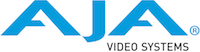 |
AJA NTV2 SDK
17.5.0.1658
NTV2 SDK 17.5.0.1658
|
Go to the documentation of this file.
18 #define OVRLFAIL BURNFAIL
19 #define OVRLWARN BURNWARN
20 #define OVRLDBG BURNDBG
21 #define OVRLNOTE BURNNOTE
22 #define OVRLINFO BURNINFO
36 mInputPixFormat (inConfig.fPixelFormat),
59 while (mPlayThread.
Active())
62 while (mCaptureThread.
Active())
84 cerr <<
"## WARNING: Device '" << mDevice.
GetDescription() <<
"' is not KONA X" << endl;
93 cerr <<
"## ERROR: Cannot acquire '" << mDevice.
GetDescription() <<
"' because another app (pid " << appPID <<
") owns it" << endl;
124 if (mDevice.IsRemote())
125 cerr <<
"Device Description: " << mDevice.
GetDescription() << endl;
128 OVRLINFO(
"Configuration: " << mConfig);
152 const UWord mixerNum (0);
221 mAVCircularBuffer.
Add(&frameData);
230 mAVCircularBuffer.
Clear();
238 for (
ULWord y(yTop); y < (yTop + height); y++)
239 for (
ULWord w(0); w < pixThickness; w++)
248 for (
ULWord v(0); v < vThickness; v++)
249 for (
ULWord x(xLeft); x < (xLeft + width); x++)
250 buf.
U32((yPos + v) * info.
linePitch + x) = argbPixValue;
258 DrawVLine (buf, info, argbPixValue, pixThickness, topLeftX, topLeftY, height);
259 DrawVLine (buf, info, argbPixValue, pixThickness, topLeftX + width - pixThickness, topLeftY, height);
260 DrawHLine (buf, info, argbPixValue, pixThickness, topLeftY, topLeftX, width);
261 DrawHLine (buf, info, argbPixValue, pixThickness, topLeftY + height - pixThickness, topLeftX, width);
267 static const ULWord sOpaqueRed(0xFFFF0000), sOpaqueBlue(0xFF0000FF), sOpaqueGreen(0xFF008000),
268 sOpaqueWhite(0xFFFFFFFF), sOpaqueBlack(0xFF000000);
275 static const vector<ULWord> sColors = {sOpaqueWhite, sOpaqueRed, sOpaqueBlue, sOpaqueGreen};
276 const ULWord hght(256), wdth(256), thickness(wdth/16);
286 for (
size_t n(0); n < sColors.size(); n++)
287 DrawBox (mBug, mBugRasterInfo, sColors.at(n), thickness, n*2*thickness, n*2*thickness, wdth-2*n*2*thickness, hght-2*n*2*thickness);
290 DrawVLine (mBug, mBugRasterInfo, sOpaqueBlack, 1, wdth/2, hght/2-thickness, 2*thickness);
291 DrawHLine (mBug, mBugRasterInfo, sOpaqueBlack, 1, hght/2, wdth/2-thickness, 2*thickness);
299 ULWord numConsecutiveFrames(0), MIN_NUM_CONSECUTIVE_FRAMES(6);
316 if (++loopCount % 500 == 0)
319 else if (numConsecutiveFrames == 0)
322 numConsecutiveFrames++;
324 else if (numConsecutiveFrames == MIN_NUM_CONSECUTIVE_FRAMES)
326 numConsecutiveFrames = 0;
330 numConsecutiveFrames = (lastVF == currVF) ? numConsecutiveFrames + 1 : 0;
390 ULWord goodWaits(0), badWaits(0), goodBlits(0), badBlits(0), goodXfers(0), badXfers(0), pingPong(0);
401 {cerr <<
"## ERROR: Failed to allocate " << rasterInfo.
GetTotalRasterBytes() <<
"-byte vid buffer" << endl;
return;}
407 {cerr <<
"## NOTE: Allocate 2-frame AC" <<
DEC(mConfig.
fOutputChannel+1) <<
" range failed" << endl;
return;}
410 <<
" raster:" << rasterInfo <<
", bug:" << mBugRasterInfo);
412 xPos (rasterInfo.GetRasterWidth() - mBugRasterInfo.GetRasterWidth()),
449 mBugRasterInfo.GetRasterWidth()))
458 pingPong = pingPong ? 0 : 1;
464 if (++loops % 500 == 0)
465 PLDBG(
DEC(thrdNum) <<
": " <<
DEC(goodXfers) <<
" xfers (" <<
DEC(badXfers) <<
" failed), " <<
DEC(goodBlits)
466 <<
" blits (" <<
DEC(badBlits) <<
" failed), " <<
DEC(goodWaits) <<
" waits ("
467 <<
DEC(badWaits) <<
" failed)");
472 PLNOTE(
DEC(thrdNum) <<
" done: " <<
DEC(goodXfers) <<
" xfers (" <<
DEC(badXfers) <<
" failed), "
473 <<
DEC(goodBlits) <<
" blits (" <<
DEC(badBlits) <<
" failed), " <<
DEC(goodWaits) <<
" waits (" <<
DEC(badWaits) <<
" failed)");
488 mCaptureThread.
