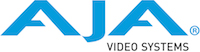 |
AJA NTV2 SDK
17.6.0.1688
NTV2 SDK 17.6.0.1688
|
Go to the documentation of this file.
8 #ifndef NTV2BITMANAGER_H
9 #define NTV2BITMANAGER_H
23 #define NTV2_BITFILE_FLAG_TANDEM BIT(0)
24 #define NTV2_BITFILE_FLAG_PARTIAL BIT(1)
25 #define NTV2_BITFILE_FLAG_CLEAR BIT(2)
70 virtual bool AddFile (
const std::string & inBitfilePath);
77 virtual bool AddDirectory (
const std::string & inDirectory);
82 virtual void Clear (
void);
88 virtual size_t GetNumBitfiles (
void);
107 virtual bool GetBitStream (
NTV2Buffer & outBitstream,
109 const ULWord inDesignVersion,
111 const ULWord inBitfileVersion,
112 const ULWord inBitfileFlags);
121 bool ReadBitstream (
const size_t inIndex);
123 typedef std::vector <NTV2Buffer> NTV2BitstreamList;
124 typedef NTV2BitstreamList::iterator NTV2BitstreamListIter;
127 NTV2BitstreamList _bitstreamList;
130 #endif // NTV2BITMANAGER_H
ULWord designVersion
Version of this core.
std::string designName
The design name for this bitfile.
Describes a user-space buffer on the host computer. I have an address and a length,...
NTV2DeviceID deviceID
The NTV2DeviceID for this firmware core+personality.
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
ULWord designID
Identifies the firmware core (the design base common to all its personalities).
ULWord bitfileID
Identifies the firmware personality.
I manage and cache any number of bitfiles for any number of NTV2 devices/designs.
virtual const NTV2BitfileInfoList & GetBitfileInfoList(void) const
Returns an NTV2BitfileInfoList standard C++ vector.
std::string bitfilePath
The path where this bitfile was found.
NTV2BitfileInfoList::iterator NTV2BitfileInfoListIter
ULWord bitfileVersion
Version of this personality.
std::vector< NTV2BitfileInfo > NTV2BitfileInfoList
NTV2BitfileInfoList::const_iterator NTV2BitfileInfoListConstIter
Declares enums and structs used by all platform drivers and the SDK.