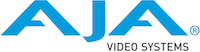 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
158 cout <<
"M31 Presets" << endl;
162 if (!presetStr.empty())
163 cout << ndx <<
": " << presetStr << endl;
170 cout <<
"M31 Formats" << endl;
174 if (!pixFormatStr.empty())
175 cout << ndx <<
": " << pixFormatStr << endl;
181 int main (
int argc,
const char ** argv)
195 UWord audioChannels (0);
203 {
"audio",
'a',
POPT_ARG_SHORT, &audioChannels, 0,
"number of audio channels",
"1-16"},
204 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model"},
205 {
"channel",
'c',
POPT_ARG_INT, &channelNumber, 0,
"channel to use",
"1-4"},
206 {
"format",
'f',
POPT_ARG_INT, &codecFormat, 0,
"format to use",
"use '-lf' for list"},
207 {
"preset",
'p',
POPT_ARG_INT, &codecPreset, 0,
"codec preset to use",
"use '-lp' for list"},
208 {
"quad",
'q',
POPT_ARG_NONE, &codecQuad, 0,
"interpret 4 inputs as UHD",
""},
209 {
"list",
'l',
POPT_ARG_STRING, &pWhatToList, 0,
"list options",
"p or f (presets or formats)"},
210 {
"tcb",
'b',
POPT_ARG_NONE, &timeCode, 0,
"add timecode burn to video",
""},
211 {
"tsi",
't',
POPT_ARG_NONE, &tsiData, 0,
"two sample interleave mode",
""},
212 {
"info",
'i',
POPT_ARG_NONE, &infoData, 0,
"write encoded info file",
""},
224 if (pWhatToList &&
string(pWhatToList) ==
"p")
226 else if (pWhatToList &&
string(pWhatToList) ==
"f")
228 else if (pWhatToList)
229 {cerr <<
"## ERROR: Invalid argument to --list option, expected 'p' or 'f'" << endl;
return 2;}
232 {cerr <<
"## ERROR: Invalid M31 format " << codecFormat <<
" -- expected 0 thru " << (
gNumCodecFormats) << endl;
return 2;}
238 {cerr <<
"## ERROR: Invalid M31 preset " << codecPreset <<
" -- expected 0 thru " << (
gNumCodecPresets) << endl;
return 2;}
245 {cerr <<
"## ERROR: Invalid channel number " << channelNumber <<
" -- expected 1 thru 4" << endl;
return 2;}
254 const uint32_t hevcMaxFrames (maxFrames >=
kNoSelection ? 0xffffffff : uint32_t(maxFrames));
255 NTV2EncodeHEVC hevcEncoder (pDeviceSpec ?
string (pDeviceSpec) :
"0", inputChannel,
256 m31Preset, pixelFormat, codecQuad ?
true :
false,
257 audioChannels, timeCode ?
true :
false,
258 infoData ?
true :
false, tsiData ?
true :
false, hevcMaxFrames);
262 {cerr <<
"## ERROR: Initialization failed" << endl;
return 1;}
271 cout <<
" Capture Capture" << endl
272 <<
" Frames Frames Buffer" << endl
273 <<
"Processed Dropped Level" << endl;
static const size_t gNumCodecFormats(sizeof(kCodecFormat)/sizeof(NTV2FrameBufferFormat))
@ M31_FILE_3840X2160_420_8_5994p
@ M31_FILE_1280X720_420_8_60p
@ M31_FILE_1920X1080_422_10_24p
@ M31_FILE_1920X1080_420_8_50i
@ NTV2_FBF_NUMFRAMEBUFFERFORMATS
@ M31_FILE_2048X1080_422_10_50p
NTV2Channel GetNTV2ChannelForIndex(const ULWord inIndex0)
@ NTV2_FBF_10BIT_YCBCR_420PL2
10-Bit 4:2:0 2-Plane YCbCr
@ M31_VIF_3840X2160_422_10_50p
@ M31_FILE_3840X2160_420_8_60p
@ M31_FILE_3840X2160_420_8_50p
@ M31_VIF_3840X2160_420_10_50p
@ M31_FILE_3840X2160_422_8_2997p
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
static const size_t gNumCodecPresets(sizeof(kCodecPreset)/sizeof(M31VideoPreset))
@ M31_FILE_3840X2160_420_8_2997p
@ M31_FILE_4096X2160_422_10_50p
@ M31_FILE_1920X1080_422_10_2398p
@ M31_FILE_1920X1080_422_10_60p
@ M31_FILE_3840X2160_422_8_5994p
@ M31_FILE_3840X2160_422_10_5994p
@ M31_FILE_4096X2160_420_10_5994p
@ M31_FILE_2048X1080_422_10_5994p
Declares the AJATime class.
@ M31_FILE_3840X2160_422_8_25p
void SignalHandler(int inSignal)
static int printFormats(void)
@ M31_VIF_3840X2160_422_10_5994p
@ M31_FILE_3840X2160_420_8_30p
@ M31_FILE_1920X1080_420_8_25p
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
@ M31_VIF_3840X2160_420_8_50p
@ M31_VIF_1280X720_420_8_5994p
int main(int argc, const char **argv)
@ M31_VIF_3840X2160_420_10_5994p
@ M31_FILE_2048X1080_420_8_60p
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
@ M31_VIF_3840X2160_420_8_5994p
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
@ M31_FILE_1920X1080_420_8_2398p
@ M31_FILE_3840X2160_420_10_60p
static bool gGlobalQuit((0))
@ M31_FILE_2048X1080_420_8_25p
@ M31_FILE_1920X1080_422_10_50i
@ NTV2_CHANNEL4
Specifies channel or Frame Store 4 (or the 4th item).