Start();
514 ULWord loops(0), bails(0), vfChgTally(0), badWaits(0), waitTally(0), goodXfers(0), badXfers(0);
533 { cerr <<
"## NOTE: Failed to [re]allocate host buffers" << endl;
540 { cerr <<
"## NOTE: Input A/C allocate device frame buffer range failed" << endl;
573 if (++loops % 500 == 0)
574 CAPDBG(
DEC(vfChgTally) <<
" sigChgs, " <<
DEC(bails) <<
" bails, " <<
DEC(waitTally)
575 <<
" waits (" <<
DEC(badWaits) <<
" failed), " <<
DEC(goodXfers) <<
" xfers ("
576 <<
DEC(badXfers) <<
" failed)");
591 CAPNOTE(
"Done: " <<
DEC(vfChgTally) <<
" sigChgs, " <<
DEC(bails) <<
" bails, " <<
DEC(waitTally)
592 <<
" waits (" <<
DEC(badWaits) <<
" failed), " <<
DEC(goodXfers) <<
" xfers ("
593 <<
DEC(badXfers) <<
" failed)");
virtual bool SubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Causes me to be notified when an output vertical blanking interrupt is generated for the given output...
virtual bool SetVANCMode(const NTV2VANCMode inVancMode, const NTV2Channel inChannel=NTV2_CHANNEL1)
Sets the VANC mode for the given FrameStore.
virtual bool SetMixerFGMatteEnabled(const UWord inWhichMixer, const bool inIsEnabled)
Answers if the given mixer/keyer's foreground matte is enabled or not.
virtual bool SetMultiFormatMode(const bool inEnable)
Enables or disables multi-format (per channel) device operation. If enabled, each device channel can ...
static bool DrawBox(NTV2Buffer &buf, const NTV2RasterInfo &info, const ULWord argbPixValue, const UWord pixThickness, const UWord topLeftX, const UWord topLeftY, const ULWord width, const ULWord height)
@ NTV2_FBF_ARGB
See 8-Bit ARGB, RGBA, ABGR Formats.
@ NTV2_AUDIO_LOOPBACK_OFF
Embeds silence (zeroes) into the data stream.
AJAStatus SetupVideo(void)
Performs all video setup.
#define NTV2_IS_VALID_TASK_MODE(__m__)
#define NTV2_FOURCC(_a_, _b_, _c_, _d_)
@ NTV2_REFERENCE_INPUT1
Specifies the SDI In 1 connector.
@ NTV2_CHANNEL2
Specifies channel or FrameStore 2 (or the 2nd item).
@ AJA_ThreadPriority_High
virtual bool SetVideoFormat(const NTV2VideoFormat inVideoFormat, const bool inIsAJARetail=(!(0)), const bool inKeepVancSettings=(0), const NTV2Channel inChannel=NTV2_CHANNEL1)
Configures the AJA device to handle a specific video format.
@ NTV2_XptFrameBuffer2RGB
void ReleaseCaptureBuffers(void)
Frees capture buffers & ring.
@ NTV2MIXERMODE_MIX
Overlays foreground video on top of background video.
Declares common types used in the ajabase library.
I encapsulate the video, audio and anc host buffers used in the AutoCirculate demos....
Describes a user-space buffer on the host computer. I have an address and a length,...
LWord acStartFrame
First frame to circulate. FIXFIXFIX Why is this signed? CHANGE TO ULWord??
static const uint32_t kAppSignature(((((uint32_t)( 'O'))<< 24)|(((uint32_t)( 'v'))<< 16)|(((uint32_t)( 'r'))<< 8)|(((uint32_t)( 'l'))<< 0)))
ULWord GetByteCount(void) const
#define NTV2_ASSERT(_expr_)
virtual bool SetMixerCoefficient(const UWord inWhichMixer, const ULWord inMixCoefficient)
Sets the current mix coefficient of the given mixer/keyer.
virtual bool SetMixerBGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the background input control value for the given mixer/keyer.
virtual AJAStatus Attach(AJAThreadFunction *pThreadFunction, void *pUserContext)
virtual bool WaitForInputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
Declares the AJATime class.
static void InputThreadStatic(AJAThread *pThread, void *pInstance)
Static output/playout thread function.
