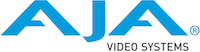 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
7 #ifndef NTV2BAREMETALPUBLICINTERFACE_H
8 #define NTV2BAREMETALPUBLICINTERFACE_H
14 #define NTV2_DEVICE_TYPE 0xBB
16 #define NTV2_BAREMETAL_DRIVER_VERSION NTV2DriverVersionEncodedBuildType | NTV2DriverVersionEncode(AJA_NTV2_SDK_VERSION_MAJOR, AJA_NTV2_SDK_VERSION_MINOR, AJA_NTV2_SDK_VERSION_POINT, AJA_NTV2_SDK_BUILD_NUMBER)
22 #define IOCTL_NTV2_WRITE_REGISTER \
23 _IOW(NTV2_DEVICE_TYPE, 48, REGISTER_ACCESS)
27 #define IOCTL_NTV2_READ_REGISTER \
28 _IOWR(NTV2_DEVICE_TYPE, 49 , REGISTER_ACCESS)
31 #define IOCTL_NTV2_DMA_READ_FRAME \
32 _IOW(NTV2_DEVICE_TYPE, 146,NTV2_DMA_CONTROL_STRUCT)
34 #define IOCTL_NTV2_DMA_WRITE_FRAME \
35 _IOW(NTV2_DEVICE_TYPE, 147,NTV2_DMA_CONTROL_STRUCT)
37 #define IOCTL_NTV2_DMA_READ_FRAME_SEGMENT \
38 _IOW(NTV2_DEVICE_TYPE, 148,NTV2_DMA_SEGMENT_CONTROL_STRUCT)
40 #define IOCTL_NTV2_DMA_WRITE_FRAME_SEGMENT \
41 _IOW(NTV2_DEVICE_TYPE, 149,NTV2_DMA_SEGMENT_CONTROL_STRUCT)
44 #define IOCTL_NTV2_DMA_READ \
45 _IOW(NTV2_DEVICE_TYPE, 175,NTV2_DMA_CONTROL_STRUCT)
47 #define IOCTL_NTV2_DMA_WRITE \
48 _IOW(NTV2_DEVICE_TYPE, 176,NTV2_DMA_CONTROL_STRUCT)
50 #define IOCTL_NTV2_DMA_READ_SEGMENT \
51 _IOW(NTV2_DEVICE_TYPE, 177,NTV2_DMA_SEGMENT_CONTROL_STRUCT)
53 #define IOCTL_NTV2_DMA_WRITE_SEGMENT \
54 _IOW(NTV2_DEVICE_TYPE, 178,NTV2_DMA_SEGMENT_CONTROL_STRUCT)
56 #define IOCTL_NTV2_DMA_P2P \
57 _IOW(NTV2_DEVICE_TYPE, 179,NTV2_DMA_P2P_CONTROL_STRUCT)
62 #define IOCTL_NTV2_INTERRUPT_CONTROL \
63 _IOW(NTV2_DEVICE_TYPE, 220, NTV2_INTERRUPT_CONTROL_STRUCT)
67 #define IOCTL_NTV2_WAITFOR_INTERRUPT \
68 _IOW(NTV2_DEVICE_TYPE, 221, NTV2_WAITFOR_INTERRUPT_STRUCT)
72 #define IOCTL_NTV2_CONTROL_DRIVER_DEBUG_MESSAGES \
73 _IOW(NTV2_DEVICE_TYPE, 230, NTV2_CONTROL_DRIVER_DEBUG_MESSAGES_STRUCT)
77 #define IOCTL_NTV2_SETUP_BOARD \
78 _IO(NTV2_DEVICE_TYPE, 231)
81 #define IOCTL_NTV2_RESTORE_HARDWARE_PROCAMP_REGISTERS \
82 _IO(NTV2_DEVICE_TYPE, 232)
88 #define IOCTL_NTV2_SET_BITFILE_INFO \
89 _IOWR(NTV2_DEVICE_TYPE, 240, BITFILE_INFO_STRUCT)
91 #define IOCTL_NTV2_GET_BITFILE_INFO \
92 _IOWR(NTV2_DEVICE_TYPE, 241, BITFILE_INFO_STRUCT)
98 #define IOCTL_NTV2_AUTOCIRCULATE_CONTROL \
99 _IOW(NTV2_DEVICE_TYPE, 250, AUTOCIRCULATE_DATA)
101 #define IOCTL_NTV2_AUTOCIRCULATE_STATUS \
102 _IOWR(NTV2_DEVICE_TYPE, 251, AUTOCIRCULATE_STATUS_STRUCT)
104 #define IOCTL_NTV2_AUTOCIRCULATE_FRAMESTAMP \
105 _IOWR(NTV2_DEVICE_TYPE, 252, AUTOCIRCULATE_FRAME_STAMP_COMBO_STRUCT)
107 #define IOCTL_NTV2_AUTOCIRCULATE_TRANSFER \
108 _IOWR(NTV2_DEVICE_TYPE, 253, AUTOCIRCULATE_TRANSFER_COMBO_STRUCT)
110 #define IOCTL_NTV2_AUTOCIRCULATE_CAPTURETASK \
111 _IOWR(NTV2_DEVICE_TYPE, 254, AUTOCIRCULATE_FRAME_STAMP_COMBO_STRUCT)
113 #define IOCTL_AJANTV2_MESSAGE \
114 _IOWR(NTV2_DEVICE_TYPE, 255, AUTOCIRCULATE_STATUS)
119 #define IOCTL_NTV2_WRITE_UART_TX \
120 _IOWR(NTV2_DEVICE_TYPE, 201, NTV2_UART_STRUCT)
123 #define IOCTL_NTV2_READ_UART_RX \
124 _IOWR(NTV2_DEVICE_TYPE, 202, NTV2_UART_STRUCT)
248 #define ntv2DMADriverbuffer(n) (n)
251 #define IOCTL_HEVC_MESSAGE \
252 _IOWR(NTV2_DEVICE_TYPE, 120, unsigned long)
INTERRUPT_ENUMS eInterruptType
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
INTERRUPT_ENUMS eInterruptType
NTV2_DriverDebugMessageSet
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
ULWord64 ullVideoBusAddress
Declares enums for virtual registers used in all platform drivers and the SDK.
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
NTV2_DriverDebugMessageSet msgSet
ULWord videoSegmentHostPitch
AUTOCIRCULATE_TASK_STRUCT acTask
ULWord ulVidSegmentCardPitch
ULWord ulVidSegmentHostPitch
Enumerations for controlling NTV2 devices.
ULWord videoSegmentCardPitch
ULWord64 ullMessageBusAddress
AUTOCIRCULATE_TRANSFER_STATUS_STRUCT acStatus
FRAME_STAMP_STRUCT acFrameStamp
NTV2Crosspoint channelSpec
NTV2RoutingTable acXena2RoutingTable
Declares enums and structs used by all platform drivers and the SDK.
NTV2Crosspoint
Logically, these are an NTV2Channel combined with an NTV2Mode.
AUTOCIRCULATE_TASK_STRUCT acTask
AUTOCIRCULATE_TRANSFER_STRUCT acTransfer