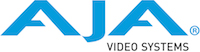 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
28 int main (
int argc,
const char ** argv)
35 int channelNumber (1);
36 int doMultiFormat (0);
47 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
48 {
"channel",
'c',
POPT_ARG_INT, &channelNumber, 0,
"channel to use",
"1-8" },
50 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
51 {
"frames", 0,
POPT_ARG_STRING, &pFramesSpec, 0,
"frames to AutoCirculate",
"num[@min] or min-max" },
52 {
"videoFormat",
'v',
POPT_ARG_STRING, &pVideoFormat, 0,
"video format to produce",
"'?' or 'list' to list" },
53 {
"anc",
'a',
POPT_ARG_STRING, &pAncFilePath, 0,
"play prerecorded anc",
"path/to/binary/data/file" },
62 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
64 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
67 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
74 if ((channelNumber < 1) || (channelNumber > 8))
75 {cerr <<
"## ERROR: Invalid channel number " << channelNumber <<
" -- expected 1 thru 8" << endl;
return 1;}
79 const string videoFormatStr (pVideoFormat ? pVideoFormat :
"");
82 if (videoFormatStr ==
"?" || videoFormatStr ==
"list")
85 { cerr <<
"## ERROR: Invalid '--videoFormat' value '" << videoFormatStr <<
"' -- expected values:" << endl
91 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
93 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
97 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
103 static const string legalFramesSpec (
"{frameCount}[@{firstFrameNum}] or {firstFrameNum}-{lastFrameNum}");
104 const string framesSpec (pFramesSpec ? pFramesSpec :
"");
105 if (!framesSpec.empty())
108 if (!parseResult.empty())
109 {cerr <<
"## ERROR: Bad 'frames' spec '" << framesSpec <<
"'\n## " << parseResult << endl;
return 1;}
112 {cerr <<
"## ERROR: Bad 'frames' spec '" << framesSpec <<
"'\n## Expected " << legalFramesSpec << endl;
return 1;}
114 if (noVideo && noAudio)
115 {cerr <<
"## ERROR: conflicting options '--novideo' and '--noaudio'" << endl;
return 1;}
118 string ancFilePath (pAncFilePath ? pAncFilePath :
"");
130 {cout <<
"## ERROR: Initialization failed: " <<
::AJAStatusToString(status) << endl;
return 1;}
141 cout <<
" Frames Frames Buffer" << endl
142 <<
" Played Dropped Level" << endl;
static NTV2VideoFormat GetVideoFormatFromString(const std::string &inStr, const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD, const std::string &inDevSpec=std::string())
Returns the NTV2VideoFormat that matches the given string.
static NTV2PixelFormat GetPixelFormatFromString(const std::string &inStr, const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2PixelFormat that matches the given string.
std::string & strip(std::string &str, const std::string &ws)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
I play out SD or HD test pattern (with timecode) to an output of an AJA device with or without audio ...
void SignalHandler(int inSignal)
ULWord GetProcessedFrameCount(void) const
ULWord GetBufferLevel(void) const
int main(int argc, const char **argv)
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
std::string setFromString(const std::string &inStr)
NTV2OutputDestination NTV2ChannelToOutputDestination(const NTV2Channel inChannel, const NTV2IOKinds inKinds=NTV2_IOKINDS_SDI)
Converts the given NTV2Channel value into its ordinary equivalent NTV2OutputDestination.
static bool IsValidDevice(const std::string &inDeviceSpec)
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
bool fSuppressAudio
If true, suppress audio; otherwise generate & xfer audio tone.
bool fSuppressVideo
If true, suppress video; otherwise generate & xfer test patterns.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
NTV2VideoFormat fVideoFormat
The video format to use.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
NTV2Channel fOutputChannel
The device channel to use.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
std::string fAncDataFilePath
Optional path to Anc binary data file to playout.
static std::string GetVideoFormatStrings(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD, const std::string inDevSpec=std::string())
static bool gGlobalQuit((0))
static AJAStatus Open(bool incrementRefCount=false)
bool fTransmitLTC
If true, embed LTC; otherwise embed VITC.
NTV2OutputDest fOutputDest
The desired output connector to use.
std::string AJAStatusToString(const AJAStatus inStatus, const bool inDetailed)
ULWord GetDroppedFrameCount(void) const
Configures an NTV2Player instance.
NTV2PixelFormat fPixelFormat
The pixel format to use.
virtual AJAStatus Run(void)
Runs me.
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
virtual void GetACStatus(AUTOCIRCULATE_STATUS &outStatus)
Provides status information about my output (playout) process.