Declares the AJAAncillaryList class.
static bool GetFirstDeviceFromArgument(const std::string &inArgument, CNTV2Card &outDevice)
Rescans the host, and returns an open CNTV2Card instance for the AJA device that matches a command li...
static ULWord gPlayEnterCount(0)
uint32_t U32(const int inIndex) const
bool fDoMultiFormat
If true, enables device-sharing; otherwise takes exclusive control of the device.
@ NTV2_AUDIOSYSTEM_1
This identifies the first Audio System.
FrameDataPtr StartConsumeNextBuffer(void)
The thread that's responsible for processing incoming frames – the consumer – calls this function to ...
virtual bool ClearRouting(void)
Removes all existing signal path connections between any and all widgets on the AJA device.
virtual bool AutoCirculateInitForInput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate ingest, designating a contiguous block of frame buffers on the ...
virtual bool ReleaseStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Releases exclusive use of the AJA device for the given process, permitting other processes to acquire...
virtual bool SetOutputFrame(const NTV2Channel inChannel, const ULWord inValue)
Sets the output frame index number for the given FrameStore. This identifies which frame in device SD...
AJAStatus SetupCaptureBuffers(void)
Allocates capture buffers & ring.
void EndProduceNextBuffer(void)
The producer thread calls this function to signal that it has finished populating the frame it obtain...
Header file for the NTV2Overlay demonstration class.
bool Allocate(const size_t inByteCount, const bool inPageAligned=false)
Allocates (or re-allocates) my user-space storage using the given byte count. I assume full responsib...
static bool DrawHLine(NTV2Buffer &buf, const NTV2RasterInfo &info, const ULWord argbPixValue, const ULWord vThickness, const ULWord yPos, const ULWord xLeft, const ULWord width)
virtual bool SetAudioLoopBack(const NTV2AudioLoopBack inMode, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Enables or disables NTV2AudioLoopBack mode for the given NTV2AudioSystem.
@ NTV2_CHANNEL1
Specifies channel or FrameStore 1 (or the first item).
void RouteOutputSignal(void)
Performs output routing.
NTV2Overlay(const OverlayConfig &inConfig)
Construct from OverlayConfig.
virtual bool SetFrameBufferFormat(NTV2Channel inChannel, NTV2FrameBufferFormat inNewFormat, bool inIsAJARetail=(!(0)), NTV2HDRXferChars inXferChars=NTV2_VPID_TC_SDR_TV, NTV2HDRColorimetry inColorimetry=NTV2_VPID_Color_Rec709, NTV2HDRLuminance inLuminance=NTV2_VPID_Luminance_YCbCr)
Sets the frame buffer format for the given FrameStore on the AJA device.
virtual bool SetSDITransmitEnable(const NTV2Channel inChannel, const bool inEnable)
Sets the specified bidirectional SDI connector to act as an input or an output.
void OutputThread(void)
The output/playout thread function.
NTV2Buffer & VideoBuffer(void)
virtual ~NTV2Overlay()
My destructor.
AJAStatus Run(void)
Runs me (only after Init called)
bool CopyRaster(const NTV2PixelFormat inPixelFormat, UByte *pDstBuffer, const ULWord inDstBytesPerLine, const UWord inDstTotalLines, const UWord inDstVertLineOffset, const UWord inDstHorzPixelOffset, const UByte *pSrcBuffer, const ULWord inSrcBytesPerLine, const UWord inSrcTotalLines, const UWord inSrcVertLineOffset, const UWord inSrcVertLinesToCopy, const UWord inSrcHorzPixelOffset, const UWord inSrcHorzPixelsToCopy)
Copies all or part of a source raster image into a destination raster at a given position.
virtual bool SetMixerBGMatteEnabled(const UWord inWhichMixer, const bool inIsEnabled)
Answers if the given mixer/keyer's background matte is enabled or not.
std::string NTV2InputSourceToString(const NTV2InputSource inValue, const bool inForRetailDisplay=false)
Declares the AJAProcess class.
@ NTV2_XptFrameBuffer1Input
static void Sleep(const int32_t inMilliseconds)
Suspends execution of the current thread for a given number of milliseconds.
AJAStatus Add(FrameDataPtr pInFrameData)
Appends a new frame buffer to me, increasing my frame storage capacity by one frame.
