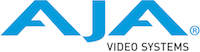 |
AJA NTV2 SDK
17.1.1.1245
NTV2 SDK 17.1.1.1245
|
Go to the documentation of this file.
11 static const double kPi(3.1415926536);
415 switch (inCoeffIndex)
428 NTV2_ASSERT (
false &&
"Unhandled coefficient index");
435 switch (inCoeffIndex)
453 switch (inOffsetIndex)
470 switch (inOffsetIndex)
489 mA0 = (pre.mA0 * current.mA0) + (pre.mB0 * current.mA1) + (pre.mC0 * current.mA2);
490 mA1 = (pre.mA1 * current.mA0) + (pre.mB1 * current.mA1) + (pre.mC1 * current.mA2);
491 mA2 = (pre.mA2 * current.mA0) + (pre.mB2 * current.mA1) + (pre.mC2 * current.mA2);
493 mB0 = (pre.mA0 * current.mB0) + (pre.mB0 * current.mB1) + (pre.mC0 * current.mB2);
494 mB1 = (pre.mA1 * current.mB0) + (pre.mB1 * current.mB1) + (pre.mC1 * current.mB2);
495 mB2 = (pre.mA2 * current.mB0) + (pre.mB2 * current.mB1) + (pre.mC2 * current.mB2);
497 mC0 = (pre.mA0 * current.mC0) + (pre.mB0 * current.mC1) + (pre.mC0 * current.mC2);
498 mC1 = (pre.mA1 * current.mC0) + (pre.mB1 * current.mC1) + (pre.mC1 * current.mC2);
499 mC2 = (pre.mA2 * current.mC0) + (pre.mB2 * current.mC1) + (pre.mC2 * current.mC2);
511 mA0 = (current.mA0 * post.mA0) + (current.mB0 * post.mA1) + (current.mC0 * post.mA2);
512 mA1 = (current.mA1 * post.mA0) + (current.mB1 * post.mA1) + (current.mC1 * post.mA2);
513 mA2 = (current.mA2 * post.mA0) + (current.mB2 * post.mA1) + (current.mC2 * post.mA2);
515 mB0 = (current.mA0 * post.mB0) + (current.mB0 * post.mB1) + (current.mC0 * post.mB2);
516 mB1 = (current.mA1 * post.mB0) + (current.mB1 * post.mB1) + (current.mC1 * post.mB2);
517 mB2 = (current.mA2 * post.mB0) + (current.mB2 * post.mB1) + (current.mC2 * post.mB2);
519 mC0 = (current.mA0 * post.mC0) + (current.mB0 * post.mC1) + (current.mC0 * post.mC2);
520 mC1 = (current.mA1 * post.mC0) + (current.mB1 * post.mC1) + (current.mC1 * post.mC2);
521 mC2 = (current.mA2 * post.mC0) + (current.mB2 * post.mC1) + (current.mC2 * post.mC2);
538 double rad =
kPi * inDegrees / 180.0;
549 mPreOffset0 = inOffset0;
550 mPreOffset1 = inOffset1;
551 mPreOffset2 = inOffset2;
558 mPreOffset0 += inOffset0;
559 mPreOffset1 += inOffset1;
560 mPreOffset2 += inOffset2;
567 mPostOffsetA = inOffsetA;
568 mPostOffsetB = inOffsetB;
569 mPostOffsetC = inOffsetC;
575 mPostOffsetA += inOffsetA;
576 mPostOffsetB += inOffsetB;
577 mPostOffsetC += inOffsetC;
586 if (mA0 != 1.0 || mA1 != 0.0 || mA2 != 0.0)
589 if (mB0 != 0.0 || mB1 != 1.0 || mB2 != 0.0)
592 if (mC0 != 0.0 || mC1 != 0.0 || mC2 != 1.0)
603 if ( (mA0 != rhs.mA0) || (mA1 != rhs.mA1) || (mA2 != rhs.mA2) )
606 if ( (mB0 != rhs.mB0) || (mB1 != rhs.mB1) || (mB2 != rhs.mB2) )
609 if ( (mC0 != rhs.mC0) || (mC1 != rhs.mC1) || (mC2 != rhs.mC2) )
612 if ( (mPreOffset0 != rhs.mPreOffset0) || (mPreOffset1 != rhs.mPreOffset1) || (mPreOffset2 != rhs.mPreOffset2) )
615 if ( (mPostOffsetA != rhs.mPostOffsetA) || (mPostOffsetB != rhs.mPostOffsetB) || (mPostOffsetC != rhs.mPostOffsetC) )
641 return ::fabs(inCoeff1 - inCoeff2) < inMaxDiff;
Declares the utility class for abstract color space matrix operations.
@ NTV2CSCCoeffIndex_A0
Proportion of input component 0 applied to A output.
@ NTV2CSCOffsetIndex_Pre1
#define NTV2_ASSERT(_expr_)
virtual void InitMatrix(const NTV2ColorSpaceMatrixType inPreset)
Replace the current matrix coefficients with a known set of coefficients.
@ NTV2CSCOffsetIndex_PostC
This class supports the color space conversion matrix described below.
virtual bool operator==(const CNTV2CSCMatrix &rhs) const
Returns true if the matrix coefficients and offsets EXACTLY match those of the specified instance.
