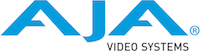 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
12 bool AJALog::bInitialized =
false;
14 #if defined(AJA_WINDOWS)
20 void __cdecl log_odprintf(
const char *format, ...)
22 char buf[4096], *p = buf;
25 va_start(args, format);
26 p += _vsnprintf_s(buf,
sizeof buf - 1, format, args);
29 while ( p > buf && isspace(p[-1]) )
36 ::OutputDebugStringA(buf);
47 if (bInitialized ==
false)
58 #elif defined(AJA_LINUX)
62 #elif defined(AJA_WINDOWS)
111 for (uint64_t i=0; i <sampleSize; i++)
114 average = average / sampleSize;
164 int64_t deltaTime = currTime -
_lastTime;
176 int64_t deltaTime = currTime -
_lastTime;
254 if (delta > threashold)
260 #if defined(AJA_DEBUG) && (AJA_LOGTYPE!=2)
262 AJA_LOG(
"%s = %lld\n",
_tag.c_str(), val);
272 #if defined(AJA_DEBUG) && (AJA_LOGTYPE!=2)
274 AJA_LOG(
"%s-%s = %lld\n",
_tag.c_str(), addedTag, val);
283 #if defined(AJA_DEBUG) && (AJA_LOGTYPE!=2)
285 AJA_LOG(
"%s-%s\n",
_tag.c_str(), str);
void Print(const char *str)
int64_t MarkDeltaAverage()
Declares the AJATime class.
static void Report(int32_t index, int32_t severity, const char *pFileName, int32_t lineNumber,...)
static uint64_t GetSystemMicroseconds(void)
Returns the current value of the host's high-resolution clock, in microseconds.
static bool IsActive(int32_t index)
void PrintValue(int64_t val)
@ AJA_DebugSeverity_Debug
virtual void Resize(uint64_t sampleSize)
void PrintDelta(bool bReset=true)
int32_t GetDelta(bool bReset=true)
virtual void Resize(uint64_t sampleSize)
static AJAStatus Open(bool incrementRefCount=false)
Declares the AJATimeLog class.
std::vector< int64_t > _samples
int64_t MarkAverage(int64_t val)