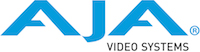 |
AJA NTV2 SDK
17.6.0.1688
NTV2 SDK 17.6.0.1688
|
Go to the documentation of this file.
19 #if defined (MSWindows) || defined (AJAWindows)
20 #define SIGQUIT SIGBREAK
49 static unsigned sAttaches (0);
50 static unsigned sDetaches (0);
58 cerr <<
"## WARNING: Terminating 'ntv2ccgrabber' due to device " << (inMessage ==
AJA_Pnp_DeviceAdded ?
"attach" :
"detach") << endl;
66 int main (
int argc,
const char ** argv)
71 const string legalFramesSpec (
"{frameCount}[@{firstFrameNum}] or {firstFrameNum}-{lastFrameNum}");
80 int channelNumber (1);
82 int doMultiFormat (0);
88 ::setlocale (LC_ALL,
"");
96 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
97 {
"channel",
'c',
POPT_ARG_INT, &channelNumber, 0,
"channel to use",
"1-8" },
99 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
100 {
"frames", 0,
POPT_ARG_STRING, &pFramesSpec, 0,
"frames to AutoCirculate",
"num[@min] or min-max" },
101 {
"input",
'i',
POPT_ARG_STRING, &pInputSrcSpec, 0,
"which SDI input",
"1-8, ?=list" },
102 {
"tcsource",
't',
POPT_ARG_STRING, &pTcSource, 0,
"timecode source",
"'?' or 'list' to list" },
103 {
"608chan", 0,
POPT_ARG_STRING, &pCaptionChannel, 0,
"608 cap chan to monitor", legalChannels.c_str() },
104 {
"608src", 0,
POPT_ARG_STRING, &pCaptionSource, 0,
"608 source to use", legal608Sources.c_str() },
105 {
"output", 0,
POPT_ARG_STRING, &pOutputMode, 0,
"608 output mode", legalOutputModes.c_str() },
116 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
118 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
121 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
128 if ((channelNumber < 1) || (channelNumber > 8))
129 {cerr <<
"## ERROR: Invalid channel number " << channelNumber <<
" -- expected 1 thru 8" << endl;
return 1;}
137 pDeviceSpec ? deviceSpec :
"");
138 if (inputSourceStr ==
"?" || inputSourceStr ==
"list")
139 {cout << legalSources << endl;
return 0;}
140 if (!inputSourceStr.empty())
143 {cerr <<
"## ERROR: Input source '" << inputSourceStr <<
"' not one of these:" << endl << legalSources << endl;
return 1;}
150 if (tcSourceStr ==
"?" || tcSourceStr ==
"list")
151 {cout << legalTCSources << endl;
return 0;}
153 {cerr <<
"## ERROR: Timecode source '" << tcSourceStr <<
"' not one of these:" << endl << legalTCSources << endl;
return 1;}
156 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
158 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
160 else if (!pixelFormatStr.empty())
164 {cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl << legalFBFs << endl;
return 2;}
172 {cerr <<
"## ERROR: Bad '608chan' value '" << pCaptionChannel <<
"' -- expected '" << legalChannels <<
"'" << endl;
return 1;}
176 const string captionSrcStr (pCaptionSource ? pCaptionSource :
"");
177 if (captionSrcStr ==
"?" || captionSrcStr ==
"list")
178 {cout <<
"## NOTE: Legal --608src values: " << legal608Sources << endl;
return 0;}
179 else if (!captionSrcStr.empty())
183 {cerr <<
"## ERROR: Bad '608src' value '" << captionSrcStr <<
"' -- expected '" << legal608Sources <<
"'" << endl;
return 1;}
187 const string outputModeStr (pOutputMode ? pOutputMode :
"");
188 if (outputModeStr ==
"?" || outputModeStr ==
"list")
189 {cout <<
"## NOTE: Legal --output values: " << legalOutputModes << endl;
return 0;}
190 else if (!outputModeStr.empty())
194 {cerr <<
"## ERROR: Bad 'output' value '" << outputModeStr <<
"' -- expected '" << legalOutputModes <<
"'" << endl;
return 1;}
199 cerr <<
"## WARNING: No input channel, or input source, or timecode source specified -- will use NTV2_INPUTSOURCE_SDI1, NTV2_CHANNEL1" << endl;
205 const string framesSpec (pFramesSpec ? pFramesSpec :
"");
206 if (!framesSpec.empty())
209 if (!parseResult.empty())
210 {cerr <<
"## ERROR: Bad 'frames' spec '" << framesSpec <<
"'\n## " << parseResult << endl;
return 1;}
213 {cerr <<
"## ERROR: Bad 'frames' spec '" << framesSpec <<
"'\n## Expected " << legalFramesSpec << endl;
return 1;}
226 {cerr <<
"## ERROR: 'ntv2ccgrabber' initialization failed with status " << status << endl;
return 2;}
236 {cerr <<
"## ERROR: 'ntv2ccgrabber' capture thread failed to run -- check for AutoCirculate messages in AJALogger?" << endl;
return 2;}
242 if (keyPressed ==
'q' || keyPressed ==
'Q')
244 else if (keyPressed >=
'1' && keyPressed <=
'9')
246 else if (keyPressed ==
'?')