@ NTV2_VANCMODE_OFF
This identifies the mode in which there are no VANC lines in the frame buffer.
virtual bool SetSDIOutputAudioSystem(const NTV2Channel inSDIOutputConnector, const NTV2AudioSystem inAudioSystem)
Sets the device's NTV2AudioSystem that will provide audio for the given SDI output's audio embedder....
virtual std::string GetDescription(void) const
NTV2Buffer fVideoBuffer
Host video buffer.
This is returned from the CNTV2Card::AutoCirculateGetStatus function.
Declares the CNTV2DeviceScanner class.
@ NTV2_XptMixer1FGKeyInput
ULWord VideoBufferSize(void) const
@ DEVICE_ID_KONAX
See KONA X™.
virtual bool GetStreamingApplication(ULWord &outAppType, int32_t &outProcessID)
Answers with the four-CC type and process ID of the application that currently "owns" the AJA device ...
virtual bool SetMode(const NTV2Channel inChannel, const NTV2Mode inNewValue, const bool inIsRetail=(!(0)))
Determines if a given FrameStore on the AJA device will be used to capture or playout video.
@ NTV2MIXERINPUTCONTROL_SHAPED
virtual bool UnsubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Unregisters me so I'm no longer notified when an input VBI is signaled on the given input channel.
static bool DrawVLine(NTV2Buffer &buf, const NTV2RasterInfo &info, const ULWord argbPixValue, const ULWord pixThickness, const ULWord xPos, const ULWord yTop, const ULWord height)
virtual bool UnsubscribeOutputVerticalEvent(const NTV2Channel inChannel)
Unregisters me so I'm no longer notified when an output VBI is signaled on the given output channel.
FrameDataPtr StartProduceNextBuffer(void)
The thread that's responsible for providing frames – the producer – calls this function to populate t...
virtual bool AutoCirculateGetStatus(const NTV2Channel inChannel, AUTOCIRCULATE_STATUS &outStatus)
Returns the current AutoCirculate status for the given channel.
This object specifies the information that will be transferred to or from the AJA device in the CNTV2...
std::string NTV2VideoFormatToString(const NTV2VideoFormat inValue, const bool inUseFrameRate=false)
bool SetVideoBuffer(ULWord *pInVideoBuffer, const ULWord inVideoByteCount)
Sets my video buffer for use in a subsequent call to CNTV2Card::AutoCirculateTransfer.
AJAStatus Init(void)
Prepares me to Run()
virtual bool IsDeviceReady(const bool inCheckValid=(0))
void RouteInputSignal(void)
Performs input routing.
ULWord GetVideoWriteSize(const NTV2VideoFormat inVideoFormat, const NTV2FrameBufferFormat inFBFormat, const NTV2VANCMode inVancMode=NTV2_VANCMODE_OFF)
Identical to the GetVideoActiveSize function, except rounds the result up to the nearest 4K page size...
void EndConsumeNextBuffer(void)
The consumer thread calls this function to signal that it has finished processing the frame it obtain...
virtual NTV2VideoFormat GetInputVideoFormat(const NTV2InputSource inVideoSource=NTV2_INPUTSOURCE_SDI1, const bool inIsProgressive=(0))
Returns the video format of the signal that is present on the given input source.
virtual bool SetEveryFrameServices(const NTV2EveryFrameTaskMode inMode)
Sets the device's task mode.
@ NTV2_DISABLE_TASKS
0: Disabled (never recommended): device configured exclusively by client application(s).
virtual bool SetMixerFGInputControl(const UWord inWhichMixer, const NTV2MixerKeyerInputControl inInputControl)
Sets the foreground input control value for the given mixer/keyer.
NTV2Channel fInputChannel
The input channel to use.
void RouteOverlaySignal(void)
Performs overlay routing.
AJAStatus SetupOverlayBug(void)
Sets up overlay "bug".
std::string fDeviceSpec
The AJA device to use.
AJAStatus SetupAudio(void)
Performs all audio setup.
NTV2InputSource fInputSource
The device input connector to use.
virtual bool Connect(const NTV2InputCrosspointID inInputXpt, const NTV2OutputCrosspointID inOutputXpt, const bool inValidate=(0))
Connects the given widget signal input (sink) to the given widget signal output (source).
@ NTV2_XptMixer1FGVidInput
@ NTV2_FBF_10BIT_YCBCR
See 10-Bit YCbCr Format.
static int32_t Increment(int32_t volatile *pTarget)
@ NTV2_LHIHDMIColorSpaceYCbCr
virtual bool SetHDMIOutAudioSource2Channel(const NTV2AudioChannelPair inNewValue, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_1)
Sets the HDMI output's 2-channel audio source.