@ NTV2_YCbCr_to_GBRFull_Rec2020_Matrix
virtual void SetHueRotate(const double inDegrees)
Set matrix for hue rotation.
@ NTV2_YCbCrRec601_to_YCbCrRec709_Matrix
@ NTV2_YCbCr_to_GBRSMPTE_Rec601_Matrix
@ NTV2CSCCoeffIndex_C0
Proportion of input component 0 applied to C output.
const int16_t NTV2_CSCMatrix_SMPTECOffset
virtual bool CoeffEqual(const double inCoeff1, const double inCoeff2, const double inMaxDiff)
Tests if two coefficients differ by less than the fiven tolerance.
@ NTV2_GBRSMPTE_to_YCbCr_Rec709_Matrix
@ NTV2CSCOffsetIndex_PostA
@ NTV2_YCbCr_to_GBRFull_Rec709_Matrix
virtual void SetOffset(const NTV2CSCOffsetIndex inOffsetIndex, const int16_t inOffset)
Changes the specified offset to the given value.
const int16_t NTV2_CSCMatrix_SMPTEYOffset
@ NTV2_GBRFull_to_GBRSMPTE_Matrix
CNTV2CSCMatrix(const NTV2ColorSpaceMatrixType inPreset=NTV2_Unity_Matrix)
Instantiates and initializes the instance with a known set of coefficients.
@ NTV2_GBRSMPTE_to_YCbCr_Rec2020_Matrix
const int16_t NTV2_CSCMatrix_ZeroOffset
@ NTV2CSCCoeffIndex_B0
Proportion of input component 0 applied to B output.
enum NTV2CSCCoeff NTV2CSCCoeffIndex
@ NTV2CSCCoeffIndex_C2
Proportion of input component 2 applied to C output.
virtual void PostMultiply(const CNTV2CSCMatrix &inPostInstance)
Post-multiply the current 3x3 matrix by the matrix of the given instance.
virtual double GetCoefficient(const NTV2CSCCoeffIndex inCoeffIndex) const
Returns the value of a requested matrix coefficient.
virtual void SetCoefficient(const NTV2CSCCoeffIndex inCoeffIndex, const double inCoefficient)
Changes the specified matrix coefficient to the given value.
virtual void SetGain(const double inGain0, const double inGain1, const double inGain2)
Set the matrix diagonals (gains).
@ NTV2CSCCoeffIndex_C1
Proportion of input component 1 applied to C output.
enum NTV2CSCOffset NTV2CSCOffsetIndex
virtual void AddPostOffsets(const int16_t inIncrementA, const int16_t inIncrementB, const int16_t inIncrementC)
Add post-offsets.
@ NTV2CSCOffsetIndex_Pre0
virtual int16_t GetOffset(const NTV2CSCOffsetIndex inOffsetIndex) const
Returns the value of a requested offset.
@ NTV2_GBRSMPTE_to_GBRFull_Matrix
@ NTV2_GBRFull_to_YCbCr_Rec2020_Matrix
virtual bool IsUnityMatrix(void)
Returns 'true' if this CSC Matrix has unity matrix coefficients.
@ NTV2_YCbCr_to_GBRFull_Rec601_Matrix
virtual bool IsEqual(const CNTV2CSCMatrix &inCSCMatrix, const double inMaxDiff=NTV2CSCMatrix_MaxCoeffDiff)
Tests if the matrix coefficients can all be considered equal to those of the specified instance,...
@ NTV2CSCOffsetIndex_Pre2
virtual void AddPreOffsets(const int16_t inIncrement0, const int16_t inIncrement1, const int16_t inIncrement2)
Add pre-offsets.
@ NTV2_YCbCrRec709_to_YCbCrRec601_Matrix
@ NTV2_GBRFull_to_YCbCr_Rec601_Matrix
@ NTV2_GBRFull_to_YCbCr_Rec709_Matrix
@ NTV2_YCbCr_to_GBRSMPTE_Rec2020_Matrix
static const double kPi(3.1415926536)
@ NTV2_YCbCr_to_GBRSMPTE_Rec709_Matrix
@ NTV2CSCCoeffIndex_B2
Proportion of input component 2 applied to B output.
@ NTV2CSCCoeffIndex_A2
Proportion of input component 2 applied to A output.
virtual void SetPreOffsets(const int16_t inOffset0, const int16_t inOffset1, const int16_t inOffset2)
Set pre-offsets.
@ NTV2CSCCoeffIndex_A1
Proportion of input component 1 applied to A output.
@ NTV2_GBRSMPTE_to_YCbCr_Rec601_Matrix
virtual void PreMultiply(const CNTV2CSCMatrix &inPreInstance)
Pre-multiply the current 3x3 matrix by the matrix of the given instance.
@ NTV2CSCCoeffIndex_B1
Proportion of input component 1 applied to B output.
@ NTV2CSCOffsetIndex_PostB
virtual void SetPostOffsets(const int16_t inOffsetA, const int16_t inOffsetB, const int16_t inOffsetC)
Set post-offsets.
@ NTV2_Unity_SMPTE_Matrix