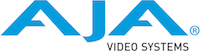 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
10 #define _NTV2CAPTURE_H
46 virtual void Quit (
void);
141 #endif // _NTV2CAPTURE_H
enum NTV2EveryFrameTaskMode NTV2TaskMode
virtual void SetupHostBuffers(void)
Sets up my circular buffers.
virtual void Quit(void)
Gracefully stops me from running.
NTV2DeviceID
Identifies a specific AJA NTV2 device model number. The NTV2DeviceID is actually the PROM part number...
static void ConsumerThreadStatic(AJAThread *pThread, void *pContext)
This is the consumer thread's static callback function that gets called when the consumer thread runs...
virtual bool RouteInputSignal(void)
Sets up device routing for capture.
static void ProducerThreadStatic(AJAThread *pThread, void *pContext)
This is the capture thread's static callback function that gets called when the capture thread runs....
virtual AJAStatus Init(void)
Initializes me and prepares me to Run.
This class is used to configure an NTV2Capture instance.
Instances of me capture frames in real time from a video signal provided to an input of an AJA device...
virtual void ConsumeFrames(void)
Repeatedly consumes frames from the circular buffer (until global quit flag set).
I interrogate and control an AJA video/audio capture/playout device.
std::set< NTV2Channel > NTV2ChannelSet
A set of distinct NTV2Channel values.
std::vector< NTV2FrameData > NTV2FrameDataArray
A vector of NTV2FrameData elements.
virtual void GetACStatus(ULWord &outGoodFrames, ULWord &outDroppedFrames, ULWord &outBufferLevel)
Provides status information about my input (capture) process.
virtual AJAStatus Run(void)
Runs me.
This file contains some structures, constants, classes and functions that are used in some of the dem...
virtual void StartConsumerThread(void)
Starts my frame consumer thread.
virtual void CaptureFrames(void)
Repeatedly captures frames using AutoCirculate (until global quit flag set).
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
virtual AJAStatus SetupVideo(void)
Sets up everything I need for capturing video.
NTV2Capture4K(const CaptureConfig &inConfig)
Constructs me using the given settings.
NTV2AudioSystem
Used to identify an Audio System on an NTV2 device. See Audio System Operation for more information.
virtual AJAStatus SetupAudio(void)
Sets up everything I need for capturing audio.
Declares the AJAThread class.
virtual void StartProducerThread(void)
Starts my capture thread.