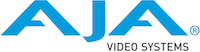 |
AJA NTV2 SDK
17.1.3.1410
NTV2 SDK 17.1.3.1410
|
Go to the documentation of this file.
112 uint8_t onesCount = 0;
113 uint8_t shiftChar = inChar;
116 for (uint8_t i = 0; i < 7; i++)
118 if ((shiftChar & 0x01) != 0)
121 shiftChar = shiftChar >> 1;
125 if ((onesCount % 2) == 1)
126 return (inChar & 0x7F);
128 return (inChar | 0x80);
152 const uint8_t char1 (
m_char1 & 0x7F);
153 const uint8_t char2 (
m_char2 & 0x7F);
157 if (
IsRaw() && GetDataLocation().GetLineNumber())
158 oss << (GetDataLocation().GetLineNumber() > 264 ?
" F2" :
" F1");
160 if (char1 >= 0x20 && char1 < 0x7F)
161 oss <<
" ('" << char1 <<
"')";
163 if (char2 >= 0x20 && char2 < 0x7F)
164 oss <<
" ('" << char2 <<
"')";
virtual AJAStatus SetCEA608Bytes(const uint8_t inByte1, const uint8_t inByte2)
Set the CEA608 payload bytes.
virtual std::ostream & Print(std::ostream &inOutStream, const bool inDetailed=false) const
Streams a human-readable representation of me to the given output stream.
virtual AJAStatus GetCEA608Bytes(uint8_t &outByte1, uint8_t &outByte2, bool &outIsValid) const
Answers with the CEA608 payload bytes.
@ AJAAncDataType_Unknown
Includes data that is valid, but we don't recognize.
virtual void Clear(void)
Frees my allocated memory, if any, and resets my members to their default values.
AJAAncDataType
Identifies the ancillary data types that are known to this module.
static AJAAncDataType RecognizeThisAncillaryData(const AJAAncillaryData *pInAncData)
virtual std::ostream & Print(std::ostream &inOutStream, const bool inDetailed=false) const
Streams a human-readable representation of me to the given output stream.
This is the base class for handling CEA-608 caption data packets.
AJAAncillaryData_Cea608()
My default constructor.
I am the principal class that stores a single SMPTE-291 SDI ancillary data packet OR the digitized co...
Declares the AJAAncillaryData_Cea608 class.
static uint8_t AddOddParity(const uint8_t inValue)
Set/Clear bit 7 of a byte to make odd parity.
bool m_rcvDataValid
This is set true (or not) by ParsePayloadData()
virtual AJAStatus GetCEA608Characters(uint8_t &outChar1, uint8_t &outChar2, bool &outIsValid) const
Answers with the CEA608 payload characters.
virtual void Clear(void)
Frees my allocated memory, if any, and resets my members to their default values.
virtual ~AJAAncillaryData_Cea608()
My destructor.
AJAAncillaryData & operator=(const AJAAncillaryData &inRHS)
virtual AJAStatus SetCEA608Characters(const uint8_t inChar1, const uint8_t inChar2)
Sets the CEA608 payload characters. Uses the least significant 7 bits of the input values and adds od...
bool IsRaw(const NTV2FrameBufferFormat format)
#define xHEX0N(__x__, __n__)
AJAAncillaryData_Cea608 & operator=(const AJAAncillaryData_Cea608 &inRHS)
Assignment operator – replaces my contents with the right-hand-side value.