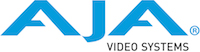 |
AJA NTV2 SDK
17.5.0.1242
NTV2 SDK 17.5.0.1242
|
Go to the documentation of this file.
17 int main (
int argc,
const char ** argv)
24 int channelNumber (1);
25 int doMultiFormat (0);
33 {
"device",
'd',
POPT_ARG_STRING, &pDeviceSpec, 0,
"device to use",
"index#, serial#, or model" },
34 {
"channel",
'c',
POPT_ARG_INT, &channelNumber, 0,
"channel to use",
"1-8" },
36 {
"pattern",
'p',
POPT_ARG_STRING, &pTestPattern, 0,
"test pattern to show",
"0-15, name or '?' to list" },
37 {
"videoFormat",
'v',
POPT_ARG_STRING, &pVideoFormat, 0,
"video format to produce",
"'?' or 'list' to list"},
38 {
"pixelFormat", 0,
POPT_ARG_STRING, &pPixelFormat, 0,
"pixel format to use",
"'?' or 'list' to list"},
39 {
"vanc", 0,
POPT_ARG_STRING, &pVancMode, 0,
"vanc mode",
"off|none|0|on|tall|1|taller|tallest|2"},
45 {cerr <<
"## ERROR: " << popt.
errorStr() << endl;
return 2;}
47 {cout << argv[0] <<
", NTV2 SDK " <<
::NTV2Version() << endl;
return 0;}
50 const string deviceSpec (pDeviceSpec ? pDeviceSpec :
"0");
58 if ((channelNumber < 1) || (channelNumber > 8))
59 {cerr <<
"## ERROR: Invalid channel number " << channelNumber <<
" -- expected 1 thru 8" << endl;
return 1;}
63 const string videoFormatStr (pVideoFormat ? pVideoFormat :
"");
68 if (videoFormatStr ==
"?" || videoFormatStr ==
"list")
71 { cerr <<
"## ERROR: Invalid '--videoFormat' value '" << videoFormatStr <<
"' -- expected values:" << endl
77 const string pixelFormatStr (pPixelFormat ? pPixelFormat :
"");
79 if (pixelFormatStr ==
"?" || pixelFormatStr ==
"list")
83 cerr <<
"## ERROR: Invalid '--pixelFormat' value '" << pixelFormatStr <<
"' -- expected values:" << endl
89 string tpSpec(pTestPattern ? pTestPattern :
"");
91 if (tpSpec ==
"?" || tpSpec ==
"list")
98 cerr <<
"## ERROR: Invalid '--pattern' value '" << tpSpec <<
"' -- expected values:" << endl
105 string vancStr (pVancMode ? pVancMode :
"");
107 if (vancStr ==
"?" || vancStr ==
"list")
109 if (videoFormatStr.empty() && !vancStr.empty())
110 {cerr <<
"## ERROR: '--vanc' option also requires --videoFormat option" << endl;
return 2;}
111 if (!vancStr.empty())
115 { cerr <<
"## ERROR: Invalid '--vanc' value '" << vancStr <<
"' -- expected values: " << endl
125 {cout <<
"## ERROR: Initialization failed: " <<
::AJAStatusToString(status) << endl;
return 1;}
130 {cout <<
"## ERROR: EmitPattern failed: " <<
::AJAStatusToString(status) << endl;
return 2;}
133 cout <<
"## NOTE: Press Enter or Return to exit..." << endl;
static NTV2VideoFormat GetVideoFormatFromString(const std::string &inStr, const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD, const std::string &inDevSpec=std::string())
Returns the NTV2VideoFormat that matches the given string.
static NTV2PixelFormat GetPixelFormatFromString(const std::string &inStr, const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
Returns the NTV2PixelFormat that matches the given string.
static NTV2VANCMode GetVANCModeFromString(const std::string &inStr)
NTV2Channel
These enum values are mostly used to identify a specific widget_framestore. They're also commonly use...
Configures an NTV2OutputTestPattern instance.
int main(int argc, const char **argv)
bool fDoMultiFormat
If true, enable device-sharing; otherwise take exclusive control of device.
NTV2VANCMode fVancMode
VANC mode to use.
AJAStatus EmitPattern(void)
Generates, transfers and displays the test pattern on the output.
static bool IsValidDevice(const std::string &inDeviceSpec)
static std::string GetTestPatternStrings(void)
std::string & lower(std::string &str)
std::string fTestPatternName
Name of the test pattern to use.
static std::string GetPixelFormatStrings(const NTV2PixelFormatKinds inKinds=PIXEL_FORMATS_ALL, const std::string inDevSpec=std::string())
NTV2VideoFormat fVideoFormat
The video format to use.
static std::string GetVANCModeStrings(void)
@ NTV2_FBF_8BIT_YCBCR
See 8-Bit YCbCr Format.
NTV2Channel fOutputChannel
The device channel to use.
virtual const std::string & errorStr(void) const
std::string NTV2Version(const bool inDetailed=false)
static std::string GetVideoFormatStrings(const NTV2VideoFormatKinds inKinds=VIDEO_FORMATS_SDHD, const std::string inDevSpec=std::string())
static std::string GetTestPatternNameFromString(const std::string &inStr)
AJAStatus Init(void)
Initializes me and prepares me to Run.
static AJAStatus Open(bool incrementRefCount=false)
Private include file for all ajabase sources.
I generate and transfer a test pattern into an AJA device's frame buffer for steady-state playout usi...
std::string AJAStatusToString(const AJAStatus inStatus, const bool inDetailed)
NTV2PixelFormat fPixelFormat
The pixel format to use.
#define NTV2_IS_VALID_FRAME_BUFFER_FORMAT(__s__)
#define AJA_FAILURE(_status_)
#define NTV2_IS_VALID_VANCMODE(__v__)