@ NTV2_XptMixer1BGVidInput
virtual bool SetMixerVancOutputFromForeground(const UWord inWhichMixer, const bool inFromForegroundSource=(!(0)))
Sets the VANC source for the given mixer/keyer to the foreground video (or not). See the SDI Ancillar...
bool IsRunning(void) const
@ NTV2_MODE_CAPTURE
Capture (input) mode, which writes into device SDRAM.
@ NTV2MIXERINPUTCONTROL_FULLRASTER
void Clear(void)
Clears my frame collection, their locks, everything.
void InputThread(void)
The input/capture thread function.
virtual bool WaitForOutputVerticalInterrupt(const NTV2Channel inChannel=NTV2_CHANNEL1, UWord inRepeatCount=1)
Efficiently sleeps the calling thread/process until the next one or more field (interlaced video) or ...
virtual bool SetMixerMode(const UWord inWhichMixer, const NTV2MixerKeyerMode inMode)
Sets the mode for the given mixer/keyer.
virtual bool AutoCirculateTransfer(const NTV2Channel inChannel, AUTOCIRCULATE_TRANSFER &transferInfo)
Transfers all or part of a frame as specified in the given AUTOCIRCULATE_TRANSFER object to/from the ...
virtual bool AutoCirculateInitForOutput(const NTV2Channel inChannel, const UWord inFrameCount=7, const NTV2AudioSystem inAudioSystem=NTV2_AUDIOSYSTEM_INVALID, const ULWord inOptionFlags=0, const UByte inNumChannels=1, const UWord inStartFrameNumber=0, const UWord inEndFrameNumber=0)
Prepares for subsequent AutoCirculate playout, designating a contiguous block of frame buffers on the...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
void StartInputThread(void)
Starts input thread.
A handy class that makes it easy to "bounce" an unsigned integer value between a minimum and maximum ...
static const size_t CIRCULAR_BUFFER_SIZE(10)
Number of NTV2FrameData's in our ring.
virtual bool AcquireStreamForApplication(ULWord inApplicationType, int32_t inProcessID)
Reserves exclusive use of the AJA device for a given process, preventing other processes on the host ...
static ULWord gPlayExitCount(0)
virtual NTV2DeviceID GetDeviceID(void)
bool HasAvailableInputFrame(void) const
virtual bool GetEveryFrameServices(NTV2EveryFrameTaskMode &outMode)
Retrieves the device's current "retail service" task mode.
static void OutputThreadStatic(AJAThread *pThread, void *pInstance)
void StartOutputThread(void)
Starts output thread.
virtual bool DMAWriteFrame(const ULWord inFrameNumber, const ULWord *pInFrameBuffer, const ULWord inByteCount)
Transfers a single frame from the host to the AJA device.
void SetAbortFlag(const bool *pAbortFlag)
Tells me the boolean variable I should monitor such that when it gets set to "true" will cause any th...
bool Fill(const T &inValue)
Fills me with the given scalar value.
virtual AJAStatus SetPriority(AJAThreadPriority priority)
virtual bool AutoCirculateStart(const NTV2Channel inChannel, const ULWord64 inStartTime=0)
Starts AutoCirculating the specified channel that was previously initialized by CNTV2Card::AutoCircul...
virtual bool SetReference(const NTV2ReferenceSource inRefSource, const bool inKeepFramePulseSelect=(0))
Sets the device's clock reference source. See Video Output Clocking & Synchronization for more inform...
virtual AJAStatus Start()
NTV2VideoFormat WaitForStableInputSignal(void)
Waits for stable input signal.
virtual bool SubscribeInputVerticalEvent(const NTV2Channel inChannel=NTV2_CHANNEL1)
Causes me to be notified when an input vertical blanking interrupt occurs on the given input channel.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
#define AJA_FAILURE(_status_)
@ NTV2_AudioChannel1_2
This selects audio channels 1 and 2 (Group 1 channels 1 and 2)
@ NTV2_XptMixer1BGKeyInput
Configures an NTV2Burn or NTV2FieldBurn instance.
virtual bool EnableChannel(const NTV2Channel inChannel)
Enables the given FrameStore.
@ NTV2_MODE_DISPLAY
Playout (output) mode, which reads from device SDRAM.
Outputs live input video overlaid with image having transparency.
virtual bool AutoCirculateStop(const NTV2Channel inChannel, const bool inAbort=(0))
Stops AutoCirculate for the given channel, and releases the on-device frame buffers that were allocat...
void Quit(void)
Gracefully stops me.
#define NTV2_IS_VALID_VIDEO_FORMAT(__f__)
NTV2Channel fOutputChannel
The output channel to use.
@ NTV2_OEM_TASKS
2: OEM (recommended): device configured by client application(s) with some driver involvement.