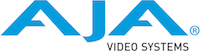 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
7 #ifndef __NTV2_CEA608_ENCODER_
8 #define __NTV2_CEA608_ENCODER_
95 virtual bool EnqueuePopOnMessage (
const std::string & inMessageStr,
97 const UWord inRowNumber = 0,
98 const UWord inColumnNumber = 0,
124 virtual bool EnqueuePaintOnMessage (
const std::string & inMessageStr,
125 const bool inEraseFirst =
false,
127 const UWord inRowNumber = 0,
128 const UWord inColumnNumber = 0,
156 virtual bool EnqueueRollUpMessage (
const std::string & inMessageStr,
176 virtual bool EnqueueTextMessage (
const std::string & inMessageStr,
177 const bool inEraseFirst =
false,
190 virtual bool EnqueueCaptionData (
const CaptionData & inCaptionData);
219 virtual bool EncodeNextCaptionBytesIntoLine21 (
void * pFrameBuffer,
230 virtual bool GetNextCaptionData (
CaptionData & outCaptionData);
295 virtual void FlushChannel (
const NTV2Line21Channel inChannel,
const bool inAlsoInProgress =
true);
314 virtual void GetQueueInfoForChannel (
const NTV2Line21Channel inChannel,
size_t & outBytesQueued,
size_t & outMessagesQueued)
const;
323 virtual inline size_t GetEnqueueByteTally (
void)
const {
return mXmitMsgQueueF1.GetEnqueueByteTally () + mXmitMsgQueueF2.GetEnqueueByteTally ();}
329 virtual inline size_t GetDequeueByteTally (
void)
const {
return mXmitMsgQueueF1.GetDequeueByteTally () + mXmitMsgQueueF2.GetDequeueByteTally ();}
334 virtual inline size_t GetEnqueueMessageTally (
void)
const {
return mXmitMsgQueueF1.GetEnqueueMessageTally () + mXmitMsgQueueF2.GetEnqueueMessageTally ();}
339 virtual inline size_t GetDequeueMessageTally (
void)
const {
return mXmitMsgQueueF1.GetDequeueMessageTally () + mXmitMsgQueueF2.GetDequeueMessageTally ();}
345 virtual inline size_t GetHighestQueueDepth (
void)
const {
return mXmitMsgQueueF1.GetHighestQueueDepth () + mXmitMsgQueueF2.GetHighestQueueDepth ();}
357 size_t & outEnqueueBytes,
size_t & outEnqueueMsgs,
358 size_t & outDequeueBytes,
size_t & outDequeueMsgs)
const;
368 #if !defined(NTV2_DEPRECATE_17_0)
370 virtual inline bool EnqueuePaintOnMessage (
const std::string & str,
const bool erase,
const NTV2Line21Channel ch,
const UWord row,
const UWord col,
const NTV2Line21AttrsPtr pAttrs) {
NTV2Line21Attrs tmp;
return EnqueuePaintOnMessage(str, erase, ch, row, col, pAttrs ? *pAttrs : tmp);}
371 virtual inline bool EnqueueRollUpMessage (
const std::string & str,
const NTV2Line21Mode mode,
const NTV2Line21Channel ch,
const UWord row,
const UWord col,
const NTV2Line21AttrsPtr pAttrs) {
NTV2Line21Attrs tmp;
return EnqueueRollUpMessage(str, mode, ch, row, col, pAttrs ? *pAttrs : tmp);}
372 #endif // defined(NTV2_DEPRECATE_17_0)
381 const UWord inRowNumber,
382 const UWord inColumnNumber,
387 const UWord inRowNumber,
388 const UWord inColumnNumber,
390 UWord & outExtraColumn);
393 const UWord inRowNumber,
412 static UByte CC608OddParity (
const UByte inByte);
464 static size_t Utf8LengthInChars (
const std::string & inUtf8Str);
474 static std::string Utf8GetCharacter (
const std::string & inUtf8Str,
const size_t inCharOffset);
478 #endif // __NTV2_CEA608_ENCODER_
virtual size_t GetDequeueMessageTally(void) const
Returns the total number of messages that have been dequeued from me since I was instantiated.
Declares several data types used with 608/SD captioning.
const UWord NTV2_CC608_MinCol(1)
The minimum column index number (located at the left edge of the screen).
virtual size_t GetEnqueueMessageTally(void) const
Returns the total number of messages that have been enqueued onto me since I was instantiated.
Declares the CNTV2Line21Captioner class.
NTV2FrameBufferFormat
Identifies a particular video frame buffer format. See Device Frame Buffer Formats for details.
virtual size_t GetDequeueByteTally(void) const
Returns the total number of bytes (including command bytes) that have been dequeued from me since I w...
virtual size_t GetHighestQueueDepth(void) const
Returns the highest queue depth – i.e., the maximum number of messages I held in either of my queues ...
A small collection of UTF-8 utility functions.
I am a thread-safe queue of caption messages. Internally, I maintain two queues: one for high-priorit...
virtual NTV2CaptionLogMask SetLogMask(const NTV2CaptionLogMask inLogMask)
Specifies what, if any, debug information I will write to my log stream.
@ NTV2_CC608_CapModeRollUp4
4-row roll-up from bottom
virtual size_t GetEnqueueByteTally(void) const
Returns the total number of bytes (including command bytes) that have been enqueued onto me since I w...
NTV2Line21Mode
The CEA-608 modes: pop-on, roll-up (2, 3 and 4-line), and paint-on.
@ NTV2_CC608_CC1
Caption channel 1, the primary caption channel.
NTV2Line21Channel
The CEA-608 caption channels: CC1 thru CC4, TX1 thru TX4, plus XDS.
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
struct NTV2Line21Attributes NTV2Line21Attrs
uint64_t NTV2CaptionLogMask
Selectors to control what information is logged.
NTV2Line21Field
The two CEA-608 interlace fields.
CEA-608 Character Attributes.
This structure encapsulates all possible CEA-608 caption data bytes that may be associated with a giv...
Instances of me can encode two ASCII characters into a "line 21" closed-captioning waveform....
Defines the AJARefPtr template class.
AJARefPtr< CNTV2CaptionEncoder608 > CNTV2CaptionEncoder608Ptr
Declares the CNTV2Caption608MessageQueue class.
const UWord NTV2_CC608_MaxRow(15)
The maximum permissible row index number (located at the bottom of the screen).