247 cerr << endl <<
"## HELP: 1=CC1 2=CC2 3=CC3 4=CC4 5=Txt1 6=Txt2 7=Txt3 8=Txt4 Q=Quit H=HUD O=Output S=608Src P=PixFmt ?=Help" << endl;
248 else if (keyPressed ==
'h' || keyPressed ==
'H')
250 else if (keyPressed ==
'v' || keyPressed ==
'V')
252 else if (keyPressed ==
'o' || keyPressed ==
'O')
254 else if (keyPressed ==
'p' || keyPressed ==
'P')
256 else if (keyPressed ==
's' || keyPressed ==
'S')
bool fDoMultiFormat
If true, use multi-format/multi-channel mode, if device supports it; otherwise normal mode.
This is a platform-agnostic plug-and-play class that notifies a client when AJA devices are attached/...
static OutputMode StringToOutputMode(const std::string &inModeStr)
OutputMode fOutputMode
Desired output (captionStream, Screen etc)
Header file for NTV2CCGrabber demonstration class.
static NTV2PixelFormat GetPixelFormatFromString(const std::string &inStr, const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2PixelFormat that matches the given string.
NTV2Line21Channel fCaptionChannel
Caption channel to monitor (defaults to CC1)
static void PnpCallback(const AJAPnpMessage inMessage, void *pUserData)
This function gets called whenever an AJA device is attached or detached to/from the host.
static char ReadCharacterPress(void)
Returns the character that represents the last key that was pressed on the keyboard without waiting f...
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
virtual void SwitchPixelFormat(void)
Switches/rotates –pixelFormat.
#define NTV2_IS_VALID_TIMECODE_INDEX(__x__)
NTV2PixelFormat fPixelFormat
Pixel format to use.
int main(int argc, const char **argv)
@ AJA_Pnp_PciVideoDevices
@ kCaptionDataSrc_INVALID
std::string setFromString(const std::string &inStr)
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
@ NTV2_IOKINDS_ANALOG
Specifies analog input/output kinds.
virtual AJAStatus Run(void)
Runs me.
CaptionDataSrc fCaptionSrc
Caption data source (Line21? 608 VANC? 608 Anc? etc)
virtual void ToggleHUD(void)
Toggles the Head-Up-Display.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
virtual void SwitchOutput(void)
Switches/rotates –output mode.
@ NTV2_INPUTSOURCE_SDI1
Identifies the 1st SDI video input.
virtual AJAStatus Uninstall()
Uninstalls any previously-installed callback notifier.
@ NTV2_IOKINDS_SDI
Specifies SDI input/output kinds.
static bool IsValidDevice(const std::string &inDeviceSpec)
static bool gGlobalQuit((0))
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
static std::string GetLine21ChannelNames(std::string inDelimiter=", ")
Returns a string containing a concatenation of human-readable names of every available NTV2Line21Chan...
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
This class is used to configure an NTV2CCGrabber instance.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
static void SignalHandler(int inSignal)
NTV2Line21Channel StrToNTV2Line21Channel(const std::string &inStr)
Converts the given string into the equivalent NTV2Line21Channel value.
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
Declares numerous NTV2 utility functions.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
static std::string CaptionDataSrcToString(const CaptionDataSrc inDataSrc)
static std::string GetTCIndexStrings(const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDeviceSpecifier=std::string(), const bool inIsInputOnly=(!(0)))
#define IS_VALID_OutputMode(_x_)
Declares the AJAPnp (plug-and-play) class.
#define IS_VALID_CaptionDataSrc(_x_)
static AJAStatus Open(bool incrementRefCount=false)
NTV2ACFrameRange fFrames
AutoCirculate frame count or range.
NTV2Channel fInputChannel
The device channel to use.
static std::string GetInputSourceStrings(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
NTV2TCIndex fTimecodeSource
Timecode source to use (if any)
virtual void SetCaptionDisplayChannel(const NTV2Line21Channel inNewChannel)
Changes the caption channel I'm displaying.
I can decode captions from frames captured from an AJA device in real time. I can optionally play the...
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
virtual void Switch608Source(void)
Switches/rotates –608src.
static std::string OutputModeToString(const OutputMode inMode)
static AJAPnp gPlugAndPlay
virtual AJAStatus Install(AJAPnpCallback pInCallback, void *inRefCon=NULL, uint32_t inDeviceMask=0xFFFFFFFF)
Installs the given plug & play notification callback function, replacing any callback function that m...
bool fUseVanc
If true, use Vanc, even if the device supports Anc insertion.
static NTV2TCIndex GetTCIndexFromString(const std::string &inStr, const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDevSpec=std::string())
Returns the NTV2TCIndex that matches the given string.
static NTV2InputSource GetInputSourceFromString(const std::string &inStr, const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2InputSource that matches the given string.
static CaptionDataSrc StringToCaptionDataSrc(const std::string &inDataSrcStr)
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
bool fBurnCaptions
If true, burn-in captions on 2nd channel.
unsigned GetNumSourceSpecs(void) const
NTV2InputSource fInputSource
The device input connector to use.
virtual void ToggleVANC(void)
Toggles the use of VANC. (Debug, experimental)
bool fWithAudio
If true, also capture Audio.