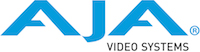 |
AJA NTV2 SDK
17.6.0.1664
NTV2 SDK 17.6.0.1664
|
Go to the documentation of this file.
7 #ifndef NTV2WINDRIVERINTERFACE_H
8 #define NTV2WINDRIVERINTERFACE_H
42 const ULWord inFrameNumber,
44 const ULWord inCardOffsetBytes,
46 const bool inSynchronous =
true);
50 const ULWord inFrameNumber,
52 const ULWord inCardOffsetBytes,
54 const ULWord inNumSegments,
55 const ULWord inSegmentHostPitch,
56 const ULWord inSegmentCardPitch,
57 const bool inSynchronous =
true);
61 const bool inIsTarget,
62 const ULWord inFrameNumber,
63 const ULWord inCardOffsetBytes,
65 const ULWord inNumSegments,
66 const ULWord inSegmentHostPitch,
67 const ULWord inSegmentCardPitch,
87 AJA_VIRTUAL bool RestoreHardwareProcampRegisters (
void);
89 #if !defined(NTV2_DEPRECATE_16_0)
104 #endif // !defined(NTV2_DEPRECATE_16_0)
106 #if !defined(NTV2_NULL_DEVICE)
111 #endif // !defined(NTV2_NULL_DEVICE)
122 #if !defined(NTV2_DEPRECATE_16_0)
125 #endif // !defined(NTV2_DEPRECATE_16_0)
128 #endif // NTV2WINDRIVERINTERFACE_H
Defines the import/export macros for producing DLLs or LIBs.
enum _INTERRUPT_ENUMS_ INTERRUPT_ENUMS
NTV2_DriverDebugMessageSet
Declares device capability functions.
ULWord _previousAudioSelection
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
virtual bool WaitForInterrupt(const INTERRUPT_ENUMS eInterrupt, const ULWord timeOutMs=68)
virtual bool GetInterruptCount(const INTERRUPT_ENUMS eInterrupt, ULWord &outCount)
Answers with the number of interrupts of the given type processed by the driver.
virtual bool WriteRegister(const ULWord inRegNum, const ULWord inValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Updates or replaces all or part of the 32-bit contents of a specific register (real or virtual) on th...
Declares the most fundamental data types used by NTV2. Since Windows NT was the first principal devel...
virtual bool HevcSendMessage(HevcMessageHeader *pMessage)
Sends an HEVC message to the NTV2 driver.
virtual bool ReadRegister(const ULWord inRegNum, ULWord &outValue, const ULWord inMask=0xFFFFFFFF, const ULWord inShift=0)
Reads all or part of the 32-bit contents of a specific register (real or virtual) on the AJA device....
virtual bool ConfigureInterrupt(const bool bEnable, const INTERRUPT_ENUMS eInterruptType)
NTV2_GlobalAudioPlaybackMode
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
virtual bool DmaTransfer(const NTV2DMAEngine inDMAEngine, const bool inIsRead, const ULWord inFrameNumber, ULWord *pFrameBuffer, const ULWord inCardOffsetBytes, const ULWord inTotalByteCount, const bool inSynchronous=(!(0)))
Transfers data between the AJA device and the host. This function will block and not return to the ca...
All new NTV2 structs start with this common header.
Physical device implementations of CNTV2DriverInterface methods through AJA Windows driver.
std::vector< ULWord * > DMA_LOCKED_VEC
Defines & structs shared between user-space and Windows kernel driver.
PSP_DEVICE_INTERFACE_DETAIL_DATA _pspDevIFaceDetailData
DMA_LOCKED_VEC _vecDmaLocked
#define NTV2_SHOULD_BE_DEPRECATED(__f__)
virtual bool ConfigureSubscription(const bool bSubscribe, const INTERRUPT_ENUMS inInterruptType, PULWord &outSubcriptionHdl)
ULWord _previousAudioState
virtual bool ControlDriverDebugMessages(NTV2_DriverDebugMessageSet msgSet, bool enable)
SP_DEVINFO_DATA _spDevInfoData
virtual bool AutoCirculate(AUTOCIRCULATE_DATA &pAutoCircData)
Sends an AutoCirculate command to the NTV2 driver.
I'm the base class that undergirds the platform-specific derived classes (from which CNTV2Card is ult...
Declares the CNTV2DriverInterface base class.
virtual bool CloseLocalPhysical(void)
Releases host resources associated with the local/physical device connection.
virtual bool NTV2Message(NTV2_HEADER *pInMessage)
Sends a message to the NTV2 driver (the new, improved, preferred way).
virtual bool DriverGetBitFileInformation(BITFILE_INFO_STRUCT &outBitFileInfo, const NTV2BitFileType inBitFileType=NTV2_VideoProcBitFile)
Answers with the currently-installed bitfile information.
virtual bool OpenLocalPhysical(const UWord inDeviceIndex)
Opens the local/physical device connection.