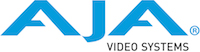 |
AJA NTV2 SDK
17.6.0.1664
NTV2 SDK 17.6.0.1664
|
Go to the documentation of this file.
30 int main (
int argc,
const char ** argv)
35 int doMultiFormat (0);
45 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
47 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
48 {
"input",
'i',
POPT_ARG_STRING, &pInputSrcSpec, 0,
"SDI input to use",
"1-8, ?=list" },
56 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
58 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
61 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
71 if (inputSourceStr ==
"?" || inputSourceStr ==
"list")
72 {cout << legalSources << endl;
return 0;}
74 {cerr <<
"## ERROR: Input source '" << inputSourceStr <<
"' not one of:" << endl << legalSources << endl;
return 1;}
77 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
79 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
83 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
101 const string hdg1 (
" Capture Playout Capture Playout"),
102 hdg2a(
" Fields Fields Fields Buffer Buffer"),
103 hdg2b(
" Frames Frames Frames Buffer Buffer"),
104 hdg3 (
"Processed Dropped Dropped Level Level"),
105 hdg2 (noFieldMode ? hdg2b : hdg2a);
110 {cerr <<
"## ERROR: Initialization failed, status=" << status << endl;
return 4;}
116 cout << hdg1 << endl << hdg2 << endl << hdg3 << endl;
119 ULWord totalFrames(0), inputDrops(0), outputDrops(0), inputBufferLevel(0), outputBufferLevel(0);
120 burner.
GetStatus (totalFrames, inputDrops, outputDrops, inputBufferLevel, outputBufferLevel);
121 cout << setw(9) << totalFrames << setw(9) << inputDrops << setw(9) << outputDrops
122 << setw(9) << inputBufferLevel << setw(9) << outputBufferLevel <<
"\r" << flush;
NTV2Channel NTV2InputSourceToChannel(const NTV2InputSource inInputSource)
Converts a given NTV2InputSource to its equivalent NTV2Channel value.
static NTV2PixelFormat GetPixelFormatFromString(const std::string &inStr, const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2PixelFormat that matches the given string.
bool fIsFieldMode
True if Field Mode, otherwise Frame Mode.
virtual void GetStatus(ULWord &outNumProcessed, ULWord &outCaptureDrops, ULWord &outPlayoutDrops, ULWord &outCaptureLevel, ULWord &outPlayoutLevel)
Provides status information about my input (capture) and output (playout) processes.
static bool gGlobalQuit((0))
Set this "true" to exit gracefully.
void SignalHandler(int inSignal)
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
bool fSuppressAudio
If true, suppress audio; otherwise include audio.
NTV2TCIndex fTimecodeSource
Timecode source to use.
int main(int argc, const char **argv)
NTV2PixelFormat fPixelFormat
The pixel format to use.
static bool IsValidDevice(const std::string &inDeviceSpec)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
NTV2TCIndex NTV2ChannelToTimecodeIndex(const NTV2Channel inChannel, const bool inEmbeddedLTC=false, const bool inIsF2=false)
Converts the given NTV2Channel value into the equivalent NTV2TCIndex value.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
I capture individual fields from an interlaced video signal provided to an SDI input....
static AJAStatus Open(bool incrementRefCount=false)
NTV2InputSource fInputSource
The device input connector to use.
static std::string GetInputSourceStrings(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
@ NTV2_IOKINDS_ALL
Specifies any/all input/output kinds.
Header file for the NTV2FieldBurn demonstration class.
static NTV2InputSource GetInputSourceFromString(const std::string &inStr, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2InputSource that matches the given string.
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
Configures an NTV2Burn or NTV2FieldBurn instance.
virtual AJAStatus Run(void)
Runs me.