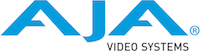 |
AJA NTV2 SDK
17.0.1.1246
NTV2 SDK 17.0.1.1246
|
Go to the documentation of this file.
8 #ifndef NTV2_2022CONFIG_H
9 #define NTV2_2022CONFIG_H
69 std::string sfp1DestIP;
77 std::string sfp2DestIP;
120 eProgSel_Default = eProgSel_AutoFirstProg
165 bool SetNetworkConfiguration(
const eSFP sfp,
const IPVNetConfig & netConfig);
167 bool SetNetworkConfiguration(
const eSFP sfp,
const std::string localIPAddress,
const std::string subnetMask,
const std::string gateway);
168 bool GetNetworkConfiguration(
const eSFP sfp, std::string & localIPAddress, std::string & subnetMask, std::string & gateway);
174 bool SetRxChannelEnable(
const NTV2Channel channel,
bool enable);
175 bool GetRxChannelEnable(
const NTV2Channel channel,
bool & enabled);
180 bool SetTxChannelEnable(
const NTV2Channel channel,
bool enable);
181 bool GetTxChannelEnable(
const NTV2Channel channel,
bool & enabled);
190 bool Set2022_7_Mode(
bool enable, uint32_t rx_networkPathDifferential);
191 bool Get2022_7_Mode(
bool & enable, uint32_t & rx_networkPathDifferential);
201 bool SetIGMPDisable(
eSFP sfp,
bool disable);
202 bool GetIGMPDisable(
eSFP sfp,
bool & disabled);
210 bool GetMACAddress(
eSFP sfp,
NTV2Stream stream, std::string remoteIP, uint32_t & hi, uint32_t & lo);
216 bool SetIPServicesControl(
const bool enable,
const bool forceReconfig);
217 bool GetIPServicesControl(
bool & enable,
bool & forceReconfig);
221 std::string getLastError();
225 void ChannelSemaphoreSet(uint32_t controlReg, uint32_t baseaddr);
226 void ChannelSemaphoreClear(uint32_t controlReg, uint32_t baseaddr);
228 bool SelectRxChannel(
NTV2Channel channel,
eSFP sfp, uint32_t & baseAddr);
229 bool SelectTxChannel(
NTV2Channel channel,
eSFP sfp, uint32_t & baseAddr);
238 uint32_t _numRx0Chans;
239 uint32_t _numRx1Chans;
240 uint32_t _numTx0Chans;
241 uint32_t _numTx1Chans;
242 uint32_t _numRxChans;
243 uint32_t _numTxChans;
247 bool _biDirectionalChannels;
252 #endif // NTV2_2022CONFIG_H
Configures a SMPTE 2022 Transmit Channel.
@ eProgSel_SpecificProgNum
uint32_t sfp1ValidRxPackets
std::vector< uint32_t > availableProgramNumbers
uint32_t mbps
Specifies the mbits per-second for J2K encode.
Declares Transport Stream helper classes.
uint32_t numAvailablePrograms
bool GetBiDirectionalChannels()
NTV2Channel
These enum values are mostly used to identify a specific Frame Store. They're also commonly used to i...
std::string sfp1RemoteIP
Specifies remote (destination) IP address.
@ eProgSel_SpecificProgPID
uint32_t videoPid
Specifies the PID for the video.
uint32_t playoutDelay
Specifies the wait time in milliseconds to SDI playout from incoming packet (0-150).
The CNTV2Config2022 class is the interface to Kona-IP network I/O using SMPTE 2022.
uint32_t sfp2RemotePort
Specifies the remote (destination) port number.
uint32_t ssrc
Specifies the SSRC identifier (if RX_MATCH_2022_SSRC set)
uint32_t sfp1RemotePort
Specifies the remote (destination) port number.
uint16_t sfp1Vlan
Specifies the VLAN TCI (if RX_MATCH_2022_VLAN set)
std::string sfp2RemoteIP
Specifies remote (destination) IP address.
J2KStreamType streamType
Specifies the stream type for J2K encode.
uint32_t audioChannels
Specifies the number of audio channels for J2K encode, a value of 0 indicates no audio.
std::string sfp1SourceIP
Specifies the source (sender) IP address (if RX_MATCH_2022_SOURCE_IP set). If it's in the multiclass ...
uint32_t sfp2LocalPort
Specifies the local (source) port number.
uint32_t sfp1LocalPort
Specifies the local (source) port number.
bool DisableNetworkInterface(eSFP port)
uint32_t bitDepth
Specifies the bit depth for J2K encode.
bool SetIGMPVersion(uint32_t version)
Defines the KonaIP/IoIP S2022 registers.
Declares the CNTV2Card class.
uint32_t numAvailableAudios
uint8_t sfp1RxMatch
Bitmap of rxMatch criteria used.
Enumerations for controlling NTV2 devices.
uint32_t ullMode
Specifies the ull mode for J2K encode.
std::vector< uint32_t > availableAudioPIDs
I interrogate and control an AJA video/audio capture/playout device.
NTV2Stream
Identifies a specific IP-based data stream.
uint32_t sfp1DestPort
Specifies the destination (target) port number (if RX_MATCH_2022_DEST_PORT set)
uint32_t sfp2SourcePort
Specifies the source (sender) port number (if RX_MATCH_2022_SOURCE_PORT set)
uint32_t pmtPid
Specifies the PID for the PMT.
uint32_t sfp2ValidRxPackets
std::string sfp2SourceIP
Specifies the source (sender) IP address (if RX_MATCH_2022_SOURCE_IP set). If it's in the multiclass ...
enum _NTV2VideoFormat NTV2VideoFormat
Identifies a particular video format.
NTV2VideoFormat videoFormat
Specifies the video format for J2K encode.
Declares the CNTV2MBController class.
void SetBiDirectionalChannels(bool bidirectional)
Configures a SMPTE 2022 Receive Channel.
J2KChromaSubSampling chromaSubsamp
Specifies the chroma sub sampling for J2K encode.
uint8_t sfp2RxMatch
Bitmap of rxMatch criteria used.
uint32_t sfp2DestPort
Specifies the destination (target) port number (if RX_MATCH_2022_DEST_PORT set)
std::vector< uint32_t > availableProgramPIDs
uint32_t pcrPid
Specifies the PID for the PCR.
uint32_t audio1Pid
Specifies the PID for audio 1.
uint32_t sfp1SourcePort
Specifies the source (sender) port number (if RX_MATCH_2022_SOURCE_PORT set)
eProgSelMode_t selectionMode
The CNTV2ConfigTs2022 class is the interface to Kona-IP SMPTE 2022 J2K encoder and TS chips.
uint16_t sfp2Vlan
Specifies the VLAN TCI (if RX_MATCH_2022_VLAN set)