@ M31_FILE_3840X2160_422_10_25p
@ M31_FILE_3840X2160_422_10_30p
@ NTV2_FBF_8BIT_YCBCR_420PL2
8-Bit 4:2:0 2-Plane YCbCr
@ M31_FILE_3840X2160_420_8_24p
@ M31_VIF_1920X1080_420_8_50p
static int printPresets(void)
@ M31_FILE_3840X2160_422_10_2398p
@ M31_FILE_1920X1080_420_8_60p
@ M31_VIF_1280X720_422_10_60p
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
@ M31_FILE_2048X1080_422_10_60p
@ M31_VIF_3840X2160_420_10_60p
@ M31_FILE_1280X720_422_10_5994p
@ M31_VIF_1280X720_420_8_60p
@ M31_FILE_1920X1080_420_8_5994p
@ M31_FILE_1920X1080_422_10_30p
std::string NTV2M31VideoPresetToString(const M31VideoPreset inValue, const bool inForRetailDisplay=false)
@ M31_FILE_1920X1080_420_8_30p
@ M31_VIF_1920X1080_420_10_60p
@ M31_FILE_3840X2160_420_10_50p
@ M31_VIF_1280X720_420_8_50p
Declares structs used for the Corvid HEVC.
Declares numerous NTV2 utility functions.
virtual AJAStatus Run(void)
Runs me.
poptContext poptFreeContext(poptContext con)
@ M31_FILE_3840X2160_422_8_2398p
@ M31_FILE_1920X1080_422_10_25p
@ M31_VIF_3840X2160_422_10_60p
@ M31_FILE_3840X2160_422_10_50p
@ M31_FILE_3840X2160_420_8_2398p
@ M31_FILE_3840X2160_422_8_60p
@ M31_FILE_4096X2160_422_10_60p_IF
@ M31_FILE_1280X720_420_8_5994p
@ NTV2_FBF_10BIT_YCBCR_422PL2
10-Bit 4:2:2 2-Plane YCbCr
@ M31_FILE_1920X1080_420_8_2997p
@ M31_FILE_2048X1080_420_8_50p
@ M31_FILE_3840X2160_422_8_50p
#define POPT_ARGFLAG_OPTIONAL
@ M31_FILE_2048X1080_420_8_2398p
@ M31_VIF_1280X720_422_10_50p
static AJAStatus Open(bool incrementRefCount=false)
#define NTV2_IS_VALID_CHANNEL(__x__)
@ M31_FILE_1920X1080_420_8_24p
@ M31_FILE_1920X1080_420_8_50p
@ M31_FILE_3840X2160_422_10_24p
@ M31_VIF_1920X1080_422_10_5994p
@ M31_FILE_4096X2160_420_10_60p
poptContext poptGetContext(const char *name, int argc, const char **argv, const struct poptOption *options, unsigned int flags)
@ M31_FILE_1280X720_422_10_50p
@ M31_FILE_3840X2160_422_8_30p
@ M31_FILE_1920X1080_422_10_50p
int poptGetNextOpt(poptContext con)
static const size_t kNoSelection(1000000000)
@ M31_FILE_2048X1080_420_8_30p
std::string NTV2FrameBufferFormatToString(const NTV2FrameBufferFormat inValue, const bool inForRetailDisplay=false)
@ M31_FILE_4096X2160_422_10_5994p_IF
static const NTV2FrameBufferFormat kCodecFormat[]
@ M31_FILE_2048X1080_420_8_2997p
@ M31_FILE_2048X1080_422_10_30p
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
virtual void Quit(void)
Gracefully stops me from running.
@ M31_FILE_1280X720_422_10_60p
virtual void GetStatus(AVHevcStatus &outStatus)
Provides status information about my input (capture) process.
virtual M31VideoPreset GetCodecPreset(void)
Get the codec preset.
@ NTV2_FBF_8BIT_YCBCR_422PL2
8-Bit 4:2:2 2-Plane YCbCr
@ M31_FILE_1920X1080_422_10_2997p
@ M31_VIF_1280X720_422_10_5994p
@ M31_FILE_3840X2160_422_10_2997p
@ M31_FILE_1920X1080_422_10_5994i
@ M31_FILE_3840X2160_422_10_60p
@ M31_FILE_2048X1080_422_10_2398p
@ M31_FILE_3840X2160_422_8_24p
@ M31_FILE_2048X1080_422_10_2997p
@ M31_VIF_1920X1080_420_8_5994p
@ M31_FILE_2048X1080_422_10_25p
static const M31VideoPreset kCodecPreset[]
@ M31_FILE_1280X720_420_8_50p
#define AJA_FAILURE(_status_)
@ M31_FILE_1920X1080_422_10_5994p
@ M31_FILE_2048X1080_422_10_24p
@ M31_FILE_1920X1080_420_8_5994i
@ M31_FILE_3840X2160_420_8_25p
Declares the NTV2EncodeHEVC class.
@ M31_VIF_1920X1080_422_10_60p
@ M31_FILE_2048X1080_420_8_24p
@ M31_FILE_3840X2160_420_10_5994p
@ M31_VIF_3840X2160_420_8_60p
@ M31_FILE_2048X1080_420_8_5994p