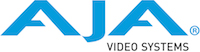 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
27 #pragma warning(disable : 4996)
35 int main(
int argc,
const char ** argv)
62 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
65 const string deviceSpec(pDeviceSpec ? pDeviceSpec :
"0");
67 {cerr <<
"## ERROR: Opening device '" << deviceSpec <<
"' failed" << endl;
return 1;}
73 const string deviceStr(pDeviceID ? pDeviceID :
"");
74 if (!deviceStr.empty())
77 size_t index =
aja::stoul(deviceStr, &checkIndex, 10);
81 if ((index == 0) || (index > deviceList.size()))
82 {cerr <<
"## ERROR: Bad device index '" << index <<
"'" << endl;
return 1;}
83 deviceID = deviceList.at(index-1);
94 <<
xHEX0N(eBoardID,8) <<
")" << endl;
106 <<
xHEX0N(deviceID,8) <<
")" << endl;
125 <<
" (" <<
xHEX0N(eBoardID,8) <<
")";
129 if (deviceID == eBoardID)
133 <<
") loaded successfully" << endl;
140 <<
" (" <<
xHEX0N(eBoardID,8) <<
")";
146 if (!deviceID && !isInfo)
149 if (deviceList.empty())
150 {cout <<
"No loadable devices found" << endl;
break;}
152 cout <<
DEC(deviceList.size()) <<
" Device(s) for dynamic loading:" << endl;
153 for (
size_t ndx(0); ndx < deviceList.size(); ndx++)
157 <<
" (" <<
xHEX0N(deviceList.at(ndx),8) <<
")" << endl;
173 UWord bitfileVersion(0);
174 string flags =
" Active";
177 <<
" DevID " <<
xHEX0N(currentDeviceID,8)
178 <<
" DesID " <<
xHEX0N(designID,2) <<
" DesVer " <<
xHEX0N(designVersion,2)
179 <<
" BitID " <<
xHEX0N(bitfileID,2) <<
" BitVer " <<
xHEX0N(bitfileVersion,2)
180 <<
" " << flags << endl;
195 <<
" DevID " <<
xHEX0N(it->deviceID,8)
196 <<
" DesID " <<
xHEX0N(it->designID,2) <<
" DesVer " <<
xHEX0N(it->designVersion,2)
197 <<
" BitID " <<
xHEX0N(it->bitfileID,2) <<
" BitVer " <<
xHEX0N(it->bitfileVersion,2)
198 <<
" " << flags << endl;
unsigned long stoul(const std::string &str, std::size_t *idx, int base)
virtual bool LoadDynamicDevice(const NTV2DeviceID inDeviceID)
Quickly, dynamically loads the given device ID firmware.
Declares device capability functions.
static ULWord ConvertToBitfileID(const NTV2DeviceID inDeviceID)
#define NTV2_BITFILE_FLAG_CLEAR
This is a clear bitfile.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
virtual bool AddDynamicDirectory(const std::string &inDirectory)
Adds all bitfiles found in the given host file directory to the list of available dynamic bitfiles.
int main(int argc, const char **argv)
#define NTV2_BITFILE_FLAG_PARTIAL
This is a partial bitfile.
#define BITSTREAM_VERSION
Bitstream version register.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
virtual bool AddDirectory(const std::string &inDirectory)
Add the bitfile(s) at the given path to the list of bitfiles.
I manage and cache any number of bitfiles for any number of NTV2 devices/designs.
virtual const NTV2BitfileInfoList & GetBitfileInfoList(void) const
Returns an NTV2BitfileInfoList standard C++ vector.
Declares the CNTV2DeviceScanner class.
virtual NTV2DeviceIDList GetDynamicDeviceList(void)
std::string NTV2DeviceIDToString(const NTV2DeviceID inValue, const bool inForRetailDisplay=false)
Enumerations for controlling NTV2 devices.
Declares numerous NTV2 utility functions.
poptContext poptFreeContext(poptContext con)
std::string NTV2Version(const bool inDetailed=false)
std::vector< NTV2BitfileInfo > NTV2BitfileInfoList
virtual bool CanLoadDynamicDevice(const NTV2DeviceID inDeviceID)
I interrogate and control an AJA video/audio capture/playout device.
std::vector< ULWord > NTV2ULWordVector
An ordered sequence of ULWords.
#define POPT_ARGFLAG_OPTIONAL
virtual bool BitstreamStatus(NTV2ULWordVector &outRegValues)
Types and defines shared between NTV2 user application interface and Linux device driver.
Declares the CNTV2Bitfile class.
poptContext poptGetContext(const char *name, int argc, const char **argv, const struct poptOption *options, unsigned int flags)
NTV2BitfileInfoList::const_iterator NTV2BitfileInfoListConstIter
int poptGetNextOpt(poptContext con)
Private include file for all ajabase sources.
virtual NTV2DeviceID GetDeviceID(void)
Declares the CNTV2BitfileManager class that manages Xilinx bitfiles.
std::vector< NTV2DeviceID > NTV2DeviceIDList
An ordered list of NTV2DeviceIDs.
#define xHEX0N(__x__, __n__)
#define NTV2_BITFILE_FLAG_TANDEM
This is a tandem bitfile.
virtual bool GetRunningFirmwareRevision(UWord &outRevision)
Reports the revision number of the currently-running firmware.
#define DECN(__x__, __n__)