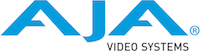 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
7 #ifndef __NTV2_CAPTION608MESSAGE__
8 #define __NTV2_CAPTION608MESSAGE__
43 static const UWord NTV2_CC608_CaptionMsgMaxBytes = 256;
66 virtual std::ostream &
Print (std::ostream & inOutStream)
const;
72 virtual inline bool HasData (
void)
const {
return GetLength () > 0;}
88 virtual UByte ReadNext (
void);
94 virtual inline void SkipNext (
void) {
if (mReadPosition < mLength) mReadPosition++;}
106 virtual inline bool IsPastEnd (
void)
const {
return GetReadPosition () >= GetLength ();}
116 virtual bool Add608Command (
const UWord inCommand);
124 virtual bool Add608String (
const std::string & inMessageStr);
132 virtual bool AddBytePair (
const UByte inByte1,
const UByte inByte2);
144 UByte mData [NTV2_CC608_CaptionMsgMaxBytes];
160 #endif // __NTV2_CAPTION608MESSAGE__
virtual bool IsData(void) const
Declares several data types used with 608/SD captioning.
virtual std::ostream & Print(std::ostream &inOutStream) const
Prints a human-readable representation of me to the given output stream.
I am a reference-counted pointer template class. I am intended to be a proxy for an underlying object...
virtual bool HasData(void) const
virtual UWord GetLength(void) const
AJARefPtr< CNTV2Caption608Message > CNTV2Caption608MessagePtr
virtual std::ostream & Print(std::ostream &inOutStream, const bool inDumpMessages=true) const
Prints a human-readable representation of me to the given output stream in oldest-to-newest order.
virtual bool IsDelay(void) const
I am a thread-safe queue of caption messages. Internally, I maintain two queues: one for high-priorit...
@ NTV2_CC608_CaptionMsgType_Data
virtual UWord GetReadPosition(void) const
Returns my internal read position.
virtual NTV2Line21Channel GetChannel(void) const
CNTV2Caption608MessagePtr NTV2CaptionMessagePtr
#define IsLine21CaptionChannel(_chan_)
@ NTV2_CC608_CaptionMsgType_Delay
CNTV2Caption608MessagePtr CNTV2608MsgPtr
virtual NTV2_CC608_CaptionMessageType GetType(void) const
virtual bool IsPastEnd(void) const
Returns "true" if my internal read position is past the end; otherwise returns "false".
virtual bool IsHighPriority(void) const
NTV2_CC608_CaptionMessageType
virtual UWord GetRemainingByteCapacity(void) const
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
std::ostream & operator<<(std::ostream &inOutStream, const CNTV2Caption608Message &inMsg)
virtual UWord GetBytesRemaining(void) const
virtual bool IsLowPriority(void) const
CNTV2Caption608Message NTV2CaptionMessage
Defines the AJARefPtr template class.
Declares the NTV2CaptionLogMask, and the CNTV2CaptionLogConfig class.
virtual void SkipNext(void)
Increments my internal read position (if not already past end).
virtual ~CNTV2Caption608Message()