.
GetACStatus (framesProcessed, framesDropped, bufferLevel);
139 cout << setw (9) << framesProcessed << setw (9) << bufferLevel << setw (9) << framesDropped <<
"\r" << flush;
static std::string GetVideoFormatStrings(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_NON_4KUHD, const std::string inDeviceSpecifier=std::string())
NTV2Channel GetNTV2ChannelForIndex(const ULWord inIndex0)
virtual bool IsRunning(void) const
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
Declares the AJATime class.
void SignalHandler(int inSignal)
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
int main(int argc, const char **argv)
virtual AJAStatus Run(void)
Runs me.
Header file for NTV2DolbyPlayer demonstration class.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
AJAStatus Open(const std::string &fileName, const int flags, const int properties)
static NTV2VideoFormat GetVideoFormatFromString(const std::string &inStr, const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_NON_4KUHD)
Returns the NTV2VideoFormat that matches the given string.
I am an object that can play out a test pattern (with timecode) to an output of an AJA device with or...
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
static NTV2FrameBufferFormat GetPixelFormatFromString(const std::string &inStr)
Returns the NTV2FrameBufferFormat that matches the given string.
Declares numerous NTV2 utility functions.
poptContext poptFreeContext(poptContext con)
static AJAStatus Open(bool incrementRefCount=false)
Declares the AJAFileIO class.
poptContext poptGetContext(const char *name, int argc, const char **argv, const struct poptOption *options, unsigned int flags)
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
@ VIDEO_FORMATS_NON_4KUHD
virtual void Quit(void)
Gracefully stops me from running.
int poptGetNextOpt(poptContext con)
static bool gGlobalQuit(false)
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDeviceSpecifier=std::string())
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual void GetACStatus(ULWord &outGoodFrames, ULWord &outDroppedFrames, ULWord &outBufferLevel)
Provides status information about my output (playout) process.
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)