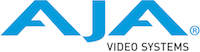 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
17 static const double kTwo24th (
double(1 << 24));
28 return double(inCoefficient) /
kTwo24th;
34 switch (inPixelFormat)
39 mInputPixelFormat = inPixelFormat;
50 switch (inPixelFormat)
55 mOutputPixelFormat = inPixelFormat;
66 switch (inChromaFilterSelect)
71 mChromaFilterSelect = inChromaFilterSelect;
82 switch (inChromaEdgeControl)
86 mChromaEdgeControl = inChromaEdgeControl;
101 mKeySource = inKeySource;
112 switch (inKeyOutputRange)
116 mKeyOutputRange = inKeyOutputRange;
128 mKeyInputOffset = inKeyInputOffset;
136 mKeyOutputOffset = inKeyOutputOffset;
144 mKeyGain = inKeyGain;
153 if (!inDevice.IsOpen ())
187 cscRegs [15] = (
ULWord(mKeyOutputOffset) << 16) |
ULWord(mKeyInputOffset);
188 cscRegs [16] = (
ULWord(mKeyGain * 4096.0));
194 regs.push_back(
NTV2RegInfo(cscBaseRegNum+num, cscRegs[num]));
205 if (!inDevice.IsOpen ())
251 mKeyInputOffset = int16_t(regs[15].registerValue & 0xFFFF);
252 mKeyOutputOffset= uint16_t(regs[15].registerValue >> 16);
253 mKeyGain = (double(regs[16].registerValue) / 4096.0);
NTV2EnhancedCSCKeyOutputRange
@ NTV2_Enhanced_CSC_Pixel_Format_YCbCr444
bool SetKeyInputOffset(const int16_t inKeyInputOffset)
virtual bool ReadRegisters(NTV2RegisterReads &inOutValues)
Reads the register(s) specified by the given NTV2RegInfo sequence.
static double ConvertCoeffULWordToDouble(const ULWord inCoefficient)
bool SendToHardware(CNTV2Card &inDevice, const NTV2Channel inChannel) const
@ NTV2CSCCoeffIndex_A0
Proportion of input component 0 applied to A output.
@ NTV2_Enhanced_CSC_Key_Output_Range_Full
@ NTV2CSCOffsetIndex_Pre1
Declares device capability functions.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
@ kK2RegShiftEnhancedCSCKeyOutputRange
bool NTV2DeviceCanDoEnhancedCSC(const NTV2DeviceID inDeviceID)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
@ NTV2CSCOffsetIndex_PostC
@ NTV2_Enhanced_CSC_Chroma_Filter_Select_Full
@ kK2RegShiftEnhancedCSCChromaFilterSelect
NTV2EnhancedCSCChromaEdgeControl
bool SetChromaFilterSelect(const NTV2EnhancedCSCChromaFilterSelect inChromaFilterSelect)
bool SetInputPixelFormat(const NTV2EnhancedCSCPixelFormat inPixelFormat)
@ kK2RegMaskEnhancedCSCKeyOutputRange
UWord NTV2DeviceGetNumCSCs(const NTV2DeviceID inDeviceID)
@ NTV2CSCCoeffIndex_C0
Proportion of input component 0 applied to C output.
@ kK2RegMaskEnhancedCSCChromaFilterSelect
@ kK2RegShiftEnhancedCSCOutputPixelFormat
std::vector< NTV2RegInfo > NTV2RegWrites
An ordered sequence of zero or more NTV2RegInfo structs intended for WriteRegister.
virtual bool WriteRegisters(const NTV2RegisterWrites &inRegWrites)
Writes the given sequence of NTV2RegInfo's.
@ NTV2CSCOffsetIndex_PostA
virtual void SetOffset(const NTV2CSCOffsetIndex inOffsetIndex, const int16_t inOffset)
Changes the specified offset to the given value.
bool SetKeyOutputRange(const NTV2EnhancedCSCKeyOutputRange inKeyOutputRange)
bool SetChromaEdgeControl(const NTV2EnhancedCSCChromaEdgeControl inChromaEdgeControl)
static const ULWord gChannelToEnhancedCSCRegNum[]
bool GetFromHardware(CNTV2Card &inDevice, const NTV2Channel inChannel)
@ NTV2_Enhanced_CSC_Chroma_Edge_Control_Extended
@ NTV2_Enhanced_CSC_Chroma_Filter_Select_None
Declares the CNTV2EnhancedCSC class.
@ NTV2_Enhanced_CSC_Pixel_Format_YCbCr422
@ NTV2_Enhanced_CSC_Chroma_Edge_Control_Black
@ NTV2CSCCoeffIndex_B0
Proportion of input component 0 applied to B output.
@ NTV2_Enhanced_CSC_Key_Output_Range_SMPTE
static const double kTwo24th(double(1<< 24))
NTV2EnhancedCSCPixelFormat
@ NTV2_Enhanced_CSC_Pixel_Format_RGB444
Declares numerous NTV2 utility functions.
@ NTV2CSCCoeffIndex_C2
Proportion of input component 2 applied to C output.
I interrogate and control an AJA video/audio capture/playout device.
virtual void SetCoefficient(const NTV2CSCCoeffIndex inCoeffIndex, const double inCoefficient)
Changes the specified matrix coefficient to the given value.
@ kK2RegShiftEnhancedCSCInputPixelFormat
@ NTV2CSCCoeffIndex_C1
Proportion of input component 1 applied to C output.
@ NTV2_Enhanced_CSC_Key_Source_Key_Input
bool SetOutputPixelFormat(const NTV2EnhancedCSCPixelFormat inPixelFormat)
@ NTV2_Enhanced_CSC_Key_Spurce_A0_Input
@ NTV2CSCOffsetIndex_Pre0
@ kK2RegMaskEnhancedCSCKeySource
CNTV2CSCMatrix & Matrix(void)
@ NTV2CSCOffsetIndex_Pre2
@ NTV2_Enhanced_CSC_Chroma_Filter_Select_Simple
bool SetKeyOutputOffset(const uint16_t inKeyOutputOffset)
static ULWord ConvertCoeffDoubleToULWord(const double inCoefficient)
@ kK2RegMaskEnhancedCSCChromaEdgeControl
@ kRegNumEnhancedCSCRegisters
virtual NTV2DeviceID GetDeviceID(void)
NTV2EnhancedCSCChromaFilterSelect
@ kK2RegShiftEnhancedCSCChromaEdgeControl
@ kK2RegShiftEnhancedCSCKeySource
NTV2RegWrites NTV2RegReads
An ordered sequence of zero or more NTV2RegInfo structs intended for ReadRegister.
bool SetKeyGain(const double inKeyGain)
Declares enums and structs used by all platform drivers and the SDK.
@ kK2RegMaskEnhancedCSCInputPixelFormat
@ NTV2CSCCoeffIndex_B2
Proportion of input component 2 applied to B output.
bool SetKeySource(const NTV2EnhancedCSCKeySource inKeySource)
@ NTV2CSCCoeffIndex_A2
Proportion of input component 2 applied to A output.
@ kK2RegMaskEnhancedCSCOutputPixelFormat
@ NTV2CSCCoeffIndex_A1
Proportion of input component 1 applied to A output.
Everything needed to call CNTV2Card::ReadRegister or CNTV2Card::WriteRegister functions.
@ NTV2CSCCoeffIndex_B1
Proportion of input component 1 applied to B output.
@ NTV2CSCOffsetIndex_PostB