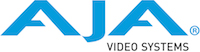 |
AJA NTV2 SDK
17.5.0.1492
NTV2 SDK 17.5.0.1492
|
Go to the documentation of this file.
32 int main (
int argc,
const char ** argv)
46 {
"input",
'i',
POPT_ARG_STRING, &pDeviceSpec, 0,
"input device to use",
"index#, serial#, or model" },
47 {
"output",
'o',
POPT_ARG_STRING, &pOutDevSpec, 0,
"output device",
"index#, serial#, or model" },
48 {
"tcsource",
't',
POPT_ARG_STRING, &pTcSource, 0,
"timecode source",
"'?' or 'list' to list" },
50 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
56 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
58 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
61 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
70 {cout <<
"## ERROR: No such output device '" << config.
fDeviceSpec2 <<
"'" << endl;
return 1;}
73 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
75 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
79 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
88 if (tcSourceStr ==
"?" || tcSourceStr ==
"list")
89 {cout << legalTCSources << endl;
return 0;}
91 {cerr <<
"## ERROR: Timecode source '" << tcSourceStr <<
"' not one of these:" << endl << legalTCSources << endl;
return 1;}
109 {cerr <<
"## ERROR: Initialization failed, status=" << status << endl;
return 4;}
115 cout <<
" Capture Playout Capture Playout" << endl
116 <<
" Frames Frames Frames Buffer Buffer" << endl
117 <<
"Processed Dropped Dropped Level Level" << endl;
ULWord acFramesProcessed
Total number of frames successfully processed since CNTV2Card::AutoCirculateStart called.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
static NTV2PixelFormat GetPixelFormatFromString(const std::string &inStr, const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2PixelFormat that matches the given string.
#define NTV2_IS_VALID_TIMECODE_INDEX(__x__)
bool fSuppressAudio
If true, suppress audio; otherwise include audio.
NTV2TCIndex fTimecodeSource
Timecode source to use.
int main(int argc, const char **argv)
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
NTV2PixelFormat fPixelFormat
The pixel format to use.
NTV2ACFrameRange fOutputFrames
Playout frame count or range.
static bool IsValidDevice(const std::string &inDeviceSpec)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
static void SignalHandler(int inSignal)
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Header file for the NTV2Burn4KQuadrant demonstration class.
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
static std::string GetTCIndexStrings(const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDeviceSpecifier=std::string(), const bool inIsInputOnly=(!(0)))
std::string fDeviceSpec2
Second AJA device to use (Burn4KQuadrant or BurnBoardToBoard only)
virtual AJAStatus Run(void)
Runs me.
ULWord acBufferLevel
Number of buffered frames in driver ready to capture or play.
static AJAStatus Open(bool incrementRefCount=false)
Instances of me can capture 4K/UHD video from one 4-channel AJA device, burn timecode into one quadra...
virtual void GetACStatus(AUTOCIRCULATE_STATUS &outInputStatus, AUTOCIRCULATE_STATUS &outOutputStatus)
Provides status information about my input (capture) and output (playout) processes.
static NTV2TCIndex GetTCIndexFromString(const std::string &inStr, const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDevSpec=std::string())
Returns the NTV2TCIndex that matches the given string.
ULWord acFramesDropped
Total number of frames dropped since CNTV2Card::AutoCirculateStart called.
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
NTV2ACFrameRange fInputFrames
Ingest frame count or range.
Configures an NTV2Burn or NTV2FieldBurn instance.
bool setCountOnly(const UWord inCount)
static bool gGlobalQuit((0))