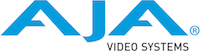 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
36 int main (
int argc,
const char ** argv)
42 int doMultiFormat (0);
53 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
55 {
"pixelFormat",
'p',
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list" },
56 {
"input",
'i',
POPT_ARG_STRING, &pInputSrcSpec, 0,
"SDI input to use",
"1-8, ?=list" },
60 {
"tcsource",
't',
POPT_ARG_STRING, &pTcSource, 0,
"time code source",
"'?' to list" },
66 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
68 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
72 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
81 if (inputSourceStr ==
"?" || inputSourceStr ==
"list")
82 {cout << legalSources << endl;
return 0;}
83 if (!inputSourceStr.empty())
87 {cerr <<
"## ERROR: Input source '" << inputSourceStr <<
"' not one of:" << endl << legalSources << endl;
return 1;}
91 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
93 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
97 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
105 if (tcSourceStr ==
"?" || tcSourceStr ==
"list")
106 {cout << legalTCSources << endl;
return 0;}
107 if (!tcSourceStr.empty())
111 {cerr <<
"## ERROR: Timecode source '" << tcSourceStr <<
"' not one of these:" << endl << legalTCSources << endl;
return 1;}
131 {cerr <<
"## ERROR: Initialization failed, status=" << status << endl;
return 4;}
137 cout <<
" Frames Frames" << endl
138 <<
"Processed Dropped" << endl;
141 ULWord framesProcessed, framesDropped;
142 burner.
GetStatus (framesProcessed, framesDropped);
143 cout << setw(9) << framesProcessed
144 << setw(9) << framesDropped
static NTV2InputSource GetInputSourceFromString(const std::string &inStr)
Returns the NTV2InputSource that matches the given string.
void SignalHandler(int inSignal)
#define NTV2_IS_VALID_TIMECODE_INDEX(__x__)
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
bool fSuppressAudio
If true, suppress audio; otherwise include audio.
NTV2TCIndex fTimecodeSource
Timecode source to use.
static bool gGlobalQuit((0))
Header file for the low latency NTV2Burn demonstration class.
int main(int argc, const char **argv)
static NTV2TCIndex GetTCIndexFromString(const std::string &inStr)
Returns the NTV2TCIndex that matches the given string.
static std::string GetTCIndexStrings(const NTV2TCIndexKinds inKinds=TC_INDEXES_ALL, const std::string inDeviceSpecifier=std::string(), const bool inIsInputOnly=true)
NTV2PixelFormat fPixelFormat
The pixel format to use.
static bool IsValidDevice(const std::string &inDeviceSpec)
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
static std::string ToLower(const std::string &inStr)
Returns the given string after converting it to lower case.
static NTV2FrameBufferFormat GetPixelFormatFromString(const std::string &inStr)
Returns the NTV2FrameBufferFormat that matches the given string.
bool fWithHanc
If true, capture & play HANC data, including audio (LLBurn). Defaults to false.
#define NTV2_IS_VALID_INPUT_SOURCE(_inpSrc_)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
virtual AJAStatus Run(void)
Runs me.
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
bool fWithAnc
If true, capture & play anc data (LLBurn). Defaults to false.
static AJAStatus Open(bool incrementRefCount=false)
NTV2InputSource fInputSource
The device input connector to use.
static std::string GetInputSourceStrings(const NTV2IOKinds inKinds=NTV2_IOKINDS_ALL, const std::string inDeviceSpecifier=std::string())
Captures video and audio from a signal provided to an input of an AJA device, burns timecode into the...
@ NTV2_IOKINDS_ALL
Specifies any/all input/output kinds.
virtual void GetStatus(ULWord &outFramesProcessed, ULWord &outFramesDropped)
Provides status information about my input (capture) and output (playout) processes.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDeviceSpecifier=std::string())
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
Configures an NTV2Burn or NTV2FieldBurn instance.